
Create a project for TT_Mxx.
Embed:
(wiki syntax)
Show/hide line numbers
MMA8652.cpp
00001 /* Copyright (c) 2010-2011 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without 00005 * restriction, including without limitation the rights to use, copy, modify, merge, publish, 00006 * distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the 00007 * Software is furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #include "MMA8652.h" 00020 #include "common_define.h" 00021 #define UINT14_MAX 16383 00022 00023 MMA8652::MMA8652(PinName sda, PinName scl) : _i2c(sda, scl) { 00024 00025 begin(); 00026 } 00027 00028 00029 MMA8652::~MMA8652() { } 00030 00031 00032 void MMA8652::RegRead( char reg, char * d, int len) 00033 { 00034 // char cmd = reg; 00035 // char i2c_addr = MMA8652_SLAVE_ADDR; 00036 // int status; 00037 // printf("i2c_addr = 0x%x \r\n",i2c_addr); 00038 // status = _i2c.write( i2c_addr, &cmd, 1); 00039 // status = _i2c.read (i2c_addr, d, len); 00040 // if(status == 0) 00041 // { 00042 // printf("success \r\n"); 00043 // } 00044 // else 00045 // { 00046 // printf("failer \r\n"); 00047 // } 00048 i2cReadForFXS_MUTIL(&_i2c,MMA8652_SLAVE_ADDR,(uint8_t*)d,reg,len); 00049 00050 } 00051 00052 void MMA8652::begin(void) 00053 { 00054 char data[2]; 00055 // write 0000 0000 = 0x00 to accelerometer control register 1 to place MMA8652 into 00056 // standby 00057 // [7-1] = 0000 000 00058 // [0]: active=0 00059 data[0] = MMA8652_CTRL_REG1; 00060 data[1] = 0x00; 00061 _i2c.write( MMA8652_SLAVE_ADDR, data, 2); 00062 00063 // write 0000 0001= 0x01 to XYZ_DATA_CFG register 00064 // [7]: reserved 00065 // [6]: reserved 00066 // [5]: reserved 00067 // [4]: hpf_out=0 00068 // [3]: reserved 00069 // [2]: reserved 00070 // [1-0]: fs=00 for accelerometer range of +/-2g range with 0.244mg/LSB 00071 data[0] = MMA8652_XYZ_DATA_CFG; 00072 data[1] = 0x00; 00073 _i2c.write( MMA8652_SLAVE_ADDR, data, 2); 00074 00075 // write 0000 1101 = 0x0D to accelerometer control register 1 00076 // [7-6]: aslp_rate=00 00077 // [5-3]: dr=100 for 50Hz data rate 00078 // [2]: 0 00079 // [1]: 0 00080 // [0]: active=1 to take the part out of standby and enable sampling 00081 data[0] = MMA8652_CTRL_REG1; 00082 data[1] = 0x21; 00083 _i2c.write( MMA8652_SLAVE_ADDR, data, 2); 00084 } 00085 00086 char MMA8652::getWhoAmI(void) 00087 { 00088 char Id = 0x00; 00089 RegRead(MMA8652_WHOAMI, &Id, 1); 00090 return Id; 00091 } 00092 00093 void MMA8652::ReadXYZ(float * a) 00094 { 00095 char d[7]; 00096 int16_t t[6]; 00097 00098 RegRead( MMA8652_STATUS, d, 7); 00099 t[0] = ((d[1] * 256) + ((unsigned short) d[2])); 00100 t[1] = ((d[3] * 256) + ((unsigned short) d[4])); 00101 t[2] = ((d[5] * 256) + ((unsigned short) d[6])); 00102 00103 a[0] = (float) t[0] / 16384.0; 00104 a[1] = (float) t[1] / 16384.0; 00105 a[2] = (float) t[2] / 16384.0; 00106 00107 } 00108 00109 00110 void MMA8652::ReadXYZraw(int16_t * d) 00111 { 00112 char res[6]; 00113 int16_t acc; 00114 RegRead( MMA8652_OUT_X_MSB, res, 6); 00115 00116 acc = (res[0] << 6) | (res[1] >> 2); 00117 if (acc > UINT14_MAX/2) 00118 acc -= UINT14_MAX; 00119 d[0] = acc; 00120 acc = (res[2] << 6) | (res[3] >> 2); 00121 if (acc > UINT14_MAX/2) 00122 acc -= UINT14_MAX; 00123 d[1] = acc; 00124 acc = (res[4] << 6) | (res[5] >> 2); 00125 if (acc > UINT14_MAX/2) 00126 acc -= UINT14_MAX; 00127 d[2] = acc; 00128 } 00129 00130
Generated on Wed Jul 13 2022 20:20:49 by
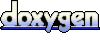