
This code holds the complete demo set for the sx1280: PingPong, PER and Ranging Outdoor demo application. >>>>> This code MUST run on the mbed library release 127 or everything will be painfully slow.
Dependencies: mbed SX1280Lib DmTftLibrary
GpsMax7.h
00001 /* 00002 ______ _ 00003 / _____) _ | | 00004 ( (____ _____ ____ _| |_ _____ ____| |__ 00005 \____ \| ___ | (_ _) ___ |/ ___) _ \ 00006 _____) ) ____| | | || |_| ____( (___| | | | 00007 (______/|_____)_|_|_| \__)_____)\____)_| |_| 00008 (C)2016 Semtech 00009 00010 Description: uBlox MAX7 GPS header 00011 00012 Maintainer: Gregory Cristian & Gilbert Menth 00013 */ 00014 00015 #ifndef MAX7_GPS_H 00016 #define MAX7_GPS_H 00017 00018 00019 /*! 00020 * \brief GPGGA format structure. 00021 */ 00022 struct GpggaStruct 00023 { 00024 char Lat[15]; 00025 char Long[15]; 00026 char NumSats[3]; 00027 bool Fixed; 00028 bool Updated; 00029 }; 00030 00031 /*! 00032 * \brief GPZDA format structure. 00033 */ 00034 struct GpzdaStruct 00035 { 00036 char Hour[3]; 00037 char Minute[3]; 00038 char Second[3]; 00039 char Day[3]; 00040 char Month[3]; 00041 char Year[5]; 00042 bool Updated; 00043 }; 00044 00045 /*! 00046 * \brief GPS data structure. 00047 */ 00048 struct GpsStruct 00049 { 00050 GpggaStruct Position; 00051 GpzdaStruct Time; 00052 }; 00053 00054 00055 /*! 00056 * \brief Initialses the hardware and variables associated with the MAX7. 00057 */ 00058 void Max7GpsInit( void ); 00059 00060 /*! 00061 * \brief Returns the required data from the MAX7. 00062 * 00063 * \retval GpsStruct* Pointer to the current GPS data. 00064 */ 00065 GpsStruct* Max7GpsgetData( void ); 00066 00067 /*! 00068 * \brief Called from the main loop in order to deal with the MAX7 communications. 00069 */ 00070 void Max7GpsHandle( void ); 00071 00072 #endif //MAX7_GPS_H 00073
Generated on Wed Jul 13 2022 01:03:22 by
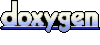