
Initial revision
Dependencies: HTTPClient PWM_Tone_Library PinDetect_KL25Z Queue cc3000_hostdriver_mbedsocket mbed
main.cpp
00001 /** 00002 * \brief CS294-84 demo \author Ben Zhang, Antonio Iannopollo 00003 * 00004 * This sampel code illustrates how to connect the mbed KL25Z platform to internet 00005 * thorugh the CC3000 wifi breakout board (http://www.adafruit.com/product/1469). 00006 * Connections between the KL25Z and the CC3000 are made according to the 00007 * guide at https://learn.adafruit.com/adafruit-cc3000-wifi -- KL25Z and arduino 00008 * UNO are pin to pin compatible -- 00009 * 00010 * This application uses the following libraries: 00011 * - cc3000_hostdriver_mbedsocket 00012 * (http://developer.mbed.org/users/Kojto/code/cc3000_hostdriver_mbedsocket/) 00013 * - HTTPClient (http://developer.mbed.org/users/donatien/code/HTTPClient/) 00014 */ 00015 00016 #include "mbed.h" 00017 #include "cc3000.h" 00018 #include "HTTPClient.h" 00019 #include "PinDetect.h" 00020 #include "pwm_tone.h" 00021 00022 // KL25Z wifi connection 00023 // we need to define connection pins for: 00024 // - IRQ => (pin D3) 00025 // - Enable => (pin D5) 00026 // - SPI CS => (pin D10) 00027 // - SPI MOSI => (pin D11) 00028 // - SPI MISO => (pin D12) 00029 // - SPI CLK => (pin D13) 00030 // plus wifi network SSID, password, security level and smart-configuration flag. 00031 mbed_cc3000::cc3000 wifi(D3, D5, D10, SPI(D11, D12, D13), 00032 "CalVisitor", "", NONE, false); 00033 00034 // create an http instance 00035 HTTPClient http; 00036 00037 // str is used to hold the response data 00038 char str[512]; 00039 char url[128]; 00040 char in[512]; 00041 char out[512]; 00042 00043 00044 // setup the serial connection, and LEDs 00045 Serial pc(USBTX, USBRX); 00046 DigitalOut led_red(LED_RED); 00047 DigitalOut led_green(LED_GREEN); 00048 00049 PinDetect p1(D4); 00050 PinDetect p2(D6); 00051 PinDetect p3(D7); 00052 PinDetect reed(D8); 00053 PwmOut Buzzer(D9); 00054 00055 int code[] = {0, 0, 0, 0}; 00056 int passwordcounter = 0; 00057 bool codeEntered = false; 00058 bool reedIsOpen = false; 00059 bool alarmOn = false; 00060 bool boxOpenLegal = false; 00061 int password[] = {3, 3, 1, 2}; 00062 float C_3 = 1000000/Do3, 00063 Cs_3 = 1000000/Do3s, 00064 D_3 = 1000000/Re3, 00065 Ds_3 = 1000000/Re3s, 00066 E_3 = 1000000/Mi3, 00067 F_3 = 1000000/Fa3, 00068 Fs_3 = 1000000/Fa3s, 00069 G_3 = 1000000/So3, 00070 Gs_3 = 1000000/So3s, 00071 A_3 = 1000000/La3, 00072 As_3 = 1000000/La3s, 00073 B_3 = 1000000/Ti3, 00074 C_4 = 1000000/Do4, 00075 Cs_4 = 1000000/Do4s, 00076 D_4 = 1000000/Re4, 00077 Ds_4 = 1000000/Re4s, 00078 E_4 = 1000000/Mi4, 00079 F_4 = 1000000/Fa4, 00080 Fs_4 = 1000000/Fa4s, 00081 G_4 = 1000000/So4, 00082 Gs_4 = 1000000/So4s, 00083 A_4 = 1000000/La4, 00084 As_4 = 1000000/La4s, 00085 B_4 = 1000000/Ti4, 00086 C_5 = 1000000/Do5, 00087 Cs_5 = 1000000/Do5s, 00088 D_5 = 1000000/Re5, 00089 Ds_5 = 1000000/Re5s, 00090 E_5 = 1000000/Mi5, 00091 F_5 = 1000000/Fa5, 00092 Fs_5 = 1000000/Fa5s, 00093 G_5 = 1000000/So5, 00094 Gs_5 = 1000000/So5s, 00095 A_5 = 1000000/La5, 00096 As_5 = 1000000/La5s, 00097 B_5 = 1000000/Ti5; 00098 00099 int tones[] = {E_4, D_4, C_4, D_4, E_4, E_4, E_4, 0, D_4, D_4, D_4, 0, E_4, G_4, G_4, 0, 00100 E_4, D_4, C_4, D_4, E_4, E_4, E_4, 0, D_4, D_4, E_4, D_4, C_4, 0, 0, 0 00101 }; 00102 int tones_num = 32; 00103 00104 //Queue buttonQ(4,4); 00105 00106 void key1Pressed( void ) 00107 { 00108 //pc.printf("Key Pressed\n"); 00109 int i = 1; 00110 code[passwordcounter] = i; 00111 passwordcounter++; 00112 //buttonQ.Put(&i); 00113 } 00114 00115 void key1Released( void ) 00116 { 00117 //pc.printf("Key Released\n"); 00118 } 00119 00120 void key1PressedHeld( void ) 00121 { 00122 //pc.printf("Key Pressed Held\n"); 00123 } 00124 00125 void key2Pressed( void ) 00126 { 00127 //pc.printf("Key Pressed\n"); 00128 int i = 2; 00129 code[passwordcounter] = i; 00130 passwordcounter++; 00131 //buttonQ.Put(&i); 00132 00133 } 00134 00135 void key2Released( void ) 00136 { 00137 //pc.printf("Key Released\n"); 00138 } 00139 00140 void key2PressedHeld( void) 00141 { 00142 //pc.printf("Key Pressed Held\n"); 00143 } 00144 00145 void key3Pressed( void ) 00146 { 00147 //pc.printf("Key Pressed\n"); 00148 int i = 3; 00149 code[passwordcounter] = i; 00150 passwordcounter++; 00151 //buttonQ.Put(&i); 00152 00153 } 00154 00155 void key3Released( void ) 00156 { 00157 //pc.printf("Key Released\n"); 00158 } 00159 00160 void key3PressedHeld( void ) 00161 { 00162 //pc.printf("Key Pressed Held\n"); 00163 } 00164 00165 void reedOpen( void ) 00166 { 00167 pc.printf("Reed Open\n"); 00168 reedIsOpen = 1; 00169 00170 } 00171 00172 void reedClosed( void ) 00173 { 00174 pc.printf("Reed Closed\n"); 00175 reedIsOpen = 0; 00176 } 00177 00178 void reedOpenHeld( void ) 00179 { 00180 //pc.printf("Key Pressed Held\n"); 00181 } 00182 00183 00184 int main() 00185 { 00186 // by default, it's red 00187 led_red = 0; 00188 led_green = 1; 00189 00190 // print message to indicate the program has started 00191 pc.printf("CC3000 Lunch Theft\r\n"); 00192 wifi.init(); 00193 00194 p1.mode(PullUp); 00195 p2.mode(PullUp); 00196 p3.mode(PullUp); 00197 reed.mode(PullUp); 00198 00199 p1.attach_asserted( &key1Pressed); 00200 p1.attach_deasserted( &key1Released); 00201 p1.attach_asserted_held( &key1PressedHeld); 00202 p1.setAssertValue( 0 ); 00203 p1.setSampleFrequency( 10000 ); 00204 00205 p2.attach_asserted( &key2Pressed); 00206 p2.attach_deasserted( &key2Released); 00207 p2.attach_asserted_held( &key2PressedHeld); 00208 p2.setAssertValue( 0 ); 00209 p2.setSampleFrequency( 10000 ); 00210 00211 p3.attach_asserted( &key3Pressed); 00212 p3.attach_deasserted( &key3Released); 00213 p3.attach_asserted_held( &key3PressedHeld); 00214 p3.setAssertValue( 0 ); 00215 p3.setSampleFrequency( 10000 ); 00216 00217 reed.attach_asserted( &reedClosed); 00218 reed.attach_deasserted( &reedOpen); 00219 reed.attach_asserted_held( &reedOpenHeld); 00220 reed.setAssertValue( 0 ); 00221 reed.setSampleFrequency( 10000 ); 00222 00223 Tune(Buzzer, C_4, 4); //4 Octave C beat 4/16 00224 wait_ms(250); 00225 Tune(Buzzer, D_4, 4); //4 Octave D beat 4/16 00226 wait_ms(250); 00227 Tune(Buzzer, E_4, 4); //4 Octave E beat 4/16 00228 wait_ms(250); 00229 00230 int i; 00231 int init = 0; // init wifi done 00232 00233 00234 while (1) { 00235 if (init == 0) { 00236 if(wifi.is_connected() == false) { 00237 // try to connect 00238 if (wifi.connect() == -1) { 00239 pc.printf("Failed to connect." 00240 "Please verify connection details and try again.\r\n"); 00241 } else { 00242 pc.printf("IP address: %s \r\n", wifi.getIPAddress()); 00243 00244 //once connected, turn green LED on and red LED off 00245 led_red = 1; 00246 led_green = 0; 00247 } 00248 } else { 00249 sprintf(url, "http://www.charlesding.net/kragl/lunchbox.php?isOpen=0&alarmOn=0"); 00250 int ret = http.get(url, str, 128); 00251 init = 1; 00252 int tries = 5; 00253 while (ret && tries > 0) { 00254 ret = http.get(url, str, 128); 00255 if (!ret) { 00256 pc.printf("Requested %s\r\n", url); 00257 pc.printf("Page fetched successfully - read %d characters\r\n", 00258 strlen(str)); 00259 pc.printf("Result: %s\r\n", str); 00260 } else { 00261 pc.printf("Error - ret = %d - HTTP return code = %d\r\n", 00262 ret, 00263 http.getHTTPResponseCode()); 00264 } 00265 tries--; 00266 } 00267 00268 } 00269 } 00270 00271 if (reedIsOpen && codeEntered) { 00272 pc.printf("LEGAL OPENING"); 00273 boxOpenLegal = true; 00274 codeEntered = false; 00275 wait(0.3); 00276 } else if (reedIsOpen && !boxOpenLegal) { 00277 // turn on alarm; 00278 pc.printf("ALARM TIME\n"); 00279 for(i=0; i<tones_num; i++) { 00280 Auto_tunes(Buzzer, tones[i], 4); // Auto performance 00281 Stop_tunes(Buzzer); 00282 } 00283 boxOpenLegal = false; 00284 00285 if(wifi.is_connected() == false) { 00286 // try to connect 00287 if (wifi.connect() == -1) { 00288 pc.printf("Failed to connect." 00289 "Please verify connection details and try again.\r\n"); 00290 } else { 00291 pc.printf("IP address: %s \r\n", wifi.getIPAddress()); 00292 00293 //once connected, turn green LED on and red LED off 00294 led_red = 1; 00295 led_green = 0; 00296 } 00297 } else { 00298 // get input url and then return the value 00299 pc.printf("SENDING TO URL\n"); 00300 sprintf(url, "http://www.charlesding.net/kragl/lunchbox.php?isOpen=1&alarmOn=1"); 00301 00302 int ret = http.get(url, str, 128); 00303 pc.printf("Trying to Request %s\r\n", url); 00304 int tries = 5; 00305 while (ret && tries > 0) { 00306 if (!ret) { 00307 pc.printf("Requested %s\r\n", url); 00308 pc.printf("Page fetched successfully - read %d characters\r\n", 00309 strlen(str)); 00310 pc.printf("Result: %s\r\n", str); 00311 } else { 00312 pc.printf("Error - ret = %d - HTTP return code = %d\r\n", 00313 ret, 00314 http.getHTTPResponseCode()); 00315 tries--; 00316 } 00317 } 00318 } 00319 00320 00321 wait(0.3); 00322 } else if (!reedIsOpen && boxOpenLegal) { 00323 boxOpenLegal = false; 00324 wait(0.3); 00325 } 00326 if (passwordcounter==sizeof(code)/4) { 00327 codeEntered = true; 00328 for (int j=0; j<sizeof(code)/4; j++) { 00329 if (code[j]!=password[j]) { 00330 codeEntered = false; 00331 } 00332 } 00333 00334 00335 pc.printf("CODE IS ENTERED\n"); 00336 passwordcounter = 0; 00337 00338 pc.printf("SENDING TO URL\n"); 00339 sprintf(url, "http://www.charlesding.net/kragl/lunchbox.php?isOpen=0&alarmOn=0"); 00340 00341 int ret = http.get(url, str, 128); 00342 pc.printf("Trying to Request %s\r\n", url); 00343 int tries = 5; 00344 while (ret && tries > 0) { 00345 if (!ret) { 00346 pc.printf("Requested %s\r\n", url); 00347 pc.printf("Page fetched successfully - read %d characters\r\n", 00348 strlen(str)); 00349 pc.printf("Result: %s\r\n", str); 00350 } else { 00351 pc.printf("Error - ret = %d - HTTP return code = %d\r\n", 00352 ret, 00353 http.getHTTPResponseCode()); 00354 tries--; 00355 } 00356 } 00357 00358 00359 } 00360 00361 } 00362 00363 }
Generated on Wed Jul 13 2022 11:33:23 by
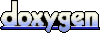