Exportable version of WizziLab's modem driver.
========================================================================={{{ Copyright (c) 2013-2016 WizziLab / All rights reserved / =========================================================================}}} More...
Go to the source code of this file.
Typedefs | |
typedef int( | fx_serial_send_t )(u8 type, u8 *buffer2, u8 size2) |
Send concatenation of 2 buffers of given size over serial link. | |
typedef void( | fx_read_t )(u8 action, u8 fid, u32 offset, u32 length, int id) |
Called when ALP-Read is requested by the modem. | |
typedef void( | fx_write_t )(u8 action, u8 fid, void *data, u32 offset, u32 length, int id) |
Called when ALP-Write is requested by the modem. | |
typedef void( | fx_read_fprop_t )(u8 action, u8 fid, int id) |
Called when ALP-Read-file-properties is requested by the modem. | |
typedef void( | fx_flush_t )(u8 action, u8 fid, int id) |
Called when ALP-Flush is requested by the modem. | |
typedef void( | fx_delete_t )(u8 action, u8 fid, int id) |
Called when ALP-Delete is requested by the modem. | |
typedef void( | fx_udata_t )(alp_payload_t *) |
Called when Unsollicited Data is received by the Modem (i.e. | |
typedef void( | fx_reset_t )(void) |
Called when RESET URC is generated by the modem. | |
typedef void( | fx_boot_t )(u8 cause, u16 nb_boot) |
Called when BOOT URC is generated by the modem. | |
typedef void( | action_callback_t )(u8 id) |
Type of function called on response(s) generated by an 'action' function. | |
Functions | |
void | modem_ref_input (u8 flowid, u8 *payload, u8 size) |
Modem Configuration. | |
void | modem_ref_open (fx_serial_send_t *send, modem_ref_callbacks_t *callbacks) |
Open Wizzilab Modem Driver. | |
int | modem_ref_get_id (action_callback_t *cb) |
Request an ID to perform modem operations. | |
int | modem_ref_free_id (u8 id) |
Release an ID. |
Detailed Description
========================================================================={{{ Copyright (c) 2013-2016 WizziLab / All rights reserved / =========================================================================}}}
Wizzilab Modem Reference Driver Implementation Source code should be disclosable and easily portable to any architecture.
Definition in file modem_ref.h.
Typedef Documentation
typedef void( action_callback_t)(u8 id) |
Type of function called on response(s) generated by an 'action' function.
Different functions of this kind can be associated to different IDs through 'get_id'. 'Action' function are subsequently called with relevant ID.
- Parameters:
-
terminal : '1' at the last call for this ID, '0' otherwise err : ALP Error code id : ID of the request
Definition at line 249 of file modem_ref.h.
typedef void( fx_boot_t)(u8 cause, u16 nb_boot) |
Called when BOOT URC is generated by the modem.
- Parameters:
-
cause : Cause of the boot 'H':Pin/Hardware reset 'P':POR reset 'S':Software reset 'L':Low-power reset 'W':Watchdog reset nb_boot : Number of boots since last POR
Definition at line 220 of file modem_ref.h.
typedef void( fx_delete_t)(u8 action, u8 fid, int id) |
Called when ALP-Delete is requested by the modem.
Function must perform required actions to fullfil the request then should call 'respond'
- Parameters:
-
fid : File ID id : ID of the request
Definition at line 188 of file modem_ref.h.
typedef void( fx_flush_t)(u8 action, u8 fid, int id) |
Called when ALP-Flush is requested by the modem.
Function must perform required actions to fullfil the request then should call 'respond'
- Parameters:
-
fid : File ID id : ID of the request
Definition at line 177 of file modem_ref.h.
typedef void( fx_read_fprop_t)(u8 action, u8 fid, int id) |
Called when ALP-Read-file-properties is requested by the modem.
Function must perform required actions to fullfil the request then should call 'respond_fprop' (or 'respond' in case of error)
- Parameters:
-
fid : File ID id : ID of the request
Definition at line 166 of file modem_ref.h.
typedef void( fx_read_t)(u8 action, u8 fid, u32 offset, u32 length, int id) |
Called when ALP-Read is requested by the modem.
Function must perform required actions to fullfil the request then should call 'respond_read' (or 'respond' in case of error)
- Parameters:
-
fid : File ID offset : Access Offset in bytes length : Access Size in bytes id : ID of the request
Definition at line 141 of file modem_ref.h.
typedef void( fx_reset_t)(void) |
Called when RESET URC is generated by the modem.
LDOWN URC is setup by the user through 'modem_ref_enable_urc'
- Parameters:
-
ifid : Interface File ID from which LDOWN is issued
Definition at line 207 of file modem_ref.h.
typedef int( fx_serial_send_t)(u8 type, u8 *buffer2, u8 size2) |
Send concatenation of 2 buffers of given size over serial link.
- Parameters:
-
buffer1 : Pointer to the 1st data buffer to be sent size1 : Size in bytes of the 1st buffer to be sent buffer2 : Pointer to the 2nd data buffer to be sent size2 : Size in bytes of the 2nd buffer to be sent
- Returns:
- number of bytes sent.
- Note:
- either buffers can be of size zero.
- buffer1 is used for WC header
- buffer2 is used actual payload
Definition at line 122 of file modem_ref.h.
typedef void( fx_udata_t)(alp_payload_t *) |
Called when Unsollicited Data is received by the Modem (i.e.
peer notifications etc).
- Parameters:
-
data : Pointer to the data buffer length : Data Size in bytes
Definition at line 198 of file modem_ref.h.
typedef void( fx_write_t)(u8 action, u8 fid, void *data, u32 offset, u32 length, int id) |
Called when ALP-Write is requested by the modem.
Function must perform required actions to fullfil the request then should call 'respond'
- Parameters:
-
fid : File ID data : Pointer to the destination data buffer offset : Access Offset in bytes length : Access Size in bytes id : ID of the request
Definition at line 155 of file modem_ref.h.
Function Documentation
int modem_ref_free_id | ( | u8 | id ) |
Release an ID.
- Parameters:
-
id : ID to release.
- Returns:
- ID value, -1 if ID was not in use.
Definition at line 300 of file modem_ref.cpp.
int modem_ref_get_id | ( | action_callback_t * | cb ) |
Request an ID to perform modem operations.
- Parameters:
-
cb : Function called on responses generated for this ID.
- Returns:
- Positive ID value, -1 if no more IDs available.
Definition at line 278 of file modem_ref.cpp.
void modem_ref_input | ( | u8 | flowid, |
u8 * | payload, | ||
u8 | size | ||
) |
Modem Configuration.
Parse packets and handles calls to relevant callbacks.
- Parameters:
-
flowid : WizziCom FlowID. payload : pointer to payload buffer. size : payload size in bytes.
Definition at line 136 of file modem_ref.cpp.
void modem_ref_open | ( | fx_serial_send_t * | send, |
modem_ref_callbacks_t * | callbacks | ||
) |
Open Wizzilab Modem Driver.
- Parameters:
-
send : User function implementing serial output. callbacks : Set of functions called by the driver upon reception of commands
- Returns:
- 0
Definition at line 311 of file modem_ref.cpp.
Generated on Tue Jul 12 2022 13:52:24 by
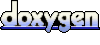