
test_IPKF
Dependencies: mbed
matrix.h
00001 /** @brief 00002 * @file matrix.h 00003 * @author Daniele Ludovico 00004 * @date 20/3/2016 00005 * @brief File containing the function to manipulates matrices. 00006 * 00007 */ 00008 00009 00010 #ifndef MATRIX_H_INCLUDED 00011 #define MATRIX_H_INCLUDED 00012 00013 00014 #ifdef __cplusplus 00015 extern "C" { 00016 #endif 00017 00018 #include <stdio.h> 00019 #include <stdlib.h> 00020 00021 /** @brief struct matrix 00022 * allows to execute matrix computation 00023 */ 00024 typedef struct s_matrix 00025 { 00026 int row;/**< number of row of the matrix */ 00027 int col;/**< number of column of the matrix */ 00028 float** element; /**< this field allows to read and write the elements of the matrix */ 00029 }matrix; 00030 00031 /********************************************//** 00032 * \brief this function allows to allocate a 00033 * matrix in memory 00034 * 00035 * \param matrix pointer to the object to 00036 * allocate 00037 * 00038 * \return 0 if the allocation is done 00039 * correctly 00040 * \return 1 if there is not enough space in 00041 * memory to create a new matrix 00042 * 00043 ***********************************************/ 00044 00045 int CreateMatrix(matrix* m); 00046 00047 /********************************************//** 00048 * \brief this function allows to delete from 00049 * memory a matrix struct 00050 * 00051 * \param matrix pointer to the object to 00052 * delete 00053 * \return void 00054 * 00055 ***********************************************/ 00056 00057 void DeleteMatrix(matrix* m); 00058 00059 /********************************************//** 00060 * \brief this function initialize a matrix 00061 * 00062 * \param m is the pointer to the matrix to 00063 * initialize 00064 * \param row is the number of row of the matrix 00065 * \param col is the number of column of the matrix 00066 * 00067 * \return 0 if the allocation is done 00068 * correctly 00069 * \return 1 if there is not enough space in 00070 * memory to create a new matrix 00071 * 00072 ***********************************************/ 00073 00074 int InitMatrix(matrix* m, int row, int col); 00075 00076 /********************************************//** 00077 * \brief this function allows to compute 00078 * the product between 2 matrices 00079 * 00080 * \param a is the first element of the product 00081 * \param b is the second element of the product 00082 * \param r is the matrix which will contain 00083 * the result 00084 * 00085 * \return 0 if the product is computed correctly 00086 * \return 1 if the number of column of a is different 00087 * from the row of b 00088 * \return 2 if the number of row of a is different 00089 * from number of row of r 00090 * \return 3 if the number of column of b is different 00091 * from number of column of r 00092 * 00093 ***********************************************/ 00094 00095 int MxM (matrix* a, matrix* b, matrix* r); 00096 00097 /********************************************//** 00098 * \brief this function allows to compute the 00099 * product between a scalar and a matrix 00100 * 00101 * \param m is the pointer to the matrix 00102 * \param a is the scalar number 00103 * \param r is the pointer to the result 00104 * 00105 * \return 0 if the the product is computed 00106 * correctly 00107 * \return 1 if the number of row of a is different 00108 * from number of row of r 00109 * \return 2 if the number of col of a is different 00110 * from number of col of r 00111 * 00112 ***********************************************/ 00113 00114 int axM (matrix* m, float a, matrix* r); 00115 00116 /********************************************//** 00117 * \brief this function allows to compute the 00118 * sum between two matrices 00119 * 00120 * \param a is the pointer to the first matrix 00121 * \param b is the pointer to the second matrix 00122 * \param c is the pointer to the result 00123 * 00124 * \return 0 if the sum is computed correctly 00125 * \return 1 if the number of column of a is different 00126 * from the column of b 00127 * \return 2 if the number of row of a is different 00128 * from number of row of b 00129 * \return 3 if the number of row of a is different 00130 * from number of row of r 00131 * \return 4 if the number of column of a is different 00132 * from number of column of r 00133 * 00134 ***********************************************/ 00135 00136 int Sum (matrix* a, matrix* b, matrix* r); 00137 00138 /********************************************//** 00139 * \brief this function allows to compute the 00140 * subtraction between two matrices 00141 * 00142 * \param a is the pointer to the first matrix 00143 * \param b is the pointer to the second matrix 00144 * \param c is the pointer to the result 00145 * 00146 * \return 0 if the subtraction is computed correctly 00147 * \return 1 if the number of column of a is different 00148 * from the column of b 00149 * \return 2 if the number of row of a is different 00150 * from number of row of b 00151 * \return 3 if the number of row of a is different 00152 * from number of row of r 00153 * \return 4 if the number of column of a is different 00154 * from number of column of r 00155 * 00156 ***********************************************/ 00157 00158 int Sub (matrix* a, matrix* b, matrix* r); 00159 00160 /********************************************//** 00161 * \brief this function allows to compute the 00162 * traspost of a matrix 00163 * 00164 * \param m is the pointer to the matrix to 00165 * be trasposed 00166 * \param r is the pointer to the result 00167 * 00168 * \return 0 if the operation is done correctly 00169 * \return 1 if the number of row of m is different 00170 * from the column of r 00171 * \return 2 if the number of column of m is different 00172 * from the row of r 00173 * 00174 ***********************************************/ 00175 00176 int Traspost(matrix* m, matrix* r); 00177 00178 /********************************************//** 00179 * \brief this function allows to compute the 00180 * inverse of a matrix using the Gauss algorithm 00181 * 00182 * \param m is the pointer to the original matrix 00183 * \param inv_m is the pointer to the result 00184 * 00185 * \return 0 if the inversion is done correctly 00186 * \return 1 if m is not a square matrix 00187 * \return 2 if the number of row of m is different 00188 * from the row of inv_m 00189 * \return 3 if the number of column of m is different 00190 * from the column of inv_m 00191 * \return 4 if m is not invertible 00192 * 00193 ***********************************************/ 00194 00195 int Inv (matrix* m, matrix* inv_m); 00196 00197 /********************************************//** 00198 * \brief Computes the cross product between two vectors 00199 * 00200 * \param v is the first vector 00201 * \param w is the second vector 00202 * \param r is address to the resulting matrix 00203 * \return void 00204 * 00205 ***********************************************/ 00206 00207 void CrossProd(matrix* v, matrix* w, matrix* r); 00208 00209 /********************************************//** 00210 * \brief print the matrix in the prompt 00211 * 00212 * \param m is the pointer to the matrix to print 00213 * 00214 * \return void 00215 * 00216 ***********************************************/ 00217 00218 void Print_Matrix(matrix* m); 00219 00220 #ifdef __cplusplus 00221 } 00222 #endif 00223 00224 #endif // MATRIX_H_INCLUDED 00225 00226 00227
Generated on Tue Jul 12 2022 11:35:17 by
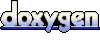