
test_IPKF
Dependencies: mbed
kinematic.h
00001 /********************************************//** 00002 * @brief 00003 * @file kinematic.h 00004 * @author Daniele Ludovico, Fiorella Sibona 00005 * @date 12/04/2016 00006 * @brief File containing the function to compute the 00007 * kinematic function of a robotic arm. 00008 * 00009 */ 00010 00011 #ifndef KINEMATIC_H_INCLUDED 00012 #define KINEMATIC_H_INCLUDED 00013 00014 #ifdef __cplusplus 00015 extern "C" { 00016 #endif 00017 00018 #include <math.h> 00019 #include "matrix.h" 00020 #include "params.h" 00021 00022 00023 /********************************************//** 00024 * @brief struct DHP 00025 * denavit hartenberg parameter 00026 ***********************************************/ 00027 typedef struct s_DHP{ 00028 float d; 00029 float theta; 00030 float a; 00031 float alpha; 00032 } DHP; 00033 00034 00035 /********************************************//** 00036 * \brief this function allows to update 00037 * the current dh parameter with the 00038 * actual joints angle 00039 * 00040 * \param pointer to the DH parameter to 00041 * update 00042 * \param pointer to the new joint angle, 00043 * value which update the DHP 00044 * 00045 * \return void 00046 * 00047 ***********************************************/ 00048 00049 void DHP_update(DHP* dhp, matrix* joints); 00050 00051 /********************************************//** 00052 * \brief this function allows to compute 00053 * the homogeneouse trasformation matrix 00054 * starting from DH parameters 00055 * 00056 * \param pointer to the actual DH parameter 00057 * \param pointer to the trasformation matrix 00058 * to compute 00059 * 00060 * \return void 00061 * 00062 ***********************************************/ 00063 00064 void DH_Transf(DHP* dhp, matrix* T); 00065 00066 /********************************************//** 00067 * \brief Function which performs the direct 00068 * position kinematic function. Given the 00069 * joints values the TCP pose is computed 00070 * 00071 * \param pointer to the structure containing 00072 * the actual joints values 00073 * \param pointer to the pose structure where 00074 * the result will be stored 00075 * 00076 * \return void 00077 ************************************************/ 00078 00079 void DPKF (matrix* joint, matrix* p); 00080 00081 /********************************************//** 00082 * \brief Function which returns the 00083 * geometric Jacobian given the joints values 00084 * 00085 * \param pointer to the structure containing 00086 * the joints values 00087 * \param Jg is the address where the 00088 * geometric Jacobian will be stored 00089 * \param T_TCP is the matrix in which the 00090 * homogeneous transformation from 0 to TCP 00091 * is stored once computed 00092 * 00093 * \return void 00094 ***********************************************/ 00095 void JacobianG(matrix* joint, matrix* Jg, matrix* T_TCP); 00096 00097 /********************************************//** 00098 * \brief Function which returns the Analytical Jacobian given the joints values 00099 * 00100 * \param joint is the address to the structure containing the joints values 00101 * \param Ja is the address where the Analytical Jacobian will be stored 00102 * 00103 * \return void 00104 * 00105 ***********************************************/ 00106 void JacobianA(matrix* joint, matrix * Ja); 00107 00108 /********************************************//** 00109 * \brief Computes the inverse kinematics (returns the joints values for a desired pose). 00110 * 00111 * \param p is the pointer to the desired pose 00112 * \param joint is where the result will be saved 00113 * 00114 * \return 0 if the inverse kinematics is computed 00115 * correctly 00116 * \return 1 if the point to reach is not in the 00117 * task space 00118 ***********************************************/ 00119 int IPKF (matrix* p, matrix* joint); 00120 00121 void Print_DHP(DHP* dhp); 00122 00123 #ifdef __cplusplus 00124 } 00125 #endif 00126 00127 #endif // KINEMATIC_H_INCLUDED 00128 00129
Generated on Tue Jul 12 2022 11:35:16 by
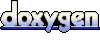