
LoRa Protocol Ricezione
Fork of SX1272PingPong by
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "main.h" 00003 #include "sx1272-hal.h" 00004 #include "debug.h" 00005 00006 00007 /* Set this flag to '1' to display debug messages on the console */ 00008 #define DEBUG_MESSAGE 1 00009 00010 /* Set this flag to '1' to use the LoRa modulation or to '0' to use FSK modulation */ 00011 #define USE_MODEM_LORA 1 00012 #define USE_MODEM_FSK !USE_MODEM_LORA 00013 00014 #define RF_FREQUENCY 868000000 // Hz 00015 #define TX_OUTPUT_POWER 14 // 14 dBm 00016 00017 #if USE_MODEM_LORA == 1 00018 00019 #define LORA_BANDWIDTH 2 // [0: 125 kHz, 00020 // 1: 250 kHz, 00021 // 2: 500 kHz, 00022 // 3: Reserved] 00023 #define LORA_SPREADING_FACTOR 7 // [SF7..SF12] 00024 #define LORA_CODINGRATE 1 // [1: 4/5, 00025 // 2: 4/6, 00026 // 3: 4/7, 00027 // 4: 4/8] 00028 #define LORA_PREAMBLE_LENGTH 8 // Same for Tx and Rx 00029 #define LORA_SYMBOL_TIMEOUT 5 // Symbols 00030 #define LORA_FIX_LENGTH_PAYLOAD_ON false 00031 #define LORA_FHSS_ENABLED false 00032 #define LORA_NB_SYMB_HOP 4 00033 #define LORA_IQ_INVERSION_ON false 00034 #define LORA_CRC_ENABLED true 00035 00036 #elif USE_MODEM_FSK == 1 00037 00038 #define FSK_FDEV 25000 // Hz 00039 #define FSK_DATARATE 19200 // bps 00040 #define FSK_BANDWIDTH 50000 // Hz 00041 #define FSK_AFC_BANDWIDTH 83333 // Hz 00042 #define FSK_PREAMBLE_LENGTH 5 // Same for Tx and Rx 00043 #define FSK_FIX_LENGTH_PAYLOAD_ON false 00044 #define FSK_CRC_ENABLED true 00045 00046 #else 00047 #error "Please define a modem in the compiler options." 00048 #endif 00049 00050 #define RX_TIMEOUT_VALUE 3500 // in ms 00051 #define BUFFER_SIZE 32 // Define the payload size here 00052 00053 #if( defined ( TARGET_KL25Z ) || defined ( TARGET_LPC11U6X ) ) 00054 DigitalOut led( LED2 ); 00055 #else 00056 DigitalOut led( LED1 ); 00057 #endif 00058 00059 Timer timer; 00060 00061 00062 /* 00063 * Global variables declarations 00064 */ 00065 typedef enum 00066 { 00067 LOWPOWER = 0, 00068 IDLE, 00069 00070 RX, 00071 RX_TIMEOUT, 00072 RX_ERROR, 00073 00074 TX, 00075 TX_TIMEOUT, 00076 00077 CAD, 00078 CAD_DONE 00079 }AppStates_t; 00080 00081 volatile AppStates_t State = LOWPOWER; 00082 00083 /*! 00084 * Radio events function pointer 00085 */ 00086 static RadioEvents_t RadioEvents; 00087 00088 /* 00089 * Global variables declarations 00090 */ 00091 SX1272MB2xAS Radio( NULL ); 00092 00093 const uint8_t PingMsg[] = "LOR3"; 00094 const uint8_t PongMs2[] = "LOR1"; 00095 const uint8_t PongMs3[] = "LOR2"; 00096 00097 uint16_t BufferSize = BUFFER_SIZE; 00098 uint8_t Buffer[BUFFER_SIZE]; 00099 00100 int16_t RssiValue = 0.0; 00101 int8_t SnrValue = 0.0; 00102 00103 int32_t TimerValue=0; 00104 int32_t TimeStampNew=0; 00105 int32_t TimeStampOld=0; 00106 00107 int main( void ) 00108 { 00109 uint8_t i; 00110 00111 00112 debug( "\n\n\r SX1272 Ping Pong Demo Application \n\n\r" ); 00113 00114 // Initialize Radio driver 00115 RadioEvents.TxDone = OnTxDone; 00116 RadioEvents.RxDone = OnRxDone; 00117 RadioEvents.RxError = OnRxError; 00118 RadioEvents.TxTimeout = OnTxTimeout; 00119 RadioEvents.RxTimeout = OnRxTimeout; 00120 Radio.Init( &RadioEvents ); 00121 00122 // verify the connection with the board 00123 while( Radio.Read( REG_VERSION ) == 0x00 ) 00124 { 00125 debug( "Radio could not be detected!\n\r", NULL ); 00126 wait( 1 ); 00127 } 00128 00129 debug_if( ( DEBUG_MESSAGE & ( Radio.DetectBoardType( ) == SX1272MB2XAS ) ), "\n\r > Board Type: SX1272MB2xAS < \n\r" ); 00130 00131 Radio.SetChannel( RF_FREQUENCY ); 00132 00133 #if USE_MODEM_LORA == 1 00134 00135 debug_if( LORA_FHSS_ENABLED, "\n\n\r > LORA FHSS Mode < \n\n\r" ); 00136 debug_if( !LORA_FHSS_ENABLED, "\n\n\r > LORA Mode < \n\n\r" ); 00137 00138 Radio.SetTxConfig( MODEM_LORA, TX_OUTPUT_POWER, 0, LORA_BANDWIDTH, 00139 LORA_SPREADING_FACTOR, LORA_CODINGRATE, 00140 LORA_PREAMBLE_LENGTH, LORA_FIX_LENGTH_PAYLOAD_ON, 00141 LORA_CRC_ENABLED, LORA_FHSS_ENABLED, LORA_NB_SYMB_HOP, 00142 LORA_IQ_INVERSION_ON, 2000 ); 00143 00144 Radio.SetRxConfig( MODEM_LORA, LORA_BANDWIDTH, LORA_SPREADING_FACTOR, 00145 LORA_CODINGRATE, 0, LORA_PREAMBLE_LENGTH, 00146 LORA_SYMBOL_TIMEOUT, LORA_FIX_LENGTH_PAYLOAD_ON, 0, 00147 LORA_CRC_ENABLED, LORA_FHSS_ENABLED, LORA_NB_SYMB_HOP, 00148 LORA_IQ_INVERSION_ON, true ); 00149 00150 #elif USE_MODEM_FSK == 1 00151 00152 debug("\n\n\r > FSK Mode < \n\n\r" ); 00153 Radio.SetTxConfig( MODEM_FSK, TX_OUTPUT_POWER, FSK_FDEV, 0, 00154 FSK_DATARATE, 0, 00155 FSK_PREAMBLE_LENGTH, FSK_FIX_LENGTH_PAYLOAD_ON, 00156 FSK_CRC_ENABLED, 0, 0, 0, 2000 ); 00157 00158 Radio.SetRxConfig( MODEM_FSK, FSK_BANDWIDTH, FSK_DATARATE, 00159 0, FSK_AFC_BANDWIDTH, FSK_PREAMBLE_LENGTH, 00160 0, FSK_FIX_LENGTH_PAYLOAD_ON, 0, FSK_CRC_ENABLED, 00161 0, 0, false, true ); 00162 00163 #else 00164 00165 #error "Please define a modem in the compiler options." 00166 00167 #endif 00168 00169 debug_if( DEBUG_MESSAGE, "Starting Ping-Pong loop\r\n" ); 00170 00171 led = 0; 00172 00173 Radio.Rx( RX_TIMEOUT_VALUE ); 00174 00175 timer.start(); 00176 00177 while( 1 ) 00178 { 00179 switch(State){ 00180 case RX: 00181 //timer.stop(); 00182 00183 //timer.reset(); 00184 //timer.start(); 00185 if( strncmp( ( const char* )Buffer, ( const char* )PongMs2, 4 ) == 0 ) 00186 { 00187 TimeStampNew=timer.read_ms(); 00188 TimerValue=TimeStampNew-TimeStampOld; 00189 TimeStampOld=TimeStampNew; 00190 00191 led = !led; 00192 debug( "I am LOR2\r\n" ); 00193 printf("Data received from...%s\r\n",Buffer); 00194 printf("TimeStamp %d\n\r",TimeStampNew); 00195 printf("Time %d\n\r",TimerValue); 00196 Radio.Sleep( ); 00197 Buffer[0] = 'A'; 00198 }//end if1 00199 wait_ms(10); 00200 Radio.Rx( RX_TIMEOUT_VALUE );//SETTA LA RADIO IN MODALITA' RICEZIONE 00201 00202 break; 00203 00204 default: 00205 break; 00206 }; 00207 }//end while 00208 }//end main 00209 00210 void OnTxDone( void ) 00211 { 00212 Radio.Sleep( ); 00213 State = TX; 00214 debug_if( DEBUG_MESSAGE, "> OnTxDone\n\r" ); 00215 } 00216 00217 void OnRxDone( uint8_t *payload, uint16_t size, int16_t rssi, int8_t snr ) 00218 { 00219 Radio.Sleep( ); 00220 BufferSize = size; 00221 memcpy( Buffer, payload, BufferSize ); 00222 RssiValue = rssi; 00223 SnrValue = snr; 00224 State = RX; 00225 debug_if( DEBUG_MESSAGE, "> OnRxDone\n\r" ); 00226 } 00227 00228 void OnTxTimeout( void ) 00229 { 00230 Radio.Sleep( ); 00231 State = TX_TIMEOUT; 00232 debug_if( DEBUG_MESSAGE, "> OnTxTimeout\n\r" ); 00233 } 00234 00235 void OnRxTimeout( void ) 00236 { 00237 Radio.Sleep( ); 00238 Buffer[BufferSize] = 0; 00239 State = RX_TIMEOUT; 00240 debug_if( DEBUG_MESSAGE, "> OnRxTimeout\n\r" ); 00241 } 00242 00243 void OnRxError( void ) 00244 { 00245 Radio.Sleep( ); 00246 State = RX_ERROR; 00247 debug_if( DEBUG_MESSAGE, "> OnRxError\n\r" ); 00248 }
Generated on Mon Jul 18 2022 01:51:30 by
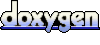