This is WIZnet Ethernet Interface using Hardware TCP/IP chip, W5500, W5200 and W5100. One of them can be selected by enabling it in wiznet.h.
Dependents: Embedded_web EmailButton EmailButton HTTPClient_Weather ... more
W5100.cpp
00001 /* Copyright (C) 2012 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #include "mbed.h" 00020 #include "mbed_debug.h" 00021 #include "wiznet.h" 00022 #include "DNSClient.h" 00023 00024 #ifdef USE_W5100 00025 00026 //Debug is disabled by default 00027 #if 0 00028 #define DBG(...) do{debug("%p %d %s ", this,__LINE__,__PRETTY_FUNCTION__); debug(__VA_ARGS__); } while(0); 00029 //#define DBG(x, ...) debug("[WIZnet_Chip:DBG]"x"\r\n", ##__VA_ARGS__); 00030 #define WARN(x, ...) debug("[WIZnet_Chip:WARN]"x"\r\n", ##__VA_ARGS__); 00031 #define ERR(x, ...) debug("[WIZnet_Chip:ERR]"x"\r\n", ##__VA_ARGS__); 00032 #else 00033 #define DBG(x, ...) 00034 #define WARN(x, ...) 00035 #define ERR(x, ...) 00036 #endif 00037 00038 #if 1 00039 #define INFO(x, ...) debug("[WIZnet_Chip:INFO]"x"\r\n", ##__VA_ARGS__); 00040 #else 00041 #define INFO(x, ...) 00042 #endif 00043 00044 #define DBG_SPI 0 00045 00046 WIZnet_Chip* WIZnet_Chip::inst; 00047 00048 WIZnet_Chip::WIZnet_Chip(PinName mosi, PinName miso, PinName sclk, PinName _cs, PinName _reset): 00049 cs(_cs), reset_pin(_reset) 00050 { 00051 spi = new SPI(mosi, miso, sclk); 00052 00053 spi->format(8,0); 00054 spi->frequency(2000000); 00055 00056 cs = 1; 00057 reset_pin = 1; 00058 inst = this; 00059 } 00060 00061 WIZnet_Chip::WIZnet_Chip(SPI* spi, PinName _cs, PinName _reset): 00062 cs(_cs), reset_pin(_reset) 00063 { 00064 this->spi = spi; 00065 00066 this->spi->format(8,0); 00067 this->spi->frequency(2000000); 00068 00069 cs = 1; 00070 reset_pin = 1; 00071 inst = this; 00072 } 00073 00074 bool WIZnet_Chip::setip() 00075 { 00076 reg_wr<uint32_t>(SIPR, ip); 00077 reg_wr<uint32_t>(GAR, gateway); 00078 reg_wr<uint32_t>(SUBR, netmask); 00079 return true; 00080 } 00081 00082 bool WIZnet_Chip::setProtocol(int socket, Protocol p) 00083 { 00084 if (socket < 0) { 00085 return false; 00086 } 00087 sreg<uint8_t>(socket, Sn_MR, p); 00088 return true; 00089 } 00090 00091 bool WIZnet_Chip::connect(int socket, const char * host, int port, int timeout_ms) 00092 { 00093 if (socket < 0) { 00094 return false; 00095 } 00096 sreg<uint8_t>(socket, Sn_MR, TCP); 00097 scmd(socket, OPEN); 00098 sreg_ip(socket, Sn_DIPR, host); 00099 sreg<uint16_t>(socket, Sn_DPORT, port); 00100 sreg<uint16_t>(socket, Sn_PORT, new_port()); 00101 scmd(socket, CONNECT); 00102 Timer t; 00103 t.reset(); 00104 t.start(); 00105 while(!is_connected(socket)) { 00106 if (t.read_ms() > timeout_ms) { 00107 return false; 00108 } 00109 } 00110 return true; 00111 } 00112 00113 bool WIZnet_Chip::gethostbyname(const char* host, uint32_t* ip) 00114 { 00115 uint32_t addr = str_to_ip(host); 00116 char buf[17]; 00117 snprintf(buf, sizeof(buf), "%d.%d.%d.%d", (addr>>24)&0xff, (addr>>16)&0xff, (addr>>8)&0xff, addr&0xff); 00118 if (strcmp(buf, host) == 0) { 00119 *ip = addr; 00120 return true; 00121 } 00122 DNSClient client; 00123 if(client.lookup(host)) { 00124 *ip = client.ip; 00125 return true; 00126 } 00127 return false; 00128 } 00129 00130 bool WIZnet_Chip::disconnect() 00131 { 00132 return true; 00133 } 00134 00135 bool WIZnet_Chip::is_connected(int socket) 00136 { 00137 if (sreg<uint8_t>(socket, Sn_SR) == SOCK_ESTABLISHED) { 00138 return true; 00139 } 00140 return false; 00141 } 00142 00143 00144 bool WIZnet_Chip::is_fin_received(int socket) 00145 { 00146 uint8_t tmpSn_SR; 00147 tmpSn_SR = sreg<uint8_t>(socket, Sn_SR); 00148 // packet sending is possible, when state is SOCK_CLOSE_WAIT. 00149 if (tmpSn_SR == SOCK_CLOSE_WAIT) { 00150 return true; 00151 } 00152 return false; 00153 } 00154 00155 void WIZnet_Chip::reset() 00156 { 00157 reset_pin = 1; 00158 reset_pin = 0; 00159 wait_us(2); // 2us 00160 reset_pin = 1; 00161 wait_ms(150); // 150ms 00162 00163 reg_wr<uint8_t>(MR, 1<<7); 00164 00165 reg_wr_mac(SHAR, mac); 00166 } 00167 00168 bool WIZnet_Chip::close(int socket) 00169 { 00170 if (socket < 0) { 00171 return false; 00172 } 00173 // if not connected, return 00174 if (sreg<uint8_t>(socket, Sn_SR) == SOCK_CLOSED) { 00175 return true; 00176 } 00177 00178 scmd(socket, CLOSE); 00179 sreg<uint8_t>(socket, Sn_IR, 0xff); 00180 return true; 00181 } 00182 00183 int WIZnet_Chip::wait_readable(int socket, int wait_time_ms, int req_size) 00184 { 00185 if (socket < 0) { 00186 return -1; 00187 } 00188 Timer t; 00189 t.reset(); 00190 t.start(); 00191 while(1) { 00192 int size = 0; int size1 = 0; 00193 do { 00194 size = sreg<uint16_t>(socket, Sn_RX_RSR); 00195 if (size != 0) size1 = sreg<uint16_t>(socket, Sn_RX_RSR); 00196 }while(size != size1); 00197 00198 if (size > req_size) { 00199 return size; 00200 } 00201 if (wait_time_ms != (-1) && t.read_ms() > wait_time_ms) { 00202 break; 00203 } 00204 } 00205 return -1; 00206 } 00207 00208 int WIZnet_Chip::wait_writeable(int socket, int wait_time_ms, int req_size) 00209 { 00210 if (socket < 0) { 00211 return -1; 00212 } 00213 Timer t; 00214 t.reset(); 00215 t.start(); 00216 while(1) { 00217 //int size = sreg<uint16_t>(socket, Sn_TX_FSR); 00218 // during the reading Sn_TX_FSR, it has the possible change of this register. 00219 // so read twice and get same value then use size information. 00220 int size, size2; 00221 do { 00222 size = sreg<uint16_t>(socket, Sn_TX_FSR); 00223 size2 = sreg<uint16_t>(socket, Sn_TX_FSR); 00224 } while (size != size2); 00225 if (size > req_size) { 00226 return size; 00227 } 00228 if (wait_time_ms != (-1) && t.read_ms() > wait_time_ms) { 00229 break; 00230 } 00231 } 00232 return -1; 00233 } 00234 00235 int WIZnet_Chip::send(int socket, const char * str, int len) 00236 { 00237 if (socket < 0) { 00238 return -1; 00239 } 00240 uint16_t base = 0x4000 + socket * 0x800; // each socket has 2K buffer 00241 uint16_t ptr = sreg<uint16_t>(socket, Sn_TX_WR); 00242 uint16_t dst = base + (ptr&(0x800-1)); 00243 if ((dst + len) > (base+0x800)) { 00244 int len2 = base + 0x800 - dst; 00245 spi_write(dst, (uint8_t*)str, len2); 00246 spi_write(base, (uint8_t*)str+len2, len-len2); 00247 } else { 00248 spi_write(dst, (uint8_t*)str, len); 00249 } 00250 sreg<uint16_t>(socket, Sn_TX_WR, ptr + len); 00251 scmd(socket, SEND); 00252 return len; 00253 } 00254 00255 int WIZnet_Chip::recv(int socket, char* buf, int len) 00256 { 00257 if (socket < 0) { 00258 return -1; 00259 } 00260 uint16_t base = 0x6000 + socket * 0x800; // each socket has 2K buffer 00261 uint16_t ptr = sreg<uint16_t>(socket, Sn_RX_RD); 00262 uint16_t src = base + (ptr&(0x800-1)); 00263 if ((src + len) > (base+0x800)) { 00264 int len2 = base + 0x800 - src; 00265 spi_read(src, (uint8_t*)buf, len2); 00266 spi_read(base, (uint8_t*)buf+len2, len-len2); 00267 } else { 00268 spi_read(src, (uint8_t*)buf, len); 00269 } 00270 sreg<uint16_t>(socket, Sn_RX_RD, ptr + len); 00271 scmd(socket, RECV); 00272 return len; 00273 } 00274 00275 int WIZnet_Chip::new_socket() 00276 { 00277 for(int s = 0; s < MAX_SOCK_NUM; s++) { 00278 if (sreg<uint8_t>(s, Sn_SR) == SOCK_CLOSED) { 00279 return s; 00280 } 00281 } 00282 return -1; 00283 } 00284 00285 uint16_t WIZnet_Chip::new_port() 00286 { 00287 uint16_t port = rand(); 00288 port |= 49152; 00289 return port; 00290 } 00291 00292 void WIZnet_Chip::scmd(int socket, Command cmd) 00293 { 00294 sreg<uint8_t>(socket, Sn_CR, cmd); 00295 while(sreg<uint8_t>(socket, Sn_CR)); 00296 } 00297 00298 void WIZnet_Chip::spi_write(uint16_t addr, const uint8_t *buf, uint16_t len) 00299 { 00300 for(int i = 0; i < len; i++) { 00301 cs = 0; 00302 spi->write(0xf0); 00303 spi->write(addr >> 8); 00304 spi->write(addr & 0xff); 00305 addr++; 00306 spi->write(buf[i]); 00307 cs = 1; 00308 } 00309 #if DBG_SPI 00310 debug("[SPI]W %04x(%d)", addr, len); 00311 for(int i = 0; i < len; i++) { 00312 debug(" %02x", buf[i]); 00313 if (i > 16) { 00314 debug(" ..."); 00315 break; 00316 } 00317 } 00318 debug("\r\n"); 00319 #endif 00320 } 00321 00322 void WIZnet_Chip::spi_read(uint16_t addr, uint8_t *buf, uint16_t len) 00323 { 00324 for(int i = 0; i < len; i++) { 00325 cs = 0; 00326 spi->write(0x0f); 00327 spi->write(addr >> 8); 00328 spi->write(addr & 0xff); 00329 addr++; 00330 buf[i] = spi->write(0); 00331 cs = 1; 00332 } 00333 #if DBG_SPI 00334 debug("[SPI]R %04x(%d)", addr, len); 00335 for(int i = 0; i < len; i++) { 00336 debug(" %02x", buf[i]); 00337 if (i > 16) { 00338 debug(" ..."); 00339 break; 00340 } 00341 } 00342 debug("\r\n"); 00343 if ((addr&0xf0ff)==0x4026 || (addr&0xf0ff)==0x4003) { 00344 wait_ms(200); 00345 } 00346 #endif 00347 } 00348 00349 uint32_t str_to_ip(const char* str) 00350 { 00351 uint32_t ip = 0; 00352 char* p = (char*)str; 00353 for(int i = 0; i < 4; i++) { 00354 ip |= atoi(p); 00355 p = strchr(p, '.'); 00356 if (p == NULL) { 00357 break; 00358 } 00359 ip <<= 8; 00360 p++; 00361 } 00362 return ip; 00363 } 00364 00365 void printfBytes(char* str, uint8_t* buf, int len) 00366 { 00367 printf("%s %d:", str, len); 00368 for(int i = 0; i < len; i++) { 00369 printf(" %02x", buf[i]); 00370 } 00371 printf("\n"); 00372 } 00373 00374 void printHex(uint8_t* buf, int len) 00375 { 00376 for(int i = 0; i < len; i++) { 00377 if ((i%16) == 0) { 00378 printf("%p", buf+i); 00379 } 00380 printf(" %02x", buf[i]); 00381 if ((i%16) == 15) { 00382 printf("\n"); 00383 } 00384 } 00385 printf("\n"); 00386 } 00387 00388 void debug_hex(uint8_t* buf, int len) 00389 { 00390 for(int i = 0; i < len; i++) { 00391 if ((i%16) == 0) { 00392 debug("%p", buf+i); 00393 } 00394 debug(" %02x", buf[i]); 00395 if ((i%16) == 15) { 00396 debug("\n"); 00397 } 00398 } 00399 debug("\n"); 00400 } 00401 00402 #endif
Generated on Wed Jul 13 2022 02:51:31 by
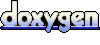