
v2 for lunchbox
Dependencies: HTTPClient PWM_Tone_Library PinDetect_KL25Z Queue cc3000_hostdriver_mbedsocket mbed
Fork of kragl by
main.cpp
00001 /** 00002 * \brief CS294-84 demo \author Ben Zhang, Antonio Iannopollo 00003 * 00004 * This sampel code illustrates how to connect the mbed KL25Z platform to internet 00005 * thorugh the CC3000 wifi breakout board (http://www.adafruit.com/product/1469). 00006 * Connections between the KL25Z and the CC3000 are made according to the 00007 * guide at https://learn.adafruit.com/adafruit-cc3000-wifi -- KL25Z and arduino 00008 * UNO are pin to pin compatible -- 00009 * 00010 * This application uses the following libraries: 00011 * - cc3000_hostdriver_mbedsocket 00012 * (http://developer.mbed.org/users/Kojto/code/cc3000_hostdriver_mbedsocket/) 00013 * - HTTPClient (http://developer.mbed.org/users/donatien/code/HTTPClient/) 00014 */ 00015 #include <stdio.h> 00016 #include <stdlib.h> 00017 #include <string.h> 00018 #include <time.h> 00019 #include "mbed.h" 00020 #include "cc3000.h" 00021 #include "HTTPClient.h" 00022 #include "PinDetect.h" 00023 #include "pwm_tone.h" 00024 00025 // KL25Z wifi connection 00026 // we need to define connection pins for: 00027 // - IRQ => (pin D3) 00028 // - Enable => (pin D5) 00029 // - SPI CS => (pin D10) 00030 // - SPI MOSI => (pin D11) 00031 // - SPI MISO => (pin D12) 00032 // - SPI CLK => (pin D13) 00033 // plus wifi network SSID, password, security level and smart-configuration flag. 00034 mbed_cc3000::cc3000 wifi(D3, D5, D10, SPI(D11, D12, D13), 00035 "Fire_2441", "", NONE, false); 00036 00037 // create an http instance 00038 HTTPClient http; 00039 00040 // str is used to hold the response data 00041 char str[512]; 00042 char url[128]; 00043 char in[512]; 00044 char out[512]; 00045 00046 00047 // setup the serial connection, and LEDs 00048 Serial pc(USBTX, USBRX); 00049 DigitalOut led_red(LED_RED); 00050 DigitalOut led_green(LED_GREEN); 00051 00052 PinDetect p1(D4); 00053 PinDetect p2(D6); 00054 PinDetect p3(D7); 00055 PinDetect reed(A3); 00056 DigitalOut RGBindicator(D2); 00057 DigitalOut Buzzer(D9); 00058 DigitalOut warningLED(A4); 00059 DigitalOut correctLED(A5); 00060 00061 int changedFromOFF = 0; 00062 int changedFromON = 0; 00063 int code[] = {0, 0, 0, 0}; 00064 int passwordcounter = 0; 00065 bool codeEntered = false; 00066 bool reedIsOpen = false; 00067 bool alarmOn = false; 00068 bool boxOpenLegal = false; 00069 int password[] = {1, 1, 1, 1}; 00070 float C_3 = 1000000/Do3, 00071 Cs_3 = 1000000/Do3s, 00072 D_3 = 1000000/Re3, 00073 Ds_3 = 1000000/Re3s, 00074 E_3 = 1000000/Mi3, 00075 F_3 = 1000000/Fa3, 00076 Fs_3 = 1000000/Fa3s, 00077 G_3 = 1000000/So3, 00078 Gs_3 = 1000000/So3s, 00079 A_3 = 1000000/La3, 00080 As_3 = 1000000/La3s, 00081 B_3 = 1000000/Ti3, 00082 C_4 = 1000000/Do4, 00083 Cs_4 = 1000000/Do4s, 00084 D_4 = 1000000/Re4, 00085 Ds_4 = 1000000/Re4s, 00086 E_4 = 1000000/Mi4, 00087 F_4 = 1000000/Fa4, 00088 Fs_4 = 1000000/Fa4s, 00089 G_4 = 1000000/So4, 00090 Gs_4 = 1000000/So4s, 00091 A_4 = 1000000/La4, 00092 As_4 = 1000000/La4s, 00093 B_4 = 1000000/Ti4, 00094 C_5 = 1000000/Do5, 00095 Cs_5 = 1000000/Do5s, 00096 D_5 = 1000000/Re5, 00097 Ds_5 = 1000000/Re5s, 00098 E_5 = 1000000/Mi5, 00099 F_5 = 1000000/Fa5, 00100 Fs_5 = 1000000/Fa5s, 00101 G_5 = 1000000/So5, 00102 Gs_5 = 1000000/So5s, 00103 A_5 = 1000000/La5, 00104 As_5 = 1000000/La5s, 00105 B_5 = 1000000/Ti5; 00106 00107 int tones[] = {E_4, D_4, C_4, D_4, E_4, E_4, E_4, 0, D_4, D_4, D_4, 0, E_4, G_4, G_4, 0, 00108 E_4, D_4, C_4, D_4, E_4, E_4, E_4, 0, D_4, D_4, E_4, D_4, C_4, 0, 0, 0 00109 }; 00110 int tones_num = 32; 00111 00112 //Queue buttonQ(4,4); 00113 00114 void key1Pressed( void ) 00115 { 00116 //pc.printf("Key Pressed\n"); 00117 int i = 1; 00118 code[passwordcounter] = i; 00119 passwordcounter++; 00120 if (codeEntered==false){ 00121 correctLED = 1; 00122 } 00123 //buttonQ.Put(&i); 00124 } 00125 00126 void key1Released( void ) 00127 { 00128 //pc.printf("Key Released\n"); 00129 if (codeEntered==false){ 00130 correctLED = 0; 00131 } 00132 } 00133 00134 void key1PressedHeld( void ) 00135 { 00136 //pc.printf("Key Pressed Held\n"); 00137 } 00138 00139 void key2Pressed( void ) 00140 { 00141 //pc.printf("Key Pressed\n"); 00142 int i = 2; 00143 code[passwordcounter] = i; 00144 passwordcounter++; 00145 //buttonQ.Put(&i); 00146 if (codeEntered==false){ 00147 correctLED = 1; 00148 } 00149 00150 } 00151 00152 void key2Released( void ) 00153 { 00154 //pc.printf("Key Released\n"); 00155 if (codeEntered==false){ 00156 correctLED = 0; 00157 } 00158 } 00159 00160 void key2PressedHeld( void) 00161 { 00162 //pc.printf("Key Pressed Held\n"); 00163 } 00164 00165 void key3Pressed( void ) 00166 { 00167 //pc.printf("Key Pressed\n"); 00168 int i = 3; 00169 code[passwordcounter] = i; 00170 passwordcounter++; 00171 //buttonQ.Put(&i); 00172 if (codeEntered==false){ 00173 correctLED = 1; 00174 } 00175 00176 } 00177 00178 void key3Released( void ) 00179 { 00180 //pc.printf("Key Released\n"); 00181 if (codeEntered==false){ 00182 correctLED = 0; 00183 } 00184 } 00185 00186 void key3PressedHeld( void ) 00187 { 00188 //pc.printf("Key Pressed Held\n"); 00189 } 00190 00191 void reedOpen( void ) 00192 { 00193 pc.printf("Reed Open\r\n"); 00194 pc.printf("boxOpenLegal: %d\r\n", boxOpenLegal); 00195 reedIsOpen = 1; 00196 if (!boxOpenLegal){ 00197 Buzzer = 1; 00198 changedFromOFF = 1; 00199 } 00200 } 00201 00202 void reedClosed( void ) 00203 { 00204 pc.printf("Reed Closed\n"); 00205 reedIsOpen = 0; 00206 if (boxOpenLegal==true){ 00207 changedFromON = 1; 00208 correctLED =0; 00209 for (int j=0; j<8; j++){ 00210 warningLED =1; 00211 wait(0.15); 00212 warningLED = 0; 00213 wait(0.15); 00214 } 00215 Buzzer = 0; 00216 } 00217 pc.printf("boxOpenLegal: %d\r\n", boxOpenLegal); 00218 } 00219 00220 void reedOpenHeld( void ) 00221 { 00222 //pc.printf("Key Pressed Held\n"); 00223 reedIsOpen = 1; 00224 } 00225 00226 int main() 00227 { 00228 // by default, it's red 00229 led_red = 0; 00230 led_green = 1; 00231 for (int j=0; j<4; j++){ 00232 warningLED =1; 00233 wait(0.5); 00234 warningLED = 0; 00235 wait(0.5); 00236 } 00237 correctLED = 0; 00238 // print message to indicate the program has started 00239 pc.printf("CC3000 Lunch Theft\r\n"); 00240 wifi.init(); 00241 00242 p1.mode(PullUp); 00243 p2.mode(PullUp); 00244 p3.mode(PullUp); 00245 reed.mode(PullUp); 00246 00247 p1.attach_asserted( &key1Pressed); 00248 p1.attach_deasserted( &key1Released); 00249 p1.attach_asserted_held( &key1PressedHeld); 00250 p1.setAssertValue( 0 ); 00251 p1.setSampleFrequency( 10000 ); 00252 00253 p2.attach_asserted( &key2Pressed); 00254 p2.attach_deasserted( &key2Released); 00255 p2.attach_asserted_held( &key2PressedHeld); 00256 p2.setAssertValue( 0 ); 00257 p2.setSampleFrequency( 10000 ); 00258 00259 p3.attach_asserted( &key3Pressed); 00260 p3.attach_deasserted( &key3Released); 00261 p3.attach_asserted_held( &key3PressedHeld); 00262 p3.setAssertValue( 0 ); 00263 p3.setSampleFrequency( 10000 ); 00264 00265 reed.attach_asserted( &reedClosed); 00266 reed.attach_deasserted( &reedOpen); 00267 reed.attach_deasserted_held( &reedOpenHeld); 00268 reed.setAssertValue( 0 ); 00269 reed.setSampleFrequency( 10000 ); 00270 00271 Buzzer = 1; //4 Octave C beat 4/16 00272 wait_ms(1000); 00273 Buzzer = 0; 00274 00275 changedFromOFF = 0; 00276 changedFromON = 0; 00277 00278 clock_t start_t, current_t, total_t; 00279 //int counterforpasswordcheck = 0; 00280 //int i; 00281 int init = 0; // init wifi done 00282 start_t = clock(); 00283 int num = 0; 00284 reedIsOpen = (reed == 1); 00285 while (1) { 00286 pc.printf("reed value:%d\r\n",reedIsOpen); 00287 pc.printf("codeEntered:%d\r\n",codeEntered); 00288 pc.printf("boxOpenLegal:%d\r\n",boxOpenLegal); 00289 00290 if (init == 0) { 00291 if(wifi.is_connected() == false) { 00292 // try to connect 00293 if (wifi.connect() == -1) { 00294 pc.printf("Failed to connect." 00295 "Please verify connection details and try again.\r\n"); 00296 } else { 00297 pc.printf("IP address: %s \r\n", wifi.getIPAddress()); 00298 00299 //once connected, turn green LED on and red LED off 00300 led_red = 1; 00301 led_green = 0; 00302 } 00303 } else { 00304 pc.printf("Test first\r\n"); 00305 sprintf(url, "http://www.charlesding.net/kragl/lunchbox.php?isOpen=NO&alarmOn=OFF&boxLocked=YES"); 00306 int ret = http.get(url, str, 128); 00307 init = 1; 00308 int tries = 15; 00309 while (tries > 0) { 00310 ret = http.get(url, str, 128); 00311 if (!ret) { 00312 pc.printf("Requested %s\r\n", url); 00313 pc.printf("Page fetched successfully - read %d characters\r\n", 00314 strlen(str)); 00315 pc.printf("Result: %s\r\n", str); 00316 break; 00317 } else { 00318 pc.printf("Error - ret = %d - HTTP return code = %d\r\n", 00319 ret, 00320 http.getHTTPResponseCode()); 00321 } 00322 tries--; 00323 } 00324 } 00325 } 00326 current_t = clock(); 00327 total_t = (double)(current_t - start_t) / (CLOCKS_PER_SEC); 00328 //pc.printf("total_t: %d\r\n", total_t); 00329 if ((total_t%1)==0){ //every t seconds 00330 pc.printf("SENDING TO URL\n"); 00331 sprintf(url, "http://www.charlesding.net/kragl/pincode_read.php?"); 00332 int ret = http.get(url, str, 128); 00333 pc.printf("Trying to Request %s\r\n", url); 00334 int tries = 50; 00335 while (tries > 0) { 00336 pc.printf("still in while lop #%d", tries); 00337 if (!ret) { 00338 pc.printf("Requested %s\r\n", url); 00339 pc.printf("Page fetched successfully - read %d characters\r\n", 00340 strlen(str)); 00341 00342 char* s = strtok(str, "\n"); 00343 pc.printf("code string: %s\r\n",s); 00344 if (true){ 00345 for (int c=1; c<5; c++){ 00346 char test[] = {s[c]}; 00347 num = atoi(test); 00348 password[c-1] = num; 00349 } 00350 } 00351 char test[] = {s[5]}; 00352 num = atoi(test); 00353 pc.printf("Result last digit: %d\r\n", num); 00354 if (num==1){ 00355 boxOpenLegal = true; 00356 warningLED = 0; 00357 for (int j=0; j<8; j++){ 00358 correctLED =1; 00359 wait(0.15); 00360 correctLED = 0; 00361 wait(0.15); 00362 } 00363 Buzzer = 0; 00364 } else { 00365 boxOpenLegal = false; 00366 if (reedIsOpen){ 00367 Buzzer = 1; 00368 } 00369 correctLED = 0; 00370 for (int j=0; j<8; j++){ 00371 warningLED =1; 00372 wait(0.15); 00373 warningLED = 0; 00374 wait(0.15); 00375 } 00376 } 00377 break; 00378 } else { 00379 pc.printf("Error - ret = %d - HTTP return code = %d\r\n", 00380 ret, 00381 http.getHTTPResponseCode()); 00382 00383 } 00384 tries--; 00385 ret = http.get(url, str, 128); 00386 } 00387 } 00388 //pc.printf("waiting to check...\r\n"); 00389 //pc.printf("boxOpenLegal: %d\r\n", boxOpenLegal); 00390 //pc.printf("reedIsOpen: %d\r\n", reedIsOpen); 00391 if (!boxOpenLegal && reedIsOpen && codeEntered) { 00392 pc.printf("LEGAL OPENING"); 00393 boxOpenLegal = true; 00394 codeEntered = false; 00395 Buzzer = 0; 00396 00397 if (changedFromON){ 00398 pc.printf("SENDING TO URL\n"); 00399 sprintf(url, "http://www.charlesding.net/kragl/lunchbox.php?isOpen=NO&alarmOn=OFF&boxLocked=YES"); 00400 00401 int ret = http.get(url, str, 128); 00402 pc.printf("Trying to Request %s\r\n", url); 00403 int tries = 20; 00404 while (tries > 0) { 00405 ret = http.get(url, str, 128); 00406 if (!ret) { 00407 pc.printf("Requested %s\r\n", url); 00408 pc.printf("Page fetched successfully - read %d characters\r\n", 00409 strlen(str)); 00410 pc.printf("Result: %s\r\n", str); 00411 break; 00412 } else { 00413 pc.printf("Error - ret = %d - HTTP return code = %d\r\n", 00414 ret, 00415 http.getHTTPResponseCode()); 00416 tries--; 00417 } 00418 } 00419 changedFromON = 0; 00420 } 00421 00422 00423 } else if (reedIsOpen && !boxOpenLegal) { 00424 // turn on alarm; 00425 pc.printf("boxOpenLegal: %d\r\n", boxOpenLegal); 00426 pc.printf("reedIsOpen: %d\r\n", reedIsOpen); 00427 pc.printf("ALARM TIME\r\n"); 00428 Buzzer = 1; 00429 00430 if(wifi.is_connected() == false) { 00431 // try to connect 00432 if (wifi.connect() == -1) { 00433 pc.printf("Failed to connect." 00434 "Please verify connection details and try again.\r\n"); 00435 } else { 00436 pc.printf("IP address: %s \r\n", wifi.getIPAddress()); 00437 00438 //once connected, turn green LED on and red LED off 00439 led_red = 1; 00440 led_green = 0; 00441 } 00442 } else { 00443 // get input url and then return the value 00444 if (changedFromOFF){ 00445 pc.printf("SENDING TO URL\n"); 00446 sprintf(url, "http://www.charlesding.net/kragl/lunchbox.php?isOpen=YES1&alarmOn=ON&boxLocked=NO"); 00447 00448 int ret = http.get(url, str, 128); 00449 pc.printf("Trying to Request %s\r\n", url); 00450 int tries = 20; 00451 while (tries > 0) { 00452 if (!ret) { 00453 pc.printf("Requested %s\r\n", url); 00454 pc.printf("Page fetched successfully - read %d characters\r\n", 00455 strlen(str)); 00456 pc.printf("Result: %s\r\n", str); 00457 break; 00458 } else { 00459 pc.printf("Error - ret = %d - HTTP return code = %d\r\n", 00460 ret, 00461 http.getHTTPResponseCode()); 00462 tries--; 00463 } 00464 ret = http.get(url, str, 128); 00465 } 00466 changedFromOFF = 0; 00467 } 00468 } 00469 //wait(0.3); 00470 } else if (!reedIsOpen && boxOpenLegal) { 00471 Buzzer = 0; 00472 // boxOpenLegal = false; 00473 00474 00475 //wait(0.3); 00476 } 00477 if (!codeEntered && passwordcounter==sizeof(code)/4) { 00478 codeEntered = true; 00479 00480 for (int j=0; j<sizeof(code)/4; j++) { 00481 if (code[j]!=password[j]) { 00482 codeEntered = false; 00483 } 00484 } 00485 if (num==1){ 00486 codeEntered = true; 00487 } 00488 if (codeEntered==true){ 00489 Buzzer = 0; 00490 warningLED = 0; 00491 for (int j=0; j<8; j++){ 00492 correctLED =1; 00493 wait(0.15); 00494 correctLED = 0; 00495 wait(0.15); 00496 } 00497 } else { 00498 correctLED = 0; 00499 for (int j=0; j<8; j++){ 00500 warningLED =1; 00501 wait(0.15); 00502 warningLED = 0; 00503 wait(0.15); 00504 } 00505 } 00506 00507 00508 pc.printf("CODE IS ENTERED\n"); 00509 passwordcounter = 0; 00510 00511 pc.printf("SENDING TO URL\n"); 00512 if (codeEntered==false){ 00513 sprintf(url, "http://www.charlesding.net/kragl/lunchbox.php?alarmOn=OFF&authorized=NO&openLock=2"); 00514 } else { 00515 sprintf(url, "http://www.charlesding.net/kragl/lunchbox.php?alarmOn=OFF&authorized=YES&openLock=1"); 00516 } 00517 00518 int ret = http.get(url, str, 128); 00519 pc.printf("Trying to Request %s\r\n", url); 00520 int tries = 50; 00521 while (tries > 0) { 00522 ret = http.get(url, str, 128); 00523 if (!ret) { 00524 pc.printf("Requested %s\r\n", url); 00525 pc.printf("Page fetched successfully - read %d characters\r\n", 00526 strlen(str)); 00527 pc.printf("Result: %s\r\n", str); 00528 break; 00529 } else { 00530 pc.printf("Error - ret = %d - HTTP return code = %d\r\n", 00531 ret, 00532 http.getHTTPResponseCode()); 00533 tries--; 00534 } 00535 } 00536 00537 00538 } 00539 //counterforpasswordcheck++; 00540 //pc.printf("C%d ", counterforpasswordcheck); 00541 } 00542 00543 00544 00545 00546 00547 }
Generated on Fri Jul 15 2022 11:29:35 by
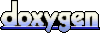