
Task 4.3.3 Solution
Dependencies: mbed
main.cpp
00001 #include "mbed.h" 00002 00003 //Constants 00004 #define N 10 00005 00006 //Global objects 00007 Serial pc(USBTX, USBRX); 00008 00009 BusOut binaryOutput(D5, D6, D7); 00010 AnalogIn POT_ADC_In(A0); 00011 AnalogIn LDR_ADC_In(A1); 00012 00013 float xPOT[N]; //Aray of potentiometer samples 00014 float xLDR[N]; //Aray of LDR samples 00015 00016 //Main function 00017 int main() 00018 { 00019 00020 //Set baud rate to 115200 00021 pc.baud(115200); 00022 00023 //Print header 00024 pc.printf("POT,LDR,avPOT, acLDR\n\n"); 00025 00026 while(1) { 00027 00028 //Move the samples in both arrays to the right 00029 for (unsigned int n=(N-1); n>0; n--) { 00030 xPOT[n] = xPOT[n-1]; 00031 xLDR[n] = xLDR[n-1]; 00032 } 00033 00034 //Insert the new sample at position 0 00035 xPOT[0] = POT_ADC_In; 00036 xLDR[0] = LDR_ADC_In; 00037 00038 //Calculate average for POT 00039 float fSum = 0.0; 00040 for (unsigned int n=0; n<N; n++) { 00041 fSum += xPOT[n]; 00042 } 00043 float fPOTAverage = fSum / (float)N; 00044 00045 //Calculate average for LDR 00046 fSum = 0.0; 00047 for (unsigned int n=0; n<N; n++) { 00048 fSum += xLDR[n]; 00049 } 00050 float fLDRAverage = fSum / (float)N; 00051 00052 //Write to terminal via Serial interface 00053 pc.printf("%6.4f,%6.4f,%6.4f,%6.4f\n", xPOT[N/2], xLDR[N/2], fPOTAverage, fLDRAverage); 00054 00055 //Check the threshold 00056 if (fLDRAverage > fPOTAverage) { 00057 binaryOutput = 0; //Binary 000 00058 } else { 00059 binaryOutput = 7; //Binary 111 00060 } 00061 00062 //Wait 1/100th second 00063 wait(0.01); 00064 00065 00066 } //end while(1) 00067 } //end main
Generated on Thu Aug 11 2022 23:11:31 by
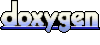