
Ironcup Mar 2020
Dependencies: mbed mbed-rtos MotionSensor EthernetInterface
receiver.h
00001 /** 00002 @file receiver.h 00003 @brief Receiver side functions declarations. 00004 */ 00005 00006 /* 00007 Copyright 2016 Erik Perillo <erik.perillo@gmail.com> 00008 00009 This file is part of piranha-ptc. 00010 00011 This is free software: you can redistribute it and/or modify 00012 it under the terms of the GNU General Public License as published by 00013 the Free Software Foundation, either version 3 of the License, or 00014 (at your option) any later version. 00015 00016 This is distributed in the hope that it will be useful, 00017 but WITHOUT ANY WARRANTY; without even the implied warranty of 00018 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. 00019 See the GNU General Public License for more details. 00020 00021 You should have received a copy of the GNU General Public License 00022 along with this. If not, see <http://www.gnu.org/licenses/>. 00023 */ 00024 00025 00026 #ifndef __PIRANHA_RCV_PROTOCOL_H__ 00027 #define __PIRANHA_RCV_PROTOCOL_H__ 00028 00029 #include "protocol.h" 00030 #include "mbed.h" 00031 #include "EthernetInterface.h" 00032 00033 #define TEST 00034 00035 class Receiver 00036 { 00037 00038 #ifdef TEST 00039 public: 00040 #else 00041 protected: 00042 #endif 00043 UDPSocket sock; 00044 char message[MSG_BUF_LEN]; 00045 Endpoint sender_addr; 00046 00047 float un_scale(uint16_t value, float min, float max); 00048 uint8_t get_header(); 00049 uint16_t get_raw_val(int pos=0); 00050 float get_val(float min, float max, int pos=0); 00051 void get_vals(float min, float max, float* vals, int size); 00052 00053 public: 00054 Receiver(); 00055 Receiver(Endpoint sender_addr, const UDPSocket& sock); 00056 Receiver(Endpoint sender_addr, int sock_port=RECEIVER_PORT, int timeout=1); 00057 00058 void set_sender_addr(const Endpoint& sender_addr); 00059 void set_socket(const UDPSocket& sock); 00060 void set_socket(int port=RECEIVER_PORT, int timeout=1); 00061 Endpoint get_sender_addr(); 00062 UDPSocket get_socket(); 00063 00064 bool receive(); 00065 uint8_t get_msg(); 00066 float get_abs_ang_ref(); 00067 float get_rel_ang_ref(); 00068 float get_mag_ang_ref(); 00069 float get_gnd_vel(); 00070 void get_brake(float* intensity, float* period); 00071 void get_jog_vel(float* period, float* ratio); 00072 void get_pid_params(float* params); 00073 void get_mag_calib(float* params); 00074 }; 00075 00076 #endif 00077
Generated on Wed Jul 13 2022 00:13:54 by
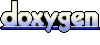