2.7V 4-Channel 12-Bit A/D Converters with SPI™ Serial Interface
Embed:
(wiki syntax)
Show/hide line numbers
MCP3204.h
00001 /* mbed MCP3204 Library, for driving the 2.7V 4-Channel/8-Channel 12-Bit A/D Converters with SPI™ Serial Interface 00002 * Copyright (c) 2015, Created by Steen Joergensen (stjo2809) 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy 00005 * of this software and associated documentation files (the "Software"), to deal 00006 * in the Software without restriction, including without limitation the rights 00007 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 * copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in 00012 * all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 * THE SOFTWARE. 00021 */ 00022 00023 #include "mbed.h" 00024 00025 #ifndef MBED_MCP3204_H 00026 #define MBED_MCP3204_H 00027 00028 //============================================================================= 00029 // Declaration of variables & custom #defines 00030 //============================================================================= 00031 00032 #define CH0 0x00 // Channel 0 for single and for differential is CH0 = IN+ and CH1 = IN- 00033 #define CH1 0x40 // Channel 1 for single and for differential is CH0 = IN- and CH1 = IN+ 00034 #define CH2 0x80 // Channel 2 for single and for differential is CH2 = IN+ and CH3 = IN- 00035 #define CH3 0xC0 // Channel 3 for single and for differential is CH2 = IN- and CH3 = IN+ 00036 #define SINGLE 0x06 // Single command 00037 #define DIFFERENTIAL 0x04 // Differential command 00038 00039 //============================================================================= 00040 // Functions Declaration 00041 //============================================================================= 00042 00043 /** Interface to the 4-Channel 12-Bit A/D Converters with SPI interface 00044 * 00045 * Using the driver: 00046 * - remenber to setup SPI in main routine or use pins instance. 00047 * 00048 * Defaults in this driver on start up: 00049 * - Datasheet start up 00050 */ 00051 class MCP3204 { 00052 public: 00053 /** Create an instance of the MCP3204 connected via specfied SPI instance. 00054 * 00055 * @param spi The mbed SPI instance (make in main routine) 00056 * @param nCs The SPI chip select pin. 00057 */ 00058 MCP3204(SPI& spi, PinName nCs); 00059 00060 /** Create an instance of the MCP4261 connected with SPI pins. 00061 * 00062 * @param mosi The SPI Master Output, Slave Input pin. 00063 * @param miso The SPI Master Input, Slave Output pin. 00064 * @param sck The SPI Serial Clock pin. 00065 * @param nCs The SPI chip select pin. 00066 */ 00067 MCP3204(PinName mosi, PinName miso,PinName sck, PinName nCs); 00068 00069 00070 /** Read from single channel. 00071 * 00072 * use defines to simplify use of function ( CH0, CH1, CH2, CH3) 00073 * @param channel The channel to receive the 12 bits value from. 00074 */ 00075 int sgl(char channel); 00076 00077 /** Read from differential channel. 00078 * 00079 * use defines to simplify use of function ( CH0, CH1, CH2, CH3) 00080 * @param channel The channel to receive the 12 bits value from. 00081 */ 00082 int diff(char channel); 00083 00084 00085 private: 00086 SPI& _spi; 00087 DigitalOut _nCs; 00088 00089 int _make_value_from_responce(int responce_msb, int responce_lsb); 00090 int _read(char sgl_or_diff, char channel); 00091 00092 }; 00093 00094 #endif
Generated on Sat Jul 16 2022 12:35:19 by
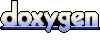