This is WIZnet Ethernet Interface using Hardware TCP/IP chip, W5500, W5200 and W5100. One of them can be selected by enabling it in wiznet.h.
Dependents: Embedded_web EmailButton EmailButton HTTPClient_Weather ... more
W5500.h
00001 /* Copyright (C) 2012 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 * 00018 */ 00019 00020 #pragma once 00021 00022 #include "mbed.h" 00023 #include "mbed_debug.h" 00024 00025 #define TEST_ASSERT(A) while(!(A)){debug("\n\n%s@%d %s ASSERT!\n\n",__PRETTY_FUNCTION__,__LINE__,#A);exit(1);}; 00026 00027 #define DEFAULT_WAIT_RESP_TIMEOUT 500 00028 00029 enum Protocol { 00030 CLOSED = 0, 00031 TCP = 1, 00032 UDP = 2, 00033 }; 00034 00035 enum Command { 00036 OPEN = 0x01, 00037 LISTEN = 0x02, 00038 CONNECT = 0x04, 00039 DISCON = 0x08, 00040 CLOSE = 0x10, 00041 SEND = 0x20, 00042 SEND_MAC = 0x21, 00043 SEND_KEEP = 0x22, 00044 RECV = 0x40, 00045 00046 }; 00047 00048 enum Interrupt { 00049 INT_CON = 0x01, 00050 INT_DISCON = 0x02, 00051 INT_RECV = 0x04, 00052 INT_TIMEOUT = 0x08, 00053 INT_SEND_OK = 0x10, 00054 }; 00055 00056 enum Status { 00057 SOCK_CLOSED = 0x00, 00058 SOCK_INIT = 0x13, 00059 SOCK_LISTEN = 0x14, 00060 SOCK_SYNSENT = 0x15, 00061 SOCK_ESTABLISHED = 0x17, 00062 SOCK_CLOSE_WAIT = 0x1c, 00063 SOCK_UDP = 0x22, 00064 }; 00065 00066 #define MAX_SOCK_NUM 8 00067 00068 #define MR 0x0000 00069 #define GAR 0x0001 00070 #define SUBR 0x0005 00071 #define SHAR 0x0009 00072 #define SIPR 0x000f 00073 #define PHYSTATUS 0x0035 00074 00075 // W5500 socket register 00076 #define Sn_MR 0x0000 00077 #define Sn_CR 0x0001 00078 #define Sn_IR 0x0002 00079 #define Sn_SR 0x0003 00080 #define Sn_PORT 0x0004 00081 #define Sn_DIPR 0x000c 00082 #define Sn_DPORT 0x0010 00083 #define Sn_RXBUF_SIZE 0x001e 00084 #define Sn_TXBUF_SIZE 0x001f 00085 #define Sn_TX_FSR 0x0020 00086 #define Sn_TX_WR 0x0024 00087 #define Sn_RX_RSR 0x0026 00088 #define Sn_RX_RD 0x0028 00089 00090 class WIZnet_Chip { 00091 public: 00092 /* 00093 * Constructor 00094 * 00095 * @param spi spi class 00096 * @param cs cs of the W5500 00097 * @param reset reset pin of the W5500 00098 */ 00099 WIZnet_Chip(PinName mosi, PinName miso, PinName sclk, PinName cs, PinName reset); 00100 WIZnet_Chip(SPI* spi, PinName cs, PinName reset); 00101 00102 /* 00103 * Connect the W5500 to the ssid contained in the constructor. 00104 * 00105 * @return true if connected, false otherwise 00106 */ 00107 bool setip(); 00108 00109 /* 00110 * Disconnect the connection 00111 * 00112 * @ returns true 00113 */ 00114 bool disconnect(); 00115 00116 /* 00117 * Open a tcp connection with the specified host on the specified port 00118 * 00119 * @param host host (can be either an ip address or a name. If a name is provided, a dns request will be established) 00120 * @param port port 00121 * @ returns true if successful 00122 */ 00123 bool connect(int socket, const char * host, int port, int timeout_ms = 10*1000); 00124 00125 /* 00126 * Set the protocol (UDP or TCP) 00127 * 00128 * @param p protocol 00129 * @ returns true if successful 00130 */ 00131 bool setProtocol(int socket, Protocol p); 00132 00133 /* 00134 * Reset the W5500 00135 */ 00136 void reset(); 00137 00138 int wait_readable(int socket, int wait_time_ms, int req_size = 0); 00139 00140 int wait_writeable(int socket, int wait_time_ms, int req_size = 0); 00141 00142 /* 00143 * Check if a tcp link is active 00144 * 00145 * @returns true if successful 00146 */ 00147 bool is_connected(int socket); 00148 00149 /* 00150 * Check if FIN received. 00151 * 00152 * @returns true if successful 00153 */ 00154 bool is_fin_received(int socket); 00155 00156 /* 00157 * Close a tcp connection 00158 * 00159 * @ returns true if successful 00160 */ 00161 bool close(int socket); 00162 00163 /* 00164 * @param str string to be sent 00165 * @param len string length 00166 */ 00167 int send(int socket, const char * str, int len); 00168 00169 int recv(int socket, char* buf, int len); 00170 00171 /* 00172 * Return true if the module is using dhcp 00173 * 00174 * @returns true if the module is using dhcp 00175 */ 00176 bool isDHCP() { 00177 return dhcp; 00178 } 00179 00180 bool gethostbyname(const char* host, uint32_t* ip); 00181 00182 static WIZnet_Chip * getInstance() { 00183 return inst; 00184 }; 00185 00186 int new_socket(); 00187 uint16_t new_port(); 00188 void scmd(int socket, Command cmd); 00189 00190 template<typename T> 00191 void sreg(int socket, uint16_t addr, T data) { 00192 reg_wr<T>(addr, (0x0C + (socket << 5)), data); 00193 } 00194 00195 template<typename T> 00196 T sreg(int socket, uint16_t addr) { 00197 return reg_rd<T>(addr, (0x08 + (socket << 5))); 00198 } 00199 00200 template<typename T> 00201 void reg_wr(uint16_t addr, T data) { 00202 return reg_wr(addr, 0x04, data); 00203 } 00204 00205 template<typename T> 00206 void reg_wr(uint16_t addr, uint8_t cb, T data) { 00207 uint8_t buf[sizeof(T)]; 00208 *reinterpret_cast<T*>(buf) = data; 00209 for(int i = 0; i < sizeof(buf)/2; i++) { // Little Endian to Big Endian 00210 uint8_t t = buf[i]; 00211 buf[i] = buf[sizeof(buf)-1-i]; 00212 buf[sizeof(buf)-1-i] = t; 00213 } 00214 spi_write(addr, cb, buf, sizeof(buf)); 00215 } 00216 00217 template<typename T> 00218 T reg_rd(uint16_t addr) { 00219 return reg_rd<T>(addr, 0x00); 00220 } 00221 00222 template<typename T> 00223 T reg_rd(uint16_t addr, uint8_t cb) { 00224 uint8_t buf[sizeof(T)]; 00225 spi_read(addr, cb, buf, sizeof(buf)); 00226 for(int i = 0; i < sizeof(buf)/2; i++) { // Big Endian to Little Endian 00227 uint8_t t = buf[i]; 00228 buf[i] = buf[sizeof(buf)-1-i]; 00229 buf[sizeof(buf)-1-i] = t; 00230 } 00231 return *reinterpret_cast<T*>(buf); 00232 } 00233 00234 void reg_rd_mac(uint16_t addr, uint8_t* data) { 00235 spi_read(addr, 0x00, data, 6); 00236 } 00237 00238 void reg_wr_ip(uint16_t addr, uint8_t cb, const char* ip) { 00239 uint8_t buf[4]; 00240 char* p = (char*)ip; 00241 for(int i = 0; i < 4; i++) { 00242 buf[i] = atoi(p); 00243 p = strchr(p, '.'); 00244 if (p == NULL) { 00245 break; 00246 } 00247 p++; 00248 } 00249 spi_write(addr, cb, buf, sizeof(buf)); 00250 } 00251 00252 void sreg_ip(int socket, uint16_t addr, const char* ip) { 00253 reg_wr_ip(addr, (0x0C + (socket << 5)), ip); 00254 } 00255 00256 protected: 00257 uint8_t mac[6]; 00258 uint32_t ip; 00259 uint32_t netmask; 00260 uint32_t gateway; 00261 uint32_t dnsaddr; 00262 bool dhcp; 00263 00264 static WIZnet_Chip* inst; 00265 00266 void reg_wr_mac(uint16_t addr, uint8_t* data) { 00267 spi_write(addr, 0x04, data, 6); 00268 } 00269 00270 void spi_write(uint16_t addr, uint8_t cb, const uint8_t *buf, uint16_t len); 00271 void spi_read(uint16_t addr, uint8_t cb, uint8_t *buf, uint16_t len); 00272 SPI* spi; 00273 DigitalOut cs; 00274 DigitalOut reset_pin; 00275 }; 00276 00277 extern uint32_t str_to_ip(const char* str); 00278 extern void printfBytes(char* str, uint8_t* buf, int len); 00279 extern void printHex(uint8_t* buf, int len); 00280 extern void debug_hex(uint8_t* buf, int len);
Generated on Wed Jul 13 2022 02:51:31 by
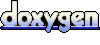