
Creating a project about IKS10A2 for TT_Mxx
Embed:
(wiki syntax)
Show/hide line numbers
LSM6DSLSensor.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file LSM6DSLSensor.h 00004 * @author AST 00005 * @version V1.0.0 00006 * @date 7 September 2017 00007 * @brief Abstract Class of an LSM6DSL Inertial Measurement Unit (IMU) 6 axes 00008 * sensor. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 00040 /* Prevent recursive inclusion -----------------------------------------------*/ 00041 00042 #ifndef __LSM6DSLSensor_H__ 00043 #define __LSM6DSLSensor_H__ 00044 00045 00046 /* Includes ------------------------------------------------------------------*/ 00047 00048 #include "common_define.h" 00049 #include "LSM6DSL_ACC_GYRO_Driver.h" 00050 00051 /* Defines -------------------------------------------------------------------*/ 00052 00053 #define LSM6DSL_ACC_SENSITIVITY_FOR_FS_2G 0.061 /**< Sensitivity value for 2 g full scale [mg/LSB] */ 00054 #define LSM6DSL_ACC_SENSITIVITY_FOR_FS_4G 0.122 /**< Sensitivity value for 4 g full scale [mg/LSB] */ 00055 #define LSM6DSL_ACC_SENSITIVITY_FOR_FS_8G 0.244 /**< Sensitivity value for 8 g full scale [mg/LSB] */ 00056 #define LSM6DSL_ACC_SENSITIVITY_FOR_FS_16G 0.488 /**< Sensitivity value for 16 g full scale [mg/LSB] */ 00057 00058 #define LSM6DSL_GYRO_SENSITIVITY_FOR_FS_125DPS 04.375 /**< Sensitivity value for 125 dps full scale [mdps/LSB] */ 00059 #define LSM6DSL_GYRO_SENSITIVITY_FOR_FS_245DPS 08.750 /**< Sensitivity value for 245 dps full scale [mdps/LSB] */ 00060 #define LSM6DSL_GYRO_SENSITIVITY_FOR_FS_500DPS 17.500 /**< Sensitivity value for 500 dps full scale [mdps/LSB] */ 00061 #define LSM6DSL_GYRO_SENSITIVITY_FOR_FS_1000DPS 35.000 /**< Sensitivity value for 1000 dps full scale [mdps/LSB] */ 00062 #define LSM6DSL_GYRO_SENSITIVITY_FOR_FS_2000DPS 70.000 /**< Sensitivity value for 2000 dps full scale [mdps/LSB] */ 00063 00064 #define LSM6DSL_PEDOMETER_THRESHOLD_LOW 0x00 /**< Lowest value of pedometer threshold */ 00065 #define LSM6DSL_PEDOMETER_THRESHOLD_MID_LOW 0x07 00066 #define LSM6DSL_PEDOMETER_THRESHOLD_MID 0x0F 00067 #define LSM6DSL_PEDOMETER_THRESHOLD_MID_HIGH 0x17 00068 #define LSM6DSL_PEDOMETER_THRESHOLD_HIGH 0x1F /**< Highest value of pedometer threshold */ 00069 00070 #define LSM6DSL_WAKE_UP_THRESHOLD_LOW 0x01 /**< Lowest value of wake up threshold */ 00071 #define LSM6DSL_WAKE_UP_THRESHOLD_MID_LOW 0x0F 00072 #define LSM6DSL_WAKE_UP_THRESHOLD_MID 0x1F 00073 #define LSM6DSL_WAKE_UP_THRESHOLD_MID_HIGH 0x2F 00074 #define LSM6DSL_WAKE_UP_THRESHOLD_HIGH 0x3F /**< Highest value of wake up threshold */ 00075 00076 #define LSM6DSL_TAP_THRESHOLD_LOW 0x01 /**< Lowest value of wake up threshold */ 00077 #define LSM6DSL_TAP_THRESHOLD_MID_LOW 0x08 00078 #define LSM6DSL_TAP_THRESHOLD_MID 0x10 00079 #define LSM6DSL_TAP_THRESHOLD_MID_HIGH 0x18 00080 #define LSM6DSL_TAP_THRESHOLD_HIGH 0x1F /**< Highest value of wake up threshold */ 00081 00082 #define LSM6DSL_TAP_SHOCK_TIME_LOW 0x00 /**< Lowest value of wake up threshold */ 00083 #define LSM6DSL_TAP_SHOCK_TIME_MID_LOW 0x01 00084 #define LSM6DSL_TAP_SHOCK_TIME_MID_HIGH 0x02 00085 #define LSM6DSL_TAP_SHOCK_TIME_HIGH 0x03 /**< Highest value of wake up threshold */ 00086 00087 #define LSM6DSL_TAP_QUIET_TIME_LOW 0x00 /**< Lowest value of wake up threshold */ 00088 #define LSM6DSL_TAP_QUIET_TIME_MID_LOW 0x01 00089 #define LSM6DSL_TAP_QUIET_TIME_MID_HIGH 0x02 00090 #define LSM6DSL_TAP_QUIET_TIME_HIGH 0x03 /**< Highest value of wake up threshold */ 00091 00092 #define LSM6DSL_TAP_DURATION_TIME_LOW 0x00 /**< Lowest value of wake up threshold */ 00093 #define LSM6DSL_TAP_DURATION_TIME_MID_LOW 0x04 00094 #define LSM6DSL_TAP_DURATION_TIME_MID 0x08 00095 #define LSM6DSL_TAP_DURATION_TIME_MID_HIGH 0x0C 00096 #define LSM6DSL_TAP_DURATION_TIME_HIGH 0x0F /**< Highest value of wake up threshold */ 00097 00098 /* Typedefs ------------------------------------------------------------------*/ 00099 typedef enum 00100 { 00101 LSM6DSL_STATUS_OK = 0, 00102 LSM6DSL_STATUS_ERROR, 00103 LSM6DSL_STATUS_TIMEOUT, 00104 LSM6DSL_STATUS_NOT_IMPLEMENTED 00105 } LSM6DSLStatusTypeDef; 00106 00107 typedef enum 00108 { 00109 LSM6DSL_INT1_PIN, 00110 LSM6DSL_INT2_PIN 00111 } LSM6DSL_Interrupt_Pin_t; 00112 00113 typedef struct 00114 { 00115 unsigned int FreeFallStatus : 1; 00116 unsigned int TapStatus : 1; 00117 unsigned int DoubleTapStatus : 1; 00118 unsigned int WakeUpStatus : 1; 00119 unsigned int StepStatus : 1; 00120 unsigned int TiltStatus : 1; 00121 unsigned int D6DOrientationStatus : 1; 00122 } LSM6DSL_Event_Status_t; 00123 00124 /* Class Declaration ---------------------------------------------------------*/ 00125 00126 /** 00127 * Abstract class of an LSM6DSL Inertial Measurement Unit (IMU) 6 axes 00128 * sensor. 00129 */ 00130 00131 00132 00133 00134 class LSM6DSLSensor 00135 { 00136 public: 00137 LSM6DSLSensor (TwoWire *i2c); 00138 LSM6DSLSensor (TwoWire *i2c, uint8_t address); 00139 LSM6DSLStatusTypeDef Enable_X (void); 00140 LSM6DSLStatusTypeDef Enable_G (void); 00141 LSM6DSLStatusTypeDef Disable_X (void); 00142 LSM6DSLStatusTypeDef Disable_G (void); 00143 LSM6DSLStatusTypeDef ReadID (uint8_t *p_id); 00144 LSM6DSLStatusTypeDef Get_X_Axes (int32_t *pData); 00145 LSM6DSLStatusTypeDef Get_G_Axes (int32_t *pData); 00146 LSM6DSLStatusTypeDef Get_X_Sensitivity (float *pfData); 00147 LSM6DSLStatusTypeDef Get_G_Sensitivity (float *pfData); 00148 LSM6DSLStatusTypeDef Get_X_AxesRaw (int16_t *pData); 00149 LSM6DSLStatusTypeDef Get_G_AxesRaw (int16_t *pData); 00150 LSM6DSLStatusTypeDef Get_X_ODR (float *odr); 00151 LSM6DSLStatusTypeDef Get_G_ODR (float *odr); 00152 LSM6DSLStatusTypeDef Set_X_ODR (float odr); 00153 LSM6DSLStatusTypeDef Set_G_ODR (float odr); 00154 LSM6DSLStatusTypeDef Get_X_FS (float *fullScale); 00155 LSM6DSLStatusTypeDef Get_G_FS (float *fullScale); 00156 LSM6DSLStatusTypeDef Set_X_FS (float fullScale); 00157 LSM6DSLStatusTypeDef Set_G_FS (float fullScale); 00158 LSM6DSLStatusTypeDef Enable_Free_Fall_Detection (void); 00159 LSM6DSLStatusTypeDef Enable_Free_Fall_Detection (LSM6DSL_Interrupt_Pin_t int_pin); 00160 LSM6DSLStatusTypeDef Disable_Free_Fall_Detection (void); 00161 LSM6DSLStatusTypeDef Set_Free_Fall_Threshold (uint8_t thr); 00162 LSM6DSLStatusTypeDef Enable_Pedometer (void); 00163 LSM6DSLStatusTypeDef Disable_Pedometer (void); 00164 LSM6DSLStatusTypeDef Get_Step_Counter (uint16_t *step_count); 00165 LSM6DSLStatusTypeDef Reset_Step_Counter (void); 00166 LSM6DSLStatusTypeDef Set_Pedometer_Threshold (uint8_t thr); 00167 LSM6DSLStatusTypeDef Enable_Tilt_Detection (void); 00168 LSM6DSLStatusTypeDef Enable_Tilt_Detection (LSM6DSL_Interrupt_Pin_t int_pin); 00169 LSM6DSLStatusTypeDef Disable_Tilt_Detection (void); 00170 LSM6DSLStatusTypeDef Enable_Wake_Up_Detection (void); 00171 LSM6DSLStatusTypeDef Enable_Wake_Up_Detection (LSM6DSL_Interrupt_Pin_t int_pin); 00172 LSM6DSLStatusTypeDef Disable_Wake_Up_Detection (void); 00173 LSM6DSLStatusTypeDef Set_Wake_Up_Threshold (uint8_t thr); 00174 LSM6DSLStatusTypeDef Enable_Single_Tap_Detection (void); 00175 LSM6DSLStatusTypeDef Enable_Single_Tap_Detection (LSM6DSL_Interrupt_Pin_t int_pin); 00176 LSM6DSLStatusTypeDef Disable_Single_Tap_Detection (void); 00177 LSM6DSLStatusTypeDef Enable_Double_Tap_Detection (void); 00178 LSM6DSLStatusTypeDef Enable_Double_Tap_Detection (LSM6DSL_Interrupt_Pin_t int_pin); 00179 LSM6DSLStatusTypeDef Disable_Double_Tap_Detection (void); 00180 LSM6DSLStatusTypeDef Set_Tap_Threshold (uint8_t thr); 00181 LSM6DSLStatusTypeDef Set_Tap_Shock_Time (uint8_t time); 00182 LSM6DSLStatusTypeDef Set_Tap_Quiet_Time (uint8_t time); 00183 LSM6DSLStatusTypeDef Set_Tap_Duration_Time (uint8_t time); 00184 LSM6DSLStatusTypeDef Enable_6D_Orientation (void); 00185 LSM6DSLStatusTypeDef Enable_6D_Orientation (LSM6DSL_Interrupt_Pin_t int_pin); 00186 LSM6DSLStatusTypeDef Disable_6D_Orientation (void); 00187 LSM6DSLStatusTypeDef Get_6D_Orientation_XL (uint8_t *xl); 00188 LSM6DSLStatusTypeDef Get_6D_Orientation_XH (uint8_t *xh); 00189 LSM6DSLStatusTypeDef Get_6D_Orientation_YL (uint8_t *yl); 00190 LSM6DSLStatusTypeDef Get_6D_Orientation_YH (uint8_t *yh); 00191 LSM6DSLStatusTypeDef Get_6D_Orientation_ZL (uint8_t *zl); 00192 LSM6DSLStatusTypeDef Get_6D_Orientation_ZH (uint8_t *zh); 00193 LSM6DSLStatusTypeDef Get_Event_Status (LSM6DSL_Event_Status_t *status); 00194 LSM6DSLStatusTypeDef ReadReg (uint8_t reg, uint8_t *data); 00195 LSM6DSLStatusTypeDef WriteReg (uint8_t reg, uint8_t data); 00196 00197 /** 00198 * @brief Utility function to read data. 00199 * @param pBuffer: pointer to data to be read. 00200 * @param RegisterAddr: specifies internal address register to be read. 00201 * @param NumByteToRead: number of bytes to be read. 00202 * @retval 0 if ok, an error code otherwise. 00203 */ 00204 uint8_t IO_Read(uint8_t* pBuffer, uint8_t RegisterAddr, uint16_t NumByteToRead) 00205 { 00206 // dev_i2c->beginTransmission(((uint8_t)(((address) >> 1) & 0x7F))); 00207 // dev_i2c->write(RegisterAddr); 00208 // dev_i2c->endTransmission(false); 00209 00210 // dev_i2c->requestFrom(((uint8_t)(((address) >> 1) & 0x7F)), (byte) NumByteToRead); 00211 00212 // int i=0; 00213 // while (dev_i2c->available()) 00214 // { 00215 // pBuffer[i] = dev_i2c->read(); 00216 // i++; 00217 // } 00218 // if(LSM6DSL_ACC_GYRO_WHO_AM_I == RegisterAddr) 00219 // { 00220 // printf("Get ID \r\n"); 00221 // } 00222 // int status; 00223 // status = dev_i2c->write((int)address,(const char *)&RegisterAddr,1); 00224 // if(status == 0) 00225 // { 00226 00227 // } 00228 // else 00229 // { 00230 // printf("Error I2C \r\n"); 00231 // } 00232 // status = dev_i2c->read((int)address,(char *)pBuffer,NumByteToRead); 00233 i2cRead(dev_i2c,address,pBuffer,RegisterAddr,NumByteToRead); 00234 return 0; 00235 } 00236 00237 /** 00238 * @brief Utility function to write data. 00239 * @param pBuffer: pointer to data to be written. 00240 * @param RegisterAddr: specifies internal address register to be written. 00241 * @param NumByteToWrite: number of bytes to write. 00242 * @retval 0 if ok, an error code otherwise. 00243 */ 00244 uint8_t IO_Write(uint8_t* pBuffer, uint8_t RegisterAddr, uint16_t NumByteToWrite) 00245 { 00246 // dev_i2c->beginTransmission(((uint8_t)(((address) >> 1) & 0x7F))); 00247 00248 // dev_i2c->write(RegisterAddr); 00249 // for (int i = 0 ; i < NumByteToWrite ; i++) 00250 // dev_i2c->write(pBuffer[i]); 00251 00252 // dev_i2c->endTransmission(true); 00253 // uint8_t temp_buffer[NumByteToWrite + 1]; 00254 // temp_buffer[0] = RegisterAddr; 00255 // memcpy(temp_buffer + 1,pBuffer,NumByteToWrite); 00256 // dev_i2c->write((int)address,(const char *)temp_buffer,NumByteToWrite + 1); 00257 i2cWrite(dev_i2c,address,pBuffer,RegisterAddr,NumByteToWrite); 00258 return 0; 00259 } 00260 00261 private: 00262 LSM6DSLStatusTypeDef Set_X_ODR_When_Enabled(float odr); 00263 LSM6DSLStatusTypeDef Set_G_ODR_When_Enabled(float odr); 00264 LSM6DSLStatusTypeDef Set_X_ODR_When_Disabled(float odr); 00265 LSM6DSLStatusTypeDef Set_G_ODR_When_Disabled(float odr); 00266 00267 /* Helper classes. */ 00268 TwoWire *dev_i2c; 00269 00270 /* Configuration */ 00271 uint8_t address; 00272 00273 uint8_t X_isEnabled; 00274 float X_Last_ODR; 00275 uint8_t G_isEnabled; 00276 float G_Last_ODR; 00277 }; 00278 00279 #ifdef __cplusplus 00280 extern "C" { 00281 #endif 00282 uint8_t LSM6DSL_IO_Write( void *handle, uint8_t WriteAddr, uint8_t *pBuffer, uint16_t nBytesToWrite ); 00283 uint8_t LSM6DSL_IO_Read( void *handle, uint8_t ReadAddr, uint8_t *pBuffer, uint16_t nBytesToRead ); 00284 #ifdef __cplusplus 00285 } 00286 #endif 00287 00288 #endif
Generated on Tue Jul 12 2022 19:05:13 by
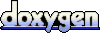