Driver for the SX1280 RF Transceiver
Dependents: SX1280PingPong RangignMaster RangingSlave MSNV2-Terminal_V1-6 ... more
sx1280.h
00001 /* 00002 ______ _ 00003 / _____) _ | | 00004 ( (____ _____ ____ _| |_ _____ ____| |__ 00005 \____ \| ___ | (_ _) ___ |/ ___) _ \ 00006 _____) ) ____| | | || |_| ____( (___| | | | 00007 (______/|_____)_|_|_| \__)_____)\____)_| |_| 00008 (C)2016 Semtech 00009 00010 Description: Driver for SX1280 devices 00011 00012 License: Revised BSD License, see LICENSE.TXT file include in the project 00013 00014 Maintainer: Miguel Luis, Gregory Cristian and Matthieu Verdy 00015 */ 00016 #ifndef __SX1280_H__ 00017 #define __SX1280_H__ 00018 00019 #include "radio.h" 00020 00021 /*! 00022 * \brief Enables/disables driver debug features 00023 */ 00024 #define SX1280_DEBUG 0 00025 00026 /*! 00027 * \brief Hardware IO IRQ callback function definition 00028 */ 00029 class SX1280; 00030 typedef void ( SX1280::*DioIrqHandler )( void ); 00031 00032 /*! 00033 * \brief IRQ triggers callback function definition 00034 */ 00035 class SX1280Hal; 00036 typedef void ( SX1280Hal::*Trigger )( void ); 00037 00038 /*! 00039 * \brief Provides the frequency of the chip running on the radio and the frequency step 00040 * 00041 * \remark These defines are used for computing the frequency divider to set the RF frequency 00042 */ 00043 #define XTAL_FREQ 52000000 00044 #define FREQ_STEP ( ( double )( XTAL_FREQ / pow( 2.0, 18.0 ) ) ) 00045 00046 /*! 00047 * \brief Compensation delay for SetAutoTx method in microseconds 00048 */ 00049 #define AUTO_TX_OFFSET 33 00050 00051 /*! 00052 * \brief The address of the register holding the firmware version MSB 00053 */ 00054 #define REG_LR_FIRMWARE_VERSION_MSB 0x0153 00055 00056 /*! 00057 * \brief The address of the register holding the first byte defining the CRC seed 00058 * 00059 * \remark Only used for packet types GFSK and Flrc 00060 */ 00061 #define REG_LR_CRCSEEDBASEADDR 0x09C8 00062 00063 /*! 00064 * \brief The address of the register holding the first byte defining the CRC polynomial 00065 * 00066 * \remark Only used for packet types GFSK and Flrc 00067 */ 00068 #define REG_LR_CRCPOLYBASEADDR 0x09C6 00069 00070 /*! 00071 * \brief The address of the register holding the first byte defining the whitening seed 00072 * 00073 * \remark Only used for packet types GFSK, FLRC and BLE 00074 */ 00075 #define REG_LR_WHITSEEDBASEADDR 0x09C5 00076 00077 /*! 00078 * \brief The address of the register holding the ranging id check length 00079 * 00080 * \remark Only used for packet type Ranging 00081 */ 00082 #define REG_LR_RANGINGIDCHECKLENGTH 0x0931 00083 00084 /*! 00085 * \brief The address of the register holding the device ranging id 00086 * 00087 * \remark Only used for packet type Ranging 00088 */ 00089 #define REG_LR_DEVICERANGINGADDR 0x0916 00090 00091 /*! 00092 * \brief The address of the register holding the device ranging id 00093 * 00094 * \remark Only used for packet type Ranging 00095 */ 00096 #define REG_LR_REQUESTRANGINGADDR 0x0912 00097 00098 /*! 00099 * \brief The address of the register holding ranging results configuration 00100 * and the corresponding mask 00101 * 00102 * \remark Only used for packet type Ranging 00103 */ 00104 #define REG_LR_RANGINGRESULTCONFIG 0x0924 00105 #define MASK_RANGINGMUXSEL 0xCF 00106 00107 /*! 00108 * \brief The address of the register holding the first byte of ranging results 00109 * Only used for packet type Ranging 00110 */ 00111 #define REG_LR_RANGINGRESULTBASEADDR 0x0961 00112 00113 /*! 00114 * \brief The address of the register allowing to read ranging results 00115 * 00116 * \remark Only used for packet type Ranging 00117 */ 00118 #define REG_LR_RANGINGRESULTSFREEZE 0x097F 00119 00120 /*! 00121 * \brief The address of the register holding the first byte of ranging calibration 00122 * 00123 * \remark Only used for packet type Ranging 00124 */ 00125 #define REG_LR_RANGINGRERXTXDELAYCAL 0x092C 00126 00127 /*! 00128 *\brief The address of the register holding the ranging filter window size 00129 * 00130 * \remark Only used for packet type Ranging 00131 */ 00132 #define REG_LR_RANGINGFILTERWINDOWSIZE 0x091E 00133 00134 /*! 00135 *\brief The address of the register to reset for clearing ranging filter 00136 * 00137 * \remark Only used for packet type Ranging 00138 */ 00139 #define REG_LR_RANGINGRESULTCLEARREG 0x0923 00140 00141 00142 #define REG_RANGING_RSSI 0x0964 00143 00144 /*! 00145 * \brief The default number of samples considered in built-in ranging filter 00146 */ 00147 #define DEFAULT_RANGING_FILTER_SIZE 127 00148 00149 /*! 00150 * \brief The address of the register holding LORA packet parameters 00151 */ 00152 #define REG_LR_PACKETPARAMS 0x903 00153 00154 /*! 00155 * \brief The address of the register holding payload length 00156 * 00157 * \remark Do NOT try to read it directly. Use GetRxBuffer( ) instead. 00158 */ 00159 #define REG_LR_PAYLOADLENGTH 0x901 00160 00161 /*! 00162 * \brief The addresses of the registers holding SyncWords values 00163 * 00164 * \remark The addresses depends on the Packet Type in use, and not all 00165 * SyncWords are available for every Packet Type 00166 */ 00167 #define REG_LR_SYNCWORDBASEADDRESS1 0x09CE 00168 #define REG_LR_SYNCWORDBASEADDRESS2 0x09D3 00169 #define REG_LR_SYNCWORDBASEADDRESS3 0x09D8 00170 00171 /*! 00172 * \brief The MSB address and mask used to read the estimated frequency 00173 * error 00174 */ 00175 #define REG_LR_ESTIMATED_FREQUENCY_ERROR_MSB 0x0954 00176 #define REG_LR_ESTIMATED_FREQUENCY_ERROR_MASK 0x0FFFFF 00177 00178 /*! 00179 * \brief Defines how many bit errors are tolerated in sync word detection 00180 */ 00181 #define REG_LR_SYNCWORDTOLERANCE 0x09CD 00182 00183 /*! 00184 * \brief Register and mask for GFSK and BLE preamble length forcing 00185 */ 00186 #define REG_LR_PREAMBLELENGTH 0x09C1 00187 #define MASK_FORCE_PREAMBLELENGTH 0x8F 00188 00189 /*! 00190 * \brief Register for MSB Access Address (BLE) 00191 */ 00192 #define REG_LR_BLE_ACCESS_ADDRESS 0x09CF 00193 #define BLE_ADVERTIZER_ACCESS_ADDRESS 0x8E89BED6 00194 00195 /*! 00196 * \brief Select high sensitivity versus power consumption 00197 */ 00198 #define REG_LNA_REGIME 0x0891 00199 #define MASK_LNA_REGIME 0xC0 00200 00201 /* 00202 * \brief Register and mask controling the enabling of manual gain control 00203 */ 00204 #define REG_ENABLE_MANUAL_GAIN_CONTROL 0x089F 00205 #define MASK_MANUAL_GAIN_CONTROL 0x80 00206 00207 /*! 00208 * \brief Register and mask controling the demodulation detection 00209 */ 00210 #define REG_DEMOD_DETECTION 0x0895 00211 #define MASK_DEMOD_DETECTION 0xFE 00212 00213 /*! 00214 * Register and mask to set the manual gain parameter 00215 */ 00216 #define REG_MANUAL_GAIN_VALUE 0x089E 00217 #define MASK_MANUAL_GAIN_VALUE 0xF0 00218 00219 /*! 00220 * \brief Represents the states of the radio 00221 */ 00222 typedef enum 00223 { 00224 RF_IDLE = 0x00, //!< The radio is idle 00225 RF_RX_RUNNING, //!< The radio is in reception state 00226 RF_TX_RUNNING, //!< The radio is in transmission state 00227 RF_CAD, //!< The radio is doing channel activity detection 00228 }RadioStates_t; 00229 00230 /*! 00231 * \brief Represents the operating mode the radio is actually running 00232 */ 00233 typedef enum 00234 { 00235 MODE_SLEEP = 0x00, //! The radio is in sleep mode 00236 MODE_CALIBRATION, //! The radio is in calibration mode 00237 MODE_STDBY_RC, //! The radio is in standby mode with RC oscillator 00238 MODE_STDBY_XOSC, //! The radio is in standby mode with XOSC oscillator 00239 MODE_FS, //! The radio is in frequency synthesis mode 00240 MODE_RX, //! The radio is in receive mode 00241 MODE_TX, //! The radio is in transmit mode 00242 MODE_CAD //! The radio is in channel activity detection mode 00243 }RadioOperatingModes_t; 00244 00245 /*! 00246 * \brief Declares the oscillator in use while in standby mode 00247 * 00248 * Using the STDBY_RC standby mode allow to reduce the energy consumption 00249 * STDBY_XOSC should be used for time critical applications 00250 */ 00251 typedef enum 00252 { 00253 STDBY_RC = 0x00, 00254 STDBY_XOSC = 0x01, 00255 }RadioStandbyModes_t; 00256 00257 /*! 00258 * \brief Declares the power regulation used to power the device 00259 * 00260 * This command allows the user to specify if DC-DC or LDO is used for power regulation. 00261 * Using only LDO implies that the Rx or Tx current is doubled 00262 */ 00263 typedef enum 00264 { 00265 USE_LDO = 0x00, //! Use LDO (default value) 00266 USE_DCDC = 0x01, //! Use DCDC 00267 }RadioRegulatorModes_t; 00268 00269 /*! 00270 * \brief Represents the possible packet type (i.e. modem) used 00271 */ 00272 typedef enum 00273 { 00274 PACKET_TYPE_GFSK = 0x00, 00275 PACKET_TYPE_LORA, 00276 PACKET_TYPE_RANGING, 00277 PACKET_TYPE_FLRC, 00278 PACKET_TYPE_BLE, 00279 PACKET_TYPE_NONE = 0x0F, 00280 }RadioPacketTypes_t; 00281 00282 /*! 00283 * \brief Represents the ramping time for power amplifier 00284 */ 00285 typedef enum 00286 { 00287 RADIO_RAMP_02_US = 0x00, 00288 RADIO_RAMP_04_US = 0x20, 00289 RADIO_RAMP_06_US = 0x40, 00290 RADIO_RAMP_08_US = 0x60, 00291 RADIO_RAMP_10_US = 0x80, 00292 RADIO_RAMP_12_US = 0xA0, 00293 RADIO_RAMP_16_US = 0xC0, 00294 RADIO_RAMP_20_US = 0xE0, 00295 }RadioRampTimes_t; 00296 00297 /*! 00298 * \brief Represents the number of symbols to be used for channel activity detection operation 00299 */ 00300 typedef enum 00301 { 00302 LORA_CAD_01_SYMBOL = 0x00, 00303 LORA_CAD_02_SYMBOLS = 0x20, 00304 LORA_CAD_04_SYMBOLS = 0x40, 00305 LORA_CAD_08_SYMBOLS = 0x60, 00306 LORA_CAD_16_SYMBOLS = 0x80, 00307 }RadioLoRaCadSymbols_t; 00308 00309 /*! 00310 * \brief Represents the possible combinations of bitrate and bandwidth for 00311 * GFSK and BLE packet types 00312 * 00313 * The bitrate is expressed in Mb/s and the bandwidth in MHz 00314 */ 00315 typedef enum 00316 { 00317 GFSK_BLE_BR_2_000_BW_2_4 = 0x04, 00318 GFSK_BLE_BR_1_600_BW_2_4 = 0x28, 00319 GFSK_BLE_BR_1_000_BW_2_4 = 0x4C, 00320 GFSK_BLE_BR_1_000_BW_1_2 = 0x45, 00321 GFSK_BLE_BR_0_800_BW_2_4 = 0x70, 00322 GFSK_BLE_BR_0_800_BW_1_2 = 0x69, 00323 GFSK_BLE_BR_0_500_BW_1_2 = 0x8D, 00324 GFSK_BLE_BR_0_500_BW_0_6 = 0x86, 00325 GFSK_BLE_BR_0_400_BW_1_2 = 0xB1, 00326 GFSK_BLE_BR_0_400_BW_0_6 = 0xAA, 00327 GFSK_BLE_BR_0_250_BW_0_6 = 0xCE, 00328 GFSK_BLE_BR_0_250_BW_0_3 = 0xC7, 00329 GFSK_BLE_BR_0_125_BW_0_3 = 0xEF, 00330 }RadioGfskBleBitrates_t; 00331 00332 /*! 00333 * \brief Represents the modulation index used in GFSK and BLE packet 00334 * types 00335 */ 00336 typedef enum 00337 { 00338 GFSK_BLE_MOD_IND_0_35 = 0, 00339 GFSK_BLE_MOD_IND_0_50 = 1, 00340 GFSK_BLE_MOD_IND_0_75 = 2, 00341 GFSK_BLE_MOD_IND_1_00 = 3, 00342 GFSK_BLE_MOD_IND_1_25 = 4, 00343 GFSK_BLE_MOD_IND_1_50 = 5, 00344 GFSK_BLE_MOD_IND_1_75 = 6, 00345 GFSK_BLE_MOD_IND_2_00 = 7, 00346 GFSK_BLE_MOD_IND_2_25 = 8, 00347 GFSK_BLE_MOD_IND_2_50 = 9, 00348 GFSK_BLE_MOD_IND_2_75 = 10, 00349 GFSK_BLE_MOD_IND_3_00 = 11, 00350 GFSK_BLE_MOD_IND_3_25 = 12, 00351 GFSK_BLE_MOD_IND_3_50 = 13, 00352 GFSK_BLE_MOD_IND_3_75 = 14, 00353 GFSK_BLE_MOD_IND_4_00 = 15, 00354 }RadioGfskBleModIndexes_t; 00355 00356 /*! 00357 * \brief Represents the possible combination of bitrate and bandwidth for FLRC 00358 * packet type 00359 * 00360 * The bitrate is in Mb/s and the bitrate in MHz 00361 */ 00362 typedef enum 00363 { 00364 FLRC_BR_1_300_BW_1_2 = 0x45, 00365 FLRC_BR_1_040_BW_1_2 = 0x69, 00366 FLRC_BR_0_650_BW_0_6 = 0x86, 00367 FLRC_BR_0_520_BW_0_6 = 0xAA, 00368 FLRC_BR_0_325_BW_0_3 = 0xC7, 00369 FLRC_BR_0_260_BW_0_3 = 0xEB, 00370 }RadioFlrcBitrates_t; 00371 00372 /*! 00373 * \brief Represents the possible values for coding rate parameter in FLRC 00374 * packet type 00375 */ 00376 typedef enum 00377 { 00378 FLRC_CR_1_2 = 0x00, 00379 FLRC_CR_3_4 = 0x02, 00380 FLRC_CR_1_0 = 0x04, 00381 }RadioFlrcCodingRates_t; 00382 00383 /*! 00384 * \brief Represents the modulation shaping parameter for GFSK, FLRC and BLE 00385 * packet types 00386 */ 00387 typedef enum 00388 { 00389 RADIO_MOD_SHAPING_BT_OFF = 0x00, //! No filtering 00390 RADIO_MOD_SHAPING_BT_1_0 = 0x10, 00391 RADIO_MOD_SHAPING_BT_0_5 = 0x20, 00392 }RadioModShapings_t; 00393 00394 /*! 00395 * \brief Represents the possible spreading factor values in LORA packet types 00396 */ 00397 typedef enum 00398 { 00399 LORA_SF5 = 0x50, 00400 LORA_SF6 = 0x60, 00401 LORA_SF7 = 0x70, 00402 LORA_SF8 = 0x80, 00403 LORA_SF9 = 0x90, 00404 LORA_SF10 = 0xA0, 00405 LORA_SF11 = 0xB0, 00406 LORA_SF12 = 0xC0, 00407 }RadioLoRaSpreadingFactors_t; 00408 00409 /*! 00410 * \brief Represents the bandwidth values for LORA packet type 00411 */ 00412 typedef enum 00413 { 00414 LORA_BW_0200 = 0x34, 00415 LORA_BW_0400 = 0x26, 00416 LORA_BW_0800 = 0x18, 00417 LORA_BW_1600 = 0x0A, 00418 }RadioLoRaBandwidths_t; 00419 00420 /*! 00421 * \brief Represents the coding rate values for LORA packet type 00422 */ 00423 typedef enum 00424 { 00425 LORA_CR_4_5 = 0x01, 00426 LORA_CR_4_6 = 0x02, 00427 LORA_CR_4_7 = 0x03, 00428 LORA_CR_4_8 = 0x04, 00429 LORA_CR_LI_4_5 = 0x05, 00430 LORA_CR_LI_4_6 = 0x06, 00431 LORA_CR_LI_4_7 = 0x07, 00432 }RadioLoRaCodingRates_t; 00433 00434 /*! 00435 * \brief Represents the preamble length values for GFSK and FLRC packet 00436 * types 00437 */ 00438 typedef enum 00439 { 00440 PREAMBLE_LENGTH_04_BITS = 0x00, //!< Preamble length: 04 bits 00441 PREAMBLE_LENGTH_08_BITS = 0x10, //!< Preamble length: 08 bits 00442 PREAMBLE_LENGTH_12_BITS = 0x20, //!< Preamble length: 12 bits 00443 PREAMBLE_LENGTH_16_BITS = 0x30, //!< Preamble length: 16 bits 00444 PREAMBLE_LENGTH_20_BITS = 0x40, //!< Preamble length: 20 bits 00445 PREAMBLE_LENGTH_24_BITS = 0x50, //!< Preamble length: 24 bits 00446 PREAMBLE_LENGTH_28_BITS = 0x60, //!< Preamble length: 28 bits 00447 PREAMBLE_LENGTH_32_BITS = 0x70, //!< Preamble length: 32 bits 00448 }RadioPreambleLengths_t; 00449 00450 /*! 00451 * \brief Represents the SyncWord length for FLRC packet type 00452 */ 00453 typedef enum 00454 { 00455 FLRC_NO_SYNCWORD = 0x00, 00456 FLRC_SYNCWORD_LENGTH_4_BYTE = 0x04, 00457 }RadioFlrcSyncWordLengths_t; 00458 00459 /*! 00460 * \brief The length of sync words for GFSK packet type 00461 */ 00462 typedef enum 00463 { 00464 GFSK_SYNCWORD_LENGTH_1_BYTE = 0x00, //!< Sync word length: 1 byte 00465 GFSK_SYNCWORD_LENGTH_2_BYTE = 0x02, //!< Sync word length: 2 bytes 00466 GFSK_SYNCWORD_LENGTH_3_BYTE = 0x04, //!< Sync word length: 3 bytes 00467 GFSK_SYNCWORD_LENGTH_4_BYTE = 0x06, //!< Sync word length: 4 bytes 00468 GFSK_SYNCWORD_LENGTH_5_BYTE = 0x08, //!< Sync word length: 5 bytes 00469 }RadioSyncWordLengths_t; 00470 00471 /*! 00472 * \brief Represents the possible combinations of SyncWord correlators 00473 * activated for GFSK and FLRC packet types 00474 */ 00475 typedef enum 00476 { 00477 RADIO_RX_MATCH_SYNCWORD_OFF = 0x00, //!< No correlator turned on, i.e. do not search for SyncWord 00478 RADIO_RX_MATCH_SYNCWORD_1 = 0x10, 00479 RADIO_RX_MATCH_SYNCWORD_2 = 0x20, 00480 RADIO_RX_MATCH_SYNCWORD_1_2 = 0x30, 00481 RADIO_RX_MATCH_SYNCWORD_3 = 0x40, 00482 RADIO_RX_MATCH_SYNCWORD_1_3 = 0x50, 00483 RADIO_RX_MATCH_SYNCWORD_2_3 = 0x60, 00484 RADIO_RX_MATCH_SYNCWORD_1_2_3 = 0x70, 00485 }RadioSyncWordRxMatchs_t; 00486 00487 /*! 00488 * \brief Radio packet length mode for GFSK and FLRC packet types 00489 */ 00490 typedef enum 00491 { 00492 RADIO_PACKET_FIXED_LENGTH = 0x00, //!< The packet is known on both sides, no header included in the packet 00493 RADIO_PACKET_VARIABLE_LENGTH = 0x20, //!< The packet is on variable size, header included 00494 }RadioPacketLengthModes_t; 00495 00496 /*! 00497 * \brief Represents the CRC length for GFSK and FLRC packet types 00498 * 00499 * \warning Not all configurations are available for both GFSK and FLRC 00500 * packet type. Refer to the datasheet for possible configuration. 00501 */ 00502 typedef enum 00503 { 00504 RADIO_CRC_OFF = 0x00, //!< No CRC in use 00505 RADIO_CRC_1_BYTES = 0x10, 00506 RADIO_CRC_2_BYTES = 0x20, 00507 RADIO_CRC_3_BYTES = 0x30, 00508 }RadioCrcTypes_t; 00509 00510 /*! 00511 * \brief Radio whitening mode activated or deactivated for GFSK, FLRC and 00512 * BLE packet types 00513 */ 00514 typedef enum 00515 { 00516 RADIO_WHITENING_ON = 0x00, 00517 RADIO_WHITENING_OFF = 0x08, 00518 }RadioWhiteningModes_t; 00519 00520 /*! 00521 * \brief Holds the packet length mode of a LORA packet type 00522 */ 00523 typedef enum 00524 { 00525 LORA_PACKET_VARIABLE_LENGTH = 0x00, //!< The packet is on variable size, header included 00526 LORA_PACKET_FIXED_LENGTH = 0x80, //!< The packet is known on both sides, no header included in the packet 00527 LORA_PACKET_EXPLICIT = LORA_PACKET_VARIABLE_LENGTH, 00528 LORA_PACKET_IMPLICIT = LORA_PACKET_FIXED_LENGTH, 00529 }RadioLoRaPacketLengthsModes_t; 00530 00531 /*! 00532 * \brief Represents the CRC mode for LORA packet type 00533 */ 00534 typedef enum 00535 { 00536 LORA_CRC_ON = 0x20, //!< CRC activated 00537 LORA_CRC_OFF = 0x00, //!< CRC not used 00538 }RadioLoRaCrcModes_t; 00539 00540 /*! 00541 * \brief Represents the IQ mode for LORA packet type 00542 */ 00543 typedef enum 00544 { 00545 LORA_IQ_NORMAL = 0x40, 00546 LORA_IQ_INVERTED = 0x00, 00547 }RadioLoRaIQModes_t; 00548 00549 /*! 00550 * \brief Represents the length of the ID to check in ranging operation 00551 */ 00552 typedef enum 00553 { 00554 RANGING_IDCHECK_LENGTH_08_BITS = 0x00, 00555 RANGING_IDCHECK_LENGTH_16_BITS, 00556 RANGING_IDCHECK_LENGTH_24_BITS, 00557 RANGING_IDCHECK_LENGTH_32_BITS, 00558 }RadioRangingIdCheckLengths_t; 00559 00560 /*! 00561 * \brief Represents the result type to be used in ranging operation 00562 */ 00563 typedef enum 00564 { 00565 RANGING_RESULT_RAW = 0x00, 00566 RANGING_RESULT_AVERAGED = 0x01, 00567 RANGING_RESULT_DEBIASED = 0x02, 00568 RANGING_RESULT_FILTERED = 0x03, 00569 }RadioRangingResultTypes_t; 00570 00571 /*! 00572 * \brief Represents the connection state for BLE packet type 00573 */ 00574 typedef enum 00575 { 00576 BLE_PAYLOAD_LENGTH_MAX_31_BYTES = 0x00, 00577 BLE_PAYLOAD_LENGTH_MAX_37_BYTES = 0x20, 00578 BLE_TX_TEST_MODE = 0x40, 00579 BLE_PAYLOAD_LENGTH_MAX_255_BYTES = 0x80, 00580 }RadioBleConnectionStates_t; 00581 00582 /*! 00583 * \brief Represents the CRC field length for BLE packet type 00584 */ 00585 typedef enum 00586 { 00587 BLE_CRC_OFF = 0x00, 00588 BLE_CRC_3B = 0x10, 00589 }RadioBleCrcTypes_t; 00590 00591 /*! 00592 * \brief Represents the specific packets to use in BLE packet type 00593 */ 00594 typedef enum 00595 { 00596 BLE_PRBS_9 = 0x00, //!< Pseudo Random Binary Sequence based on 9th degree polynomial 00597 BLE_PRBS_15 = 0x0C, //!< Pseudo Random Binary Sequence based on 15th degree polynomial 00598 BLE_EYELONG_1_0 = 0x04, //!< Repeated '11110000' sequence 00599 BLE_EYELONG_0_1 = 0x18, //!< Repeated '00001111' sequence 00600 BLE_EYESHORT_1_0 = 0x08, //!< Repeated '10101010' sequence 00601 BLE_EYESHORT_0_1 = 0x1C, //!< Repeated '01010101' sequence 00602 BLE_ALL_1 = 0x10, //!< Repeated '11111111' sequence 00603 BLE_ALL_0 = 0x14, //!< Repeated '00000000' sequence 00604 }RadioBleTestPayloads_t; 00605 00606 /*! 00607 * \brief Represents the interruption masks available for the radio 00608 * 00609 * \remark Note that not all these interruptions are available for all packet types 00610 */ 00611 typedef enum 00612 { 00613 IRQ_RADIO_NONE = 0x0000, 00614 IRQ_TX_DONE = 0x0001, 00615 IRQ_RX_DONE = 0x0002, 00616 IRQ_SYNCWORD_VALID = 0x0004, 00617 IRQ_SYNCWORD_ERROR = 0x0008, 00618 IRQ_HEADER_VALID = 0x0010, 00619 IRQ_HEADER_ERROR = 0x0020, 00620 IRQ_CRC_ERROR = 0x0040, 00621 IRQ_RANGING_SLAVE_RESPONSE_DONE = 0x0080, 00622 IRQ_RANGING_SLAVE_REQUEST_DISCARDED = 0x0100, 00623 IRQ_RANGING_MASTER_RESULT_VALID = 0x0200, 00624 IRQ_RANGING_MASTER_TIMEOUT = 0x0400, 00625 IRQ_RANGING_SLAVE_REQUEST_VALID = 0x0800, 00626 IRQ_CAD_DONE = 0x1000, 00627 IRQ_CAD_DETECTED = 0x2000, 00628 IRQ_RX_TX_TIMEOUT = 0x4000, 00629 IRQ_PREAMBLE_DETECTED = 0x8000, 00630 IRQ_RADIO_ALL = 0xFFFF, 00631 }RadioIrqMasks_t; 00632 00633 /*! 00634 * \brief Represents the digital input/output of the radio 00635 */ 00636 typedef enum 00637 { 00638 RADIO_DIO1 = 0x02, 00639 RADIO_DIO2 = 0x04, 00640 RADIO_DIO3 = 0x08, 00641 }RadioDios_t; 00642 00643 /*! 00644 * \brief Represents the tick size available for Rx/Tx timeout operations 00645 */ 00646 typedef enum 00647 { 00648 RADIO_TICK_SIZE_0015_US = 0x00, 00649 RADIO_TICK_SIZE_0062_US = 0x01, 00650 RADIO_TICK_SIZE_1000_US = 0x02, 00651 RADIO_TICK_SIZE_4000_US = 0x03, 00652 }RadioTickSizes_t; 00653 00654 /*! 00655 * \brief Represents the role of the radio during ranging operations 00656 */ 00657 typedef enum 00658 { 00659 RADIO_RANGING_ROLE_SLAVE = 0x00, 00660 RADIO_RANGING_ROLE_MASTER = 0x01, 00661 }RadioRangingRoles_t; 00662 00663 /*! 00664 * \brief Represents the possible Mask settings for sensitivity selection 00665 */ 00666 typedef enum 00667 { 00668 LNA_LOW_POWER_MODE, 00669 LNA_HIGH_SENSITIVITY_MODE, 00670 }RadioLnaSettings_t; 00671 00672 /*! 00673 * \brief Represents an amount of time measurable by the radio clock 00674 * 00675 * @code 00676 * Time = PeriodBase * PeriodBaseCount 00677 * Example: 00678 * PeriodBase = RADIO_TICK_SIZE_4000_US( 4 ms ) 00679 * PeriodBaseCount = 1000 00680 * Time = 4e-3 * 1000 = 4 seconds 00681 * @endcode 00682 */ 00683 typedef struct TickTime_s 00684 { 00685 RadioTickSizes_t PeriodBase; //!< The base time of ticktime 00686 /*! 00687 * \brief The number of periodBase for ticktime 00688 * Special values are: 00689 * - 0x0000 for single mode 00690 * - 0xFFFF for continuous mode 00691 */ 00692 uint16_t PeriodBaseCount; 00693 }TickTime_t; 00694 00695 /*! 00696 * \brief RX_TX_CONTINUOUS and RX_TX_SINGLE are two particular values for TickTime. 00697 * The former keep the radio in Rx or Tx mode, even after successfull reception 00698 * or transmission. It should never generate Timeout interrupt. 00699 * The later let the radio enought time to make one reception or transmission. 00700 * No Timeout interrupt is generated, and the radio fall in StandBy mode after 00701 * reception or transmission. 00702 */ 00703 #define RX_TX_CONTINUOUS ( TickTime_t ){ RADIO_TICK_SIZE_0015_US, 0xFFFF } 00704 #define RX_TX_SINGLE ( TickTime_t ){ RADIO_TICK_SIZE_0015_US, 0 } 00705 00706 /*! 00707 * \brief The type describing the modulation parameters for every packet types 00708 */ 00709 typedef struct 00710 { 00711 RadioPacketTypes_t PacketType; //!< Packet to which the modulation parameters are referring to. 00712 struct 00713 { 00714 /*! 00715 * \brief Holds the GFSK modulation parameters 00716 * 00717 * In GFSK modulation, the bit-rate and bandwidth are linked together. In this structure, its values are set using the same token. 00718 */ 00719 struct 00720 { 00721 RadioGfskBleBitrates_t BitrateBandwidth; //!< The bandwidth and bit-rate values for BLE and GFSK modulations 00722 RadioGfskBleModIndexes_t ModulationIndex; //!< The coding rate for BLE and GFSK modulations 00723 RadioModShapings_t ModulationShaping; //!< The modulation shaping for BLE and GFSK modulations 00724 }Gfsk; 00725 /*! 00726 * \brief Holds the LORA modulation parameters 00727 * 00728 * LORA modulation is defined by Spreading Factor (SF), Bandwidth and Coding Rate 00729 */ 00730 struct 00731 { 00732 RadioLoRaSpreadingFactors_t SpreadingFactor; //!< Spreading Factor for the LORA modulation 00733 RadioLoRaBandwidths_t Bandwidth; //!< Bandwidth for the LORA modulation 00734 RadioLoRaCodingRates_t CodingRate; //!< Coding rate for the LORA modulation 00735 }LoRa; 00736 /*! 00737 * \brief Holds the FLRC modulation parameters 00738 * 00739 * In FLRC modulation, the bit-rate and bandwidth are linked together. In this structure, its values are set using the same token. 00740 */ 00741 struct 00742 { 00743 RadioFlrcBitrates_t BitrateBandwidth; //!< The bandwidth and bit-rate values for FLRC modulation 00744 RadioFlrcCodingRates_t CodingRate; //!< The coding rate for FLRC modulation 00745 RadioModShapings_t ModulationShaping; //!< The modulation shaping for FLRC modulation 00746 }Flrc; 00747 /*! 00748 * \brief Holds the BLE modulation parameters 00749 * 00750 * In BLE modulation, the bit-rate and bandwidth are linked together. In this structure, its values are set using the same token. 00751 */ 00752 struct 00753 { 00754 RadioGfskBleBitrates_t BitrateBandwidth; //!< The bandwidth and bit-rate values for BLE and GFSK modulations 00755 RadioGfskBleModIndexes_t ModulationIndex; //!< The coding rate for BLE and GFSK modulations 00756 RadioModShapings_t ModulationShaping; //!< The modulation shaping for BLE and GFSK modulations 00757 }Ble; 00758 }Params; //!< Holds the modulation parameters structure 00759 }ModulationParams_t; 00760 00761 /*! 00762 * \brief The type describing the packet parameters for every packet types 00763 */ 00764 typedef struct 00765 { 00766 RadioPacketTypes_t PacketType; //!< Packet to which the packet parameters are referring to. 00767 struct 00768 { 00769 /*! 00770 * \brief Holds the GFSK packet parameters 00771 */ 00772 struct 00773 { 00774 RadioPreambleLengths_t PreambleLength; //!< The preamble length for GFSK packet type 00775 RadioSyncWordLengths_t SyncWordLength; //!< The synchronization word length for GFSK packet type 00776 RadioSyncWordRxMatchs_t SyncWordMatch; //!< The synchronization correlator to use to check synchronization word 00777 RadioPacketLengthModes_t HeaderType; //!< If the header is explicit, it will be transmitted in the GFSK packet. If the header is implicit, it will not be transmitted 00778 uint8_t PayloadLength; //!< Size of the payload in the GFSK packet 00779 RadioCrcTypes_t CrcLength; //!< Size of the CRC block in the GFSK packet 00780 RadioWhiteningModes_t Whitening; //!< Usage of whitening on payload and CRC blocks plus header block if header type is variable 00781 }Gfsk; 00782 /*! 00783 * \brief Holds the LORA packet parameters 00784 */ 00785 struct 00786 { 00787 uint8_t PreambleLength; //!< The preamble length is the number of LORA symbols in the preamble. To set it, use the following formula @code Number of symbols = PreambleLength[3:0] * ( 2^PreambleLength[7:4] ) @endcode 00788 RadioLoRaPacketLengthsModes_t HeaderType; //!< If the header is explicit, it will be transmitted in the LORA packet. If the header is implicit, it will not be transmitted 00789 uint8_t PayloadLength; //!< Size of the payload in the LORA packet 00790 RadioLoRaCrcModes_t Crc; //!< Size of CRC block in LORA packet 00791 RadioLoRaIQModes_t InvertIQ; //!< Allows to swap IQ for LORA packet 00792 }LoRa; 00793 /*! 00794 * \brief Holds the FLRC packet parameters 00795 */ 00796 struct 00797 { 00798 RadioPreambleLengths_t PreambleLength; //!< The preamble length for FLRC packet type 00799 RadioFlrcSyncWordLengths_t SyncWordLength; //!< The synchronization word length for FLRC packet type 00800 RadioSyncWordRxMatchs_t SyncWordMatch; //!< The synchronization correlator to use to check synchronization word 00801 RadioPacketLengthModes_t HeaderType; //!< If the header is explicit, it will be transmitted in the FLRC packet. If the header is implicit, it will not be transmitted. 00802 uint8_t PayloadLength; //!< Size of the payload in the FLRC packet 00803 RadioCrcTypes_t CrcLength; //!< Size of the CRC block in the FLRC packet 00804 RadioWhiteningModes_t Whitening; //!< Usage of whitening on payload and CRC blocks plus header block if header type is variable 00805 }Flrc; 00806 /*! 00807 * \brief Holds the BLE packet parameters 00808 */ 00809 struct 00810 { 00811 RadioBleConnectionStates_t ConnectionState; //!< The BLE state 00812 RadioBleCrcTypes_t CrcLength; //!< Size of the CRC block in the BLE packet 00813 RadioBleTestPayloads_t BleTestPayload; //!< Special BLE payload for test purpose 00814 RadioWhiteningModes_t Whitening; //!< Usage of whitening on PDU and CRC blocks of BLE packet 00815 }Ble; 00816 }Params; //!< Holds the packet parameters structure 00817 }PacketParams_t; 00818 00819 /*! 00820 * \brief Represents the packet status for every packet type 00821 */ 00822 typedef struct 00823 { 00824 RadioPacketTypes_t packetType; //!< Packet to which the packet status are referring to. 00825 union 00826 { 00827 struct 00828 { 00829 int8_t RssiSync; //!< The RSSI measured on last packet 00830 struct 00831 { 00832 bool SyncError :1; //!< SyncWord error on last packet 00833 bool LengthError :1; //!< Length error on last packet 00834 bool CrcError :1; //!< CRC error on last packet 00835 bool AbortError :1; //!< Abort error on last packet 00836 bool HeaderReceived :1; //!< Header received on last packet 00837 bool PacketReceived :1; //!< Packet received 00838 bool PacketControlerBusy :1; //!< Packet controller busy 00839 }ErrorStatus; //!< The error status Byte 00840 struct 00841 { 00842 bool RxNoAck :1; //!< No acknowledgment received for Rx with variable length packets 00843 bool PacketSent :1; //!< Packet sent, only relevant in Tx mode 00844 }TxRxStatus; //!< The Tx/Rx status Byte 00845 uint8_t SyncAddrStatus :3; //!< The id of the correlator who found the packet 00846 }Gfsk; 00847 struct 00848 { 00849 int8_t RssiPkt; //!< The RSSI of the last packet 00850 int8_t SnrPkt; //!< The SNR of the last packet 00851 }LoRa; 00852 struct 00853 { 00854 int8_t RssiSync; //!< The RSSI of the last packet 00855 struct 00856 { 00857 bool SyncError :1; //!< SyncWord error on last packet 00858 bool LengthError :1; //!< Length error on last packet 00859 bool CrcError :1; //!< CRC error on last packet 00860 bool AbortError :1; //!< Abort error on last packet 00861 bool HeaderReceived :1; //!< Header received on last packet 00862 bool PacketReceived :1; //!< Packet received 00863 bool PacketControlerBusy :1; //!< Packet controller busy 00864 }ErrorStatus; //!< The error status Byte 00865 struct 00866 { 00867 uint8_t RxPid :2; //!< PID of the Rx 00868 bool RxNoAck :1; //!< No acknowledgment received for Rx with variable length packets 00869 bool RxPidErr :1; //!< Received PID error 00870 bool PacketSent :1; //!< Packet sent, only relevant in Tx mode 00871 }TxRxStatus; //!< The Tx/Rx status Byte 00872 uint8_t SyncAddrStatus :3; //!< The id of the correlator who found the packet 00873 }Flrc; 00874 struct 00875 { 00876 int8_t RssiSync; //!< The RSSI of the last packet 00877 struct 00878 { 00879 bool SyncError :1; //!< SyncWord error on last packet 00880 bool LengthError :1; //!< Length error on last packet 00881 bool CrcError :1; //!< CRC error on last packet 00882 bool AbortError :1; //!< Abort error on last packet 00883 bool HeaderReceived :1; //!< Header received on last packet 00884 bool PacketReceived :1; //!< Packet received 00885 bool PacketControlerBusy :1; //!< Packet controller busy 00886 }ErrorStatus; //!< The error status Byte 00887 struct 00888 { 00889 bool PacketSent :1; //!< Packet sent, only relevant in Tx mode 00890 }TxRxStatus; //!< The Tx/Rx status Byte 00891 uint8_t SyncAddrStatus :3; //!< The id of the correlator who found the packet 00892 }Ble; 00893 }; 00894 }PacketStatus_t; 00895 00896 /*! 00897 * \brief Represents the Rx internal counters values when GFSK or LORA packet type is used 00898 */ 00899 typedef struct 00900 { 00901 RadioPacketTypes_t packetType; //!< Packet to which the packet status are referring to. 00902 union 00903 { 00904 struct 00905 { 00906 uint16_t PacketReceived; //!< Number of received packets 00907 uint16_t CrcError; //!< Number of CRC errors 00908 uint16_t LengthError; //!< Number of length errors 00909 uint16_t SyncwordError; //!< Number of sync-word errors 00910 }Gfsk; 00911 struct 00912 { 00913 uint16_t PacketReceived; //!< Number of received packets 00914 uint16_t CrcError; //!< Number of CRC errors 00915 uint16_t HeaderValid; //!< Number of valid headers 00916 }LoRa; 00917 }; 00918 }RxCounter_t; 00919 00920 /*! 00921 * \brief Represents a calibration configuration 00922 */ 00923 typedef struct 00924 { 00925 uint8_t RC64KEnable : 1; //!< Calibrate RC64K clock 00926 uint8_t RC13MEnable : 1; //!< Calibrate RC13M clock 00927 uint8_t PLLEnable : 1; //!< Calibrate PLL 00928 uint8_t ADCPulseEnable : 1; //!< Calibrate ADC Pulse 00929 uint8_t ADCBulkNEnable : 1; //!< Calibrate ADC bulkN 00930 uint8_t ADCBulkPEnable : 1; //!< Calibrate ADC bulkP 00931 }CalibrationParams_t; 00932 00933 /*! 00934 * \brief Represents a sleep mode configuration 00935 */ 00936 typedef struct 00937 { 00938 uint8_t WakeUpRTC : 1; //!< Get out of sleep mode if wakeup signal received from RTC 00939 uint8_t InstructionRamRetention : 1; //!< InstructionRam is conserved during sleep 00940 uint8_t DataBufferRetention : 1; //!< Data buffer is conserved during sleep 00941 uint8_t DataRamRetention : 1; //!< Data ram is conserved during sleep 00942 }SleepParams_t; 00943 00944 /*! 00945 * \brief Represents the SX1280 and its features 00946 * 00947 * It implements the commands the SX1280 can understands 00948 */ 00949 class SX1280 : public Radio 00950 { 00951 public: 00952 /*! 00953 * \brief Instantiates a SX1280 object and provides API functions to communicates with the radio 00954 * 00955 * \param [in] callbacks Pointer to the callbacks structure defining 00956 * all callbacks function pointers 00957 */ 00958 SX1280( RadioCallbacks_t *callbacks ): 00959 // The class members are value-initialiazed in member-initilaizer list 00960 Radio( callbacks ), OperatingMode( MODE_STDBY_RC ), PacketType( PACKET_TYPE_NONE ), 00961 LoRaBandwidth( LORA_BW_1600 ), IrqState( false ), PollingMode( false ) 00962 { 00963 this->dioIrq = &SX1280::OnDioIrq; 00964 00965 // Warning: this constructor set the LoRaBandwidth member to a valid 00966 // value, but it is not related to the actual radio configuration! 00967 } 00968 00969 virtual ~SX1280( ) 00970 { 00971 } 00972 00973 private: 00974 /*! 00975 * \brief Holds the internal operating mode of the radio 00976 */ 00977 RadioOperatingModes_t OperatingMode; 00978 00979 /*! 00980 * \brief Stores the current packet type set in the radio 00981 */ 00982 RadioPacketTypes_t PacketType; 00983 00984 /*! 00985 * \brief Stores the current LORA bandwidth set in the radio 00986 */ 00987 RadioLoRaBandwidths_t LoRaBandwidth; 00988 00989 /*! 00990 * \brief Holds a flag raised on radio interrupt 00991 */ 00992 bool IrqState; 00993 00994 /*! 00995 * \brief Hardware DIO IRQ functions 00996 */ 00997 DioIrqHandler dioIrq; 00998 00999 /*! 01000 * \brief Holds the polling state of the driver 01001 */ 01002 bool PollingMode; 01003 01004 /*! 01005 * \brief Compute the two's complement for a register of size lower than 01006 * 32bits 01007 * 01008 * \param [in] num The register to be two's complemented 01009 * \param [in] bitCnt The position of the sign bit 01010 */ 01011 static int32_t complement2( const uint32_t num, const uint8_t bitCnt ); 01012 01013 /*! 01014 * \brief Returns the value of LoRa bandwidth from driver's value 01015 * 01016 * The value is returned in Hz so that it can be represented as an integer 01017 * type. Most computation should be done as integer to reduce floating 01018 * point related errors. 01019 * 01020 * \retval loRaBw The value of the current bandwidth in Hz 01021 */ 01022 int32_t GetLoRaBandwidth( void ); 01023 01024 protected: 01025 /*! 01026 * \brief Sets a function to be triggered on radio interrupt 01027 * 01028 * \param [in] irqHandler A pointer to a function to be run on interrupt 01029 * from the radio 01030 */ 01031 virtual void IoIrqInit( DioIrqHandler irqHandler ) = 0; 01032 01033 /*! 01034 * \brief DIOs interrupt callback 01035 * 01036 * \remark Called to handle all 3 DIOs pins 01037 */ 01038 void OnDioIrq( void ); 01039 01040 /*! 01041 * \brief Set the role of the radio during ranging operations 01042 * 01043 * \param [in] role Role of the radio 01044 */ 01045 void SetRangingRole( RadioRangingRoles_t role ); 01046 01047 public: 01048 /*! 01049 * \brief Initializes the radio driver 01050 */ 01051 void Init( void ); 01052 01053 /*! 01054 * \brief Set the driver in polling mode. 01055 * 01056 * In polling mode the application is responsible to call ProcessIrqs( ) to 01057 * execute callbacks functions. 01058 * The default mode is Interrupt Mode. 01059 * @code 01060 * // Initializations and callbacks declaration/definition 01061 * radio = SX1280( mosi, miso, sclk, nss, busy, int1, int2, int3, rst, &callbacks ); 01062 * radio.Init( ); 01063 * radio.SetPollingMode( ); 01064 * 01065 * while( true ) 01066 * { 01067 * // IRQ processing is automatically done 01068 * radio.ProcessIrqs( ); // <-- here, as well as callback functions 01069 * // calls 01070 * // Do some applicative work 01071 * } 01072 * @endcode 01073 * 01074 * \see SX1280::SetInterruptMode 01075 */ 01076 void SetPollingMode( void ); 01077 01078 /*! 01079 * \brief Set the driver in interrupt mode. 01080 * 01081 * In interrupt mode, the driver communicate with the radio during the 01082 * interruption by direct calls to ProcessIrqs( ). The main advantage is 01083 * the possibility to have low power application architecture. 01084 * This is the default mode. 01085 * @code 01086 * // Initializations and callbacks declaration/definition 01087 * radio = SX1280( mosi, miso, sclk, nss, busy, int1, int2, int3, rst, &callbacks ); 01088 * radio.Init( ); 01089 * radio.SetInterruptMode( ); // Optionnal. Driver default behavior 01090 * 01091 * while( true ) 01092 * { 01093 * // Do some applicative work 01094 * } 01095 * @endcode 01096 * 01097 * \see SX1280::SetPollingMode 01098 */ 01099 void SetInterruptMode( void ); 01100 01101 /*! 01102 * \brief Initializes the radio registers to the recommended default values 01103 */ 01104 void SetRegistersDefault( void ); 01105 01106 /*! 01107 * \brief Returns the current device firmware version 01108 * 01109 * \retval version Firmware version 01110 */ 01111 virtual uint16_t GetFirmwareVersion( void ); 01112 01113 /*! 01114 * \brief Resets the radio 01115 */ 01116 virtual void Reset( void ) = 0; 01117 01118 /*! 01119 * \brief Wake-ups the radio from Sleep mode 01120 */ 01121 virtual void Wakeup( void ) = 0; 01122 01123 /*! 01124 * \brief Writes the given command to the radio 01125 * 01126 * \param [in] opcode Command opcode 01127 * \param [in] buffer Command parameters byte array 01128 * \param [in] size Command parameters byte array size 01129 */ 01130 virtual void WriteCommand( RadioCommands_t opcode, uint8_t *buffer, uint16_t size ) = 0; 01131 01132 /*! 01133 * \brief Reads the given command from the radio 01134 * 01135 * \param [in] opcode Command opcode 01136 * \param [in] buffer Command parameters byte array 01137 * \param [in] size Command parameters byte array size 01138 */ 01139 virtual void ReadCommand( RadioCommands_t opcode, uint8_t *buffer, uint16_t size ) = 0; 01140 01141 /*! 01142 * \brief Writes multiple radio registers starting at address 01143 * 01144 * \param [in] address First Radio register address 01145 * \param [in] buffer Buffer containing the new register's values 01146 * \param [in] size Number of registers to be written 01147 */ 01148 virtual void WriteRegister( uint16_t address, uint8_t *buffer, uint16_t size ) = 0; 01149 01150 /*! 01151 * \brief Writes the radio register at the specified address 01152 * 01153 * \param [in] address Register address 01154 * \param [in] value New register value 01155 */ 01156 virtual void WriteRegister( uint16_t address, uint8_t value ) = 0; 01157 01158 /*! 01159 * \brief Reads multiple radio registers starting at address 01160 * 01161 * \param [in] address First Radio register address 01162 * \param [out] buffer Buffer where to copy the registers data 01163 * \param [in] size Number of registers to be read 01164 */ 01165 virtual void ReadRegister( uint16_t address, uint8_t *buffer, uint16_t size ) = 0; 01166 01167 /*! 01168 * \brief Reads the radio register at the specified address 01169 * 01170 * \param [in] address Register address 01171 * 01172 * \retval data Register value 01173 */ 01174 virtual uint8_t ReadRegister( uint16_t address ) = 0; 01175 01176 /*! 01177 * \brief Writes Radio Data Buffer with buffer of size starting at offset. 01178 * 01179 * \param [in] offset Offset where to start writing 01180 * \param [in] buffer Buffer pointer 01181 * \param [in] size Buffer size 01182 */ 01183 virtual void WriteBuffer( uint8_t offset, uint8_t *buffer, uint8_t size ) = 0; 01184 01185 /*! 01186 * \brief Reads Radio Data Buffer at offset to buffer of size 01187 * 01188 * \param [in] offset Offset where to start reading 01189 * \param [out] buffer Buffer pointer 01190 * \param [in] size Buffer size 01191 */ 01192 virtual void ReadBuffer( uint8_t offset, uint8_t *buffer, uint8_t size ) = 0; 01193 01194 /*! 01195 * \brief Gets the current status of the radio DIOs 01196 * 01197 * \retval status [Bit #3: DIO3, Bit #2: DIO2, 01198 * Bit #1: DIO1, Bit #0: BUSY] 01199 */ 01200 virtual uint8_t GetDioStatus( void ) = 0; 01201 01202 /*! 01203 * \brief Gets the current Operation Mode of the Radio 01204 * 01205 * \retval opMode Last operating mode 01206 */ 01207 virtual RadioOperatingModes_t GetOpMode( void ); 01208 01209 /*! 01210 * \brief Gets the current radio status 01211 * 01212 * \retval status Radio status 01213 */ 01214 virtual RadioStatus_t GetStatus( void ); 01215 01216 /*! 01217 * \brief Sets the radio in sleep mode 01218 * 01219 * \param [in] sleepConfig The sleep configuration describing data 01220 * retention and RTC wake-up 01221 */ 01222 void SetSleep( SleepParams_t sleepConfig ); 01223 01224 /*! 01225 * \brief Sets the radio in configuration mode 01226 * 01227 * \param [in] mode The standby mode to put the radio into 01228 */ 01229 void SetStandby( RadioStandbyModes_t mode ); 01230 01231 /*! 01232 * \brief Sets the radio in FS mode 01233 */ 01234 void SetFs( void ); 01235 01236 /*! 01237 * \brief Sets the radio in transmission mode 01238 * 01239 * \param [in] timeout Structure describing the transmission timeout value 01240 */ 01241 void SetTx( TickTime_t timeout ); 01242 01243 /*! 01244 * \brief Sets the radio in reception mode 01245 * 01246 * \param [in] timeout Structure describing the reception timeout value 01247 */ 01248 void SetRx( TickTime_t timeout ); 01249 01250 /*! 01251 * \brief Sets the Rx duty cycle management parameters 01252 * 01253 * \param [in] periodBase Base time for Rx and Sleep TickTime sequences 01254 * \param [in] periodBaseCountRx Number of base time for Rx TickTime sequence 01255 * \param [in] periodBaseCountSleep Number of base time for Sleep TickTime sequence 01256 */ 01257 void SetRxDutyCycle( RadioTickSizes_t periodBase, uint16_t periodBaseCountRx, uint16_t periodBaseCountSleep ); 01258 01259 /*! 01260 * \brief Sets the radio in CAD mode 01261 * 01262 * \see SX1280::SetCadParams 01263 */ 01264 void SetCad( void ); 01265 01266 /*! 01267 * \brief Sets the radio in continuous wave transmission mode 01268 */ 01269 void SetTxContinuousWave( void ); 01270 01271 /*! 01272 * \brief Sets the radio in continuous preamble transmission mode 01273 */ 01274 void SetTxContinuousPreamble( void ); 01275 01276 /*! 01277 * \brief Sets the radio for the given protocol 01278 * 01279 * \param [in] packetType [PACKET_TYPE_GFSK, PACKET_TYPE_LORA, 01280 * PACKET_TYPE_RANGING, PACKET_TYPE_FLRC, 01281 * PACKET_TYPE_BLE] 01282 * 01283 * \remark This method has to be called before SetRfFrequency, 01284 * SetModulationParams and SetPacketParams 01285 */ 01286 void SetPacketType( RadioPacketTypes_t packetType ); 01287 01288 /*! 01289 * \brief Gets the current radio protocol 01290 * 01291 * Default behavior return the packet type returned by the radio. To 01292 * reduce the SPI activity and return the packet type stored by the 01293 * driver, a second optional argument must be provided evaluating as true 01294 * boolean 01295 * 01296 * \param [in] returnLocalCopy If true, the packet type returned is the last 01297 * saved in the driver 01298 * If false, the packet type is obtained from 01299 * the chip 01300 * Default: false 01301 * 01302 * \retval packetType [PACKET_TYPE_GENERIC, PACKET_TYPE_LORA, 01303 * PACKET_TYPE_RANGING, PACKET_TYPE_FLRC, 01304 * PACKET_TYPE_BLE, PACKET_TYPE_NONE] 01305 */ 01306 RadioPacketTypes_t GetPacketType( bool returnLocalCopy = false ); 01307 01308 /*! 01309 * \brief Sets the RF frequency 01310 * 01311 * \param [in] rfFrequency RF frequency [Hz] 01312 */ 01313 void SetRfFrequency( uint32_t rfFrequency ); 01314 01315 /*! 01316 * \brief Sets the transmission parameters 01317 * 01318 * \param [in] power RF output power [-18..13] dBm 01319 * \param [in] rampTime Transmission ramp up time 01320 */ 01321 void SetTxParams( int8_t power, RadioRampTimes_t rampTime ); 01322 01323 /*! 01324 * \brief Sets the number of symbols to be used for Channel Activity 01325 * Detection operation 01326 * 01327 * \param [in] cadSymbolNum The number of symbol to use for Channel Activity 01328 * Detection operations [LORA_CAD_01_SYMBOL, LORA_CAD_02_SYMBOLS, 01329 * LORA_CAD_04_SYMBOLS, LORA_CAD_08_SYMBOLS, LORA_CAD_16_SYMBOLS] 01330 */ 01331 void SetCadParams( RadioLoRaCadSymbols_t cadSymbolNum ); 01332 01333 /*! 01334 * \brief Sets the data buffer base address for transmission and reception 01335 * 01336 * \param [in] txBaseAddress Transmission base address 01337 * \param [in] rxBaseAddress Reception base address 01338 */ 01339 void SetBufferBaseAddresses( uint8_t txBaseAddress, uint8_t rxBaseAddress ); 01340 01341 /*! 01342 * \brief Set the modulation parameters 01343 * 01344 * \param [in] modParams A structure describing the modulation parameters 01345 */ 01346 void SetModulationParams( ModulationParams_t *modParams ); 01347 01348 /*! 01349 * \brief Sets the packet parameters 01350 * 01351 * \param [in] packetParams A structure describing the packet parameters 01352 */ 01353 void SetPacketParams( PacketParams_t *packetParams ); 01354 01355 /*! 01356 * \brief Gets the last received packet buffer status 01357 * 01358 * \param [out] rxPayloadLength Last received packet payload length 01359 * \param [out] rxStartBufferPointer Last received packet buffer address pointer 01360 */ 01361 void GetRxBufferStatus( uint8_t *rxPayloadLength, uint8_t *rxStartBufferPointer ); 01362 01363 /*! 01364 * \brief Gets the last received packet status 01365 * 01366 * The packet status structure returned depends on the modem type selected 01367 * 01368 * \see PacketStatus_t for the description of availabled informations 01369 * 01370 * \param [out] packetStatus A structure of packet status 01371 */ 01372 void GetPacketStatus( PacketStatus_t *packetStatus ); 01373 01374 /*! 01375 * \brief Returns the instantaneous RSSI value for the last packet received 01376 * 01377 * \retval rssiInst Instantaneous RSSI 01378 */ 01379 int8_t GetRssiInst( void ); 01380 01381 /*! 01382 * \brief Sets the IRQ mask and DIO masks 01383 * 01384 * \param [in] irqMask General IRQ mask 01385 * \param [in] dio1Mask DIO1 mask 01386 * \param [in] dio2Mask DIO2 mask 01387 * \param [in] dio3Mask DIO3 mask 01388 */ 01389 void SetDioIrqParams( uint16_t irqMask, uint16_t dio1Mask, uint16_t dio2Mask, uint16_t dio3Mask ); 01390 01391 /*! 01392 * \brief Returns the current IRQ status 01393 * 01394 * \retval irqStatus IRQ status 01395 */ 01396 uint16_t GetIrqStatus( void ); 01397 01398 /*! 01399 * \brief Clears the IRQs 01400 * 01401 * \param [in] irqMask IRQ(s) to be cleared 01402 */ 01403 void ClearIrqStatus( uint16_t irqMask ); 01404 01405 /*! 01406 * \brief Calibrates the given radio block 01407 * 01408 * \param [in] calibParam The description of blocks to be calibrated 01409 */ 01410 void Calibrate( CalibrationParams_t calibParam ); 01411 01412 /*! 01413 * \brief Sets the power regulators operating mode 01414 * 01415 * \param [in] mode [0: LDO, 1:DC_DC] 01416 */ 01417 void SetRegulatorMode( RadioRegulatorModes_t mode ); 01418 01419 /*! 01420 * \brief Saves the current selected modem configuration into data RAM 01421 */ 01422 void SetSaveContext( void ); 01423 01424 /*! 01425 * \brief Sets the chip to automatically send a packet after the end of a packet reception 01426 * 01427 * \remark The offset is automatically compensated inside the function 01428 * 01429 * \param [in] time The delay in us after which a Tx is done 01430 */ 01431 void SetAutoTx( uint16_t time ); 01432 01433 /*! 01434 * \brief Stop the chip to automatically send a packet after the end of a packet reception 01435 * if previously activated with SX1280::SetAutoTx command 01436 */ 01437 void StopAutoTx( void ); 01438 01439 /*! 01440 * \brief Sets the chip to stay in FS mode after sending a packet 01441 * 01442 * \param [in] enableAutoFs Turn on auto FS 01443 */ 01444 void SetAutoFs( bool enableAutoFs ); 01445 01446 /*! 01447 * \brief Enables or disables long preamble detection mode 01448 * 01449 * \param [in] enable Turn on long preamble mode 01450 */ 01451 void SetLongPreamble( bool enable ); 01452 01453 /*! 01454 * \brief Saves the payload to be send in the radio buffer 01455 * 01456 * \param [in] payload A pointer to the payload 01457 * \param [in] size The size of the payload 01458 * \param [in] offset The address in FIFO where writting first byte (default = 0x00) 01459 */ 01460 void SetPayload( uint8_t *payload, uint8_t size, uint8_t offset = 0x00 ); 01461 01462 /*! 01463 * \brief Reads the payload received. If the received payload is longer 01464 * than maxSize, then the method returns 1 and do not set size and payload. 01465 * 01466 * \param [out] payload A pointer to a buffer into which the payload will be copied 01467 * \param [out] size A pointer to the size of the payload received 01468 * \param [in] maxSize The maximal size allowed to copy into the buffer 01469 */ 01470 uint8_t GetPayload( uint8_t *payload, uint8_t *size, uint8_t maxSize ); 01471 01472 /*! 01473 * \brief Sends a payload 01474 * 01475 * \param [in] payload A pointer to the payload to send 01476 * \param [in] size The size of the payload to send 01477 * \param [in] timeout The timeout for Tx operation 01478 * \param [in] offset The address in FIFO where writting first byte (default = 0x00) 01479 */ 01480 void SendPayload( uint8_t *payload, uint8_t size, TickTime_t timeout, uint8_t offset = 0x00 ); 01481 01482 /*! 01483 * \brief Sets the Sync Word given by index used in GFSK, FLRC and BLE protocols 01484 * 01485 * \remark 5th byte isn't used in FLRC and BLE protocols 01486 * 01487 * \param [in] syncWordIdx Index of SyncWord to be set [1..3] 01488 * \param [in] syncWord SyncWord bytes ( 5 bytes ) 01489 * 01490 * \retval status [0: OK, 1: NOK] 01491 */ 01492 uint8_t SetSyncWord( uint8_t syncWordIdx, uint8_t *syncWord ); 01493 01494 /*! 01495 * \brief Defines how many error bits are tolerated in sync word detection 01496 * 01497 * \param [in] errorBits Number of error bits supported to validate the Sync word detection 01498 * ( default is 4 bit, minimum is 1 bit ) 01499 */ 01500 void SetSyncWordErrorTolerance( uint8_t errorBits ); 01501 01502 /*! 01503 * \brief Sets the Initial value for the LFSR used for the CRC calculation 01504 * 01505 * \param [in] seed Initial LFSR value ( 4 bytes ) 01506 * 01507 * \retval updated [0: failure, 1: success] 01508 * 01509 */ 01510 uint8_t SetCrcSeed( uint8_t *seed ); 01511 01512 /*! 01513 * \brief Set the Access Address field of BLE packet 01514 * 01515 * \param [in] accessAddress The access address to be used for next BLE packet sent 01516 * 01517 * \see SX1280::SetBleAdvertizerAccessAddress 01518 */ 01519 void SetBleAccessAddress( uint32_t accessAddress ); 01520 01521 /*! 01522 * \brief Set the Access Address for Advertizer BLE packets 01523 * 01524 * All advertizer BLE packets must use a particular value for Access 01525 * Address field. This method sets it. 01526 * 01527 * \see SX1280::SetBleAccessAddress 01528 */ 01529 void SetBleAdvertizerAccessAddress( void ); 01530 01531 /*! 01532 * \brief Sets the seed used for the CRC calculation 01533 * 01534 * \param [in] polynomial The seed value 01535 * 01536 */ 01537 void SetCrcPolynomial( uint16_t polynomial ); 01538 01539 /*! 01540 * \brief Sets the Initial value of the LFSR used for the whitening in GFSK, FLRC and BLE protocols 01541 * 01542 * \param [in] seed Initial LFSR value 01543 */ 01544 void SetWhiteningSeed( uint8_t seed ); 01545 01546 /*! 01547 * \brief Enable manual gain control and disable AGC 01548 * 01549 * \see SX1280::SetManualGainValue, SX1280::DisableManualGain 01550 */ 01551 void EnableManualGain( void ); 01552 01553 /*! 01554 * \brief Disable the manual gain control and enable AGC 01555 * 01556 * \see SX1280::EnableManualGain 01557 */ 01558 void DisableManualGain( void ); 01559 01560 /*! 01561 * \brief Set the gain for the AGC 01562 * 01563 * SX1280::EnableManualGain must be called before using this method 01564 * 01565 * \param [in] gain The value of gain to set, refer to datasheet for value meaning 01566 * 01567 * \see SX1280::EnableManualGain, SX1280::DisableManualGain 01568 */ 01569 void SetManualGainValue( uint8_t gain ); 01570 01571 /*! 01572 * \brief Configure the LNA regime of operation 01573 * 01574 * \param [in] lnaSetting The LNA setting. Possible values are 01575 * LNA_LOW_POWER_MODE and 01576 * LNA_HIGH_SENSITIVITY_MODE 01577 */ 01578 void SetLNAGainSetting( const RadioLnaSettings_t lnaSetting ); 01579 01580 /*! 01581 * \brief Sets the number of bits used to check that ranging request match ranging ID 01582 * 01583 * \param [in] length [0: 8 bits, 1: 16 bits, 01584 * 2: 24 bits, 3: 32 bits] 01585 */ 01586 void SetRangingIdLength( RadioRangingIdCheckLengths_t length ); 01587 01588 /*! 01589 * \brief Sets ranging device id 01590 * 01591 * \param [in] address Device address 01592 */ 01593 void SetDeviceRangingAddress( uint32_t address ); 01594 01595 /*! 01596 * \brief Sets the device id to ping in a ranging request 01597 * 01598 * \param [in] address Address of the device to ping 01599 */ 01600 void SetRangingRequestAddress( uint32_t address ); 01601 01602 /*! 01603 * \brief Return the ranging result value 01604 * 01605 * \param [in] resultType Specifies the type of result. 01606 * [0: RAW, 1: Averaged, 01607 * 2: De-biased, 3:Filtered] 01608 * 01609 * \retval ranging The ranging measure filtered according to resultType [m] 01610 */ 01611 double GetRangingResult( RadioRangingResultTypes_t resultType ); 01612 01613 /*! 01614 * \brief Return the last ranging result power indicator 01615 * 01616 * The value returned is not an absolute power measurement. It is 01617 * a relative power measurement. 01618 * 01619 * \retval deltaThreshold A relative power indicator 01620 */ 01621 uint8_t GetRangingPowerDeltaThresholdIndicator( void ); 01622 01623 01624 01625 /*! 01626 * \brief Sets the standard processing delay between Master and Slave 01627 * 01628 * \param [in] cal RxTx delay offset for correcting ranging bias. 01629 * 01630 * The calibration value reflects the group delay of the radio front end and 01631 * must be re-performed for each new SX1280 PCB design. The value is obtained 01632 * empirically by either conducted measurement in a known electrical length 01633 * coaxial RF cable (where the design is connectorised) or by radiated 01634 * measurement, at a known distance, where an antenna is present. 01635 * The result of the calibration process is that the SX1280 ranging result 01636 * accurately reflects the physical range, the calibration procedure therefore 01637 * removes the average timing error from the time-of-flight measurement for a 01638 * given design. 01639 * 01640 * The values are Spreading Factor dependents, and depend also of the board 01641 * design. 01642 */ 01643 void SetRangingCalibration( uint16_t cal ); 01644 01645 /*! 01646 * \brief Clears the ranging filter 01647 */ 01648 void RangingClearFilterResult( void ); 01649 01650 /*! 01651 * \brief Set the number of samples considered in the built-in filter 01652 * 01653 * \param [in] numSample The number of samples to use built-in filter 01654 * [8..255] 01655 * 01656 * \remark Value inferior to 8 will be silently set to 8 01657 */ 01658 void RangingSetFilterNumSamples( uint8_t numSample ); 01659 01660 /*! 01661 * \brief Return the Estimated Frequency Error in LORA and RANGING operations 01662 * 01663 * \retval efe The estimated frequency error [Hz] 01664 */ 01665 double GetFrequencyError( ); 01666 01667 /*! 01668 * \brief Process the analysis of radio IRQs and calls callback functions 01669 * depending on radio state 01670 */ 01671 void ProcessIrqs( void ); 01672 01673 /*! 01674 * \brief Force the preamble length in GFSK and BLE mode 01675 * 01676 * \param [in] preambleLength The desired preamble length 01677 */ 01678 void ForcePreambleLength( RadioPreambleLengths_t preambleLength ); 01679 }; 01680 01681 #endif // __SX1280_H__
Generated on Tue Jul 12 2022 19:08:29 by
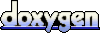