
display a graph of temperature from the NTC on the OLED
Dependencies: SSD1308_128x64_I2C Grove_temperature
main.cpp
00001 #include "mbed.h" 00002 #include "SSD1308.h" 00003 #include "wave.h" 00004 #include "Grove_temperature.h" 00005 00006 #define NUM_TEMP 64 //The number of points that will be displayed on the OLED 00007 00008 AnalogIn varRes(A3); //variable resistor 00009 Grove_temperature temp_obj(A1); //Create a Crove_temperature object named "temp_obj", it's connected to Pin A1. 00010 I2C i2c(PB_9,PB_8); //The OLED uses port I2C to connect to the L073RZ 00011 SSD1308 oled = SSD1308(&i2c, SSD1308_SA0); 00012 00013 void tempArrayInit(Temperature *p); //temperature struct initialization 00014 void tempArrayRefresh(Temperature *p); //temperature struct refresh 00015 void tempArrayDisplayOnOLED(Temperature *p);//temperature struct display the point on the OLED 00016 00017 int main() { 00018 Temperature t[NUM_TEMP]; //temperature array 00019 char buffer[10]; //store a string 00020 int interval; //refresh time 00021 00022 tempArrayInit(t); //temperature array initialization 00023 oled.clearDisplay(); 00024 while(1){ 00025 interval = (int)(varRes.read() * 200); //variable resistor control the refresh speed 00026 wait_ms(interval); 00027 00028 tempArrayRefresh(t); //refresh the array 00029 tempArrayDisplayOnOLED(t); //display the pixel about the tempearture 00030 00031 sprintf(buffer," T:%2dC",t[NUM_TEMP - 1].u8_temperature); //sprintf the temperature to the buffer 00032 oled.writeString(3,8,buffer); 00033 // sprintf(buffer," t:%3dms",interval); //sprintf the temperature to the buffer 00034 // oled.writeString(5,8,buffer); 00035 } 00036 } 00037 00038 void tempArrayInit(Temperature *p) 00039 { 00040 uint8_t i; 00041 for(i = 0;i < NUM_TEMP;i++){ 00042 p[i].u8_col = i; 00043 p[i].u8_temperature = i % 64; 00044 } 00045 } 00046 void tempArrayRefresh(Temperature *p) 00047 { 00048 //refresh array 00049 uint8_t i; 00050 for(i = 0;i < (NUM_TEMP - 1);i++){ 00051 p[i].u8_page = p[i + 1].u8_page; 00052 //don't refresh the column 00053 //p[i].u8_col = p[i + 1].u8_col; 00054 p[i].u8_temperature = p[i + 1].u8_temperature; 00055 p[i].u8_value = p[i + 1].u8_value; 00056 } 00057 00058 //get new value 00059 p[NUM_TEMP - 1].u8_temperature = (uint8_t)(temp_obj.getTemperature()); 00060 00061 //calculate value 00062 p[NUM_TEMP - 1].calcPage(); 00063 p[NUM_TEMP - 1].calcValue(); 00064 } 00065 00066 void tempArrayDisplayOnOLED(Temperature *p) 00067 { 00068 uint8_t i; 00069 00070 for(i = 0;i < NUM_TEMP;i++){ 00071 00072 oled.writeBitmap(&p[i].u8_value,p[i].u8_page,p[i].u8_page,p[i].u8_col,p[i].u8_col); 00073 /* clear the data on other pages withins the same column*/ 00074 for(uint8_t j = 0;j < 8;j++){ 00075 if(j == p[i].u8_page){ 00076 continue; 00077 } 00078 else{ 00079 oled.writeBitmap(0,j,j,p[i].u8_col,p[i].u8_col); 00080 } 00081 } 00082 00083 } 00084 }
Generated on Sun Jul 17 2022 22:34:28 by
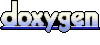