Add function of delete all ID.
Fork of GT511C3 by
Embed:
(wiki syntax)
Show/hide line numbers
GT511C3.cpp
00001 /** 00002 * @section LICENSE 00003 * 00004 * Copyright (c) 2013 @tosihisa, MIT License 00005 * 00006 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00007 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00008 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00009 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00010 * furnished to do so, subject to the following conditions: 00011 * 00012 * The above copyright notice and this permission notice shall be included in all copies or 00013 * substantial portions of the Software. 00014 * 00015 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00016 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00017 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00018 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00020 * 00021 * @section DESCRIPTION 00022 * 00023 * Fingerprint reader module "GT-511C3" class. 00024 * 00025 * http://www.adh-tech.com.tw/?22,gt-511c3-gt-511c31 00026 * http://www.adh-tech.com.tw/files/GT-511C3_datasheet_V1%201_20131127[1].pdf 00027 * https://www.sparkfun.com/products/11792 00028 * https://github.com/sparkfun/Fingerprint_Scanner-TTL/ 00029 */ 00030 00031 #include "mbed.h" 00032 #include "GT511C3.hpp" 00033 00034 #define SET_AND_SUMADD(idx,val) sendbuf[idx]=((unsigned char)(val));sum += sendbuf[idx] 00035 00036 int GT511C3::Init(void) 00037 { 00038 baud(9600); 00039 ClearLine(); 00040 return 0; 00041 } 00042 00043 int GT511C3::SendCommand(unsigned long Parameter,unsigned short Command) 00044 { 00045 unsigned char sendbuf[12]; 00046 unsigned short sum = 0; 00047 int idx = 0; 00048 int i; 00049 00050 SET_AND_SUMADD(idx,0x55); idx++; 00051 SET_AND_SUMADD(idx,0xAA); idx++; 00052 SET_AND_SUMADD(idx,0x01); idx++; 00053 SET_AND_SUMADD(idx,0x00); idx++; 00054 SET_AND_SUMADD(idx,Parameter & 0xff); idx++; 00055 SET_AND_SUMADD(idx,(Parameter >> 8) & 0xff); idx++; 00056 SET_AND_SUMADD(idx,(Parameter >> 16) & 0xff); idx++; 00057 SET_AND_SUMADD(idx,(Parameter >> 24) & 0xff); idx++; 00058 SET_AND_SUMADD(idx,Command & 0xff); idx++; 00059 SET_AND_SUMADD(idx,(Command >> 8) & 0xff); idx++; 00060 sendbuf[idx] = sum & 0xff; idx++; 00061 sendbuf[idx] = (sum >> 8) & 0xff; idx++; 00062 00063 for(i = 0;i < idx;i++){ 00064 while(!writeable()); 00065 putc(sendbuf[i]); 00066 } 00067 return 0; 00068 } 00069 00070 int GT511C3::RecvResponse(unsigned long *Parameter,unsigned short *Response) 00071 { 00072 const unsigned char fixedbuf[4] = { 0x55,0xAA,0x01,0x00 }; 00073 unsigned char buf[12]; 00074 unsigned short sum = 0; 00075 int i; 00076 00077 *Parameter = 0; 00078 *Response = CMD_Nack; 00079 00080 for(i = 0;i < sizeof(buf);i++){ 00081 while(!readable()); 00082 buf[i] = getc(); 00083 if(i < 9){ 00084 sum += buf[i]; 00085 } 00086 if(i < 4){ 00087 if(buf[i] != fixedbuf[i]){ 00088 return -1; 00089 } 00090 } 00091 } 00092 if(buf[10] != (sum & 0xff)) 00093 return -2; 00094 if(buf[11] != ((sum >> 8) & 0xff)) 00095 return -2; 00096 00097 *Parameter = buf[7]; 00098 *Parameter = (*Parameter << 8) | buf[6]; 00099 *Parameter = (*Parameter << 8) | buf[5]; 00100 *Parameter = (*Parameter << 8) | buf[4]; 00101 00102 *Response = buf[9]; 00103 *Response = (*Response << 8) | buf[8]; 00104 00105 return 0; 00106 } 00107 00108 int GT511C3::RecvData(unsigned char *data,unsigned long size) 00109 { 00110 const unsigned char fixedbuf[4] = { 0x5A,0xA5,0x01,0x00 }; 00111 unsigned short sum = 0; 00112 int i; 00113 00114 for(i = 0;i < size;i++){ 00115 while(!readable()); 00116 *(data + i) = getc(); 00117 if(i < (size-2)){ 00118 sum += *(data + i); 00119 } 00120 if(i < 4){ 00121 if(*(data + i) != fixedbuf[i]){ 00122 return -1; 00123 } 00124 } 00125 } 00126 if(*(data + size - 2) != (sum & 0xff)) 00127 return -2; 00128 if(*(data + size - 1) != ((sum >> 8) & 0xff)) 00129 return -2; 00130 return 0; 00131 } 00132 00133 int GT511C3::SendRecv(unsigned short Command,unsigned long *Parameter,unsigned short *Response) 00134 { 00135 int sts; 00136 if((sts = SendCommand(*Parameter,Command)) == 0){ 00137 *Parameter = 0; 00138 if((sts = RecvResponse(Parameter,Response)) != 0){ 00139 *Response = CMD_Nack; 00140 *Parameter = NACK_IO_ERR; 00141 } 00142 } 00143 if(*Response == CMD_Nack){ 00144 LastError = *Parameter; 00145 } 00146 return sts; 00147 } 00148 00149 int GT511C3::ClearLine(void) 00150 { 00151 while(readable()){ 00152 (void)getc(); 00153 } 00154 return 0; 00155 } 00156 00157 int GT511C3::Open(void) 00158 { 00159 unsigned long Parameter = 1; 00160 unsigned short Response = 0; 00161 unsigned char buf[4+sizeof(FirmwareVersion)+sizeof(IsoAreaMaxSize)+sizeof(DeviceSerialNumber)+2]; 00162 int sts = 0; 00163 00164 if((sts = Init()) != 0) 00165 return -1; 00166 00167 sts = SendRecv(CMD_Open,&Parameter,&Response); 00168 if((sts != 0) || (Response != CMD_Ack)){ 00169 return -1; 00170 } 00171 if((sts = RecvData(buf,sizeof(buf))) == 0){ 00172 memcpy(&FirmwareVersion,&buf[4+0],sizeof(FirmwareVersion)); 00173 memcpy(&IsoAreaMaxSize,&buf[4+sizeof(FirmwareVersion)],sizeof(IsoAreaMaxSize)); 00174 memcpy(DeviceSerialNumber,&buf[4+sizeof(FirmwareVersion)+sizeof(IsoAreaMaxSize)],sizeof(DeviceSerialNumber)); 00175 } 00176 return sts; 00177 } 00178 00179 int GT511C3::WaitPress(int press) 00180 { 00181 while(IsPress() != press); 00182 return 0; 00183 } 00184 00185 int GT511C3::CmosLed(int onoff) 00186 { 00187 unsigned long Parameter = onoff & 1; 00188 unsigned short Response = 0; 00189 int sts = 0; 00190 00191 sts = SendRecv(CMD_CmosLed,&Parameter,&Response); 00192 if((sts != 0) || (Response != CMD_Ack)){ 00193 return -1; 00194 } 00195 return 0; 00196 } 00197 00198 int GT511C3::IsPress(void) 00199 { 00200 unsigned long Parameter = 0; 00201 unsigned short Response = 0; 00202 int sts = 0; 00203 sts = SendRecv(CMD_IsPressFinger,&Parameter,&Response); 00204 if((sts != 0) || (Response != CMD_Ack)) 00205 return 0; 00206 if(Parameter != 0) 00207 return 0; 00208 return 1; 00209 } 00210 00211 int GT511C3::Capture(int best) 00212 { 00213 unsigned long Parameter = best; 00214 unsigned short Response = 0; 00215 int sts = 0; 00216 00217 sts = SendRecv(CMD_CaptureFinger,&Parameter,&Response); 00218 if((sts != 0) || (Response != CMD_Ack)) 00219 return -1; 00220 return 0; 00221 } 00222 00223 int GT511C3::Enroll_N(int N) 00224 { 00225 unsigned long Parameter = 0; 00226 unsigned short Response = 0; 00227 int sts = 0; 00228 enum Command cmd; 00229 00230 switch(N){ 00231 default: 00232 case 1: cmd = CMD_Enroll1; break; 00233 case 2: cmd = CMD_Enroll2; break; 00234 case 3: cmd = CMD_Enroll3; break; 00235 } 00236 sts = SendRecv(cmd,&Parameter,&Response); 00237 if((sts != 0) || (Response != CMD_Ack)) 00238 return -1; 00239 return 0; 00240 } 00241 00242 int GT511C3::Identify(void) 00243 { 00244 unsigned long Parameter = 0; 00245 unsigned short Response = 0; 00246 int sts = 0; 00247 00248 sts = SendRecv(CMD_Identify,&Parameter,&Response); 00249 if((sts != 0) || (Response != CMD_Ack)) 00250 return -1; 00251 return Parameter; 00252 } 00253 00254 int GT511C3::Enroll(int ID,int (*progress)(int status,char *msg)) 00255 { 00256 unsigned long Parameter = 0; 00257 unsigned short Response = 0; 00258 int sts = 0; 00259 00260 CmosLed(1); 00261 00262 while(1){ 00263 if((sts = (*progress)(1,"EnrollStart\r\n")) != 0) 00264 return -9999; 00265 Parameter = ID; 00266 sts = SendRecv(CMD_EnrollStart,&Parameter,&Response); 00267 if(sts != 0) 00268 return sts; 00269 if(Response != CMD_Ack) 00270 return -100; 00271 00272 if((sts = (*progress)(0,"Remove finger\r\n")) != 0) 00273 return -9999; 00274 WaitPress(0); 00275 00276 while(1){ 00277 if((sts = (*progress)(10,"Press finger to Enroll (1st)\r\n")) != 0) 00278 return -9999; 00279 WaitPress(1); 00280 if(Capture(1) == 0) 00281 break; 00282 } 00283 00284 if((sts = (*progress)(0,"Remove finger\r\n")) != 0) 00285 return -9999; 00286 if(Enroll_N(1) != 0) 00287 continue; 00288 WaitPress(0); 00289 00290 while(1){ 00291 if((sts = (*progress)(20,"Press finger to Enroll (2nd)\r\n")) != 0) 00292 return -9999; 00293 WaitPress(1); 00294 if(Capture(1) == 0) 00295 break; 00296 } 00297 00298 if((sts = (*progress)(0,"Remove finger\r\n")) != 0) 00299 return -9999; 00300 if(Enroll_N(2) != 0) 00301 continue; 00302 WaitPress(0); 00303 00304 while(1){ 00305 if((sts = (*progress)(30,"Press finger to Enroll (3rd)\r\n")) != 0) 00306 return -9999; 00307 WaitPress(1); 00308 if(Capture(1) == 0) 00309 break; 00310 } 00311 00312 if((sts = (*progress)(0,"Remove finger\r\n")) != 0) 00313 return -9999; 00314 if(Enroll_N(3) != 0) 00315 continue; 00316 WaitPress(0); 00317 00318 if((sts = (*progress)(100,"Enroll OK\r\n")) != 0) 00319 return -9999; 00320 00321 break; 00322 } 00323 return 0; 00324 } 00325 00326 int GT511C3::CheckEnrolled(int ID) 00327 { 00328 unsigned long Parameter = ID; 00329 unsigned short Response = 0; 00330 int sts = 0; 00331 00332 sts = SendRecv(CMD_CheckEnrolled,&Parameter,&Response); 00333 if((sts == 0) && (Response == CMD_Ack)) 00334 return 0; //This ID is enrolled 00335 return -1; 00336 } 00337 00338 int GT511C3::DeleteID(int ID) 00339 { 00340 unsigned long Parameter = ID; 00341 unsigned short Response = 0; 00342 int sts = 0; 00343 00344 sts = SendRecv(CMD_DeleteID,&Parameter,&Response); 00345 if((sts == 0) && (Response == CMD_Ack)) 00346 return 0; 00347 return -1; 00348 } 00349 00350 int GT511C3::DeleteID_All(void) 00351 { 00352 unsigned long Parameter = 0; 00353 unsigned short Response = 0; 00354 int sts = 0; 00355 00356 sts = SendRecv(CMD_DeleteAll,&Parameter,&Response); 00357 if((sts == 0) && (Response == CMD_Ack)) 00358 return 0; 00359 return -1; 00360 } 00361
Generated on Sat Jul 16 2022 07:25:13 by
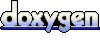