
This code holds the complete demo set for the sx1280: PingPong, PER and Ranging Outdoor demo application. >>>>> This code MUST run on the mbed library release 127 or everything will be painfully slow.
Dependencies: mbed SX1280Lib DmTftLibrary
SX9306.h
00001 /* 00002 ______ _ 00003 / _____) _ | | 00004 ( (____ _____ ____ _| |_ _____ ____| |__ 00005 \____ \| ___ | (_ _) ___ |/ ___) _ \ 00006 _____) ) ____| | | || |_| ____( (___| | | | 00007 (______/|_____)_|_|_| \__)_____)\____)_| |_| 00008 (C)2016 Semtech 00009 00010 Description: SX9306 Proximity header 00011 00012 Maintainer: Gregory Cristian & Gilbert Menth 00013 */ 00014 00015 #ifndef PROXIMITY_H 00016 #define PROXIMITY_H 00017 00018 00019 #define ANTENNA_1 0 00020 #define ANTENNA_2 1 00021 00022 00023 #define REG_IRQ_SRC ( 0x00 ) // Interrupt sources 00024 #define REG_STATUS ( 0x01 ) // Status 00025 #define REG_IRQ_MASK ( 0x03 ) // Irq mask 00026 #define REG_SENSORSEL ( 0x20 ) // Select which sensor 00027 #define REG_PROXUSEFUL ( 0x21 ) // Instantaneous sensor value 00028 #define REG_PROXAVG ( 0x23 ) // Averaged sensor value 00029 #define REG_CONTROL_0 ( 0x06 ) // Enable and scan period 00030 #define REG_CONTROL_1 ( 0x07 ) // 00031 #define REG_CONTROL_2 ( 0x08 ) // 00032 #define REG_CONTROL_3 ( 0x09 ) // Doze and filter 00033 #define REG_CONTROL_4 ( 0x0A ) // 00034 #define REG_CONTROL_5 ( 0x0B ) // 00035 #define REG_CONTROL_6 ( 0x0C ) // 00036 #define REG_CONTROL_7 ( 0x0D ) // 00037 #define REG_CONTROL_8 ( 0x0E ) // 00038 00039 #define SAR_RATIO_THRESH ( 0x10 ) //Just for test 00040 00041 #define SENSOR_SEL_1 ( 0x02 ) // Select antenna 1 00042 #define SENSOR_SEL_2 ( 0x03 ) // Select antenna 2 00043 #define SENSOR_ENABLE_23 ( 0x0C ) // Enabled sensors 2 & 3 (anteenas 1 & 2) 00044 #define SENSOR_DOZE_OFF ( 0x00 ) // Prevents doze mode starting 00045 #define PROXIMITY_I2C_ADDR ( 0x54 ) // Proximity IC I2C address 00046 #define MAX_GAIN ( 0x77 ) // Maximum gain and best granularity 00047 00048 struct ProximityStruct 00049 { 00050 int16_t Instantaneous; 00051 int16_t Averaged; 00052 }; 00053 00054 00055 /*! 00056 * \brief Initialses the hardware and variables associated with the SX9306. 00057 */ 00058 void SX9306ProximityInit( void ); 00059 00060 /*! 00061 * \brief Called from the main loop in order to deal with SX9306 communications. 00062 */ 00063 void SX9306ProximityHandle( void ); 00064 00065 /*! 00066 * \brief Generic command used to read and write from the various SX9306 00067 * registers. Called from the USB serial port 00068 * 00069 * \param [in] WriteNotRead If true defines a write operation 00070 * \param [in] Address Addess of the register inside the SX9306 to access 00071 * \param [in] WriteValue Value to be written to the defined register, if a 00072 * write is specified 00073 * \param [in] *ReadValue Pointer to the where a read operation should place 00074 * the data 00075 * 00076 * \retval Status Non zero = sucess. 00077 */ 00078 uint8_t SX9306proximitySerialCommand( uint8_t writeNotRead, uint8_t address, \ 00079 uint8_t writeValue, uint8_t *readValue ); 00080 00081 /*! 00082 * \brief Generic command used to read and write from the various SX9306 00083 * registers. Called from the USB serial port 00084 * 00085 * \param [in] ThisAntenna Defines which antenna is to be read (0 or 1) 00086 * 00087 * \retval Value The value read from the defined antenna. 00088 */ 00089 uint16_t SX9306proximityGetReadValue( uint32_t thisAntenna ); 00090 00091 #endif //PROXIMITY_H 00092
Generated on Wed Jul 13 2022 01:03:22 by
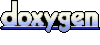