
PICO I2C FW
Dependencies: USBDevice
PicoCommands.h
00001 #ifndef PICOCOMMANDS_H 00002 #define PICOCOMMANDS_H 00003 00004 /// <summary> 00005 /// PICO USB HID supported commands (Report ID byte) 00006 /// </summary> 00007 enum UsbHidReportID 00008 { 00009 GenericHID = 0x00 00010 }; 00011 00012 enum UsbHidOpCode 00013 { 00014 GetEmbApp = 0x01, 00015 SetEmbApp = 0x02, 00016 00017 I2CGet = 0x10, 00018 I2CSetCfg = 0x11, 00019 I2CWriteReg = 0x12, 00020 I2CReadReg = 0x13, 00021 I2CReadNoReg = 0x14, 00022 00023 SpiGet = 0x20, 00024 SpiSet = 0x21, 00025 SpiWrite = 0x22, 00026 00027 GpioGet = 0x30, 00028 GpioSetCfg = 0x31, 00029 GpioWrite = 0x32, 00030 GpioRead = 0x33, 00031 GpioIntFuncState = 0x34, 00032 GpioIntNotify = 0x35, 00033 00034 PwmSetRaw = 0x40, 00035 PwmSetCfgRaw = 0x41, 00036 }; 00037 00038 /// <summary> 00039 /// PICO USB HID General Get/Set opcodes 00040 /// </summary> 00041 enum UsbHidGetSet 00042 { 00043 VerNum = 0x00, 00044 VerStr = 0x01, 00045 BoardID = 0x02, 00046 ProdIDStr = 0x03, 00047 }; 00048 00049 /// <summary> 00050 /// PICO USB HID SPI Get/Set opcodes 00051 /// </summary> 00052 enum UsbHidSpi 00053 { 00054 SetCfg = 0x00, 00055 GetCfg = 0x00, 00056 00057 GetBusSpeedAll = 0x01, 00058 GetBusSpeedCur = 0x02, 00059 SetChipSelect = 0x03, 00060 }; 00061 00062 /// <summary> 00063 /// PICO Supported Ports 00064 /// </summary> 00065 enum GpioPortID 00066 { 00067 GpioA = 0, 00068 GpioB = 1, 00069 GpioC = 2, 00070 }; 00071 00072 /// <summary> 00073 /// PICO Supported Pin IDs 00074 /// </summary> 00075 enum GpioPinID 00076 { 00077 PinID0 = 0, 00078 PinID1 = 1, 00079 PinID2 = 2, 00080 PinID3 = 3, 00081 PinID4 = 4, 00082 PinID5 = 5, 00083 PinID6 = 6, 00084 PinID7 = 7, 00085 PinID8 = 8, 00086 PinID9 = 9, 00087 PinID10 = 10, 00088 PinID11 = 11, 00089 PinID12 = 12, 00090 PinID13 = 13, 00091 PinID14 = 14, 00092 PinID15 = 15, 00093 }; 00094 00095 /// <summary> 00096 /// PICO Supported Pin modes 00097 /// </summary> 00098 enum GpioPinMode 00099 { 00100 InputFloating = 0, 00101 InputPullup = 1, 00102 InputPulldown = 2, 00103 OutputOpenDrain = 3, 00104 OutputPushpull = 4 00105 }; 00106 00107 /// <summary> 00108 /// PICO Supported Interrupt Functional States 00109 /// </summary> 00110 enum GpioIntFuncState 00111 { 00112 Disable = 0, 00113 Enable = 1 00114 }; 00115 00116 /// <summary> 00117 /// PICO Supported Pin States 00118 /// </summary> 00119 enum GpioPinState 00120 { 00121 Low = 0, 00122 High = 1 00123 }; 00124 00125 enum CmdStatus 00126 { 00127 Success = 0x00, 00128 Failure = 0x01, 00129 00130 I2CBusy = 0x10, 00131 I2CResourceError = 0x11, 00132 I2CNotSupported = 0x12, 00133 I2CUnknown = 0x13, 00134 I2CMrmsErr = 0x14, 00135 I2CMtmsErr = 0x15, 00136 I2CMbtErr = 0x16 00137 }; 00138 00139 /// <summary> 00140 /// PICO Supported Hardware Blocks 00141 /// </summary> 00142 enum HardwareBlockID 00143 { 00144 One = 1, 00145 Two = 2 00146 }; 00147 00148 /// <summary> 00149 /// PICO Firmware Version 00150 /// </summary> 00151 struct FWVersion 00152 { 00153 uint8_t Major; 00154 uint8_t Minor; 00155 uint8_t Inc; 00156 }; 00157 00158 #endif
Generated on Mon Jul 25 2022 20:04:22 by
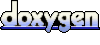