pH works but not temp
Dependencies: BLE_nRF8001 DebounceIn mbed mbed_BLEtry2
Fork of mbed_BLEtry2 by
main.cpp
00001 #include "mbed.h" 00002 #include "BLEPeripheral.h" 00003 00004 //serial connection via USB 00005 Serial serial(USBTX, USBRX); 00006 00007 //Declare Input and Output pins 00008 DigitalIn pb(D3); 00009 AnalogIn pH_sensor(A0); 00010 InterruptIn button(D2); 00011 DigitalOut led(LED_RED); 00012 00013 // The SPI construct, REQN and RDYN IO construct should be modified manually 00014 // It depend on the board you are using and the REQN&RDYN configuration on BLE Shield 00015 SPI spi(PTD2, PTD3, PTD1); 00016 DigitalInOut BLE_RDY(PTD5); 00017 DigitalInOut BLE_REQ(PTD0); 00018 DigitalInOut BLE_RESET(PTA13); 00019 00020 //for BLE 00021 int count = 0; 00022 unsigned char tempbuf[16] = {0}; 00023 unsigned char templen = 0; 00024 unsigned char decbuf[16] = {0}; 00025 unsigned char declen = 0; 00026 unsigned char pHbuf[16] = {0}; 00027 unsigned char pHlen = 0; 00028 00029 /*----- BLE Utility -------------------------------------------------------------------------*/ 00030 // create peripheral instance, see pinouts above 00031 BLEPeripheral blePeripheral = BLEPeripheral(&BLE_REQ, &BLE_RDY, NULL); 00032 00033 // create service 00034 BLEService uartService = BLEService("713d0000503e4c75ba943148f18d941e"); 00035 00036 // create characteristic 00037 BLECharacteristic txCharacteristic = BLECharacteristic("713d0002503e4c75ba943148f18d941e", BLENotify, 30, 0); 00038 BLECharacteristic rxCharacteristic = BLECharacteristic("713d0003503e4c75ba943148f18d941e", BLENotify, 20, 1); 00039 /*--------------------------------------------------------------------------------------------*/ 00040 unsigned int interval = 0; 00041 unsigned char count_on = 0; 00042 00043 //for pH measurement 00044 int calib = 0; 00045 int avgReading = 0; 00046 bool calibrationORnot = true; 00047 bool ready = false; 00048 int* reading[] = {0,0}; 00049 00050 00051 //This loop takes a reading by taking 15 measurements and averaging them when it is called in the loop "measure" 00052 int takeReading() 00053 { 00054 double totalVal = 0.0; 00055 double pH_sensor_read = 0.0; 00056 int totalReadings = 0; 00057 while(totalReadings < 16){ 00058 pH_sensor_read = pH_sensor.read(); 00059 totalVal = totalVal + pH_sensor_read; 00060 totalReadings++; 00061 } 00062 int avgSensor = totalVal/15; 00063 return avgSensor; 00064 } 00065 00066 void calibration() 00067 { 00068 //serial.printf("enter calibration loop\n"); 00069 //Loop to take calibration value 00070 calib = takeReading(); 00071 //serial.printf("calib is %d\n", calib); 00072 } 00073 00074 void calculate() 00075 { 00076 //serial.printf("entered calc\n"); 00077 //Take average of readings over 10 seconds 00078 avgReading = takeReading(); 00079 //serial.printf("took reading\n"); 00080 ready = true; 00081 } 00082 00083 void measure() 00084 { 00085 serial.baud(115200); 00086 //serial.printf("start measurement"); 00087 //cb.mode(PullUp); 00088 if(calibrationORnot) 00089 { 00090 calibration(); 00091 calibrationORnot = !calibrationORnot; 00092 } 00093 //Loop to take measurement 00094 else if(!calibrationORnot) 00095 { 00096 // serial.printf("enter measurement loop\n"); 00097 // serial.printf("calib is %d\n", calib); 00098 calculate(); 00099 } 00100 } 00101 00102 00103 int main() 00104 { 00105 pb.mode(PullUp); 00106 serial.baud(115200); 00107 serial.printf("Hello SmartD!\n"); 00108 serial.printf("Serial begin!\r\n"); 00109 float pH,yint,tempC,tempF; 00110 00111 /*----- BLE Utility ---------------------------------------------*/ 00112 // set advertised local name and service UUID 00113 blePeripheral.setLocalName("Sita"); 00114 00115 blePeripheral.setAdvertisedServiceUuid(uartService.uuid()); 00116 00117 // add service and characteristic 00118 blePeripheral.addAttribute(uartService); 00119 blePeripheral.addAttribute(rxCharacteristic); 00120 blePeripheral.addAttribute(txCharacteristic); 00121 00122 // begin initialization 00123 blePeripheral.begin(); 00124 /*---------------------------------------------------------------*/ 00125 00126 //serial.printf("GOT IT pH is %d, Temp is %d\n\r", reading[0], reading[1]); 00127 serial.printf("\nBLE UART Peripheral begin!\r\n"); 00128 if (calibrationORnot) 00129 { 00130 button.rise(&measure); 00131 } 00132 00133 while(1) 00134 { 00135 BLECentral central = blePeripheral.central(); 00136 if (ready) 00137 { 00138 //calcuating yintercept of the ADC -> pH equation using calibration value and given that calibration liquid is pH4 00139 yint = (68.97*calib)-4; 00140 //serial.printf("yint is %f", yint); 00141 //assumes linear relationship between pin readout -> voltage ->pH 00142 pH = (0.0209*3300)*(avgReading)-yint; 00143 //conversion to degrees C from sensor output voltage 00144 tempC = (((3000*avgReading/1000) - 1.022129)/-0.0018496); 00145 tempF = (tempC *9/5) + 32; 00146 //print current pH and temp 00147 serial.printf("pH = %f & temp = %f F\n\r", pH, tempF); 00148 ready = !ready; 00149 } 00150 00151 if (central) 00152 { 00153 // central connected to peripheral 00154 serial.printf("Connected to central\r\n"); 00155 while (central.connected()) 00156 { 00157 if(!pb) 00158 { 00159 tempF = 98.5; 00160 int tempint = tempF; 00161 int tempdecimal = (tempF-tempint)*100; 00162 int pHint = pH; 00163 int pHdecimal = (pH-pHint)*100; 00164 // int tempint = 20; 00165 // int tempdecimal = 23; 00166 // int pHint = 9; 00167 tempbuf[0]= tempint; 00168 tempbuf[1] = tempdecimal; 00169 //tempbuf[templen] = tempint; 00170 //decbuf[declen] = decimal; 00171 pHbuf[0] = pHint; 00172 pHbuf[1] = pHdecimal; 00173 interval++; 00174 } 00175 00176 if(interval == 1) 00177 { // If there is no char available last the interval, send the received chars to central. 00178 //serial.printf("Received from terminal: %d bytes\r\n", templen); 00179 txCharacteristic.setValue((const unsigned char *)tempbuf, 2); 00180 txCharacteristic.setValue((const unsigned char *)pHbuf, 2); 00181 serial.printf("done"); 00182 wait(2); 00183 interval = 0; 00184 } 00185 } 00186 // central disconnected 00187 serial.printf("Disconnected from central\r\n"); 00188 } 00189 } 00190 }
Generated on Tue Aug 2 2022 05:54:55 by
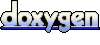