This is WIZnet Ethernet Interface using Hardware TCP/IP chip, W5500, W5200 and W5100. One of them can be selected by enabling it in wiznet.h.
Dependents: Embedded_web EmailButton EmailButton HTTPClient_Weather ... more
TCPSocketConnection.cpp
00001 /* Copyright (C) 2012 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 #include "TCPSocketConnection.h" 00019 #include <cstring> 00020 00021 using std::memset; 00022 using std::memcpy; 00023 00024 // not a big code. 00025 // refer from EthernetInterface by mbed official driver 00026 TCPSocketConnection::TCPSocketConnection() : 00027 _is_connected(false) 00028 { 00029 } 00030 00031 int TCPSocketConnection::connect(const char* host, const int port) 00032 { 00033 if (_sock_fd < 0) { 00034 _sock_fd = eth->new_socket(); 00035 if (_sock_fd < 0) { 00036 return -1; 00037 } 00038 } 00039 if (set_address(host, port) != 0) { 00040 return -1; 00041 } 00042 if (!eth->connect(_sock_fd, get_address(), port)) { 00043 return -1; 00044 } 00045 set_blocking(false); 00046 // add code refer from EthernetInterface. 00047 _is_connected = true; 00048 return 0; 00049 } 00050 00051 bool TCPSocketConnection::is_connected(void) 00052 { 00053 // force update recent state. 00054 _is_connected = eth->is_connected(_sock_fd); 00055 return _is_connected; 00056 } 00057 00058 00059 bool TCPSocketConnection::is_fin_received(void) 00060 { 00061 return eth->is_fin_received(_sock_fd); 00062 } 00063 00064 int TCPSocketConnection::send(char* data, int length) 00065 { 00066 // add to cover exception. 00067 if ((_sock_fd < 0) || !_is_connected) 00068 return -1; 00069 00070 int size = eth->wait_writeable(_sock_fd, _blocking ? -1 : _timeout); 00071 if (size < 0) { 00072 return -1; 00073 } 00074 if (size > length) { 00075 size = length; 00076 } 00077 return eth->send(_sock_fd, data, size); 00078 } 00079 00080 // -1 if unsuccessful, else number of bytes written 00081 int TCPSocketConnection::send_all(char* data, int length) 00082 { 00083 00084 int writtenLen = 0; 00085 while (writtenLen < length) { 00086 int size = eth->wait_writeable(_sock_fd, _blocking ? -1 : _timeout); 00087 if (size < 0) { 00088 return -1; 00089 } 00090 if (size > (length-writtenLen)) { 00091 size = (length-writtenLen); 00092 } 00093 int ret = eth->send(_sock_fd, data + writtenLen, size); 00094 if (ret < 0) { 00095 return -1; 00096 } 00097 writtenLen += ret; 00098 } 00099 return writtenLen; 00100 } 00101 00102 // -1 if unsuccessful, else number of bytes received 00103 int TCPSocketConnection::receive(char* data, int length) 00104 { 00105 // add to cover exception. 00106 if ((_sock_fd < 0) || !_is_connected) 00107 return -1; 00108 00109 int size = eth->wait_readable(_sock_fd, _blocking ? -1 : _timeout); 00110 if (size < 0) { 00111 return -1; 00112 } 00113 if (size > length) { 00114 size = length; 00115 } 00116 return eth->recv(_sock_fd, data, size); 00117 } 00118 00119 // -1 if unsuccessful, else number of bytes received 00120 int TCPSocketConnection::receive_all(char* data, int length) 00121 { 00122 int readLen = 0; 00123 while (readLen < length) { 00124 int size = eth->wait_readable(_sock_fd, _blocking ? -1 :_timeout); 00125 if (size <= 0) { 00126 break; 00127 } 00128 if (size > (length - readLen)) { 00129 size = length - readLen; 00130 } 00131 int ret = eth->recv(_sock_fd, data + readLen, size); 00132 if (ret < 0) { 00133 return -1; 00134 } 00135 readLen += ret; 00136 } 00137 return readLen; 00138 } 00139
Generated on Wed Jul 13 2022 02:51:31 by
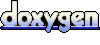