Lcd companion boards support (VKLCD50RTA & VKLCD70RT)
Embed:
(wiki syntax)
Show/hide line numbers
Touch.h
Go to the documentation of this file.
00001 /**************************************************************************//** 00002 * @file Touch.h 00003 * @brief Touch driver class for VK-LCD panels 00004 ******************************************************************************/ 00005 00006 #ifndef MBED_TOUCH_H 00007 #define MBED_TOUCH_H 00008 00009 #include "mbed.h" 00010 #include <string> 00011 #include "stmpe811iic.h" 00012 00013 /*! @enum Touch_type_t 00014 @brief Type of the touch controller 00015 */ 00016 typedef enum { 00017 RESISTIVE = 0, /*!< Resistive */ 00018 CAPACITIVE /*!< Capacitive */ 00019 } Touch_type_t; 00020 00021 /*! @enum Touch_interface_type_t 00022 @brief Communication interface 00023 */ 00024 typedef enum { 00025 I_2_C = 0, /*!< I2C */ 00026 S_P_I /*!< SPI */ 00027 } Touch_communication_type_t ; 00028 00029 /*! @enum IRQ_trigger_t 00030 @brief Type of the interrupt 00031 */ 00032 typedef enum { 00033 INT_ON_EDGE = 0, /*!< generate INT on front change */ 00034 INT_ON_LEVEL /*!< generate INT on active level */ 00035 } IRQ_trigger_t; 00036 00037 /*! @enum IRQ_polarity_t 00038 @brief Edge of a signal 00039 */ 00040 typedef enum { 00041 RISING_OR_ACTIVE_HI = 0, /*!< Rising edge/HI level */ 00042 FALLING_OR_ACTIVE_LO /*!< Falling edge/LOW level */ 00043 } IRQ_polarity_t; 00044 00045 /*! @struct touch_config_t 00046 @brief Touch Config structure 00047 */ 00048 typedef struct { 00049 string name ; /*!< Name of the Touch driver */ 00050 int screen ; /*!< reserved */ 00051 Touch_type_t type ; /*!< Resistive or Capacitive */ 00052 struct { /*!< I2C or SPI & the pins of selected periphery */ 00053 Touch_communication_type_t type ; 00054 PinName sda; 00055 PinName scl; 00056 PinName mosi; 00057 PinName miso; 00058 PinName sclk; 00059 PinName ssel; 00060 int freq; 00061 }interface; 00062 struct { /*!< IRQ : front and pin of selected periphery */ 00063 IRQ_trigger_t trigger ; 00064 IRQ_polarity_t polarity; 00065 PinName pin; 00066 }activity_irq; 00067 }touch_config_t; 00068 00069 extern const touch_config_t STMPE811_cfg; 00070 00071 /*! @class Touch 00072 * @brief Touch driver class for VK-LCD panels 00073 */ 00074 00075 class Touch : public I2C, public InterruptIn 00076 { 00077 public: 00078 /*! @enum Init_err_t 00079 @brief Error codes 00080 */ 00081 typedef enum { 00082 TOUCH_OK = 0, /*!< Initialization successful */ 00083 TOUCH_INIT_ERR = -1, /*!< Communication interface err while configuring driver */ 00084 TOUCH_UNSUPP_ERR = -2, /*!< Unsupported driver */ 00085 TOUCH_ERR = -3, /*!< unknown error */ 00086 } init_err_t ; 00087 00088 /** Constructor method of Touch object 00089 */ 00090 Touch( const touch_config_t * tp_cfg = &STMPE811_cfg ); 00091 00092 /** Destructor method of Touch object 00093 */ 00094 virtual ~Touch( void ); 00095 00096 /** Touch controller initialization 00097 * @retval Error code 00098 */ 00099 init_err_t Init( void ); 00100 00101 /** New Data available 00102 */ 00103 static void Irq_Alert( void ); 00104 00105 /** Set Calibration data (raw data interpretation) 00106 * @retval Error code 00107 */ 00108 virtual init_err_t Clb_Setup(void); 00109 00110 /** Set Touch Controller settings 00111 * @retval Error code 00112 */ 00113 virtual init_err_t Drv_Setup(void); 00114 00115 /** Get one sample of data 00116 * @param[in] raw: pointer to ring buffer to store the samples 00117 */ 00118 virtual void Get_Data( unsigned long long * raw ); 00119 00120 /** Get all available samples of data 00121 * @param[in] raw: pointer to ring buffer to store the samples 00122 * @retval samples count 00123 */ 00124 virtual int Get_Fifo( unsigned long long * raw ); 00125 00126 /** Transfer function (from raw coordinates to screen coordinates) 00127 * @param[in] points : number of samples to transfer 00128 */ 00129 virtual void Get_XYZ( int points ); 00130 00131 /** Pull the new samples if new data is available 00132 * @param[in] pts: pointer to a variable to put the count of the new samples 00133 * @retval Status of the pen => up:false; down:true 00134 */ 00135 bool Handle_touch( unsigned char *pts ); 00136 00137 /** Get index of the last sample in the ring buffer 00138 */ 00139 int Get_Last_Idx( void ); 00140 00141 short x; 00142 short y; 00143 float z; 00144 const touch_screen_data_t *xyz_data; 00145 00146 unsigned short adc_x; 00147 unsigned short adc_y; 00148 unsigned short adc_z; 00149 const touch_raw_data_t *adc_data; 00150 00151 protected: 00152 static bool new_data; 00153 int last_xyz_idx; 00154 touch_raw_data_t raw_data[FIFO_DEPTH]; 00155 touch_screen_data_t screen_data[FIFO_DEPTH]; 00156 const touch_config_t *touch_cfg; 00157 touch_calib_data_t calib; 00158 }; 00159 00160 #endif /* MBED_TOUCH_H */ 00161
Generated on Tue Jul 12 2022 14:32:48 by
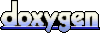