exeption of receive(TCPSocketconnection)
Dependents: FTP_SDCard_File_Client_WIZwiki-W7500 FTPClient_example Freedman DigitalCamera_OV5642_WIZwiki-W7500 ... more
Fork of WIZnetInterface by
W7500x_toe.h
00001 /* Copyright (C) 2012 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 * 00018 */ 00019 #pragma once 00020 00021 #include "mbed.h" 00022 #include "mbed_debug.h" 00023 00024 #define TEST_ASSERT(A) while(!(A)){debug("\n\n%s@%d %s ASSERT!\n\n",__PRETTY_FUNCTION__,__LINE__,#A);exit(1);}; 00025 00026 #define DEFAULT_WAIT_RESP_TIMEOUT 500 00027 00028 00029 #define MAX_SOCK_NUM 8 00030 00031 // Peripheral base address 00032 #define W7500x_WZTOE_BASE (0x46000000) 00033 #define W7500x_TXMEM_BASE (W7500x_WZTOE_BASE + 0x00020000) 00034 #define W7500x_RXMEM_BASE (W7500x_WZTOE_BASE + 0x00030000) 00035 // Common register 00036 #define MR (0x2300) 00037 #define GAR (0x6008) 00038 #define SUBR (0x600C) 00039 #define SHAR (0x6000) 00040 #define SIPR (0x6010) 00041 00042 // Added Common register @W7500 00043 #define TIC100US (0x2000) 00044 00045 // Socket register 00046 #define Sn_MR (0x0000) 00047 #define Sn_CR (0x0010) 00048 #define Sn_IR (0x0020) //--Sn_ISR 00049 #define Sn_SR (0x0030) 00050 #define Sn_PORT (0x0114) 00051 #define Sn_DIPR (0x0124) 00052 #define Sn_DPORT (0x0120) 00053 #define Sn_RXBUF_SIZE (0x0200) 00054 #define Sn_TXBUF_SIZE (0x0220) 00055 #define Sn_TX_FSR (0x0204) 00056 #define Sn_TX_WR (0x020C) 00057 #define Sn_RX_RSR (0x0224) 00058 #define Sn_RX_RD (0x0228) 00059 // added Socket register @W7500 00060 #define Sn_ICR (0x0028) 00061 enum PHYMode { 00062 AutoNegotiate = 0, 00063 HalfDuplex10 = 1, 00064 FullDuplex10 = 2, 00065 HalfDuplex100 = 3, 00066 FullDuplex100 = 4, 00067 }; 00068 00069 //bool plink(int wait_time_ms= 3*1000); 00070 00071 class WIZnet_Chip { 00072 public: 00073 enum Protocol { 00074 CLOSED = 0, 00075 TCP = 1, 00076 UDP = 2, 00077 }; 00078 00079 enum Command { 00080 OPEN = 0x01, 00081 LISTEN = 0x02, 00082 CONNECT = 0x04, 00083 DISCON = 0x08, 00084 CLOSE = 0x10, 00085 SEND = 0x20, 00086 SEND_MAC = 0x21, 00087 SEND_KEEP = 0x22, 00088 RECV = 0x40, 00089 00090 }; 00091 00092 enum Interrupt { 00093 INT_CON = 0x01, 00094 INT_DISCON = 0x02, 00095 INT_RECV = 0x04, 00096 INT_TIMEOUT = 0x08, 00097 INT_SEND_OK = 0x10, 00098 }; 00099 enum Status { 00100 SOCK_CLOSED = 0x00, 00101 SOCK_INIT = 0x13, 00102 SOCK_LISTEN = 0x14, 00103 SOCK_SYNSENT = 0x15, 00104 SOCK_ESTABLISHED = 0x17, 00105 SOCK_CLOSE_WAIT = 0x1c, 00106 SOCK_UDP = 0x22, 00107 }; 00108 enum Mode { 00109 MR_RST = 0x80, 00110 MR_WOL = 0x20, 00111 MR_PB = 0x10, 00112 MR_FARP = 0x02, 00113 }; 00114 00115 WIZnet_Chip(); 00116 00117 /* 00118 * Set MAC Address to W7500x_TOE 00119 * 00120 * @return true if connected, false otherwise 00121 */ 00122 bool setmac(); 00123 00124 /* 00125 * Connect the W7500 WZTOE to the ssid contained in the constructor. 00126 * 00127 * @return true if connected, false otherwise 00128 */ 00129 bool setip(); 00130 00131 00132 /* 00133 * Open a tcp connection with the specified host on the specified port 00134 * 00135 * @param host host (can be either an ip address or a name. If a name is provided, a dns request will be established) 00136 * @param port port 00137 * @ returns true if successful 00138 */ 00139 bool connect(int socket, const char * host, int port, int timeout_ms = 10*1000); 00140 00141 /* 00142 * Set the protocol (UDP or TCP) 00143 * 00144 * @param p protocol 00145 * @ returns true if successful 00146 */ 00147 bool setProtocol(int socket, Protocol p); 00148 00149 /* 00150 * Reset the W7500 WZTOE 00151 */ 00152 void reset(); 00153 00154 int wait_readable(int socket, int wait_time_ms= 3*1000, int req_size = 0); 00155 00156 int wait_writeable(int socket, int wait_time_ms= 3*1000, int req_size = 0); 00157 00158 /* 00159 * Check if an ethernet link is pressent or not. 00160 * 00161 * @returns true if successful 00162 */ 00163 bool link(int wait_time_ms= 3*1000); 00164 00165 /* 00166 * Sets the speed and duplex parameters of an ethernet link. 00167 * 00168 * @returns true if successful 00169 */ 00170 void set_link(PHYMode phymode); 00171 00172 /* 00173 * Check if a tcp link is active 00174 * 00175 * @returns true if successful 00176 */ 00177 bool is_connected(int socket); 00178 00179 /* 00180 * Close a tcp connection 00181 * 00182 * @ returns true if successful 00183 */ 00184 bool close(int socket); 00185 00186 /* 00187 * @param str string to be sent 00188 * @param len string length 00189 */ 00190 int send(int socket, const char * str, int len); 00191 00192 int recv(int socket, char* buf, int len); 00193 00194 /* 00195 * Return true if the module is using dhcp 00196 * 00197 * @returns true if the module is using dhcp 00198 */ 00199 bool isDHCP() { 00200 return dhcp; 00201 } 00202 00203 bool gethostbyname(const char* host, uint32_t* ip); 00204 00205 static WIZnet_Chip * getInstance() { 00206 return inst; 00207 }; 00208 00209 int new_socket(); 00210 uint16_t new_port(); 00211 00212 void scmd(int socket, Command cmd); 00213 00214 template<typename T> 00215 void sreg(int socket, uint16_t addr, T data) { 00216 reg_wr<T>(addr, (uint8_t)(0x01+(socket<<2)), data); 00217 } 00218 00219 template<typename T> 00220 T sreg(int socket, uint16_t addr) { 00221 return reg_rd<T>(addr, (uint8_t)(0x01+(socket<<2))); 00222 } 00223 00224 template<typename T> 00225 void reg_wr(uint16_t addr, T data) { 00226 return reg_wr(addr, 0x00, data); 00227 } 00228 00229 template<typename T> 00230 void reg_wr(uint16_t addr, uint8_t cb, T data) { 00231 uint8_t buf[sizeof(T)]; 00232 *reinterpret_cast<T*>(buf) = data; 00233 /* 00234 for(int i = 0; i < sizeof(buf)/2; i++) { // Little Endian to Big Endian 00235 uint8_t t = buf[i]; 00236 buf[i] = buf[sizeof(buf)-1-i]; 00237 buf[sizeof(buf)-1-i] = t; 00238 } 00239 */ 00240 for(int i = 0; i < sizeof(buf); i++) { // Little Endian to Big Endian 00241 *(volatile uint8_t *)(W7500x_WZTOE_BASE + (uint32_t)((cb<<16)+addr)+i) = buf[i]; 00242 } 00243 } 00244 00245 template<typename T> 00246 T reg_rd(uint16_t addr) { 00247 return reg_rd<T>(addr, (uint8_t)(0x00)); 00248 } 00249 00250 template<typename T> 00251 T reg_rd(uint16_t addr, uint8_t cb) { 00252 uint8_t buf[sizeof(T)] = {0,}; 00253 for(int i = 0; i < sizeof(buf); i++) { // Little Endian to Big Endian 00254 buf[i] = *(volatile uint8_t *)(W7500x_WZTOE_BASE + (uint32_t)((cb<<16)+addr)+i); 00255 } 00256 /* 00257 for(int i = 0; i < sizeof(buf)/2; i++) { // Big Endian to Little Endian 00258 uint8_t t = buf[i]; 00259 buf[i] = buf[sizeof(buf)-1-i]; 00260 buf[sizeof(buf)-1-i] = t; 00261 } 00262 */ 00263 return *reinterpret_cast<T*>(buf); 00264 } 00265 00266 void reg_rd_mac(uint16_t addr, uint8_t* data); 00267 00268 void reg_wr_ip(uint16_t addr, uint8_t cb, const char* ip); 00269 00270 void sreg_ip(int socket, uint16_t addr, const char* ip); 00271 00272 int ethernet_link(void); 00273 00274 void ethernet_set_link(int speed, int duplex); 00275 00276 00277 protected: 00278 uint8_t mac[6]; 00279 uint32_t ip; 00280 uint32_t netmask; 00281 uint32_t gateway; 00282 uint32_t dnsaddr; 00283 bool dhcp; 00284 00285 static WIZnet_Chip* inst; 00286 00287 void reg_wr_mac(uint16_t addr, uint8_t* data) { 00288 *(volatile uint8_t *)(W7500x_WZTOE_BASE + (uint32_t)(addr+3)) = data[0] ; 00289 *(volatile uint8_t *)(W7500x_WZTOE_BASE + (uint32_t)(addr+2)) = data[1] ; 00290 *(volatile uint8_t *)(W7500x_WZTOE_BASE + (uint32_t)(addr+1)) = data[2] ; 00291 *(volatile uint8_t *)(W7500x_WZTOE_BASE + (uint32_t)(addr+0)) = data[3] ; 00292 *(volatile uint8_t *)(W7500x_WZTOE_BASE + (uint32_t)(addr+7)) = data[4] ; 00293 *(volatile uint8_t *)(W7500x_WZTOE_BASE + (uint32_t)(addr+6)) = data[5] ; 00294 } 00295 }; 00296 00297 extern uint32_t str_to_ip(const char* str); 00298 extern void printfBytes(char* str, uint8_t* buf, int len); 00299 extern void printHex(uint8_t* buf, int len); 00300 extern void debug_hex(uint8_t* buf, int len);
Generated on Thu Jul 14 2022 05:42:13 by
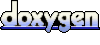