
This program demonstrates the usage of the PWM. Program sets PWM0 chanel 1 and outputs it to the pin P1.2 where we get a PWM signal with a constantly changing working cycle.
Dependencies: mbed
main.c
00001 #include "LPC4088-ioconfig.h" 00002 #include "LPC4088-system.h" 00003 #include "LPC4088-pwm.h" 00004 00005 00006 //prototipi funkcij 00007 void delay(void); 00008 00009 00010 int main(){ 00011 00012 //omogocimo PWM0, ki ob zagonu ni prizgan 00013 PCONP = PCONP | (0x1<<5); 00014 00015 //resetiranje PWM0 00016 //RSTCON0 = RSTCON0 | (0x1<<5); 00017 00018 //nastavitev pina P1.2 kot izhoda PWM0_1 - v headerju zakomentiramo timer PWM1 00019 IOCON_P1_2 = IOCON_P1_2 & !(0x67F); 00020 IOCON_P1_2 = IOCON_P1_2 | 0x3; 00021 00022 //nastavitev pina P1.18 kot izhoda PWM1_1 - v headerju zakomentiramo timer PWM0 00023 //IOCON_P1_18 = IOCON_P1_18 & !(0x67F); 00024 //IOCON_P1_18 = IOCON_P1_18 | 0b010; 00025 00026 //pocistimo interrupte za PWM0 00027 IR = IR | 0x73F; 00028 00029 //nastavimo "individual use" 00030 TCR = TCR & !(1<<4); 00031 00032 //nastavimo "Timer mode" 00033 CTCR = CTCR & !(0x3); 00034 00035 //omogočimo PWMx_1 kanal (izhod) 00036 PCR = PCR | (1<<9); 00037 00038 //nastavimo match register MR0 tako, da ob TC == MR0 resetira 00039 //celotni PWM0. Uporabili bomo kanal PWM0_1 in tega setamo in 00040 //resetamo z MR0 in MR1 (UM, tabela 556). 1 00041 MCR = MCR | (1<<1); 00042 MR0 = 0x7FFFF; 00043 00044 //ko so vrednosti vpisane v MR0 in MR1, obe vrednosti omogočimo (istočasno) 00045 LER = LER | 0x1; 00046 00047 //nastavimo "timer mode" in ne "PWM mode" (ne deluje) 00048 //POZOR! Mora biti za nastavitvijo MR0/MR1 00049 TCR = TCR & !(1<<3); 00050 00051 //vklopimo PWM0 - mora biti za nastavitvijo MR0/MR1 00052 //POZOR! Mora biti za nastavitvijo MR0/MR1 00053 TCR = TCR | (1<<0); 00054 00055 while(1){ 00056 00057 int count = 0x7FFFF; 00058 00059 while(count){ 00060 00061 count = count-1; 00062 delay(); 00063 MR1 = count; 00064 LER = LER | 0x2; 00065 00066 } 00067 00068 00069 } 00070 00071 } 00072 00073 void delay(void){ 00074 volatile int stej = 100; 00075 while(stej){ 00076 stej = stej - 1; 00077 } 00078 }
Generated on Wed Jul 13 2022 05:09:54 by
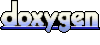