Team Riedel - display
Dependencies: LCD_fonts SPI_TFT_ILI9341 CMSIS_DSP_401_without_cm4 mbed-src SDFileSystem wavfile
audio_if.cpp
00001 /** 00002 ****************************************************************************** 00003 * @file /Src/audio_if.c 00004 * @author Central Labs 00005 * @version V1.0.0 00006 * @date 7-May-2015 00007 * @brief USB Device Audio interface file. 00008 ****************************************************************************** 00009 @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2014 STMicroelectronics</center></h2> 00012 * 00013 * Licensed under MCD-ST Liberty SW License Agreement V2, (the "License"); 00014 * You may not use this file except in compliance with the License. 00015 * You may obtain a copy of the License at: 00016 * 00017 * http://www.st.com/software_license_agreement_liberty_v2 00018 * 00019 * Redistribution and use in source and binary forms, with or without modification, 00020 * are permitted provided that the following conditions are met: 00021 * 1. Redistributions of source code must retain the above copyright notice, 00022 * this list of conditions and the following disclaimer. 00023 * 2. Redistributions in binary form must reproduce the above copyright notice, 00024 * this list of conditions and the following disclaimer in the documentation 00025 * and/or other materials provided with the distribution. 00026 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00027 * may be used to endorse or promote products derived from this software 00028 * without specific prior written permission. 00029 * 00030 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00031 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00032 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00033 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00034 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00035 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00036 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00037 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00038 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00039 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00040 * 00041 ****************************************************************************** 00042 */ 00043 00044 /* Includes ------------------------------------------------------------------*/ 00045 #include "audio_if.h" 00046 00047 //uint16_t PDM_Buffer[AUDIO_CHANNELS*AUDIO_SAMPLING_FREQUENCY/1000*64/8]; 00048 //static uint16_t PCM_Buffer[AUDIO_CHANNELS*AUDIO_SAMPLING_FREQUENCY/1000]; 00049 00050 /* Private typedef -----------------------------------------------------------*/ 00051 /* Private define ------------------------------------------------------------*/ 00052 /* Private macro -------------------------------------------------------------*/ 00053 /* Private function prototypes -----------------------------------------------*/ 00054 /* Private variables ---------------------------------------------------------*/ 00055 00056 00057 /* Private functions ---------------------------------------------------------*/ 00058 /* This table maps the audio device class setting in 1/256 dB to a 00059 * linear 0-64 scaling used in pdm_filter.c. It is computed as 00060 * 256*20*log10(index/64). */ 00061 //const int16_t vol_table[65] = 00062 //{ 0x8000, 0xDBE0, 0xE1E6, 0xE56B, 0xE7EB, 0xE9DB, 0xEB70, 0xECC7, 00063 //0xEDF0, 0xEEF6, 0xEFE0, 0xF0B4, 0xF176, 0xF228, 0xF2CD, 0xF366, 00064 //0xF3F5, 0xF47C, 0xF4FB, 0xF574, 0xF5E6, 0xF652, 0xF6BA, 0xF71C, 00065 //0xF778, 0xF7D6, 0xF82D, 0xF881, 0xF8D2, 0xF920, 0xF96B, 0xF9B4, 00066 //0xF9FB, 0xFA3F, 0xFA82, 0xFAC2, 0xFB01, 0xFB3E, 0xFB79, 0xFBB3, 00067 //0xFBEB, 0xFC22, 0xFC57, 0xFC8C, 0xFCBF, 0xFCF1, 0xFD22, 0xFD51, 00068 //0xFD80, 0xFDAE, 0xFDDB, 0xFE07, 0xFE32, 0xFE5D, 0xFE86, 0xFEAF, 00069 //0xFED7, 0xFF00, 0xFF25, 0xFF4B, 0xFF70, 0xFF95, 0xFFB9, 0xFFD0, 00070 //0x0000 }; 00071 00072 //volatile uint8_t VolumeSetting=64; 00073 00074 /** 00075 * @brief Initializes the AUDIO media low layer. 00076 * @param AudioFreq: Audio frequency used to play the audio stream. 00077 * @param BitRes: desired bit resolution 00078 * @param ChnlNbr: number of channel to be configured 00079 * @retval AUDIO_OK in case of success, AUDIO_ERROR otherwise 00080 */ 00081 int8_t Audio_Init(void) 00082 { 00083 return BSP_AUDIO_IN_Init(48000, 16, 1); 00084 00085 } 00086 00087 00088 00089 /** 00090 * @brief Start audio recording engine 00091 * @retval AUDIO_OK in case of success, AUDIO_ERROR otherwise 00092 */ 00093 int8_t Audio_Record(uint16_t * recbuff) 00094 { 00095 int8_t res = BSP_AUDIO_IN_Record(recbuff, 2048); 00096 // AudioProcess(pbuf, recbuff); 00097 return res; 00098 } 00099 00100 /** 00101 * @brief Controls AUDIO Volume. 00102 * @param vol: Volume level 00103 * @retval AUDIO_OK in case of success, AUDIO_ERROR otherwise 00104 */ 00105 //int8_t Audio_VolumeCtl(int16_t Volume) 00106 //{ 00107 // /* Call low layer volume setting function */ 00108 // int j; 00109 // 00110 // j = 0; 00111 // /* Find the setting nearest to the desired setting */ 00112 // while(j<64 && 00113 // abs(Volume-vol_table[j]) > abs(Volume-vol_table[j+1])) { 00114 // j++; 00115 // } 00116 // /* Now do the volume adjustment */ 00117 // VolumeSetting = (uint8_t)j; 00118 // return BSP_AUDIO_IN_SetVolume(VolumeSetting); 00119 // 00120 // 00121 //} 00122 00123 /** 00124 * @brief Controls AUDIO Mute. 00125 * @param cmd: Command opcode 00126 * @retval AUDIO_OK in case of success, AUDIO_ERROR otherwise 00127 */ 00128 //int8_t Audio_MuteCtl(uint8_t cmd) 00129 //{ 00130 // return AUDIO_OK; 00131 //} 00132 00133 00134 /** 00135 * @brief Stops audio acquisition 00136 * @param none 00137 * @retval AUDIO_OK in case of success, AUDIO_ERROR otherwise 00138 */ 00139 int8_t Audio_Stop(void) 00140 { 00141 return BSP_AUDIO_IN_Stop(); 00142 00143 } 00144 00145 /** 00146 * @brief Pauses audio acquisition 00147 * @param none 00148 * @retval AUDIO_OK in case of success, AUDIO_ERROR otherwise 00149 */ 00150 // 00151 //int8_t Audio_Pause(void) 00152 //{ 00153 // return 0; 00154 //} 00155 00156 00157 /** 00158 * @brief Resumes audio acquisition 00159 * @param none 00160 * @retval AUDIO_OK in case of success, AUDIO_ERROR otherwise 00161 */ 00162 //int8_t Audio_Resume(void) 00163 //{ 00164 // return AUDIO_OK; 00165 //} 00166 00167 /** 00168 * @brief Manages command from usb 00169 * @param None 00170 * @retval AUDIO_OK in case of success, AUDIO_ERROR otherwise 00171 */ 00172 // 00173 //int8_t Audio_CommandMgr(uint8_t cmd) 00174 //{ 00175 // return AUDIO_OK; 00176 //} 00177 // 00178 //void AudioProcess(uint16_t * pbuf, uint16_t * recbuff) 00179 //{ 00180 // BSP_AUDIO_IN_PDMToPCM(pbuf,recbuff); 00181 // // need to copy recbuff to final output buff (in chunks) 00182 //} 00183 00184 void PrintData(uint16_t * recbuff) 00185 { 00186 for (int i=0; i < AUDIO_CHANNELS*AUDIO_SAMPLING_FREQUENCY/1000; ++i) { 00187 if (!(i%10)) 00188 pc.printf("\r\n\t"); 00189 pc.printf("%d ", recbuff[i]); 00190 } 00191 pc.printf("\r\n\r\n"); 00192 } 00193 00194 void WriteData(uint16_t * recbuff) 00195 { 00196 00197 //Try to mount the SD card 00198 pc.printf("Mounting SD card..."); 00199 if (sd.mount() != 0) { 00200 pc.printf("failed!\r\n"); 00201 return; 00202 } 00203 pc.printf("mounted successfully!\r\n"); 00204 00205 // write event to log 00206 FILE *fp = fopen("/sd/event_log.txt", "a"); 00207 if (fp != NULL) { 00208 for (int i=0; i < AUDIO_CHANNELS*AUDIO_SAMPLING_FREQUENCY/1000; ++i) { 00209 if (!(i%10)) 00210 fprintf(fp, "\r\n"); 00211 fprintf(fp, "%d ", recbuff[i]); 00212 } 00213 fprintf(fp, "\r\n\r\n"); 00214 fprintf(fp, "Woohoo! I made a file!\r\n"); 00215 fclose(fp); 00216 PrintData(recbuff); 00217 pc.printf("success!\r\n"); 00218 } else { 00219 pc.printf("failed!\r\n"); 00220 } 00221 00222 pc.printf("creating WAV file"); 00223 static const char *target_filename = "/sd/rec-test.wav"; 00224 rec(target_filename, recbuff, 48); 00225 00226 00227 sd.unmount(); 00228 pc.printf("sd card unmounted\r\n\r\n"); 00229 00230 } 00231 00232 #define WAVFILE_ERROR_PRINT(RESULT) \ 00233 do { \ 00234 WavFileResult R = RESULT; \ 00235 if (R != WavFileResultOK) { \ 00236 char wavfile_error_print_text[BUFSIZ]; \ 00237 wavfile_result_string(R, wavfile_error_print_text, sizeof(wavfile_error_print_text)); \ 00238 printf("%s (code=%d)\r\n", wavfile_error_print_text, R); \ 00239 return 1; \ 00240 } \ 00241 } while(0) 00242 00243 int rec(const char *filename, uint16_t * recbuff, uint16_t size) 00244 { 00245 WavFileResult result; 00246 wavfile_info_t info; 00247 wavfile_data_t data; 00248 00249 WAVFILE_INFO_AUDIO_FORMAT(&info) = 1; 00250 WAVFILE_INFO_NUM_CHANNELS(&info) = 1; 00251 WAVFILE_INFO_SAMPLE_RATE(&info) = 1000; 00252 WAVFILE_INFO_BYTE_RATE(&info) = 2000; 00253 WAVFILE_INFO_BLOCK_ALIGN(&info) = 2; 00254 WAVFILE_INFO_BITS_PER_SAMPLE(&info) = 16; 00255 00256 WAVFILE *wf = wavfile_open(filename, WavFileModeWrite, &result); 00257 WAVFILE_ERROR_PRINT(result); 00258 WAVFILE_ERROR_PRINT(wavfile_write_info(wf, &info)); 00259 00260 pc.printf("[REC:%s]\r\n", filename); 00261 pc.printf("\tWAVFILE_INFO_AUDIO_FORMAT(&info) = %d\r\n", WAVFILE_INFO_AUDIO_FORMAT(&info)); 00262 pc.printf("\tWAVFILE_INFO_NUM_CHANNELS(&info) = %d\r\n", WAVFILE_INFO_NUM_CHANNELS(&info)); 00263 pc.printf("\tWAVFILE_INFO_SAMPLE_RATE(&info) = %d\r\n", WAVFILE_INFO_SAMPLE_RATE(&info)); 00264 pc.printf("\tWAVFILE_INFO_BYTE_RATE(&info) = %d\r\n", WAVFILE_INFO_BYTE_RATE(&info)); 00265 pc.printf("\tWAVFILE_INFO_BLOCK_ALIGN(&info) = %d\r\n", WAVFILE_INFO_BLOCK_ALIGN(&info)); 00266 pc.printf("\tWAVFILE_INFO_BITS_PER_SAMPLE(&info) = %d\r\n", WAVFILE_INFO_BITS_PER_SAMPLE(&info)); 00267 00268 // const int interval_us = 1000000 / WAVFILE_INFO_SAMPLE_RATE(&info); 00269 00270 int dropout = 0; 00271 // unsigned int count = 0; 00272 WAVFILE_DATA_NUM_CHANNELS(&data) = 1; 00273 // for (int i=0; i<size; ++i) { 00274 // WAVFILE_DATA_CHANNEL_DATA(&data, 0) = 0; //buffer[rp]; 00275 // WAVFILE_ERROR_PRINT(wavfile_write_data(wf, &data)); 00276 WAVFILE_ERROR_PRINT(wavfile_write_data_bulk_uint16(wf, recbuff, AUDIO_CHANNELS, size)); 00277 // } 00278 00279 pc.printf("\t-- Rec done. (dropout=%d) --\r\n", dropout); 00280 00281 WAVFILE_ERROR_PRINT(wavfile_close(wf)); 00282 return 0; 00283 } 00284 00285 00286 00287 00288 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00289
Generated on Tue Jul 12 2022 15:34:17 by
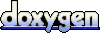