
Joystick deneme modülü (internetteki kod)
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 // Joystick Library Example 00002 // 00003 // Reads the joystick x axis and fades green and red LEDs in response. 00004 // The blue LED is turned on while the pushbutton is pressed. 00005 00006 #include "mbed.h" 00007 #include <Joystick.h> 00008 00009 PwmOut led_R(LED1); 00010 PwmOut led_G(LED2); 00011 PwmOut led_B(LED3); 00012 00013 Joystick j(PTB0, PTB1, PTB2); 00014 00015 // call on joystick button release 00016 void jrise(void) { 00017 led_B = 1.0f; 00018 } 00019 00020 // call on joystick button press 00021 void jfall(void) { 00022 led_B = 0.0f; 00023 } 00024 00025 int main () { 00026 // setup 00027 led_R = 1.0f; 00028 led_G = 1.0f; 00029 led_B = 0.0f; 00030 joypos p; // joystick position 00031 // attach joystick button interrupts 00032 j.rise(&jrise); 00033 j.fall(&jfall); 00034 float fade = 0.0f; 00035 00036 // loop 00037 while (1) { 00038 p = j.read(); 00039 fade = p.x; 00040 if (fade > 0.0) { 00041 led_R = 1.0f; 00042 led_G = 1.0f - (float)fade; 00043 } else if (fade < 0.0) { 00044 led_R = 1.0f + (float)fade; 00045 led_G = 1.0f; 00046 } else { 00047 led_R = 1.0f; 00048 led_G = 1.0f; 00049 } 00050 wait(0.01); 00051 } 00052 }
Generated on Wed Jul 13 2022 00:29:04 by
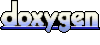