Lcd companion boards support (VKLCD50RTA & VKLCD70RT)
Embed:
(wiki syntax)
Show/hide line numbers
CallChain.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef MBED_CALLCHAIN_H 00017 #define MBED_CALLCHAIN_H 00018 00019 #include "Callback.h" 00020 #include <string.h> 00021 00022 namespace mbed { 00023 00024 /** Group one or more functions in an instance of a CallChain, then call them in 00025 * sequence using CallChain::call(). Used mostly by the interrupt chaining code, 00026 * but can be used for other purposes. 00027 * 00028 * @Note Synchronization level: Not protected 00029 * 00030 * Example: 00031 * @code 00032 * #include "mbed.h" 00033 * 00034 * CallChain chain; 00035 * 00036 * void first(void) { 00037 * printf("'first' function.\n"); 00038 * } 00039 * 00040 * void second(void) { 00041 * printf("'second' function.\n"); 00042 * } 00043 * 00044 * class Test { 00045 * public: 00046 * void f(void) { 00047 * printf("A::f (class member).\n"); 00048 * } 00049 * }; 00050 * 00051 * int main() { 00052 * Test test; 00053 * 00054 * chain.add(second); 00055 * chain.add_front(first); 00056 * chain.add(&test, &Test::f); 00057 * chain.call(); 00058 * } 00059 * @endcode 00060 */ 00061 00062 typedef Callback<void()> *pFunctionPointer_t; 00063 class CallChainLink; 00064 00065 class CallChain { 00066 public: 00067 /** Create an empty chain 00068 * 00069 * @param size (optional) Initial size of the chain 00070 */ 00071 CallChain(int size = 4); 00072 virtual ~CallChain(); 00073 00074 /** Add a function at the end of the chain 00075 * 00076 * @param func A pointer to a void function 00077 * 00078 * @returns 00079 * The function object created for 'func' 00080 */ 00081 pFunctionPointer_t add(Callback<void()> func); 00082 00083 /** Add a function at the end of the chain 00084 * 00085 * @param obj pointer to the object to call the member function on 00086 * @param method pointer to the member function to be called 00087 * 00088 * @returns 00089 * The function object created for 'obj' and 'method' 00090 */ 00091 template<typename T, typename M> 00092 pFunctionPointer_t add(T *obj, M method) { 00093 return add(Callback<void()>(obj, method)); 00094 } 00095 00096 /** Add a function at the beginning of the chain 00097 * 00098 * @param func A pointer to a void function 00099 * 00100 * @returns 00101 * The function object created for 'func' 00102 */ 00103 pFunctionPointer_t add_front(Callback<void()> func); 00104 00105 /** Add a function at the beginning of the chain 00106 * 00107 * @param tptr pointer to the object to call the member function on 00108 * @param mptr pointer to the member function to be called 00109 * 00110 * @returns 00111 * The function object created for 'tptr' and 'mptr' 00112 */ 00113 template<typename T, typename M> 00114 pFunctionPointer_t add_front(T *obj, M method) { 00115 return add_front(Callback<void()>(obj, method)); 00116 } 00117 00118 /** Get the number of functions in the chain 00119 */ 00120 int size() const; 00121 00122 /** Get a function object from the chain 00123 * 00124 * @param i function object index 00125 * 00126 * @returns 00127 * The function object at position 'i' in the chain 00128 */ 00129 pFunctionPointer_t get(int i) const; 00130 00131 /** Look for a function object in the call chain 00132 * 00133 * @param f the function object to search 00134 * 00135 * @returns 00136 * The index of the function object if found, -1 otherwise. 00137 */ 00138 int find(pFunctionPointer_t f) const; 00139 00140 /** Clear the call chain (remove all functions in the chain). 00141 */ 00142 void clear(); 00143 00144 /** Remove a function object from the chain 00145 * 00146 * @arg f the function object to remove 00147 * 00148 * @returns 00149 * true if the function object was found and removed, false otherwise. 00150 */ 00151 bool remove(pFunctionPointer_t f); 00152 00153 /** Call all the functions in the chain in sequence 00154 */ 00155 void call(); 00156 00157 void operator ()(void) { 00158 call(); 00159 } 00160 pFunctionPointer_t operator [](int i) const { 00161 return get(i); 00162 } 00163 00164 /* disallow copy constructor and assignment operators */ 00165 private: 00166 CallChain(const CallChain&); 00167 CallChain & operator = (const CallChain&); 00168 CallChainLink *_chain; 00169 }; 00170 00171 } // namespace mbed 00172 00173 #endif 00174
Generated on Tue Jul 12 2022 14:32:41 by
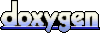