
Display four graphs of each value (NTC, variable resistor, DHT11-Temperature, DHT11-Humidity).
Dependencies: SSD1308_128x64_I2C Grove_temperature DHT
main.cpp
00001 #include "mbed.h" 00002 #include "SSD1308.h" 00003 #include "logo.h" 00004 #include "Grove_temperature.h" 00005 #include "DHT.h" 00006 #include<stdio.h> 00007 00008 ////OLED 00009 I2C i2c(PB_9,PB_8); 00010 SSD1308 oled = SSD1308(&i2c, SSD1308_SA0); 00011 ///////////// 00012 Grove_temperature temp(PA_1); //thermistance 00013 00014 DigitalOut myled(LED1); //DHT11 // we will use the led embedded on the map 00015 DHT dht11(PB_10,DHT11); 00016 00017 AnalogIn potar(A3); //potaranalogic 00018 00019 ///////////// 00020 InterruptIn mybutton(USER_BUTTON); 00021 int j=1; 00022 int count=1; 00023 int tem1[30]; 00024 00025 void pressed() 00026 { 00027 oled.clearDisplay_zone(); //clear the zone of displaying graph 00028 j++; 00029 if(j>4)j=1; 00030 count=1; 00031 for(int t=0;t<30;t++) 00032 tem1[t]=32; ///The data is line 32 of the grah2_point[][4] array, which is {0x00,0x00,0x00,0x00} 00033 00034 } 00035 00036 00037 //////main 00038 int main() { 00039 int i; 00040 float x; 00041 00042 00043 00044 int err; 00045 float temperature; 00046 float humidite; 00047 00048 char buffer_1[10]; 00049 char buffer_2[10]; 00050 char buffer_3[10]; 00051 char buffer_4[10]; 00052 00053 oled.display_Axis_y(); 00054 oled.display_Axis_x(); 00055 00056 while (true) 00057 { 00058 mybutton.fall(&pressed); 00059 switch(j) 00060 {case 1: 00061 for(i=0;i<30;i++) 00062 { 00063 x=temp.getTemperature(); 00064 if(count==1)tem1[i]=(int)(x-15);//offset 00065 else 00066 { 00067 for(int m=0;m<29;m++) 00068 { tem1[m]=tem1[m+1]; } 00069 tem1[29]=(int)(x-15); 00070 } 00071 00072 printf("temperature = %2d\n", tem1[i]); 00073 sprintf(buffer_1, "tem_NTC: %5.2f C",x); 00074 oled.setHorizontalAddressingMode(); 00075 oled.writeString(0,0,buffer_1); 00076 00077 if(j!=1) 00078 { 00079 oled.clearDisplay_zone(); //clear the zone of displaying graph 00080 i=30; //End the loop 00081 count=1; 00082 for(int t=0;t<30;t++) //Empty array 00083 tem1[t]=32; ///The data is line 32 of the grah2_point[][4] array, which is {0x00,0x00,0x00,0x00} 00084 } 00085 else if(count==1) oled.graph(tem1,i,count); 00086 else if(count!=1) oled.graph(tem1,30,count); 00087 } 00088 count++; 00089 break; 00090 00091 case 2: //////display temperature of DHT11 00092 for(i=0;i<30;i++) 00093 { 00094 err = dht11.readData(); // data recovery 00095 if (err == 0) { 00096 temperature = dht11.ReadTemperature(CELCIUS); 00097 if(count==1) tem1[i]=(int)(temperature-15); 00098 else 00099 { 00100 for(int m=0;m<29;m++) 00101 { tem1[m]=tem1[m+1]; } 00102 tem1[29]=(int)(temperature-15); 00103 } 00104 printf("Temperature : %4.2f C \n",temperature); // sends data serial port (default 9600bauds) 00105 00106 00107 } 00108 else { 00109 printf("\r\nErreur %i \n",err); 00110 tem1[i]=32; 00111 } 00112 00113 sprintf(buffer_2, "Tem_DHT: %5.2f C", temperature); 00114 oled.setHorizontalAddressingMode(); 00115 oled.writeString(0,0,buffer_2); 00116 00117 if(j!=2) 00118 { 00119 oled.clearDisplay_zone(); 00120 i=30; 00121 count=1; 00122 for(int t=0;t<30;t++) 00123 tem1[t]=32; 00124 } 00125 else if(count==1) {oled.graph(tem1,i,count); wait(2.1);} 00126 else if(count!=1) {oled.graph(tem1,30,count); wait(2.1);} 00127 } 00128 count++; 00129 break; 00130 00131 case 3: //////////display humidite of DHT11 00132 for(i=0;i<30;i++) 00133 { 00134 err = dht11.readData(); // data recovery 00135 if (err == 0) { 00136 humidite = dht11.ReadHumidity(); 00137 if(count==1) tem1[i]=(int)(humidite/5); 00138 else 00139 { 00140 for(int m=0;m<29;m++) 00141 { tem1[m]=tem1[m+1]; } 00142 tem1[29]=(int)(humidite/5); 00143 } 00144 printf("Humidite : %4.2f %% \n",humidite); 00145 00146 } 00147 else 00148 printf("\r\nErreur %i \n",err); 00149 00150 00151 sprintf(buffer_3, "Hum_DHT: %5.2f%% ", humidite); 00152 oled.setHorizontalAddressingMode(); 00153 oled.writeString(0,0,buffer_3); 00154 00155 if(j!=3) 00156 { 00157 oled.clearDisplay_zone(); 00158 i=30; 00159 count=1; 00160 for(int t=0;t<30;t++) 00161 tem1[t]=32; 00162 } 00163 else if(count==1) {oled.graph(tem1,i,count); wait(2.1);} 00164 else if(count!=1) {oled.graph(tem1,30,count); wait(2.1);} 00165 } 00166 count++; 00167 break; 00168 00169 case 4: ////////////////////potaranalogic 00170 for(i=0;i<30;i++) 00171 { 00172 x=potar.read_u16()/65535.0; 00173 if(count==1) tem1[i]=(int)(x*25); 00174 else 00175 { 00176 for(int m=0;m<29;m++) 00177 { tem1[m]=tem1[m+1]; } 00178 tem1[29]=(int)(x*25); 00179 } 00180 printf("normalized value(0-1):%3.3f\n",x); 00181 00182 sprintf(buffer_4, "potarlogic:%5.2f", potar.read_u16()/65535.0); 00183 oled.setHorizontalAddressingMode(); 00184 oled.writeString(0,0,buffer_4); 00185 00186 if(j!=4) 00187 { 00188 oled.clearDisplay_zone(); 00189 i=30; 00190 count=1; 00191 for(int t=0;t<30;t++) 00192 tem1[t]=32; 00193 } 00194 else if(count==1) {oled.graph(tem1,i,count); wait(1);} 00195 else if(count!=1) {oled.graph(tem1,30,count); wait(1);} 00196 } 00197 count++; 00198 break; 00199 00200 } 00201 00202 } 00203 00204 } 00205
Generated on Tue Jul 26 2022 20:00:21 by
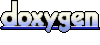