exeption of receive(TCPSocketconnection)
Dependents: FTP_SDCard_File_Client_WIZwiki-W7500 FTPClient_example Freedman DigitalCamera_OV5642_WIZwiki-W7500 ... more
Fork of WIZnetInterface by
TCPSocketConnection.cpp
00001 /* Copyright (C) 2012 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #include "TCPSocketConnection.h" 00020 #include <cstring> 00021 00022 using std::memset; 00023 using std::memcpy; 00024 00025 // not a big code. 00026 // refer from EthernetInterface by mbed official driver 00027 TCPSocketConnection::TCPSocketConnection() : 00028 _is_connected(false) 00029 { 00030 } 00031 00032 int TCPSocketConnection::connect(const char* host, const int port) 00033 { 00034 if (_sock_fd < 0) { 00035 _sock_fd = eth->new_socket(); 00036 if (_sock_fd < 0) { 00037 return -1; 00038 } 00039 } 00040 if (set_address(host, port) != 0) { 00041 return -1; 00042 } 00043 if (!eth->connect(_sock_fd, get_address(), port)) { 00044 return -1; 00045 } 00046 set_blocking(false); 00047 // add code refer from EthernetInterface. 00048 _is_connected = true; 00049 00050 return 0; 00051 } 00052 00053 bool TCPSocketConnection::is_connected(void) 00054 { 00055 // force update recent state. 00056 _is_connected = eth->is_connected(_sock_fd); 00057 return _is_connected; 00058 } 00059 00060 int TCPSocketConnection::send(char* data, int length) 00061 { 00062 if((_sock_fd<0) || !(eth->is_connected(_sock_fd))) 00063 return -1; 00064 00065 int size = eth->wait_writeable(_sock_fd, _blocking ? -1 : _timeout); 00066 if (size < 0) 00067 return -1; 00068 00069 if (size > length) 00070 size = length; 00071 00072 return eth->send(_sock_fd, data, size); 00073 } 00074 00075 // -1 if unsuccessful, else number of bytes written 00076 int TCPSocketConnection::send_all(char* data, int length) 00077 { 00078 int writtenLen = 0; 00079 00080 if(_sock_fd<0) 00081 return -1; 00082 00083 while (writtenLen < length) { 00084 00085 if(!(eth->is_connected(_sock_fd))) 00086 return -1; 00087 00088 int size = eth->wait_writeable(_sock_fd, _blocking ? -1 : _timeout); 00089 if (size < 0) { 00090 return -1; 00091 } 00092 if (size > (length-writtenLen)) { 00093 size = (length-writtenLen); 00094 } 00095 int ret = eth->send(_sock_fd, data + writtenLen, size); 00096 if (ret < 0) { 00097 return -1; 00098 } 00099 writtenLen += ret; 00100 } 00101 return writtenLen; 00102 } 00103 00104 // -1 if unsuccessful, else number of bytes received 00105 int TCPSocketConnection::receive(char* data, int length) 00106 { 00107 if((_sock_fd<0) || !(eth->is_connected(_sock_fd))) 00108 return -1; 00109 00110 int size = eth->wait_readable(_sock_fd, _blocking ? -1 : _timeout); 00111 //int size = eth->wait_readable(_sock_fd, _blocking ? _timeout : -1 ); 00112 if (size < 0) { 00113 return -1; 00114 } 00115 00116 if (size > length) { 00117 size = length; 00118 } 00119 return eth->recv(_sock_fd, data, size); 00120 } 00121 00122 // -1 if unsuccessful, else number of bytes received 00123 int TCPSocketConnection::receive_all(char* data, int length) 00124 { 00125 if(_sock_fd<0) 00126 return -1; 00127 00128 int readLen = 0; 00129 while (readLen < length) { 00130 00131 if(!(eth->is_connected(_sock_fd))) 00132 return -1; 00133 00134 int size = eth->wait_readable(_sock_fd, _blocking ? -1 :_timeout); 00135 if (size <= 0) { 00136 break; 00137 } 00138 if (size > (length - readLen)) { 00139 size = length - readLen; 00140 } 00141 int ret = eth->recv(_sock_fd, data + readLen, size); 00142 if (ret < 0) { 00143 return -1; 00144 } 00145 readLen += ret; 00146 } 00147 return readLen; 00148 }
Generated on Thu Jul 14 2022 05:42:13 by
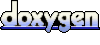