fdsf
Dependents: sisk_proj_stat MQTT Hello_FXOS8700Q WireFSHandControl ... more
vj.h
00001 /* 00002 * Definitions for tcp compression routines. 00003 * 00004 * $Id: vj.h,v 1.7 2010/02/22 17:52:09 goldsimon Exp $ 00005 * 00006 * Copyright (c) 1989 Regents of the University of California. 00007 * All rights reserved. 00008 * 00009 * Redistribution and use in source and binary forms are permitted 00010 * provided that the above copyright notice and this paragraph are 00011 * duplicated in all such forms and that any documentation, 00012 * advertising materials, and other materials related to such 00013 * distribution and use acknowledge that the software was developed 00014 * by the University of California, Berkeley. The name of the 00015 * University may not be used to endorse or promote products derived 00016 * from this software without specific prior written permission. 00017 * THIS SOFTWARE IS PROVIDED ``AS IS'' AND WITHOUT ANY EXPRESS OR 00018 * IMPLIED WARRANTIES, INCLUDING, WITHOUT LIMITATION, THE IMPLIED 00019 * WARRANTIES OF MERCHANTIBILITY AND FITNESS FOR A PARTICULAR PURPOSE. 00020 * 00021 * Van Jacobson (van@helios.ee.lbl.gov), Dec 31, 1989: 00022 * - Initial distribution. 00023 */ 00024 00025 #ifndef VJ_H 00026 #define VJ_H 00027 00028 #include "lwip/ip.h" 00029 #include "lwip/tcp_impl.h" 00030 00031 #define MAX_SLOTS 16 /* must be > 2 and < 256 */ 00032 #define MAX_HDR 128 00033 00034 /* 00035 * Compressed packet format: 00036 * 00037 * The first octet contains the packet type (top 3 bits), TCP 00038 * 'push' bit, and flags that indicate which of the 4 TCP sequence 00039 * numbers have changed (bottom 5 bits). The next octet is a 00040 * conversation number that associates a saved IP/TCP header with 00041 * the compressed packet. The next two octets are the TCP checksum 00042 * from the original datagram. The next 0 to 15 octets are 00043 * sequence number changes, one change per bit set in the header 00044 * (there may be no changes and there are two special cases where 00045 * the receiver implicitly knows what changed -- see below). 00046 * 00047 * There are 5 numbers which can change (they are always inserted 00048 * in the following order): TCP urgent pointer, window, 00049 * acknowlegement, sequence number and IP ID. (The urgent pointer 00050 * is different from the others in that its value is sent, not the 00051 * change in value.) Since typical use of SLIP links is biased 00052 * toward small packets (see comments on MTU/MSS below), changes 00053 * use a variable length coding with one octet for numbers in the 00054 * range 1 - 255 and 3 octets (0, MSB, LSB) for numbers in the 00055 * range 256 - 65535 or 0. (If the change in sequence number or 00056 * ack is more than 65535, an uncompressed packet is sent.) 00057 */ 00058 00059 /* 00060 * Packet types (must not conflict with IP protocol version) 00061 * 00062 * The top nibble of the first octet is the packet type. There are 00063 * three possible types: IP (not proto TCP or tcp with one of the 00064 * control flags set); uncompressed TCP (a normal IP/TCP packet but 00065 * with the 8-bit protocol field replaced by an 8-bit connection id -- 00066 * this type of packet syncs the sender & receiver); and compressed 00067 * TCP (described above). 00068 * 00069 * LSB of 4-bit field is TCP "PUSH" bit (a worthless anachronism) and 00070 * is logically part of the 4-bit "changes" field that follows. Top 00071 * three bits are actual packet type. For backward compatibility 00072 * and in the interest of conserving bits, numbers are chosen so the 00073 * IP protocol version number (4) which normally appears in this nibble 00074 * means "IP packet". 00075 */ 00076 00077 /* packet types */ 00078 #define TYPE_IP 0x40 00079 #define TYPE_UNCOMPRESSED_TCP 0x70 00080 #define TYPE_COMPRESSED_TCP 0x80 00081 #define TYPE_ERROR 0x00 00082 00083 /* Bits in first octet of compressed packet */ 00084 #define NEW_C 0x40 /* flag bits for what changed in a packet */ 00085 #define NEW_I 0x20 00086 #define NEW_S 0x08 00087 #define NEW_A 0x04 00088 #define NEW_W 0x02 00089 #define NEW_U 0x01 00090 00091 /* reserved, special-case values of above */ 00092 #define SPECIAL_I (NEW_S|NEW_W|NEW_U) /* echoed interactive traffic */ 00093 #define SPECIAL_D (NEW_S|NEW_A|NEW_W|NEW_U) /* unidirectional data */ 00094 #define SPECIALS_MASK (NEW_S|NEW_A|NEW_W|NEW_U) 00095 00096 #define TCP_PUSH_BIT 0x10 00097 00098 00099 /* 00100 * "state" data for each active tcp conversation on the wire. This is 00101 * basically a copy of the entire IP/TCP header from the last packet 00102 * we saw from the conversation together with a small identifier 00103 * the transmit & receive ends of the line use to locate saved header. 00104 */ 00105 struct cstate { 00106 struct cstate *cs_next; /* next most recently used state (xmit only) */ 00107 u_short cs_hlen; /* size of hdr (receive only) */ 00108 u_char cs_id; /* connection # associated with this state */ 00109 u_char cs_filler; 00110 union { 00111 char csu_hdr[MAX_HDR]; 00112 struct ip_hdr csu_ip; /* ip/tcp hdr from most recent packet */ 00113 } vjcs_u; 00114 }; 00115 #define cs_ip vjcs_u.csu_ip 00116 #define cs_hdr vjcs_u.csu_hdr 00117 00118 00119 struct vjstat { 00120 unsigned long vjs_packets; /* outbound packets */ 00121 unsigned long vjs_compressed; /* outbound compressed packets */ 00122 unsigned long vjs_searches; /* searches for connection state */ 00123 unsigned long vjs_misses; /* times couldn't find conn. state */ 00124 unsigned long vjs_uncompressedin; /* inbound uncompressed packets */ 00125 unsigned long vjs_compressedin; /* inbound compressed packets */ 00126 unsigned long vjs_errorin; /* inbound unknown type packets */ 00127 unsigned long vjs_tossed; /* inbound packets tossed because of error */ 00128 }; 00129 00130 /* 00131 * all the state data for one serial line (we need one of these per line). 00132 */ 00133 struct vjcompress { 00134 struct cstate *last_cs; /* most recently used tstate */ 00135 u_char last_recv; /* last rcvd conn. id */ 00136 u_char last_xmit; /* last sent conn. id */ 00137 u_short flags; 00138 u_char maxSlotIndex; 00139 u_char compressSlot; /* Flag indicating OK to compress slot ID. */ 00140 #if LINK_STATS 00141 struct vjstat stats; 00142 #endif 00143 struct cstate tstate[MAX_SLOTS]; /* xmit connection states */ 00144 struct cstate rstate[MAX_SLOTS]; /* receive connection states */ 00145 }; 00146 00147 /* flag values */ 00148 #define VJF_TOSS 1U /* tossing rcvd frames because of input err */ 00149 00150 extern void vj_compress_init (struct vjcompress *comp); 00151 extern u_int vj_compress_tcp (struct vjcompress *comp, struct pbuf *pb); 00152 extern void vj_uncompress_err (struct vjcompress *comp); 00153 extern int vj_uncompress_uncomp(struct pbuf *nb, struct vjcompress *comp); 00154 extern int vj_uncompress_tcp (struct pbuf **nb, struct vjcompress *comp); 00155 00156 #endif /* VJ_H */
Generated on Tue Jul 12 2022 15:52:22 by
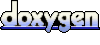