RadioHead
RH_RF95 Class Reference
Driver to send and receive unaddressed, unreliable datagrams via a LoRa capable radio transceiver. More...
#include <RH_RF95.h>
Inherits RHGenericDriver.
Data Structures | |
struct | ModemConfig |
Defines register values for a set of modem configuration registers. More... | |
Public Types | |
enum | ModemConfigChoice { Bw125Cr45Sf128 = 0, Bw500Cr45Sf128, Bw31_25Cr48Sf512, Bw125Cr48Sf4096 } |
Choices for setModemConfig() for a selected subset of common data rates. More... | |
enum | RHMode { RHModeInitialising = 0, RHModeSleep, RHModeIdle, RHModeTx, RHModeRx, RHModeCad } |
Defines different operating modes for the transport hardware. More... | |
Public Member Functions | |
RH_RF95 (swspi &spi, int ssNum) | |
Constructor. | |
virtual bool | init () |
Initialise the Driver transport hardware and software. | |
bool | printRegisters () |
Prints the value of all chip registers to the Serial device if RH_HAVE_SERIAL is defined for the current platform For debugging purposes only. | |
void | setModemRegisters (const ModemConfig *config) |
Sets all the registered required to configure the data modem in the RF95/96/97/98, including the bandwidth, spreading factor etc. | |
bool | setModemConfig (ModemConfigChoice index) |
Select one of the predefined modem configurations. | |
virtual bool | available () |
Tests whether a new message is available from the Driver. | |
virtual bool | recv (uint8_t *buf, uint8_t *len) |
Turns the receiver on if it not already on. | |
virtual bool | send (const uint8_t *data, uint8_t len) |
Waits until any previous transmit packet is finished being transmitted with waitPacketSent(). | |
void | setPreambleLength (uint16_t bytes) |
Sets the length of the preamble in bytes. | |
virtual uint8_t | maxMessageLength () |
Returns the maximum message length available in this Driver. | |
bool | setFrequency (float centre) |
Sets the transmitter and receiver centre frequency. | |
void | setModeIdle () |
If current mode is Rx or Tx changes it to Idle. | |
void | setModeRx () |
If current mode is Tx or Idle, changes it to Rx. | |
void | setModeTx () |
If current mode is Rx or Idle, changes it to Rx. | |
void | setTxPower (int8_t power, bool useRFO=false) |
Sets the transmitter power output level, and configures the transmitter pin. | |
virtual bool | sleep () |
Sets the radio into low-power sleep mode. | |
virtual bool | isChannelActive () |
Use the radio's Channel Activity Detect (CAD) function to detect channel activity. | |
void | enableTCXO () |
Enable TCXO mode Call this immediately after init(), to force your radio to use an external frequency source, such as a Temperature Compensated Crystal Oscillator (TCXO). | |
int | frequencyError () |
Returns the last measured frequency error. | |
int | lastSNR () |
Returns the Signal-to-noise ratio (SNR) of the last received message, as measured by the receiver. | |
void | handleInterrupt () |
This is a low level function to handle the interrupts for one instance of RH_RF95. | |
virtual void | waitAvailable () |
Starts the receiver and blocks until a valid received message is available. | |
virtual bool | waitPacketSent () |
Blocks until the transmitter is no longer transmitting. | |
virtual bool | waitPacketSent (uint16_t timeout) |
Blocks until the transmitter is no longer transmitting. | |
virtual bool | waitAvailableTimeout (uint16_t timeout) |
Starts the receiver and blocks until a received message is available or a timeout. | |
virtual bool | waitCAD () |
Channel Activity Detection (CAD). | |
void | setCADTimeout (unsigned long cad_timeout) |
Sets the Channel Activity Detection timeout in milliseconds to be used by waitCAD(). | |
virtual void | setThisAddress (uint8_t thisAddress) |
Sets the address of this node. | |
virtual void | setHeaderTo (uint8_t to) |
Sets the TO header to be sent in all subsequent messages. | |
virtual void | setHeaderFrom (uint8_t from) |
Sets the FROM header to be sent in all subsequent messages. | |
virtual void | setHeaderId (uint8_t id) |
Sets the ID header to be sent in all subsequent messages. | |
virtual void | setHeaderFlags (uint8_t set, uint8_t clear=RH_FLAGS_APPLICATION_SPECIFIC) |
Sets and clears bits in the FLAGS header to be sent in all subsequent messages First it clears he FLAGS according to the clear argument, then sets the flags according to the set argument. | |
virtual void | setPromiscuous (bool promiscuous) |
Tells the receiver to accept messages with any TO address, not just messages addressed to thisAddress or the broadcast address. | |
virtual uint8_t | headerTo () |
Returns the TO header of the last received message. | |
virtual uint8_t | headerFrom () |
Returns the FROM header of the last received message. | |
virtual uint8_t | headerId () |
Returns the ID header of the last received message. | |
virtual uint8_t | headerFlags () |
Returns the FLAGS header of the last received message. | |
int8_t | lastRssi () |
Returns the most recent RSSI (Receiver Signal Strength Indicator). | |
RHMode | mode () |
Returns the operating mode of the library. | |
void | setMode (RHMode mode) |
Sets the operating mode of the transport. | |
uint16_t | rxBad () |
Returns the count of the number of bad received packets (ie packets with bad lengths, checksum etc) which were rejected and not delivered to the application. | |
uint16_t | rxGood () |
Returns the count of the number of good received packets. | |
uint16_t | txGood () |
Returns the count of the number of packets successfully transmitted (though not necessarily received by the destination) | |
Static Public Member Functions | |
static void | printBuffer (const char *prompt, const uint8_t *buf, uint8_t len) |
Prints a data buffer in HEX. | |
Protected Member Functions | |
void | validateRxBuf () |
Examine the revceive buffer to determine whether the message is for this node. | |
void | clearRxBuf () |
Clear our local receive buffer. | |
Protected Attributes | |
volatile RHMode | _mode |
The current transport operating mode. | |
uint8_t | _thisAddress |
This node id. | |
bool | _promiscuous |
Whether the transport is in promiscuous mode. | |
volatile uint8_t | _rxHeaderTo |
TO header in the last received mesasge. | |
volatile uint8_t | _rxHeaderFrom |
FROM header in the last received mesasge. | |
volatile uint8_t | _rxHeaderId |
ID header in the last received mesasge. | |
volatile uint8_t | _rxHeaderFlags |
FLAGS header in the last received mesasge. | |
uint8_t | _txHeaderTo |
TO header to send in all messages. | |
uint8_t | _txHeaderFrom |
FROM header to send in all messages. | |
uint8_t | _txHeaderId |
ID header to send in all messages. | |
uint8_t | _txHeaderFlags |
FLAGS header to send in all messages. | |
volatile int8_t | _lastRssi |
The value of the last received RSSI value, in some transport specific units. | |
volatile uint16_t | _rxBad |
Count of the number of bad messages (eg bad checksum etc) received. | |
volatile uint16_t | _rxGood |
Count of the number of successfully transmitted messaged. | |
volatile uint16_t | _txGood |
Count of the number of bad messages (correct checksum etc) received. | |
volatile bool | _cad |
Channel activity detected. | |
unsigned int | _cad_timeout |
Channel activity timeout in ms. |
Detailed Description
Driver to send and receive unaddressed, unreliable datagrams via a LoRa capable radio transceiver.
For Semtech SX1276/77/78/79 and HopeRF RF95/96/97/98 and other similar LoRa capable radios. Based on http://www.hoperf.com/upload/rf/RFM95_96_97_98W.pdf and http://www.hoperf.cn/upload/rfchip/RF96_97_98.pdf and http://www.semtech.com/images/datasheet/LoraDesignGuide_STD.pdf and http://www.semtech.com/images/datasheet/sx1276.pdf and http://www.semtech.com/images/datasheet/sx1276_77_78_79.pdf FSK/GFSK/OOK modes are not (yet) supported.
Works with
- the excellent MiniWirelessLoRa from Anarduino http://www.anarduino.com/miniwireless
- The excellent Modtronix inAir4 http://modtronix.com/inair4.html and inAir9 modules http://modtronix.com/inair9.html.
- the excellent Rocket Scream Mini Ultra Pro with the RFM95W http://www.rocketscream.com/blog/product/mini-ultra-pro-with-radio/
- Lora1276 module from NiceRF http://www.nicerf.com/product_view.aspx?id=99
- Adafruit Feather M0 with RFM95
- Overview
This class provides basic functions for sending and receiving unaddressed, unreliable datagrams of arbitrary length to 251 octets per packet.
Manager classes may use this class to implement reliable, addressed datagrams and streams, mesh routers, repeaters, translators etc.
Naturally, for any 2 radios to communicate that must be configured to use the same frequency and modulation scheme.
This Driver provides an object-oriented interface for sending and receiving data messages with Hope-RF RFM95/96/97/98(W), Semtech SX1276/77/78/79 and compatible radio modules in LoRa mode.
The Hope-RF (http://www.hoperf.com) RFM95/96/97/98(W) and Semtech SX1276/77/78/79 is a low-cost ISM transceiver chip. It supports FSK, GFSK, OOK over a wide range of frequencies and programmable data rates, and it also supports the proprietary LoRA (Long Range) mode, which is the only mode supported in this RadioHead driver.
This Driver provides functions for sending and receiving messages of up to 251 octets on any frequency supported by the radio, in a range of predefined Bandwidths, Spreading Factors and Coding Rates. Frequency can be set with 61Hz precision to any frequency from 240.0MHz to 960.0MHz. Caution: most modules only support a more limited range of frequencies due to antenna tuning.
Up to 2 modules can be connected to an Arduino (3 on a Mega), permitting the construction of translators and frequency changers, etc.
Support for other features such as transmitter power control etc is also provided.
Tested on MinWirelessLoRa with arduino-1.0.5 on OpenSuSE 13.1. Also tested with Teensy3.1, Modtronix inAir4 and Arduino 1.6.5 on OpenSuSE 13.1
- Packet Format
All messages sent and received by this RH_RF95 Driver conform to this packet format:
- LoRa mode:
- 8 symbol PREAMBLE
- Explicit header with header CRC (handled internally by the radio)
- 4 octets HEADER: (TO, FROM, ID, FLAGS)
- 0 to 251 octets DATA
- CRC (handled internally by the radio)
- Connecting RFM95/96/97/98 and Semtech SX1276/77/78/79 to Arduino
We tested with Anarduino MiniWirelessLoRA, which is an Arduino Duemilanove compatible with a RFM96W module on-board. Therefore it needs no connections other than the USB programming connection and an antenna to make it work.
If you have a bare RFM95/96/97/98 that you want to connect to an Arduino, you might use these connections (untested): CAUTION: you must use a 3.3V type Arduino, otherwise you will also need voltage level shifters between the Arduino and the RFM95. CAUTION, you must also ensure you connect an antenna.
Arduino RFM95/96/97/98 GND----------GND (ground in) 3V3----------3.3V (3.3V in) interrupt 0 pin D2-----------DIO0 (interrupt request out) SS pin D10----------NSS (CS chip select in) SCK pin D13----------SCK (SPI clock in) MOSI pin D11----------MOSI (SPI Data in) MISO pin D12----------MISO (SPI Data out)
With these connections, you can then use the default constructor RH_RF95(). You can override the default settings for the SS pin and the interrupt in the RH_RF95 constructor if you wish to connect the slave select SS to other than the normal one for your Arduino (D10 for Diecimila, Uno etc and D53 for Mega) or the interrupt request to other than pin D2 (Caution, different processors have different constraints as to the pins available for interrupts).
You can connect a Modtronix inAir4 or inAir9 directly to a 3.3V part such as a Teensy 3.1 like this (tested).
Teensy inAir4 inAir9 GND----------GND (ground in) 3V3----------3.3V (3.3V in) interrupt 0 pin D2-----------D00 (interrupt request out) SS pin D10----------CS (CS chip select in) SCK pin D13----------CK (SPI clock in) MOSI pin D11----------SI (SPI Data in) MISO pin D12----------SO (SPI Data out)
With these connections, you can then use the default constructor RH_RF95(). you must also set the transmitter power with useRFO: driver.setTxPower(13, true);
Note that if you are using Modtronix inAir4 or inAir9,or any other module which uses the transmitter RFO pins and not the PA_BOOST pins that you must configure the power transmitter power for -1 to 14 dBm and with useRFO true. Failure to do that will result in extremely low transmit powers.
If you have an Arduino M0 Pro from arduino.org, you should note that you cannot use Pin 2 for the interrupt line (Pin 2 is for the NMI only). The same comments apply to Pin 4 on Arduino Zero from arduino.cc. Instead you can use any other pin (we use Pin 3) and initialise RH_RF69 like this:
// Slave Select is pin 10, interrupt is Pin 3 RH_RF95 driver(10, 3);
If you have a Rocket Scream Mini Ultra Pro with the RFM95W:
- Ensure you have Arduino SAMD board support 1.6.5 or later in Arduino IDE 1.6.8 or later.
- The radio SS is hardwired to pin D5 and the DIO0 interrupt to pin D2, so you need to initialise the radio like this:
RH_RF95 driver(5, 2);
- The name of the serial port on that board is 'SerialUSB', not 'Serial', so this may be helpful at the top of our sample sketches:
#define Serial SerialUSB
- You also need this in setup before radio initialisation
// Ensure serial flash is not interfering with radio communication on SPI bus pinMode(4, OUTPUT); digitalWrite(4, HIGH);
- and if you have a 915MHz part, you need this after driver/manager intitalisation: which adds up to modifying sample sketches something like:
rf95.setFrequency(915.0);
#include <SPI.h> #include <RH_RF95.h> RH_RF95 rf95(5, 2); // Rocket Scream Mini Ultra Pro with the RFM95W #define Serial SerialUSB void setup() { // Ensure serial flash is not interfering with radio communication on SPI bus pinMode(4, OUTPUT); digitalWrite(4, HIGH); Serial.begin(9600); while (!Serial) ; // Wait for serial port to be available if (!rf95.init()) Serial.println("init failed"); rf95.setFrequency(915.0); } ...
For Adafruit Feather M0 with RFM95, construct the driver like this:
RH_RF95 rf95(8, 3);
It is possible to have 2 or more radios connected to one Arduino, provided each radio has its own SS and interrupt line (SCK, SDI and SDO are common to all radios)
Caution: on some Arduinos such as the Mega 2560, if you set the slave select pin to be other than the usual SS pin (D53 on Mega 2560), you may need to set the usual SS pin to be an output to force the Arduino into SPI master mode.
Caution: Power supply requirements of the RFM module may be relevant in some circumstances: RFM95/96/97/98 modules are capable of pulling 120mA+ at full power, where Arduino's 3.3V line can give 50mA. You may need to make provision for alternate power supply for the RFM module, especially if you wish to use full transmit power, and/or you have other shields demanding power. Inadequate power for the RFM is likely to cause symptoms such as:
- reset's/bootups terminate with "init failed" messages
- random termination of communication after 5-30 packets sent/received
- "fake ok" state, where initialization passes fluently, but communication doesn't happen
- shields hang Arduino boards, especially during the flashing
- Interrupts
The RH_RF95 driver uses interrupts to react to events in the RFM module, such as the reception of a new packet, or the completion of transmission of a packet. The RH_RF95 driver interrupt service routine reads status from and writes data to the the RFM module via the SPI interface. It is very important therefore, that if you are using the RH_RF95 driver with another SPI based deviced, that you disable interrupts while you transfer data to and from that other device. Use cli() to disable interrupts and sei() to reenable them.
- Memory
The RH_RF95 driver requires non-trivial amounts of memory. The sample programs all compile to about 8kbytes each, which will fit in the flash proram memory of most Arduinos. However, the RAM requirements are more critical. Therefore, you should be vary sparing with RAM use in programs that use the RH_RF95 driver.
It is often hard to accurately identify when you are hitting RAM limits on Arduino. The symptoms can include:
- Mysterious crashes and restarts
- Changes in behaviour when seemingly unrelated changes are made (such as adding print() statements)
- Hanging
- Output from Serial.print() not appearing
- Range
We have made some simple range tests under the following conditions:
- rf95_client base station connected to a VHF discone antenna at 8m height above ground
- rf95_server mobile connected to 17.3cm 1/4 wavelength antenna at 1m height, no ground plane.
- Both configured for 13dBm, 434MHz, Bw = 125 kHz, Cr = 4/8, Sf = 4096chips/symbol, CRC on. Slow+long range
- Minimum reported RSSI seen for successful comms was about -91
- Range over flat ground through heavy trees and vegetation approx 2km.
- At 20dBm (100mW) otherwise identical conditions approx 3km.
- At 20dBm, along salt water flat sandy beach, 3.2km.
It should be noted that at this data rate, a 12 octet message takes 2 seconds to transmit.
At 20dBm (100mW) with Bw = 125 kHz, Cr = 4/5, Sf = 128chips/symbol, CRC on. (Default medium range) in the conditions described above.
- Range over flat ground through heavy trees and vegetation approx 2km.
Caution: the performance of this radio, especially with narrow bandwidths is strongly dependent on the accuracy and stability of the chip clock. HopeRF and Semtech do not appear to recommend bandwidths of less than 62.5 kHz unless you have the optional Temperature Compensated Crystal Oscillator (TCXO) installed and enabled on your radio module. See the refernece manual for more data. Also https://lowpowerlab.com/forum/rf-range-antennas-rfm69-library/lora-library-experiences-range/15/ and http://www.semtech.com/images/datasheet/an120014-xo-guidance-lora-modulation.pdf
- Transmitter Power
You can control the transmitter power on the RF transceiver with the RH_RF95::setTxPower() function. The argument can be any of +5 to +23 (for modules that use PA_BOOST) -1 to +14 (for modules that use RFO transmitter pin) The default is 13. Eg:
driver.setTxPower(10); // use PA_BOOST transmitter pin driver.setTxPower(10, true); // use PA_RFO pin transmitter pin
We have made some actual power measurements against programmed power for Anarduino MiniWirelessLoRa (which has RFM96W-433Mhz installed)
- MiniWirelessLoRa RFM96W-433Mhz, USB power
- 30cm RG316 soldered direct to RFM96W module ANT and GND
- SMA connector
- 12db attenuator
- SMA connector
- MiniKits AD8307 HF/VHF Power Head (calibrated against Rohde&Schwartz 806.2020 test set)
- Tektronix TDS220 scope to measure the Vout from power head
Program power Measured Power dBm dBm 5 5 7 7 9 8 11 11 13 13 15 15 17 16 19 18 20 20 21 21 22 22 23 23
We have also measured the actual power output from a Modtronix inAir4 http://modtronix.com/inair4.html connected to a Teensy 3.1: Teensy 3.1 this is a 3.3V part, connected directly to: Modtronix inAir4 with SMA antenna connector, connected as above: 10cm SMA-SMA cable
- MiniKits AD8307 HF/VHF Power Head (calibrated against Rohde&Schwartz 806.2020 test set)
- Tektronix TDS220 scope to measure the Vout from power head (Caution: we dont claim laboratory accuracy for these power measurements) You would not expect to get anywhere near these powers to air with a simple 1/4 wavelength wire antenna.
Program power Measured Power dBm dBm -1 0 1 2 3 4 5 7 7 10 9 13 11 14.2 13 15 14 16
Definition at line 546 of file RH_RF95.h.
Member Enumeration Documentation
enum ModemConfigChoice |
Choices for setModemConfig() for a selected subset of common data rates.
If you need another configuration, determine the necessary settings and call setModemRegisters() with your desired settings. It might be helpful to use the LoRa calculator mentioned in http://www.semtech.com/images/datasheet/LoraDesignGuide_STD.pdf These are indexes into MODEM_CONFIG_TABLE. We strongly recommend you use these symbolic definitions and not their integer equivalents: its possible that new values will be introduced in later versions (though we will try to avoid it). Caution: if you are using slow packet rates and long packets with RHReliableDatagram or subclasses you may need to change the RHReliableDatagram timeout for reliable operations. Caution: for some slow rates nad with ReliableDatagrams youi may need to increase the reply timeout with manager.setTimeout() to deal with the long transmission times.
- Enumerator:
enum RHMode [inherited] |
Defines different operating modes for the transport hardware.
These are the different values that can be adopted by the _mode variable and returned by the mode() member function,
- Enumerator:
RHModeInitialising Transport is initialising. Initial default value until init() is called..
RHModeSleep Transport hardware is in low power sleep mode (if supported)
RHModeIdle Transport is idle.
RHModeTx Transport is in the process of transmitting a message.
RHModeRx Transport is in the process of receiving a message.
RHModeCad Transport is in the process of detecting channel activity (if supported)
Definition at line 54 of file RHGenericDriver.h.
Constructor & Destructor Documentation
RH_RF95 | ( | swspi & | spi, |
int | ssNum | ||
) |
Constructor.
You can have multiple instances, but each instance must have its own interrupt and slave select pin. After constructing, you must call init() to initialise the interface and the radio module. A maximum of 3 instances can co-exist on one processor, provided there are sufficient distinct interrupt lines, one for each instance.
- Parameters:
-
[in] slaveSelectPin the Arduino pin number of the output to use to select the RH_RF22 before accessing it. Defaults to the normal SS pin for your Arduino (D10 for Diecimila, Uno etc, D53 for Mega, D10 for Maple) [in] interruptPin The interrupt Pin number that is connected to the RFM DIO0 interrupt line. Defaults to pin 2, as required by Anarduino MinWirelessLoRa module. Caution: You must specify an interrupt capable pin. On many Arduino boards, there are limitations as to which pins may be used as interrupts. On Leonardo pins 0, 1, 2 or 3. On Mega2560 pins 2, 3, 18, 19, 20, 21. On Due and Teensy, any digital pin. On Arduino Zero from arduino.cc, any digital pin other than 4. On Arduino M0 Pro from arduino.org, any digital pin other than 2. On other Arduinos pins 2 or 3. See http://arduino.cc/en/Reference/attachInterrupt for more details. On Chipkit Uno32, pins 38, 2, 7, 8, 35. On other boards, any digital pin may be used. [in] spi Pointer to the SPI interface object to use. [in] ssNum the swspi slave select number to use
Definition at line 23 of file RH_RF95.cpp.
Member Function Documentation
bool available | ( | ) | [virtual] |
Tests whether a new message is available from the Driver.
On most drivers, this will also put the Driver into RHModeRx mode until a message is actually received by the transport, when it wil be returned to RHModeIdle. This can be called multiple times in a timeout loop
- Returns:
- true if a new, complete, error-free uncollected message is available to be retreived by recv()
Implements RHGenericDriver.
Definition at line 151 of file RH_RF95.cpp.
void clearRxBuf | ( | ) | [protected] |
Clear our local receive buffer.
Definition at line 160 of file RH_RF95.cpp.
void enableTCXO | ( | ) |
Enable TCXO mode Call this immediately after init(), to force your radio to use an external frequency source, such as a Temperature Compensated Crystal Oscillator (TCXO).
See the comments in the main documentation about the sensitivity of this radio to clock frequency especially when using narrow bandwidths. Leaves the module in sleep mode. Caution, this function has not been tested by us.
Definition at line 363 of file RH_RF95.cpp.
int frequencyError | ( | ) |
Returns the last measured frequency error.
The LoRa receiver estimates the frequency offset between the receiver centre frequency and that of the received LoRa signal. This function returns the estimates offset (in Hz) of the last received message. Caution: this measurement is not absolute, but is measured relative to the local receiver's oscillator. Apparent errors may be due to the transmitter, the receiver or both.
- Returns:
- The estimated centre frequency offset in Hz of the last received message. If the modem bandwidth selector in register RH_RF95_REG_1D_MODEM_CONFIG1 is invalid, returns 0.
Definition at line 374 of file RH_RF95.cpp.
void handleInterrupt | ( | ) |
This is a low level function to handle the interrupts for one instance of RH_RF95.
Must be called from user code in mbed because the swspi library mutex cannot be executed in an ISR. It should be executed when the ISR pin is seen low.
Definition at line 75 of file RH_RF95.cpp.
uint8_t headerFlags | ( | ) | [virtual, inherited] |
Returns the FLAGS header of the last received message.
- Returns:
- The FLAGS header
Definition at line 163 of file RHGenericDriver.cpp.
uint8_t headerFrom | ( | ) | [virtual, inherited] |
Returns the FROM header of the last received message.
- Returns:
- The FROM header
Definition at line 153 of file RHGenericDriver.cpp.
uint8_t headerId | ( | ) | [virtual, inherited] |
Returns the ID header of the last received message.
- Returns:
- The ID header
Definition at line 158 of file RHGenericDriver.cpp.
uint8_t headerTo | ( | ) | [virtual, inherited] |
Returns the TO header of the last received message.
- Returns:
- The TO header
Definition at line 148 of file RHGenericDriver.cpp.
bool init | ( | ) | [virtual] |
Initialise the Driver transport hardware and software.
Make sure the Driver is properly configured before calling init().
- Returns:
- true if initialisation succeeded.
Reimplemented from RHGenericDriver.
Definition at line 31 of file RH_RF95.cpp.
bool isChannelActive | ( | ) | [virtual] |
Use the radio's Channel Activity Detect (CAD) function to detect channel activity.
Sets the RF95 radio into CAD mode and waits until CAD detection is complete. To be used in a listen-before-talk mechanism (Collision Avoidance) with a reasonable time backoff algorithm. This is called automatically by waitCAD().
- Returns:
- true if channel is in use.
Reimplemented from RHGenericDriver.
Definition at line 347 of file RH_RF95.cpp.
int8_t lastRssi | ( | ) | [inherited] |
Returns the most recent RSSI (Receiver Signal Strength Indicator).
Usually it is the RSSI of the last received message, which is measured when the preamble is received. If you called readRssi() more recently, it will return that more recent value.
- Returns:
- The most recent RSSI measurement in dBm.
Definition at line 168 of file RHGenericDriver.cpp.
int lastSNR | ( | ) |
Returns the Signal-to-noise ratio (SNR) of the last received message, as measured by the receiver.
- Returns:
- SNR of the last received message in dB
Definition at line 396 of file RH_RF95.cpp.
uint8_t maxMessageLength | ( | ) | [virtual] |
Returns the maximum message length available in this Driver.
- Returns:
- The maximum legal message length
Implements RHGenericDriver.
Definition at line 221 of file RH_RF95.cpp.
RHGenericDriver::RHMode mode | ( | ) | [inherited] |
Returns the operating mode of the library.
- Returns:
- the current mode, one of RF69_MODE_*
Definition at line 173 of file RHGenericDriver.cpp.
void printBuffer | ( | const char * | prompt, |
const uint8_t * | buf, | ||
uint8_t | len | ||
) | [static, inherited] |
Prints a data buffer in HEX.
For diagnostic use
- Parameters:
-
[in] prompt string to preface the print [in] buf Location of the buffer to print [in] len Length of the buffer in octets.
Definition at line 189 of file RHGenericDriver.cpp.
bool printRegisters | ( | ) |
Prints the value of all chip registers to the Serial device if RH_HAVE_SERIAL is defined for the current platform For debugging purposes only.
- Returns:
- true on success
Definition at line 209 of file RH_RF95.cpp.
bool recv | ( | uint8_t * | buf, |
uint8_t * | len | ||
) | [virtual] |
Turns the receiver on if it not already on.
If there is a valid message available, copy it to buf and return true else return false. If a message is copied, *len is set to the length (Caution, 0 length messages are permitted). You should be sure to call this function frequently enough to not miss any messages It is recommended that you call it in your main loop.
- Parameters:
-
[in] buf Location to copy the received message [in,out] len Pointer to available space in buf. Set to the actual number of octets copied.
- Returns:
- true if a valid message was copied to buf
Implements RHGenericDriver.
Definition at line 166 of file RH_RF95.cpp.
uint16_t rxBad | ( | ) | [inherited] |
Returns the count of the number of bad received packets (ie packets with bad lengths, checksum etc) which were rejected and not delivered to the application.
Caution: not all drivers can correctly report this count. Some underlying hardware only report good packets.
- Returns:
- The number of bad packets received.
Definition at line 206 of file RHGenericDriver.cpp.
uint16_t rxGood | ( | ) | [inherited] |
Returns the count of the number of good received packets.
- Returns:
- The number of good packets received.
Definition at line 211 of file RHGenericDriver.cpp.
bool send | ( | const uint8_t * | data, |
uint8_t | len | ||
) | [virtual] |
Waits until any previous transmit packet is finished being transmitted with waitPacketSent().
Then optionally waits for Channel Activity Detection (CAD) to show the channnel is clear (if the radio supports CAD) by calling waitCAD(). Then loads a message into the transmitter and starts the transmitter. Note that a message length of 0 is permitted.
- Parameters:
-
[in] data Array of data to be sent [in] len Number of bytes of data to send specify the maximum time in ms to wait. If 0 (the default) do not wait for CAD before transmitting.
- Returns:
- true if the message length was valid and it was correctly queued for transmit. Return false if CAD was requested and the CAD timeout timed out before clear channel was detected.
Implements RHGenericDriver.
Definition at line 182 of file RH_RF95.cpp.
void setCADTimeout | ( | unsigned long | cad_timeout ) | [inherited] |
Sets the Channel Activity Detection timeout in milliseconds to be used by waitCAD().
The default is 0, which means do not wait for CAD detection. CAD detection depends on support for isChannelActive() by your particular radio.
Definition at line 221 of file RHGenericDriver.cpp.
bool setFrequency | ( | float | centre ) |
Sets the transmitter and receiver centre frequency.
- Parameters:
-
[in] centre Frequency in MHz. 137.0 to 1020.0. Caution: RFM95/96/97/98 comes in several different frequency ranges, and setting a frequency outside that range of your radio will probably not work
- Returns:
- true if the selected frquency centre is within range
Definition at line 226 of file RH_RF95.cpp.
void setHeaderFlags | ( | uint8_t | set, |
uint8_t | clear = RH_FLAGS_APPLICATION_SPECIFIC |
||
) | [virtual, inherited] |
Sets and clears bits in the FLAGS header to be sent in all subsequent messages First it clears he FLAGS according to the clear argument, then sets the flags according to the set argument.
The default for clear always clears the application specific flags.
- Parameters:
-
[in] set bitmask of bits to be set. Flags are cleared with the clear mask before being set. [in] clear bitmask of flags to clear. Defaults to RH_FLAGS_APPLICATION_SPECIFIC which clears the application specific flags, resulting in new application specific flags identical to the set.
Definition at line 142 of file RHGenericDriver.cpp.
void setHeaderFrom | ( | uint8_t | from ) | [virtual, inherited] |
Sets the FROM header to be sent in all subsequent messages.
- Parameters:
-
[in] from The new FROM header value
Definition at line 132 of file RHGenericDriver.cpp.
void setHeaderId | ( | uint8_t | id ) | [virtual, inherited] |
Sets the ID header to be sent in all subsequent messages.
- Parameters:
-
[in] id The new ID header value
Definition at line 137 of file RHGenericDriver.cpp.
void setHeaderTo | ( | uint8_t | to ) | [virtual, inherited] |
Sets the TO header to be sent in all subsequent messages.
- Parameters:
-
[in] to The new TO header value
Definition at line 127 of file RHGenericDriver.cpp.
void setMode | ( | RHMode | mode ) | [inherited] |
Sets the operating mode of the transport.
Definition at line 178 of file RHGenericDriver.cpp.
void setModeIdle | ( | ) |
If current mode is Rx or Tx changes it to Idle.
If the transmitter or receiver is running, disables them.
Definition at line 238 of file RH_RF95.cpp.
bool setModemConfig | ( | ModemConfigChoice | index ) |
Select one of the predefined modem configurations.
If you need a modem configuration not provided here, use setModemRegisters() with your own ModemConfig.
- Parameters:
-
[in] index The configuration choice.
- Returns:
- true if index is a valid choice.
Definition at line 329 of file RH_RF95.cpp.
void setModemRegisters | ( | const ModemConfig * | config ) |
Sets all the registered required to configure the data modem in the RF95/96/97/98, including the bandwidth, spreading factor etc.
You can use this to configure the modem with custom configurations if none of the canned configurations in ModemConfigChoice suit you.
- Parameters:
-
[in] config A ModemConfig structure containing values for the modem configuration registers.
Definition at line 320 of file RH_RF95.cpp.
void setModeRx | ( | ) |
If current mode is Tx or Idle, changes it to Rx.
Starts the receiver in the RF95/96/97/98.
Definition at line 257 of file RH_RF95.cpp.
void setModeTx | ( | ) |
If current mode is Rx or Idle, changes it to Rx.
F Starts the transmitter in the RF95/96/97/98.
Definition at line 267 of file RH_RF95.cpp.
void setPreambleLength | ( | uint16_t | bytes ) |
Sets the length of the preamble in bytes.
Caution: this should be set to the same value on all nodes in your network. Default is 8. Sets the message preamble length in RH_RF95_REG_??_PREAMBLE_?SB
- Parameters:
-
[in] bytes Preamble length in bytes.
Definition at line 341 of file RH_RF95.cpp.
void setPromiscuous | ( | bool | promiscuous ) | [virtual, inherited] |
Tells the receiver to accept messages with any TO address, not just messages addressed to thisAddress or the broadcast address.
- Parameters:
-
[in] promiscuous true if you wish to receive messages with any TO address
Definition at line 117 of file RHGenericDriver.cpp.
void setThisAddress | ( | uint8_t | thisAddress ) | [virtual, inherited] |
Sets the address of this node.
Defaults to 0xFF. Subclasses or the user may want to change this. This will be used to test the adddress in incoming messages. In non-promiscuous mode, only messages with a TO header the same as thisAddress or the broadcast addess (0xFF) will be accepted. In promiscuous mode, all messages will be accepted regardless of the TO header. In a conventional multinode system, all nodes will have a unique address (which you could store in EEPROM). You would normally set the header FROM address to be the same as thisAddress (though you dont have to, allowing the possibilty of address spoofing).
- Parameters:
-
[in] thisAddress The address of this node.
Definition at line 122 of file RHGenericDriver.cpp.
void setTxPower | ( | int8_t | power, |
bool | useRFO = false |
||
) |
Sets the transmitter power output level, and configures the transmitter pin.
Be a good neighbour and set the lowest power level you need. Some SX1276/77/78/79 and compatible modules (such as RFM95/96/97/98) use the PA_BOOST transmitter pin for high power output (and optionally the PA_DAC) while some (such as the Modtronix inAir4 and inAir9) use the RFO transmitter pin for lower power but higher efficiency. You must set the appropriate power level and useRFO argument for your module. Check with your module manufacturer which transmtter pin is used on your module to ensure you are setting useRFO correctly. Failure to do so will result in very low transmitter power output. Caution: legal power limits may apply in certain countries. After init(), the power will be set to 13dBm, with useRFO false (ie PA_BOOST enabled).
- Parameters:
-
[in] power Transmitter power level in dBm. For RFM95/96/97/98 LORA with useRFO false, valid values are from +5 to +23. For Modtronix inAir4 and inAir9 with useRFO true (ie RFO pins in use), valid values are from -1 to 14. [in] useRFO If true, enables the use of the RFO transmitter pins instead of the PA_BOOST pin (false). Choose the correct setting for your module.
Definition at line 277 of file RH_RF95.cpp.
bool sleep | ( | ) | [virtual] |
Sets the radio into low-power sleep mode.
If successful, the transport will stay in sleep mode until woken by changing mode it idle, transmit or receive (eg by calling send(), recv(), available() etc) Caution: there is a time penalty as the radio takes a finite time to wake from sleep mode.
- Returns:
- true if sleep mode was successfully entered.
Reimplemented from RHGenericDriver.
Definition at line 247 of file RH_RF95.cpp.
uint16_t txGood | ( | ) | [inherited] |
Returns the count of the number of packets successfully transmitted (though not necessarily received by the destination)
- Returns:
- The number of packets successfully transmitted
Definition at line 216 of file RHGenericDriver.cpp.
void validateRxBuf | ( | ) | [protected] |
Examine the revceive buffer to determine whether the message is for this node.
Definition at line 132 of file RH_RF95.cpp.
void waitAvailable | ( | ) | [virtual, inherited] |
Starts the receiver and blocks until a valid received message is available.
Definition at line 32 of file RHGenericDriver.cpp.
bool waitAvailableTimeout | ( | uint16_t | timeout ) | [virtual, inherited] |
Starts the receiver and blocks until a received message is available or a timeout.
- Parameters:
-
[in] timeout Maximum time to wait in milliseconds.
- Returns:
- true if a message is available
Definition at line 41 of file RHGenericDriver.cpp.
bool waitCAD | ( | ) | [virtual, inherited] |
Channel Activity Detection (CAD).
Blocks until channel activity is finished or CAD timeout occurs. Uses the radio's CAD function (if supported) to detect channel activity. Implements random delays of 100 to 1000ms while activity is detected and until timeout. Caution: the random() function is not seeded. If you want non-deterministic behaviour, consider using something like randomSeed(analogRead(A0)); in your sketch. Permits the implementation of listen-before-talk mechanism (Collision Avoidance). Calls the isChannelActive() member function for the radio (if supported) to determine if the channel is active. If the radio does not support isChannelActive(), always returns true immediately
- Returns:
- true if the radio-specific CAD (as returned by isChannelActive()) shows the channel is clear within the timeout period (or the timeout period is 0), else returns false.
Definition at line 84 of file RHGenericDriver.cpp.
bool waitPacketSent | ( | uint16_t | timeout ) | [virtual, inherited] |
Blocks until the transmitter is no longer transmitting.
or until the timeout occuers, whichever happens first
- Parameters:
-
[in] timeout Maximum time to wait in milliseconds.
- Returns:
- true if the radio completed transmission within the timeout period. False if it timed out.
Definition at line 66 of file RHGenericDriver.cpp.
bool waitPacketSent | ( | ) | [virtual, inherited] |
Blocks until the transmitter is no longer transmitting.
Definition at line 59 of file RHGenericDriver.cpp.
Field Documentation
volatile bool _cad [protected, inherited] |
Channel activity detected.
Definition at line 304 of file RHGenericDriver.h.
unsigned int _cad_timeout [protected, inherited] |
Channel activity timeout in ms.
Definition at line 307 of file RHGenericDriver.h.
volatile int8_t _lastRssi [protected, inherited] |
The value of the last received RSSI value, in some transport specific units.
Definition at line 292 of file RHGenericDriver.h.
The current transport operating mode.
Definition at line 259 of file RHGenericDriver.h.
bool _promiscuous [protected, inherited] |
Whether the transport is in promiscuous mode.
Definition at line 265 of file RHGenericDriver.h.
volatile uint16_t _rxBad [protected, inherited] |
Count of the number of bad messages (eg bad checksum etc) received.
Definition at line 295 of file RHGenericDriver.h.
volatile uint16_t _rxGood [protected, inherited] |
Count of the number of successfully transmitted messaged.
Definition at line 298 of file RHGenericDriver.h.
volatile uint8_t _rxHeaderFlags [protected, inherited] |
FLAGS header in the last received mesasge.
Definition at line 277 of file RHGenericDriver.h.
volatile uint8_t _rxHeaderFrom [protected, inherited] |
FROM header in the last received mesasge.
Definition at line 271 of file RHGenericDriver.h.
volatile uint8_t _rxHeaderId [protected, inherited] |
ID header in the last received mesasge.
Definition at line 274 of file RHGenericDriver.h.
volatile uint8_t _rxHeaderTo [protected, inherited] |
TO header in the last received mesasge.
Definition at line 268 of file RHGenericDriver.h.
uint8_t _thisAddress [protected, inherited] |
This node id.
Definition at line 262 of file RHGenericDriver.h.
volatile uint16_t _txGood [protected, inherited] |
Count of the number of bad messages (correct checksum etc) received.
Definition at line 301 of file RHGenericDriver.h.
uint8_t _txHeaderFlags [protected, inherited] |
FLAGS header to send in all messages.
Definition at line 289 of file RHGenericDriver.h.
uint8_t _txHeaderFrom [protected, inherited] |
FROM header to send in all messages.
Definition at line 283 of file RHGenericDriver.h.
uint8_t _txHeaderId [protected, inherited] |
ID header to send in all messages.
Definition at line 286 of file RHGenericDriver.h.
uint8_t _txHeaderTo [protected, inherited] |
TO header to send in all messages.
Definition at line 280 of file RHGenericDriver.h.
Generated on Mon Jul 18 2022 11:18:50 by
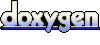