
Task 4.4.4 Solution
Dependencies: mbed
main.cpp
00001 #include "mbed.h" 00002 00003 //Function prototype 00004 void doSample1Hz(); 00005 00006 //Global objects 00007 Serial pc(USBTX, USBRX); 00008 AnalogIn POT_ADC_In(A0); 00009 DigitalOut led(LED1); 00010 00011 #define N 16 00012 00013 //Shared variables 00014 volatile static unsigned short sample16 = 0; 00015 00016 unsigned int sum = 0; 00017 unsigned int count = 0; 00018 00019 //The ticker, used to sample data at a fixed rate 00020 Ticker t; 00021 00022 //Main function 00023 int main() 00024 { 00025 //Set baud rate to 115200 00026 pc.baud(115200); 00027 00028 //Set up the ticker - 100Hz 00029 t.attach(doSample1Hz, 0.01); 00030 00031 while(1) { 00032 00033 //Sleep 00034 sleep(); 00035 00036 //Add new sample to the sum 00037 sum += sample16; 00038 00039 //Increment the count 00040 count++; 00041 00042 //Enough to calculate the average? 00043 if (count == N) { 00044 //Divide sum by 16 00045 unsigned short mean = (sum >> 4); 00046 00047 //Display 00048 pc.printf("Mean = %hu\n", mean); 00049 00050 //Reset sum and count 00051 count = sum = 0; 00052 } 00053 00054 } //end while(1) 00055 } //end main 00056 00057 //ISR for the ticker - simply there to perform sampling 00058 void doSample1Hz() 00059 { 00060 //Toggle on board led 00061 led = !led; 00062 00063 //READ ADC as an unsigned integer. 00064 //Shift right 4 bits (this is a 12bit ADC) & store in static global variable 00065 sample16 = POT_ADC_In.read_u16() >> 4; 00066 } 00067 00068
Generated on Thu Aug 11 2022 18:42:25 by
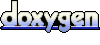