
This code holds the complete demo set for the sx1280: PingPong, PER and Ranging Outdoor demo application. >>>>> This code MUST run on the mbed library release 127 or everything will be painfully slow.
Dependencies: mbed SX1280Lib DmTftLibrary
SX9306.cpp
00001 /* 00002 ______ _ 00003 / _____) _ | | 00004 ( (____ _____ ____ _| |_ _____ ____| |__ 00005 \____ \| ___ | (_ _) ___ |/ ___) _ \ 00006 _____) ) ____| | | || |_| ____( (___| | | | 00007 (______/|_____)_|_|_| \__)_____)\____)_| |_| 00008 (C)2016 Semtech 00009 00010 Description: SX9306 Proximity sensor 00011 00012 Maintainer: Gregory Cristian & Gilbert Menth 00013 */ 00014 00015 #include "mbed.h" 00016 #include <stdio.h> 00017 #include "DmTftBase.h" 00018 #include "SX9306.h" 00019 #include "Timers.h" 00020 #include "Eeprom.h" 00021 00022 union ProximityData_t 00023 { 00024 ProximityStruct ThisAntenna; 00025 char Buffer[sizeof( ProximityStruct )]; 00026 }; 00027 00028 typedef enum 00029 { 00030 PROXIMITY_STATE_START_RESET, 00031 PROXIMITY_STATE_WAIT_RESET, 00032 PROXIMITY_STATE_INIT, 00033 PROXIMITY_STATE_READ, 00034 PROXIMITY_STATE_WAIT 00035 }ProximityAppState_t; 00036 00037 00038 DigitalOut ProximityIcReset( PA_1 ); 00039 I2C ProxI2C( I2C_SDA, I2C_SCL ); 00040 00041 static uint32_t ProximityTimer; 00042 static char ReadDataBuffer[2]; 00043 static char WriteDataBuffer[2]; 00044 static ProximityAppState_t ProximityAppState; 00045 static ProximityData_t ProximityReading; 00046 static ProximityStruct Antenna[2]; 00047 00048 00049 static bool SX9306ProximityReadRegister( char thisRegAddress, char *value ); 00050 static bool SX9306ProximityWriteRegister( char thisRegAddress, char value ); 00051 static bool SX9306ProximityReadAntennaValues( void ); 00052 00053 00054 void SX9306ProximityInit( void ) 00055 { 00056 int count; 00057 00058 ProximityAppState = PROXIMITY_STATE_START_RESET; 00059 for( count = 0; count < 2; count++ ) 00060 { 00061 Antenna[count].Averaged = 0; 00062 Antenna[count].Instantaneous = 0; 00063 } 00064 } 00065 00066 void SX9306ProximityHandle( void ) 00067 { 00068 bool i2cResult; 00069 00070 switch( ProximityAppState ) 00071 { 00072 case PROXIMITY_STATE_START_RESET: 00073 ProximityIcReset = false; 00074 TimersSetTimer( &ProximityTimer, 10 * TIM_MSEC ); 00075 ProximityAppState = PROXIMITY_STATE_WAIT_RESET; 00076 break; 00077 00078 case PROXIMITY_STATE_WAIT_RESET: 00079 if( TimersTimerHasExpired( &ProximityTimer ) ) 00080 { 00081 ProximityIcReset = true; 00082 TimersSetTimer( &ProximityTimer, 10 * TIM_MSEC ); 00083 ProximityAppState = PROXIMITY_STATE_INIT; 00084 } 00085 break; 00086 00087 case PROXIMITY_STATE_INIT: 00088 if( TimersTimerHasExpired( &ProximityTimer ) ) 00089 { 00090 TimersSetTimer( &ProximityTimer, 1 * TIM_SEC ); 00091 //Clear interrupts after power on 00092 i2cResult = SX9306ProximityReadRegister( REG_IRQ_SRC, \ 00093 &ReadDataBuffer[0] ); 00094 if( !i2cResult ) 00095 { 00096 //Enable antennas 1 & 2 00097 i2cResult = SX9306ProximityWriteRegister( REG_CONTROL_0, \ 00098 SENSOR_ENABLE_23 ); 00099 if( !i2cResult ) 00100 { 00101 //Stop doze mode 00102 i2cResult = SX9306ProximityWriteRegister( REG_CONTROL_3, \ 00103 SENSOR_DOZE_OFF ); 00104 if( !i2cResult ) 00105 { 00106 //Set max gain and granularity 00107 i2cResult = SX9306ProximityWriteRegister( REG_CONTROL_2, \ 00108 MAX_GAIN ); 00109 } 00110 } 00111 } 00112 if( !i2cResult ) 00113 { 00114 ProximityAppState = PROXIMITY_STATE_READ; 00115 } 00116 else 00117 { 00118 ProximityAppState = PROXIMITY_STATE_START_RESET; 00119 } 00120 } 00121 break; 00122 00123 case PROXIMITY_STATE_READ: 00124 TimersSetTimer( &ProximityTimer, 1 * TIM_SEC ); 00125 //Select antenna 1 00126 i2cResult = SX9306ProximityWriteRegister( REG_SENSORSEL, SENSOR_SEL_1 ); 00127 if( i2cResult ) 00128 { 00129 ProximityAppState = PROXIMITY_STATE_START_RESET; 00130 break; 00131 } 00132 i2cResult = SX9306ProximityReadAntennaValues( ); //Read values 00133 if( i2cResult ) 00134 { 00135 ProximityAppState = PROXIMITY_STATE_START_RESET; 00136 break; 00137 } 00138 Antenna[ANTENNA_1] = ProximityReading.ThisAntenna; 00139 //Select antenna 2 00140 i2cResult = SX9306ProximityWriteRegister( REG_SENSORSEL, SENSOR_SEL_2 ); 00141 if( i2cResult ) 00142 { 00143 ProximityAppState = PROXIMITY_STATE_START_RESET; 00144 break; 00145 } 00146 i2cResult = SX9306ProximityReadAntennaValues( ); //Read values 00147 if( i2cResult ) 00148 { 00149 ProximityAppState = PROXIMITY_STATE_START_RESET; 00150 break; 00151 } 00152 Antenna[ANTENNA_2] = ProximityReading.ThisAntenna; 00153 ProximityAppState = PROXIMITY_STATE_WAIT; 00154 break; 00155 00156 case PROXIMITY_STATE_WAIT: 00157 if( TimersTimerHasExpired( &ProximityTimer ) ) 00158 { 00159 ProximityAppState = PROXIMITY_STATE_READ; 00160 } 00161 break; 00162 } 00163 } 00164 00165 static bool SX9306ProximityReadRegister( char thisRegAddress, char *value ) 00166 { 00167 WriteDataBuffer[0] = thisRegAddress; 00168 // Write the register address 00169 if( ProxI2C.write( PROXIMITY_I2C_ADDR, &WriteDataBuffer[0], 1, 0 ) ) 00170 { 00171 return true; 00172 } 00173 // Read data from the address 00174 if( ProxI2C.read( PROXIMITY_I2C_ADDR, value, 1, 0 ) ) 00175 { 00176 return true; 00177 } 00178 return false; 00179 } 00180 00181 static bool SX9306ProximityWriteRegister( char thisRegAddress, char value ) 00182 { 00183 WriteDataBuffer[0] = thisRegAddress; 00184 WriteDataBuffer[1] = value; 00185 00186 // Write the register address and data byte 00187 if( ProxI2C.write( PROXIMITY_I2C_ADDR, &WriteDataBuffer[0], 2, 0 ) ) 00188 { 00189 return true; 00190 } 00191 return false; 00192 } 00193 00194 static bool SX9306ProximityReadAntennaValues( void ) 00195 { 00196 bool i2cResult; 00197 00198 i2cResult = SX9306ProximityReadRegister( REG_PROXUSEFUL, \ 00199 &ProximityReading.Buffer[1] ); 00200 if( i2cResult ) 00201 { 00202 return true; 00203 } 00204 i2cResult = SX9306ProximityReadRegister( REG_PROXUSEFUL + 1, \ 00205 &ProximityReading.Buffer[0] ); 00206 if( i2cResult ) 00207 { 00208 return true; 00209 } 00210 i2cResult = SX9306ProximityReadRegister( REG_PROXAVG, \ 00211 &ProximityReading.Buffer[3] ); 00212 if( i2cResult ) 00213 { 00214 return true; 00215 } 00216 i2cResult = SX9306ProximityReadRegister( REG_PROXAVG + 1, \ 00217 &ProximityReading.Buffer[2] ); 00218 if( i2cResult ) 00219 { 00220 return true; 00221 } 00222 return false; 00223 } 00224 00225 uint8_t SX9306proximitySerialCommand( uint8_t writeNotRead, uint8_t address, \ 00226 uint8_t writeValue, uint8_t *readValue ) 00227 { 00228 char valueRead = 0; 00229 bool i2cResult; 00230 00231 if( writeNotRead ) 00232 { 00233 i2cResult = SX9306ProximityWriteRegister( address, writeValue ); 00234 } 00235 else 00236 { 00237 i2cResult = SX9306ProximityReadRegister( address, &valueRead ); 00238 *readValue = ( uint8_t )valueRead; 00239 } 00240 if( i2cResult ) 00241 { 00242 return 0; 00243 } 00244 return 1; 00245 } 00246 00247 uint16_t SX9306proximityGetReadValue( uint32_t thisAntenna ) 00248 { 00249 uint16_t retVal; 00250 00251 switch( thisAntenna ) 00252 { 00253 case 0: 00254 case 1: 00255 retVal = Antenna[thisAntenna].Instantaneous; 00256 break; 00257 00258 default: 00259 retVal = Antenna[0].Instantaneous; 00260 break; 00261 } 00262 return retVal; 00263 } 00264
Generated on Wed Jul 13 2022 01:03:22 by
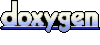