exeption of receive(TCPSocketconnection)
Dependents: FTP_SDCard_File_Client_WIZwiki-W7500 FTPClient_example Freedman DigitalCamera_OV5642_WIZwiki-W7500 ... more
Fork of WIZnetInterface by
EthernetInterface.h
00001 /* Copyright (C) 2012 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #pragma once 00020 #include "eth_arch.h" 00021 /** Interface using Wiznet chip to connect to an IP-based network 00022 * 00023 */ 00024 class EthernetInterface: public WIZnet_Chip { 00025 public: 00026 00027 #if not defined(TARGET_WIZwiki_W7500) 00028 /** 00029 * Constructor 00030 * 00031 * \param mosi mbed pin to use for SPI 00032 * \param miso mbed pin to use for SPI 00033 * \param sclk mbed pin to use for SPI 00034 * \param cs chip select of the WIZnet_Chip 00035 * \param reset reset pin of the WIZnet_Chip 00036 */ 00037 EthernetInterface(PinName mosi, PinName miso, PinName sclk, PinName cs, PinName reset); 00038 EthernetInterface(SPI* spi, PinName cs, PinName reset); 00039 #endif 00040 00041 /** Initialize the interface with DHCP. 00042 * Initialize the interface and configure it to use DHCP (no connection at this point). 00043 * \return 0 on success, a negative number on failure 00044 */ 00045 int init(uint8_t * mac); //With DHCP 00046 00047 /** Initialize the interface with a static IP address. 00048 * Initialize the interface and configure it with the following static configuration (no connection at this point). 00049 * \param ip the IP address to use 00050 * \param mask the IP address mask 00051 * \param gateway the gateway to use 00052 * \return 0 on success, a negative number on failure 00053 */ 00054 int init(uint8_t * mac, const char* ip, const char* mask, const char* gateway); 00055 00056 /** Connect 00057 * Bring the interface up, start DHCP if needed. 00058 * \return 0 on success, a negative number on failure 00059 */ 00060 int connect(); 00061 00062 /** Disconnect 00063 * Bring the interface down 00064 * \return 0 on success, a negative number on failure 00065 */ 00066 int disconnect(); 00067 00068 /** Get IP address & MAC address 00069 * 00070 * @ returns ip address 00071 */ 00072 char* getIPAddress(); 00073 char* getNetworkMask(); 00074 char* getGateway(); 00075 char* getMACAddress(); 00076 00077 int IPrenew(int timeout_ms = 15*1000); 00078 00079 private: 00080 char ip_string[20]; 00081 char mask_string[20]; 00082 char gw_string[20]; 00083 char mac_string[20]; 00084 bool ip_set; 00085 }; 00086 00087 #include "TCPSocketConnection.h" 00088 #include "TCPSocketServer.h" 00089 #include "UDPSocket.h"
Generated on Thu Jul 14 2022 05:42:13 by
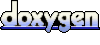