Lcd companion boards support (VKLCD50RTA & VKLCD70RT)
Embed:
(wiki syntax)
Show/hide line numbers
port_api.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef MBED_PORTMAP_H 00017 #define MBED_PORTMAP_H 00018 00019 #include "device.h" 00020 00021 #if DEVICE_PORTIN || DEVICE_PORTOUT 00022 00023 #ifdef __cplusplus 00024 extern "C" { 00025 #endif 00026 00027 /** Port HAL structure. port_s is declared in the target's HAL 00028 */ 00029 typedef struct port_s port_t; 00030 00031 /** 00032 * \defgroup hal_port Port HAL functions 00033 * @{ 00034 */ 00035 00036 /** Get the pin name from the port's pin number 00037 * 00038 * @param port The port name 00039 * @param pin_n The pin number within the specified port 00040 * @return The pin name for the port's pin number 00041 */ 00042 PinName port_pin(PortName port, int pin_n); 00043 00044 /** Initilize the port 00045 * 00046 * @param obj The port object to initialize 00047 * @param port The port name 00048 * @param mask The bitmask to identify which bits in the port should be included (0 - ignore) 00049 * @param dir The port direction 00050 */ 00051 void port_init(port_t *obj, PortName port, int mask, PinDirection dir); 00052 00053 /** Set the input port mode 00054 * 00055 * @param obj The port object 00056 * @param mode THe port mode to be set 00057 */ 00058 void port_mode(port_t *obj, PinMode mode); 00059 00060 /** Set port direction (in/out) 00061 * 00062 * @param obj The port object 00063 * @param dir The port direction to be set 00064 */ 00065 void port_dir(port_t *obj, PinDirection dir); 00066 00067 /** Write value to the port 00068 * 00069 * @param obj The port object 00070 * @param value The value to be set 00071 */ 00072 void port_write(port_t *obj, int value); 00073 00074 /** Read the current value on the port 00075 * 00076 * @param obj The port object 00077 * @return An integer with each bit corresponding to an associated port pin setting 00078 */ 00079 int port_read(port_t *obj); 00080 00081 /**@}*/ 00082 00083 #ifdef __cplusplus 00084 } 00085 #endif 00086 #endif 00087 00088 #endif 00089
Generated on Tue Jul 12 2022 14:32:44 by
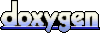