Driver for the SX1276 RF Transceiver
Dependents: LoRa_PIR LoRaWAN-lmic-app SX1276PingPong LoRaWAN-lmic-app ... more
radio.h
00001 /* 00002 / _____) _ | | 00003 ( (____ _____ ____ _| |_ _____ ____| |__ 00004 \____ \| ___ | (_ _) ___ |/ ___) _ \ 00005 _____) ) ____| | | || |_| ____( (___| | | | 00006 (______/|_____)_|_|_| \__)_____)\____)_| |_| 00007 (C) 2014 Semtech 00008 00009 Description: Interface for the radios, contains the main functions that a radio needs, and 5 callback functions 00010 00011 License: Revised BSD License, see LICENSE.TXT file include in the project 00012 00013 Maintainers: Miguel Luis, Gregory Cristian and Nicolas Huguenin 00014 */ 00015 #ifndef __RADIO_H__ 00016 #define __RADIO_H__ 00017 00018 #include "mbed.h" 00019 00020 #include "./enums/enums.h" 00021 00022 /*! 00023 * @brief Radio driver callback functions 00024 */ 00025 typedef struct 00026 { 00027 /*! 00028 * @brief Tx Done callback prototype. 00029 */ 00030 void ( *TxDone )( void ); 00031 /*! 00032 * @brief Tx Timeout callback prototype. 00033 */ 00034 void ( *TxTimeout )( void ); 00035 /*! 00036 * @brief Rx Done callback prototype. 00037 * 00038 * @param [IN] payload Received buffer pointer 00039 * @param [IN] size Received buffer size 00040 * @param [IN] rssi RSSI value computed while receiving the frame [dBm] 00041 * @param [IN] snr Raw SNR value given by the radio hardware 00042 * FSK : N/A ( set to 0 ) 00043 * LoRa: SNR value in dB 00044 */ 00045 void ( *RxDone )( uint8_t *payload, uint16_t size, int16_t rssi, int8_t snr ); 00046 /*! 00047 * @brief Rx Timeout callback prototype. 00048 */ 00049 void ( *RxTimeout )( void ); 00050 /*! 00051 * @brief Rx Error callback prototype. 00052 */ 00053 void ( *RxError )( void ); 00054 /*! 00055 * \brief FHSS Change Channel callback prototype. 00056 * 00057 * \param [IN] currentChannel Index number of the current channel 00058 */ 00059 void ( *FhssChangeChannel )( uint8_t currentChannel ); 00060 /*! 00061 * @brief CAD Done callback prototype. 00062 * 00063 * @param [IN] channelDetected Channel Activity detected during the CAD 00064 */ 00065 void ( *CadDone ) ( bool channelActivityDetected ); 00066 }RadioEvents_t; 00067 00068 /*! 00069 * Interface for the radios, contains the main functions that a radio needs, and 5 callback functions 00070 */ 00071 class Radio 00072 { 00073 protected: 00074 RadioEvents_t* RadioEvents; 00075 00076 public: 00077 //------------------------------------------------------------------------- 00078 // Constructor 00079 //------------------------------------------------------------------------- 00080 /*! 00081 * @brief Constructor of the radio object, the parameters are the callback functions described in the header. 00082 * 00083 * @param [IN] events Structure containing the driver callback functions 00084 */ 00085 Radio( RadioEvents_t *events ); 00086 virtual ~Radio ( ) {}; 00087 00088 //------------------------------------------------------------------------- 00089 // Pure virtual functions 00090 //------------------------------------------------------------------------- 00091 /*! 00092 * @brief Initializes the radio 00093 * 00094 * @param [IN] events Structure containing the driver callback functions 00095 */ 00096 virtual void Init( RadioEvents_t *events ) = 0; 00097 /*! 00098 * @brief Return current radio status 00099 * 00100 * @param status Radio status.[RF_IDLE, RF_RX_RUNNING, RF_TX_RUNNING] 00101 */ 00102 virtual RadioState GetStatus( void ) = 0; 00103 /*! 00104 * @brief Configures the radio with the given modem 00105 * 00106 * @param [IN] modem Modem to be used [0: FSK, 1: LoRa] 00107 */ 00108 virtual void SetModem( RadioModems_t modem ) = 0; 00109 /*! 00110 * @brief Sets the channel frequency 00111 * 00112 * @param [IN] freq Channel RF frequency 00113 */ 00114 virtual void SetChannel( uint32_t freq ) = 0; 00115 /*! 00116 * @brief Sets the channels configuration 00117 * 00118 * @param [IN] modem Radio modem to be used [0: FSK, 1: LoRa] 00119 * @param [IN] freq Channel RF frequency 00120 * @param [IN] rssiThresh RSSI threshold 00121 * 00122 * @retval isFree [true: Channel is free, false: Channel is not free] 00123 */ 00124 virtual bool IsChannelFree( RadioModems_t modem, uint32_t freq, int16_t rssiThresh ) = 0; 00125 /*! 00126 * @brief Generates a 32 bits random value based on the RSSI readings 00127 * 00128 * \remark This function sets the radio in LoRa modem mode and disables 00129 * all interrupts. 00130 * After calling this function either Radio.SetRxConfig or 00131 * Radio.SetTxConfig functions must be called. 00132 * 00133 * @retval randomValue 32 bits random value 00134 */ 00135 virtual uint32_t Random( void )= 0; 00136 /*! 00137 * @brief Sets the reception parameters 00138 * 00139 * @param [IN] modem Radio modem to be used [0: FSK, 1: LoRa] 00140 * @param [IN] bandwidth Sets the bandwidth 00141 * FSK : >= 2600 and <= 250000 Hz 00142 * LoRa: [0: 125 kHz, 1: 250 kHz, 00143 * 2: 500 kHz, 3: Reserved] 00144 * @param [IN] datarate Sets the Datarate 00145 * FSK : 600..300000 bits/s 00146 * LoRa: [6: 64, 7: 128, 8: 256, 9: 512, 00147 * 10: 1024, 11: 2048, 12: 4096 chips] 00148 * @param [IN] coderate Sets the coding rate ( LoRa only ) 00149 * FSK : N/A ( set to 0 ) 00150 * LoRa: [1: 4/5, 2: 4/6, 3: 4/7, 4: 4/8] 00151 * @param [IN] bandwidthAfc Sets the AFC Bandwidth ( FSK only ) 00152 * FSK : >= 2600 and <= 250000 Hz 00153 * LoRa: N/A ( set to 0 ) 00154 * @param [IN] preambleLen Sets the Preamble length ( LoRa only ) 00155 * FSK : N/A ( set to 0 ) 00156 * LoRa: Length in symbols ( the hardware adds 4 more symbols ) 00157 * @param [IN] symbTimeout Sets the RxSingle timeout value 00158 * FSK : timeout number of bytes 00159 * LoRa: timeout in symbols 00160 * @param [IN] fixLen Fixed length packets [0: variable, 1: fixed] 00161 * @param [IN] payloadLen Sets payload length when fixed lenght is used 00162 * @param [IN] crcOn Enables/Disables the CRC [0: OFF, 1: ON] 00163 * @param [IN] freqHopOn Enables disables the intra-packet frequency hopping [0: OFF, 1: ON] (LoRa only) 00164 * @param [IN] hopPeriod Number of symbols bewteen each hop (LoRa only) 00165 * @param [IN] iqInverted Inverts IQ signals ( LoRa only ) 00166 * FSK : N/A ( set to 0 ) 00167 * LoRa: [0: not inverted, 1: inverted] 00168 * @param [IN] rxContinuous Sets the reception in continuous mode 00169 * [false: single mode, true: continuous mode] 00170 */ 00171 virtual void SetRxConfig ( RadioModems_t modem, uint32_t bandwidth, 00172 uint32_t datarate, uint8_t coderate, 00173 uint32_t bandwidthAfc, uint16_t preambleLen, 00174 uint16_t symbTimeout, bool fixLen, 00175 uint8_t payloadLen, 00176 bool crcOn, bool freqHopOn, uint8_t hopPeriod, 00177 bool iqInverted, bool rxContinuous ) = 0; 00178 /*! 00179 * @brief Sets the transmission parameters 00180 * 00181 * @param [IN] modem Radio modem to be used [0: FSK, 1: LoRa] 00182 * @param [IN] power Sets the output power [dBm] 00183 * @param [IN] fdev Sets the frequency deviation ( FSK only ) 00184 * FSK : [Hz] 00185 * LoRa: 0 00186 * @param [IN] bandwidth Sets the bandwidth ( LoRa only ) 00187 * FSK : 0 00188 * LoRa: [0: 125 kHz, 1: 250 kHz, 00189 * 2: 500 kHz, 3: Reserved] 00190 * @param [IN] datarate Sets the Datarate 00191 * FSK : 600..300000 bits/s 00192 * LoRa: [6: 64, 7: 128, 8: 256, 9: 512, 00193 * 10: 1024, 11: 2048, 12: 4096 chips] 00194 * @param [IN] coderate Sets the coding rate ( LoRa only ) 00195 * FSK : N/A ( set to 0 ) 00196 * LoRa: [1: 4/5, 2: 4/6, 3: 4/7, 4: 4/8] 00197 * @param [IN] preambleLen Sets the preamble length 00198 * @param [IN] fixLen Fixed length packets [0: variable, 1: fixed] 00199 * @param [IN] crcOn Enables disables the CRC [0: OFF, 1: ON] 00200 * @param [IN] freqHopOn Enables disables the intra-packet frequency hopping [0: OFF, 1: ON] (LoRa only) 00201 * @param [IN] hopPeriod Number of symbols bewteen each hop (LoRa only) 00202 * @param [IN] iqInverted Inverts IQ signals ( LoRa only ) 00203 * FSK : N/A ( set to 0 ) 00204 * LoRa: [0: not inverted, 1: inverted] 00205 * @param [IN] timeout Transmission timeout [us] 00206 */ 00207 virtual void SetTxConfig( RadioModems_t modem, int8_t power, uint32_t fdev, 00208 uint32_t bandwidth, uint32_t datarate, 00209 uint8_t coderate, uint16_t preambleLen, 00210 bool fixLen, bool crcOn, bool freqHopOn, 00211 uint8_t hopPeriod, bool iqInverted, uint32_t timeout ) = 0; 00212 /*! 00213 * @brief Checks if the given RF frequency is supported by the hardware 00214 * 00215 * @param [IN] frequency RF frequency to be checked 00216 * @retval isSupported [true: supported, false: unsupported] 00217 */ 00218 virtual bool CheckRfFrequency( uint32_t frequency ) = 0; 00219 /*! 00220 * @brief Computes the packet time on air for the given payload 00221 * 00222 * \Remark Can only be called once SetRxConfig or SetTxConfig have been called 00223 * 00224 * @param [IN] modem Radio modem to be used [0: FSK, 1: LoRa] 00225 * @param [IN] pktLen Packet payload length 00226 * 00227 * @retval airTime Computed airTime for the given packet payload length 00228 */ 00229 virtual uint32_t TimeOnAir ( RadioModems_t modem, uint8_t pktLen ) = 0; 00230 /*! 00231 * @brief Sends the buffer of size. Prepares the packet to be sent and sets 00232 * the radio in transmission 00233 * 00234 * @param [IN]: buffer Buffer pointer 00235 * @param [IN]: size Buffer size 00236 */ 00237 virtual void Send( uint8_t *buffer, uint8_t size ) = 0; 00238 /*! 00239 * @brief Sets the radio in sleep mode 00240 */ 00241 virtual void Sleep( void ) = 0; 00242 /*! 00243 * @brief Sets the radio in standby mode 00244 */ 00245 virtual void Standby( void ) = 0; 00246 /*! 00247 * @brief Sets the radio in CAD mode 00248 */ 00249 virtual void StartCad( void ) = 0; 00250 /*! 00251 * @brief Sets the radio in reception mode for the given time 00252 * @param [IN] timeout Reception timeout [us] 00253 * [0: continuous, others timeout] 00254 */ 00255 virtual void Rx( uint32_t timeout ) = 0; 00256 /*! 00257 * @brief Sets the radio in transmission mode for the given time 00258 * @param [IN] timeout Transmission timeout [us] 00259 * [0: continuous, others timeout] 00260 */ 00261 virtual void Tx( uint32_t timeout ) = 0; 00262 /*! 00263 * @brief Sets the radio in continuous wave transmission mode 00264 * 00265 * @param [IN]: freq Channel RF frequency 00266 * @param [IN]: power Sets the output power [dBm] 00267 * @param [IN]: time Transmission mode timeout [s] 00268 */ 00269 virtual void SetTxContinuousWave( uint32_t freq, int8_t power, uint16_t time ) = 0; 00270 /*! 00271 * @brief Reads the current RSSI value 00272 * 00273 * @retval rssiValue Current RSSI value in [dBm] 00274 */ 00275 virtual int16_t GetRssi ( RadioModems_t modem ) = 0; 00276 /*! 00277 * @brief Writes the radio register at the specified address 00278 * 00279 * @param [IN]: addr Register address 00280 * @param [IN]: data New register value 00281 */ 00282 virtual void Write ( uint8_t addr, uint8_t data ) = 0; 00283 /*! 00284 * @brief Reads the radio register at the specified address 00285 * 00286 * @param [IN]: addr Register address 00287 * @retval data Register value 00288 */ 00289 virtual uint8_t Read ( uint8_t addr ) = 0; 00290 /*! 00291 * @brief Writes multiple radio registers starting at address 00292 * 00293 * @param [IN] addr First Radio register address 00294 * @param [IN] buffer Buffer containing the new register's values 00295 * @param [IN] size Number of registers to be written 00296 */ 00297 virtual void Write( uint8_t addr, uint8_t *buffer, uint8_t size ) = 0; 00298 /*! 00299 * @brief Reads multiple radio registers starting at address 00300 * 00301 * @param [IN] addr First Radio register address 00302 * @param [OUT] buffer Buffer where to copy the registers data 00303 * @param [IN] size Number of registers to be read 00304 */ 00305 virtual void Read ( uint8_t addr, uint8_t *buffer, uint8_t size ) = 0; 00306 /*! 00307 * @brief Writes the buffer contents to the Radio FIFO 00308 * 00309 * @param [IN] buffer Buffer containing data to be put on the FIFO. 00310 * @param [IN] size Number of bytes to be written to the FIFO 00311 */ 00312 virtual void WriteFifo( uint8_t *buffer, uint8_t size ) = 0; 00313 /*! 00314 * @brief Reads the contents of the Radio FIFO 00315 * 00316 * @param [OUT] buffer Buffer where to copy the FIFO read data. 00317 * @param [IN] size Number of bytes to be read from the FIFO 00318 */ 00319 virtual void ReadFifo( uint8_t *buffer, uint8_t size ) = 0; 00320 /*! 00321 * @brief Sets the maximum payload length. 00322 * 00323 * @param [IN] modem Radio modem to be used [0: FSK, 1: LoRa] 00324 * @param [IN] max Maximum payload length in bytes 00325 */ 00326 virtual void SetMaxPayloadLength( RadioModems_t modem, uint8_t max ) = 0; 00327 /*! 00328 * @brief Sets the network to public or private. Updates the sync byte. 00329 * 00330 * @remark Applies to LoRa modem only 00331 * 00332 * @param [IN] enable if true, it enables a public network 00333 */ 00334 virtual void SetPublicNetwork( bool enable ) = 0; 00335 }; 00336 00337 #endif // __RADIO_H__
Generated on Tue Jul 12 2022 20:53:59 by
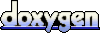