WiFi DipCortex USB CDC
Dependencies: HTTPClient NTPClient USBDevice WebSocketClient cc3000_hostdriver_mbedsocket mbed
Fork of WiFiDip-UsbKitchenSink by
tcpTests.cpp
00001 #include "mbed.h" 00002 #include "cc3000.h" 00003 #include "USBSerial.h" 00004 00005 #include "TCPSocketConnection.h" 00006 #include "TCPSocketServer.h" 00007 #include "HTTPClient.h" 00008 #include "Websocket.h" 00009 00010 using namespace mbed_cc3000; 00011 00012 extern cc3000 wifi; 00013 extern USBSerial pc; 00014 00015 HTTPClient http; 00016 00017 // NOTE : Change SolderSplashLabs to anything else for your own channel 00018 // Goto the viewier page here : http://sockets.mbed.org/SolderSplashLabs/viewer to see the websocket writes 00019 const char WEB_SOCKET_URL[] = {"ws://sockets.mbed.org/ws/SolderSplashLabs/rw"}; 00020 const char* ECHO_SERVER_ADDRESS = "192.168.0.10"; 00021 const int ECHO_SERVER_PORT_TCP = 80; 00022 char hello[] = "Hello World\r\n"; 00023 00024 // Put the tmpbuffer into RAM1 00025 char tmpBuffer[512] __attribute__((section("AHBSRAM0"))); 00026 00027 // Add your own feed Id and API key here.. 00028 char XivelyHostname[] = "api.xively.com"; 00029 char XivelyPath[] = "/v2/feeds/YOUR-FEED-ID-HERE"; 00030 char XivelyApiKey[] = "YOUR-API-KEY-HERE"; 00031 00032 AnalogIn adc0(P0_11); //P2 00033 AnalogIn adc1(P0_12); //P3 00034 AnalogIn adc2(P0_13); //P4 00035 AnalogIn adc3(P0_14); //P5 00036 AnalogIn adc5(P0_16); //P8 00037 AnalogIn adc6(P0_22); //P9 00038 AnalogIn adc7(P0_23); //P10 00039 00040 // ------------------------------------------------------------------------------------------------------------ 00041 /*! 00042 @brief Post all analog inputs to xively every second 00043 */ 00044 // ------------------------------------------------------------------------------------------------------------ 00045 void XivelySimpleTest ( void ) 00046 { 00047 TCPSocketConnection socket; 00048 int res = 0; 00049 uint16_t counter = 0; 00050 int httpCmdLen = 0; 00051 00052 //if ( wifi.is_connected() ) 00053 00054 while (1) 00055 { 00056 if (socket.connect(XivelyHostname, 80) < 0) 00057 { 00058 pc.printf("\r\nUnable to connect to (%s) on port (%d)\r\n", XivelyHostname, 80); 00059 socket.close(); 00060 } 00061 else 00062 { 00063 // Block for 1 second 00064 socket.set_blocking( true, 1000 ); 00065 counter ++; 00066 00067 // Build the header 00068 httpCmdLen = sprintf(&tmpBuffer[0], "PUT %s.csv HTTP/1.1\r\n", XivelyPath ); 00069 httpCmdLen += sprintf(&tmpBuffer[httpCmdLen], "Host: %s\r\nUser-Agent: WiFi-DipCortex\r\n", XivelyHostname); 00070 httpCmdLen += sprintf(&tmpBuffer[httpCmdLen], "X-ApiKey: %s\r\n", XivelyApiKey); 00071 // TODO : hardcoded data length of 91, using printf with padding to fix the string length 00072 httpCmdLen += sprintf(&tmpBuffer[httpCmdLen], "Content-Type: text/csv\r\nContent-Length: 91\r\n"); 00073 httpCmdLen += sprintf(&tmpBuffer[httpCmdLen], "Connection: close\r\n\r\n"); 00074 00075 // add the data 00076 httpCmdLen += sprintf(&tmpBuffer[httpCmdLen], "ADC0, %05d\r\nADC1, %05d\r\nADC2, %05d\r\nADC3, %05d\r\nADC5, %05d\r\nADC6, %05d\r\nADC7, %05d\r\n\0", 00077 adc0.read_u16(), adc1.read_u16(), adc2.read_u16(), adc3.read_u16(), adc5.read_u16(), adc6.read_u16(), adc7.read_u16()); 00078 00079 pc.printf("Data to be sent : \r\n %s", tmpBuffer); 00080 00081 pc.printf("Posting ADC's to Xively \r\n"); 00082 res = socket.send_all(tmpBuffer, httpCmdLen); 00083 00084 if ( res > 0 ) 00085 { 00086 pc.printf("%05d : Data Sent \r\n", counter); 00087 } 00088 else 00089 { 00090 pc.printf("Failed to send\r\n"); 00091 break; 00092 } 00093 00094 res = socket.receive(tmpBuffer, 512); 00095 00096 if ( res > 0 ) 00097 { 00098 pc.printf("TCP Socket Recv'd : \r\n %s", tmpBuffer); 00099 tmpBuffer[res] = '\0'; 00100 } 00101 else 00102 { 00103 tmpBuffer[0] = '\0'; 00104 pc.printf("TCP : Failed to Recv\r\n"); 00105 break; 00106 } 00107 00108 socket.close(); 00109 } 00110 00111 wait_ms(1000); 00112 00113 if ( pc.readable() ) 00114 { 00115 pc.printf("Ending Xively Post \r\n"); 00116 pc.getc(); 00117 break; 00118 } 00119 } 00120 } 00121 00122 // ------------------------------------------------------------------------------------------------------------ 00123 /*! 00124 @brief Exercise the HTTP Client library 00125 */ 00126 // ------------------------------------------------------------------------------------------------------------ 00127 void HttpClientTest ( void ) 00128 { 00129 00130 //GET data 00131 pc.printf("\r\nTrying to fetch page... \r\n"); 00132 int ret = http.get("http://mbed.org/media/uploads/donatien/hello.txt", tmpBuffer, 128); 00133 if (!ret) 00134 { 00135 pc.printf("Page fetched successfully - read %d characters \r\n", strlen(tmpBuffer)); 00136 pc.printf("Result: %s \r\n", tmpBuffer); 00137 } 00138 else 00139 { 00140 pc.printf("Error - ret = %d - HTTP return code = %d \r\n", tmpBuffer, http.getHTTPResponseCode()); 00141 } 00142 00143 //POST data 00144 HTTPMap map; 00145 HTTPText inText(tmpBuffer, 512); 00146 map.put("Hello", "World"); 00147 map.put("test", "1234"); 00148 pc.printf(" \r\nTrying to post data... \r\n"); 00149 ret = http.post("http://httpbin.org/post", map, &inText); 00150 if (!ret) 00151 { 00152 pc.printf("Executed POST successfully - read %d characters \r\n", strlen(tmpBuffer)); 00153 pc.printf("Result: %s \r\n", tmpBuffer); 00154 } 00155 else 00156 { 00157 pc.printf("Error - ret = %d - HTTP return code = %d \r\n", ret, http.getHTTPResponseCode()); 00158 } 00159 00160 //PUT data 00161 strcpy(tmpBuffer, "This is a PUT test!"); 00162 HTTPText outText(tmpBuffer); 00163 //HTTPText inText(tmpBuffer, 512); 00164 pc.printf(" \r\nTrying to put resource... \r\n"); 00165 ret = http.put("http://httpbin.org/put", outText, &inText); 00166 if (!ret) 00167 { 00168 pc.printf("Executed PUT successfully - read %d characters \r\n", strlen(tmpBuffer)); 00169 pc.printf("Result: %s \r\n", tmpBuffer); 00170 } 00171 else 00172 { 00173 pc.printf("Error - ret = %d - HTTP return code = %d \r\n", ret, http.getHTTPResponseCode()); 00174 } 00175 00176 //DELETE data 00177 //HTTPText inText(tmpBuffer, 512); 00178 pc.printf(" \r\nTrying to delete resource... \r\n"); 00179 ret = http.del("http://httpbin.org/delete", &inText); 00180 if (!ret) 00181 { 00182 pc.printf("Executed DELETE successfully - read %d characters \r\n", strlen(tmpBuffer)); 00183 pc.printf("Result: %s \r\n", tmpBuffer); 00184 } 00185 else 00186 { 00187 pc.printf("Error - ret = %d - HTTP return code = %d \r\n", ret, http.getHTTPResponseCode()); 00188 } 00189 } 00190 00191 // ------------------------------------------------------------------------------------------------------------ 00192 /*! 00193 @brief Open a WebSocket, send a string 00194 */ 00195 // ------------------------------------------------------------------------------------------------------------ 00196 void WebSocketAdcStream ( void ) 00197 { 00198 00199 int res = 0; 00200 uint16_t counter = 0; 00201 uint16_t reconnects = 0; 00202 uint8_t myMAC[8]; 00203 00204 wifi.get_mac_address(myMAC); 00205 00206 Websocket ws((char *)WEB_SOCKET_URL); 00207 if ( ws.connect() ) 00208 { 00209 pc.printf("Connected to websocket server.\r\n"); 00210 00211 pc.printf("\r\n!! Press any key to stop sending !!\r\n\r\n"); 00212 while (1) 00213 { 00214 counter ++; 00215 sprintf(tmpBuffer, "{\"id\":\"wifly_acc\",\"ax\":\"%d\",\"ay\":\"%d\",\"az\":\"%d\"}", adc0.read_u16(), adc1.read_u16(), adc2.read_u16()); 00216 00217 if ( wifi.is_connected() ) 00218 { 00219 res = ws.send(tmpBuffer); 00220 pc.printf("Reconnects : %05d, Messages Sent : %05d, Websocket send returned : %d.\r\n", reconnects, counter, res); 00221 00222 if ( -1 == res ) 00223 { 00224 pc.printf("Websocket Failure, reconnecting .... \r\n"); 00225 ws.close(); 00226 if ( ws.connect() ) 00227 { 00228 // Reconnected 00229 reconnects ++; 00230 } 00231 else 00232 { 00233 // Failure! 00234 break; 00235 } 00236 } 00237 00238 wait_ms(1000); 00239 } 00240 else 00241 { 00242 pc.printf("WiFi Connection Lost .... \r\n"); 00243 } 00244 00245 if ( pc.readable() ) 00246 { 00247 pc.printf("Closing Socket \r\n"); 00248 pc.getc(); 00249 break; 00250 } 00251 } 00252 00253 ws.close(); 00254 pc.printf("Websocket Closed \r\n"); 00255 } 00256 else 00257 { 00258 pc.printf("Websocket connection failed\r\n"); 00259 } 00260 } 00261 00262 // ------------------------------------------------------------------------------------------------------------ 00263 /*! 00264 @brief Open a WebSocket, send a string 00265 */ 00266 // ------------------------------------------------------------------------------------------------------------ 00267 void WebSocketTest ( void ) 00268 { 00269 00270 int res = 0; 00271 uint16_t counter = 0; 00272 uint16_t reconnects = 0; 00273 uint8_t myMAC[8]; 00274 00275 wifi.get_mac_address(myMAC); 00276 00277 Websocket ws((char *)WEB_SOCKET_URL); 00278 if ( ws.connect() ) 00279 { 00280 pc.printf("Connected to websocket server.\r\n"); 00281 00282 pc.printf("\r\n!! Press any key to stop sending !!\r\n\r\n"); 00283 while (1) 00284 { 00285 counter ++; 00286 sprintf(tmpBuffer, "WiFi DipCortex / CC3000 - %05d - %02x:%02x:%02x:%02x:%02x:%02x\r\n", counter, myMAC[0], myMAC[1], myMAC[2], myMAC[3], myMAC[4], myMAC[5]); 00287 00288 if ( wifi.is_connected() ) 00289 { 00290 res = ws.send(tmpBuffer); 00291 pc.printf("Reconnects : %05d, Messages Sent : %05d, Websocket send returned : %d.\r\n", reconnects, counter, res); 00292 00293 if ( -1 == res ) 00294 { 00295 pc.printf("Websocket Failure, reconnecting .... \r\n"); 00296 ws.close(); 00297 if ( ws.connect() ) 00298 { 00299 // Reconnected 00300 reconnects ++; 00301 } 00302 else 00303 { 00304 // Failure! 00305 break; 00306 } 00307 } 00308 00309 wait_ms(1000); 00310 } 00311 else 00312 { 00313 pc.printf("WiFi Connection Lost .... \r\n"); 00314 } 00315 00316 if ( pc.readable() ) 00317 { 00318 pc.printf("Closing Socket \r\n"); 00319 pc.getc(); 00320 break; 00321 } 00322 } 00323 00324 ws.close(); 00325 pc.printf("Websocket Closed \r\n"); 00326 } 00327 else 00328 { 00329 pc.printf("Websocket connection failed\r\n"); 00330 } 00331 } 00332 00333 // ------------------------------------------------------------------------------------------------------------ 00334 /*! 00335 @brief Open a WebSocket, send a string 00336 */ 00337 // ------------------------------------------------------------------------------------------------------------ 00338 void WebSocketReadTest ( void ) 00339 { 00340 00341 int res = 0; 00342 uint16_t counter = 0; 00343 uint16_t reconnects = 0; 00344 uint8_t myMAC[8]; 00345 00346 wifi.get_mac_address(myMAC); 00347 00348 Websocket ws((char *)WEB_SOCKET_URL); 00349 if ( ws.connect() ) 00350 { 00351 pc.printf("Connected to websocket server.\r\n"); 00352 00353 pc.printf("\r\n!! Press any key to stop receiving !!\r\n\r\n"); 00354 while (1) 00355 { 00356 counter ++; 00357 //sprintf(tmpBuffer, "WiFi DipCortex / CC3000 - %05d - %02x:%02x:%02x:%02x:%02x:%02x\r\n", counter, myMAC[0], myMAC[1], myMAC[2], myMAC[3], myMAC[4], myMAC[5]); 00358 00359 if ( wifi.is_connected() ) 00360 { 00361 res = ws.read(tmpBuffer); 00362 00363 if ( res ) 00364 { 00365 pc.printf("Websocket Received : %s (Reconnects : %05d, Messages Recv : %05d)\r\n", tmpBuffer, reconnects, counter); 00366 } 00367 00368 if ( ws.is_connected() ) 00369 { 00370 00371 } 00372 else 00373 { 00374 pc.printf("Websocket Closed, reconnecting .... \r\n"); 00375 ws.close(); 00376 if ( ws.connect() ) 00377 { 00378 // Reconnected 00379 reconnects ++; 00380 } 00381 else 00382 { 00383 // Failure! 00384 break; 00385 } 00386 } 00387 } 00388 else 00389 { 00390 pc.printf("WiFi Connection Lost .... \r\n"); 00391 } 00392 00393 if ( pc.readable() ) 00394 { 00395 pc.printf("Closing Socket \r\n"); 00396 pc.getc(); 00397 break; 00398 } 00399 } 00400 00401 ws.close(); 00402 pc.printf("Websocket Closed \r\n"); 00403 } 00404 else 00405 { 00406 pc.printf("Websocket connection failed\r\n"); 00407 } 00408 } 00409 00410 // ------------------------------------------------------------------------------------------------------------ 00411 /*! 00412 @brief Open a TCP port send a string and wait for a reply 00413 */ 00414 // ------------------------------------------------------------------------------------------------------------ 00415 void TcpClientTest ( void ) 00416 { 00417 uint16_t counter = 0; 00418 TCPSocketConnection socket; 00419 int n = 0; 00420 00421 if (socket.connect(ECHO_SERVER_ADDRESS, ECHO_SERVER_PORT_TCP) < 0) 00422 { 00423 pc.printf("\r\nUnable to connect to (%s) on port (%d)\r\n", ECHO_SERVER_ADDRESS, ECHO_SERVER_PORT_TCP); 00424 } 00425 else 00426 { 00427 // Block for 1 second 00428 socket.set_blocking( true, 1000 ); 00429 00430 pc.printf("\r\n!! Press any key to stop sending !!\r\n\r\n"); 00431 while (1) 00432 { 00433 counter ++; 00434 00435 n = socket.send_all(hello, sizeof(hello) - 1); 00436 00437 if ( n > 0 ) 00438 { 00439 pc.printf("%05d : TCP Socket Sent : Hello World\r\n", counter); 00440 } 00441 else 00442 { 00443 pc.printf("Failed to send\r\n"); 00444 break; 00445 } 00446 00447 n = socket.receive(tmpBuffer, 256); 00448 00449 if ( n > 0 ) 00450 { 00451 pc.printf("TCP Socket Recv'd : %s \r\n", tmpBuffer); 00452 tmpBuffer[n] = '\0'; 00453 } 00454 else 00455 { 00456 tmpBuffer[0] = '\0'; 00457 pc.printf("TCP : Failed to Recv\r\n"); 00458 break; 00459 } 00460 00461 wait_ms(50); 00462 00463 // Should we stop? 00464 if ( pc.readable() ) 00465 { 00466 pc.printf("Closing Socket \r\n"); 00467 pc.getc(); 00468 break; 00469 } 00470 } 00471 if ( wifi.is_connected() ) 00472 { 00473 socket.close(); 00474 } 00475 pc.printf("Completed.\r\n"); 00476 } 00477 } 00478 00479 00480 // ------------------------------------------------------------------------------------------------------------ 00481 /*! 00482 @brief Opens a sockets to listen for connections, upon connection a message is sent and the 00483 client disconnected 00484 */ 00485 // ------------------------------------------------------------------------------------------------------------ 00486 void TcpServerTest ( void ) 00487 { 00488 int32_t status; 00489 TCPSocketServer server; 00490 TCPSocketConnection client; 00491 00492 server.bind(80); 00493 server.listen(); 00494 pc.printf("\r\n!! Press any key to stop listening !!\r\n\r\n"); 00495 while (1) 00496 { 00497 status = server.accept(client); 00498 if (status >= 0) 00499 { 00500 client.set_blocking(false, 1500); // Timeout after (1.5)s 00501 pc.printf("Connection from: %s \r\n", client.get_address()); 00502 //client.receive(buffer, sizeof(buffer)); 00503 //pc.printf("Received: %s \r\n",buffer); 00504 pc.printf("Sending the message to the server. \r\n"); 00505 client.send_all(hello, sizeof(hello)); 00506 client.close(); 00507 } 00508 00509 // Should we stop? 00510 if ( pc.readable() ) 00511 { 00512 pc.printf("Closing Socket \r\n"); 00513 pc.getc(); 00514 break; 00515 } 00516 00517 if (! wifi.is_connected() ) 00518 { 00519 pc.printf("WiFi Connection lost \r\n"); 00520 break; 00521 } 00522 } 00523 00524 if ( wifi.is_connected() ) 00525 { 00526 server.close(); 00527 } 00528 }
Generated on Wed Jul 13 2022 03:02:38 by
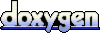