This is WIZnet Ethernet Interface using Hardware TCP/IP chip, W5500, W5200 and W5100. One of them can be selected by enabling it in wiznet.h.
Dependents: Embedded_web EmailButton EmailButton HTTPClient_Weather ... more
UDPSocket.cpp
00001 /* Copyright (C) 2012 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #include "UDPSocket.h" 00020 00021 static int udp_local_port; 00022 00023 UDPSocket::UDPSocket() 00024 { 00025 } 00026 00027 // After init function, bind() should be called. 00028 int UDPSocket::init(void) 00029 { 00030 if (_sock_fd < 0) { 00031 _sock_fd = eth->new_socket(); 00032 } 00033 if (eth->setProtocol(_sock_fd, UDP) == false) return -1; 00034 return 0; 00035 } 00036 00037 // Server initialization 00038 int UDPSocket::bind(int port) 00039 { 00040 if (_sock_fd < 0) { 00041 _sock_fd = eth->new_socket(); 00042 if (_sock_fd < 0) { 00043 return -1; 00044 } 00045 } 00046 // set local port 00047 if (port != 0) { 00048 eth->sreg<uint16_t>(_sock_fd, Sn_PORT, port); 00049 } else { 00050 udp_local_port++; 00051 eth->sreg<uint16_t>(_sock_fd, Sn_PORT, udp_local_port); 00052 } 00053 // set udp protocol 00054 eth->setProtocol(_sock_fd, UDP); 00055 eth->scmd(_sock_fd, OPEN); 00056 return 0; 00057 } 00058 00059 // -1 if unsuccessful, else number of bytes written 00060 int UDPSocket::sendTo(Endpoint &remote, char *packet, int length) 00061 { 00062 int size = eth->wait_writeable(_sock_fd, _blocking ? -1 : _timeout, length-1); 00063 if (size < 0) { 00064 return -1; 00065 } 00066 confEndpoint(remote); 00067 int ret = eth->send(_sock_fd, packet, length); 00068 return ret; 00069 } 00070 00071 // -1 if unsuccessful, else number of bytes received 00072 int UDPSocket::receiveFrom(Endpoint &remote, char *buffer, int length) 00073 { 00074 uint8_t info[8]; 00075 int size = eth->wait_readable(_sock_fd, _blocking ? -1 : _timeout, sizeof(info)); 00076 if (size < 0) { 00077 return -1; 00078 } 00079 eth->recv(_sock_fd, (char*)info, sizeof(info)); 00080 readEndpoint(remote, info); 00081 int udp_size = info[6]<<8|info[7]; 00082 //TEST_ASSERT(udp_size <= (size-sizeof(info))); 00083 if (udp_size > (size-sizeof(info))) { 00084 return -1; 00085 } 00086 00087 /* Perform Length check here to prevent buffer overrun */ 00088 /* fixed by Sean Newton (https://developer.mbed.org/users/SeanNewton/) */ 00089 if (udp_size > length) { 00090 //printf("udp_size: %d\n",udp_size); 00091 return -1; 00092 } 00093 return eth->recv(_sock_fd, buffer, udp_size); 00094 } 00095 00096 void UDPSocket::confEndpoint(Endpoint & ep) 00097 { 00098 char * host = ep.get_address(); 00099 // set remote host 00100 eth->sreg_ip(_sock_fd, Sn_DIPR, host); 00101 // set remote port 00102 eth->sreg<uint16_t>(_sock_fd, Sn_DPORT, ep.get_port()); 00103 } 00104 00105 void UDPSocket::readEndpoint(Endpoint & ep, uint8_t info[]) 00106 { 00107 char addr[17]; 00108 snprintf(addr, sizeof(addr), "%d.%d.%d.%d", info[0], info[1], info[2], info[3]); 00109 uint16_t port = info[4]<<8|info[5]; 00110 ep.set_address(addr, port); 00111 } 00112
Generated on Wed Jul 13 2022 02:51:31 by
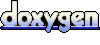