3/26/16 12:25 am JJ
Dependents: steppertest R5 2016 Robotics Team 1
Fork of scanner by
scanner.h
00001 #ifndef SCANNER_H 00002 #define SCANNER_H 00003 #include "mbed.h" 00004 #include "LongRangeSensor.h" 00005 #include "ShortRangeSensor.h" 00006 #include "StepperDrive.h" 00007 #include "Gripper.h" 00008 #include <stack> 00009 00010 class Scanner 00011 { 00012 public: 00013 Scanner(Serial &pc1, StepperDrive &_drive, PinName _servoL, PinName _servoR, 00014 ShortRangeSensor &_shortRangeL, ShortRangeSensor &_shortRangeR, 00015 LongRangeSensor &_longRangeL, LongRangeSensor &_longRangeR, 00016 Gripper &_robotGrip, float _period = 0.2, ColorSensor colorSensor); 00017 void huntMode(); 00018 void hunt(); 00019 void avoidMode(); 00020 void localize(); 00021 void localizeRight(); 00022 void localizeLeft(); 00023 void localizeRightMode(); 00024 void localizeLeftMode(); 00025 float getDistLeft() {return distLeft;} 00026 float getDistRight() {return distRight;} 00027 float getDistForwardL() {return distForwardL;} 00028 float getDistForwardR() {return distForwardR;} 00029 void setLocalizeRightFlag(bool value) {localizeRightFlag = value;} 00030 void setLocalizeLeftFlag(bool value) {localizeLeftFlag = value;} 00031 bool getYellowFlag() {return yellowFlag;} 00032 bool getObjectFound() {return objectFound;} 00033 00034 private: 00035 static const int YELLOW = 1; 00036 static const int RED = 0; 00037 static const int MIN_DUTY = 0; 00038 static const int MAX_DUTY = 6; 00039 static const int HALF_DUTY = 3; 00040 static const float DELTA_DUTY = 4.2e-3; 00041 float DUTY[13]; // {0.0500, 0.0542, 0.0583, 0.0625, 0.0667, 0.0708, 00042 // 0.0750, 0.0792, 0.0833, 0.0875, 0.0917, 0.0958, 00043 // 0.1000}; 00044 bool pitEnable; 00045 int invertL; 00046 int invertR; 00047 int dutyL; 00048 int dutyR; 00049 float distLeft; 00050 float distRight; 00051 float distForwardL; 00052 float distForwardR; 00053 Serial &pc; 00054 StepperDrive &drive; 00055 PwmOut servoL; 00056 PwmOut servoR; 00057 ShortRangeSensor &shortRangeL; 00058 ShortRangeSensor &shortRangeR; 00059 LongRangeSensor &longRangeL; 00060 LongRangeSensor &longRangeR; 00061 Gripper &robotGrip; 00062 float period; 00063 bool obstacle; 00064 bool huntFlag; 00065 bool avoidFlag; 00066 bool objectFound; 00067 bool localizeRightFlag; 00068 bool localizeLeftFlag; 00069 bool yellowFlag; 00070 00071 struct huntMove 00072 { 00073 float distance; 00074 float angle; 00075 }; 00076 00077 Ticker scanPit; // periodic interrupt timer 00078 00079 void scan(); 00080 00081 std::stack<huntMove> myStack; 00082 }; 00083 00084 #endif // SCANNER_H
Generated on Fri Jul 15 2022 05:45:51 by
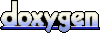