exeption of receive(TCPSocketconnection)
Dependents: FTP_SDCard_File_Client_WIZwiki-W7500 FTPClient_example Freedman DigitalCamera_OV5642_WIZwiki-W7500 ... more
Fork of WIZnetInterface by
EthernetInterface.cpp
00001 /* Copyright (C) 2012 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #include "EthernetInterface.h" 00020 #include "DHCPClient.h" 00021 00022 #if not defined(TARGET_WIZwiki_W7500) 00023 EthernetInterface::EthernetInterface(PinName mosi, PinName miso, PinName sclk, PinName cs, PinName reset) : 00024 WIZnet_Chip(mosi, miso, sclk, cs, reset) 00025 { 00026 ip_set = false; 00027 } 00028 00029 EthernetInterface::EthernetInterface(SPI* spi, PinName cs, PinName reset) : 00030 WIZnet_Chip(spi, cs, reset) 00031 { 00032 ip_set = false; 00033 } 00034 #endif 00035 00036 int EthernetInterface::init(uint8_t * mac) 00037 { 00038 dhcp = true; 00039 // 00040 for (int i =0; i < 6; i++) this->mac[i] = mac[i]; 00041 // 00042 reset(); 00043 00044 return 0; 00045 } 00046 00047 int EthernetInterface::init(uint8_t * mac, const char* ip, const char* mask, const char* gateway) 00048 { 00049 dhcp = false; 00050 // 00051 for (int i =0; i < 6; i++) this->mac[i] = mac[i]; 00052 // 00053 this->ip = str_to_ip(ip); 00054 strcpy(ip_string, ip); 00055 ip_set = true; 00056 this->netmask = str_to_ip(mask); 00057 this->gateway = str_to_ip(gateway); 00058 reset(); 00059 00060 // @Jul. 8. 2014 add code. should be called to write chip. 00061 setmac(); 00062 setip(); 00063 00064 return 0; 00065 } 00066 00067 // Connect Bring the interface up, start DHCP if needed. 00068 int EthernetInterface::connect() 00069 { 00070 if (dhcp) { 00071 int r = IPrenew(); 00072 if (r < 0) { 00073 return r; 00074 } 00075 } 00076 00077 if (WIZnet_Chip::setip() == false) return -1; 00078 return 0; 00079 } 00080 00081 // Disconnect Bring the interface down. 00082 int EthernetInterface::disconnect() 00083 { 00084 //if (WIZnet_Chip::disconnect() == false) return -1; 00085 return 0; 00086 } 00087 00088 00089 char* EthernetInterface::getIPAddress() 00090 { 00091 uint32_t ip = reg_rd<uint32_t>(SIPR); 00092 snprintf(ip_string, sizeof(ip_string), "%d.%d.%d.%d", 00093 (uint8_t)((ip>>24)&0xff), 00094 (uint8_t)((ip>>16)&0xff), 00095 (uint8_t)((ip>>8)&0xff), 00096 (uint8_t)(ip&0xff)); 00097 return ip_string; 00098 } 00099 00100 char* EthernetInterface::getNetworkMask() 00101 { 00102 uint32_t ip = reg_rd<uint32_t>(SUBR); 00103 snprintf(mask_string, sizeof(mask_string), "%d.%d.%d.%d", 00104 (uint8_t)((ip>>24)&0xff), 00105 (uint8_t)((ip>>16)&0xff), 00106 (uint8_t)((ip>>8)&0xff), 00107 (uint8_t)(ip&0xff)); 00108 return mask_string; 00109 } 00110 00111 char* EthernetInterface::getGateway() 00112 { 00113 uint32_t ip = reg_rd<uint32_t>(GAR); 00114 snprintf(gw_string, sizeof(gw_string), "%d.%d.%d.%d", 00115 (uint8_t)((ip>>24)&0xff), 00116 (uint8_t)((ip>>16)&0xff), 00117 (uint8_t)((ip>>8)&0xff), 00118 (uint8_t)(ip&0xff)); 00119 return gw_string; 00120 } 00121 00122 char* EthernetInterface::getMACAddress() 00123 { 00124 uint8_t mac[6]; 00125 reg_rd_mac(SHAR, mac); 00126 snprintf(mac_string, sizeof(mac_string), "%02X:%02X:%02X:%02X:%02X:%02X", mac[0], mac[1], mac[2], mac[3], mac[4], mac[5]); 00127 //ethernet_address(mac_string); 00128 return mac_string; 00129 00130 } 00131 00132 int EthernetInterface::IPrenew(int timeout_ms) 00133 { 00134 DHCPClient dhcp; 00135 int err = dhcp.setup(timeout_ms); 00136 if (err == (-1)) { 00137 return -1; 00138 } 00139 // printf("Connected, IP: %d.%d.%d.%d\n", dhcp.yiaddr[0], dhcp.yiaddr[1], dhcp.yiaddr[2], dhcp.yiaddr[3]); 00140 ip = (dhcp.yiaddr[0] <<24) | (dhcp.yiaddr[1] <<16) | (dhcp.yiaddr[2] <<8) | dhcp.yiaddr[3]; 00141 gateway = (dhcp.gateway[0]<<24) | (dhcp.gateway[1]<<16) | (dhcp.gateway[2]<<8) | dhcp.gateway[3]; 00142 netmask = (dhcp.netmask[0]<<24) | (dhcp.netmask[1]<<16) | (dhcp.netmask[2]<<8) | dhcp.netmask[3]; 00143 dnsaddr = (dhcp.dnsaddr[0]<<24) | (dhcp.dnsaddr[1]<<16) | (dhcp.dnsaddr[2]<<8) | dhcp.dnsaddr[3]; 00144 return 0; 00145 } 00146
Generated on Thu Jul 14 2022 05:42:13 by
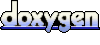