
funcionando
Dependencies: EPD_GDE021A1 mbed reScale
Fork of DISCO-L053C8_ePD_demo by
main.cpp
00001 /*Código escrito por Marcelo Costanzo Miranda 00002 *Projeto feito conforme pedido do professor Silvio Szafir 00003 * 00004 * Placa ST discovery com display ePaper funcionando como LCD 20x4 00005 * 00006 * São Paulo, SP 00007 * 30 de agosto de 2018 00008 * 00009 */ 00010 00011 #include "mbed.h" 00012 #include "EPD_GDE021A1.h" 00013 00014 00015 00016 #define EPD_CS PA_15 00017 #define EPD_DC PB_11 00018 #define EPD_RESET PB_2 00019 #define EPD_BUSY PA_8 00020 #define EPD_POWER PB_10 00021 #define EPD_SPI_MOSI PB_5 00022 #define EPD_SPI_MISO PB_4 00023 #define EPD_SPI_SCK PB_3 00024 00025 EPD_GDE021A1 epd(EPD_CS, EPD_DC, EPD_RESET, EPD_BUSY, EPD_POWER, EPD_SPI_MOSI, EPD_SPI_MISO, EPD_SPI_SCK); 00026 00027 DigitalOut led1(LED1); 00028 DigitalIn button(PA_0); 00029 Ticker flipper; 00030 Serial pc(USBTX, USBRX); // tx, rx 00031 00032 //width 48 00033 //height 26 00034 00035 static uint8_t Battery_img[] = { 00036 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 00037 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 00038 0xfe, 0xff, 0xff, 0xff, 0xff, 0x0f, 00039 0xfe, 0xff, 0xff, 0x3f, 0x00, 0x0c, 00040 0xfe, 0xff, 0xff, 0x3f, 0x00, 0x08, 00041 0xfe, 0xff, 0xff, 0x3f, 0x00, 0x08, 00042 0xfe, 0xff, 0xff, 0x3f, 0x00, 0x08, 00043 0xfe, 0xff, 0xff, 0x3f, 0x00, 0x08, 00044 0xfe, 0xff, 0xff, 0x3f, 0x00, 0x18, 00045 0xfe, 0xff, 0xff, 0x3f, 0x10, 0x38, 00046 0xfe, 0xff, 0xff, 0x3f, 0x10, 0x38, 00047 0xfe, 0xff, 0xff, 0x3f, 0x10, 0x38, 00048 0xfe, 0xff, 0xff, 0x3f, 0xff, 0x39, 00049 0xfe, 0xff, 0xff, 0x3f, 0x10, 0x38, 00050 0xfe, 0xff, 0xff, 0x3f, 0x10, 0x38, 00051 0xfe, 0xff, 0xff, 0x3f, 0x10, 0x38, 00052 0xfe, 0xff, 0xff, 0x3f, 0x00, 0x38, 00053 0xfe, 0xff, 0xff, 0x3f, 0x00, 0x18, 00054 0xfe, 0xff, 0xff, 0x3f, 0x00, 0x08, 00055 0xfe, 0xff, 0xff, 0x3f, 0x00, 0x08, 00056 0xfe, 0xff, 0xff, 0x3f, 0x00, 0x08, 00057 0xfe, 0xff, 0xff, 0x3f, 0x00, 0x0c, 00058 0xfe, 0xff, 0xff, 0x3f, 0x00, 0x0c, 00059 0xfe, 0xff, 0xff, 0xff, 0xff, 0x0f, 00060 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 00061 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 00062 }; 00063 00064 void flip() 00065 { 00066 led1=!led1; 00067 } 00068 00069 uint16_t x=0, y=0, i=0; 00070 char c, c1, c2; 00071 char s[100]; 00072 00073 00074 int main() 00075 { 00076 00077 flipper.attach(&flip, 0.1);//interrupção para piscar o led, debug para saber se o uC travou 00078 00079 00080 //mensagens de boas vindas 00081 epd.Clear(EPD_COLOR_WHITE); 00082 epd.DisplayStringAtLine(5, (uint8_t*)"MBED", CENTER_MODE); 00083 epd.DisplayStringAtLine(3, (uint8_t*)"Epaper display", LEFT_MODE); 00084 epd.DisplayStringAtLine(2, (uint8_t*)"demo", LEFT_MODE); 00085 epd.DrawImage(130, 0, 48, 26, Battery_img); 00086 epd.DrawRect(50, 4, 60, 4); 00087 epd.RefreshDisplay(); 00088 wait(1); 00089 epd.Clear(EPD_COLOR_WHITE); 00090 epd.RefreshDisplay(); 00091 epd.Clear(EPD_COLOR_WHITE); 00092 00093 00094 epd.SetFont(&Font16); 00095 while(1) 00096 { 00097 00098 //----------ROTINA PARA ESCREVER NO EPAPER------------------------ 00099 00100 pc.printf("\nEscolha a linha Y, de 0 a 3:"); 00101 c = pc.getc(); 00102 switch (c) 00103 { 00104 case '3': y = 0;break; 00105 case '2': y = 4;break; 00106 case '1': y = 8;break; 00107 case '0': y = 12;break; 00108 } 00109 pc.printf("\n%u",y); 00110 00111 pc.printf("\nEscolha a coluna X, de 00 a 19:"); 00112 c1 = pc.getc(); 00113 c2 = pc.getc(); 00114 switch (c2) 00115 { 00116 case '0': x = 0;break; 00117 case '1': x = 1;break; 00118 case '2': x = 2;break; 00119 case '3': x = 3;break; 00120 case '4': x = 4;break; 00121 case '5': x = 5;break; 00122 case '6': x = 6;break; 00123 case '7': x = 7;break; 00124 case '8': x = 8;break; 00125 case '9': x = 9;break; 00126 } 00127 00128 switch (c1) 00129 { 00130 case '0': x = x + 0;break; 00131 case '1': x = x + 10;break; 00132 00133 } 00134 00135 x = x * 10; 00136 pc.printf("\n%u",x); 00137 00138 pc.printf("\nEntre com seu texto (com # no final):"); 00139 00140 do 00141 { 00142 c = pc.getc(); 00143 s[i] = c; 00144 ++i; 00145 } 00146 while(c!='#'); //entra em loop, coletando os caracteres até achar o #, e então sai do loop 00147 00148 s[i-1] = '\0'; //elimina o caracter terminador de strings 00149 pc.printf("%s", s); 00150 for(int o = 0; o < i - 1; o++) 00151 { 00152 epd.DisplayChar(x, y,s[o]); 00153 x = x + 10; 00154 } 00155 epd.RefreshDisplay(); 00156 i=0; 00157 00158 //FIM DA ROTINA PARA ESCREVER NO EPAPER 00159 00160 }//FIM DA WHILE 00161 }//FIM DO PROGRAMA
Generated on Thu Aug 4 2022 23:49:19 by
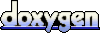