SX1261 and sx1262 common library
Dependents: SX126xDevKit SX1262PingPong SX126X_TXonly SX126X_PingPong_Demo ... more
Fork of SX126xLib by
radio.h
00001 /* 00002 ______ _ 00003 / _____) _ | | 00004 ( (____ _____ ____ _| |_ _____ ____| |__ 00005 \____ \| ___ | (_ _) ___ |/ ___) _ \ 00006 _____) ) ____| | | || |_| ____( (___| | | | 00007 (______/|_____)_|_|_| \__)_____)\____)_| |_| 00008 (C)2016 Semtech 00009 00010 Description: Handling of the node configuration protocol 00011 00012 License: Revised BSD License, see LICENSE.TXT file include in the project 00013 00014 Maintainer: Miguel Luis, Gregory Cristian and Matthieu Verdy 00015 */ 00016 #ifndef __RADIO_H__ 00017 #define __RADIO_H__ 00018 00019 #include "mbed.h" 00020 00021 /*! 00022 * \brief Structure describing the radio status 00023 */ 00024 typedef union RadioStatus_u 00025 { 00026 uint8_t Value; 00027 struct 00028 { //bit order is lsb -> msb 00029 uint8_t Reserved : 1; //!< Reserved 00030 uint8_t CmdStatus : 3; //!< Command status 00031 uint8_t ChipMode : 3; //!< Chip mode 00032 uint8_t CpuBusy : 1; //!< Flag for CPU radio busy 00033 }Fields; 00034 }RadioStatus_t; 00035 00036 /*! 00037 * \brief Structure describing the error codes for callback functions 00038 */ 00039 typedef enum 00040 { 00041 IRQ_HEADER_ERROR_CODE = 0x01, 00042 IRQ_SYNCWORD_ERROR_CODE = 0x02, 00043 IRQ_CRC_ERROR_CODE = 0x04, 00044 }IrqErrorCode_t; 00045 00046 00047 enum IrqPblSyncHeaderCode_t 00048 { 00049 IRQ_PBL_DETECT_CODE = 0x01, 00050 IRQ_SYNCWORD_VALID_CODE = 0x02, 00051 IRQ_HEADER_VALID_CODE = 0x04, 00052 }; 00053 00054 enum IrqTimeoutCode_t 00055 { 00056 IRQ_RX_TIMEOUT = 0x00, 00057 IRQ_TX_TIMEOUT = 0x01, 00058 IRQ_XYZ = 0xFF, 00059 }; 00060 00061 /*! 00062 * \brief The radio command opcodes 00063 */ 00064 typedef enum RadioCommands_e RadioCommands_t; 00065 00066 /*! 00067 * \brief The radio callbacks structure 00068 * Holds function pointers to be called on radio interrupts 00069 */ 00070 typedef struct 00071 { 00072 void ( *txDone )( void ); //!< Pointer to a function run on successful transmission 00073 void ( *rxDone )( void ); //!< Pointer to a function run on successful reception 00074 void ( *rxPreambleDetect )( void ); //!< Pointer to a function run on successful Preamble detection 00075 void ( *rxSyncWordDone )( void ); //!< Pointer to a function run on successful SyncWord reception 00076 void ( *rxHeaderDone )( void ); //!< Pointer to a function run on successful Header reception 00077 void ( *txTimeout )( void ); //!< Pointer to a function run on transmission timeout 00078 void ( *rxTimeout )( void ); //!< Pointer to a function run on reception timeout 00079 void ( *rxError )( IrqErrorCode_t errCode ); //!< Pointer to a function run on reception error 00080 void ( *cadDone )( bool cadFlag ); //!< Pointer to a function run on channel activity detected 00081 }RadioCallbacks_t; 00082 00083 /*! 00084 * \brief Class holding the basic communications with a radio 00085 * 00086 * It sets the functions to read/write registers, send commands and read/write 00087 * payload. 00088 * It also provides functions to run callback functions depending on the 00089 * interrupts generated from the radio. 00090 */ 00091 class Radio 00092 { 00093 protected: 00094 /*! 00095 * \brief Callback on Tx done interrupt 00096 */ 00097 void ( *txDone )( void ); 00098 00099 /*! 00100 * \brief Callback on Rx done interrupt 00101 */ 00102 void ( *rxDone )( void ); 00103 00104 /*! 00105 * \brief Callback on Rx preamble detection interrupt 00106 */ 00107 void ( *rxPreambleDetect )( void ); 00108 00109 /*! 00110 * \brief Callback on Rx SyncWord interrupt 00111 */ 00112 void ( *rxSyncWordDone )( void ); 00113 00114 /*! 00115 * \brief Callback on Rx header received interrupt 00116 */ 00117 void ( *rxHeaderDone )( void ); 00118 00119 /*! 00120 * \brief Callback on Tx timeout interrupt 00121 */ 00122 void ( *txTimeout )( void ); 00123 00124 /*! 00125 * \brief Callback on Rx timeout interrupt 00126 */ 00127 void ( *rxTimeout )( void ); 00128 00129 /*! 00130 * \brief Callback on Rx error interrupt 00131 * 00132 * \param [out] errCode A code indicating the type of interrupt (SyncWord error or CRC error) 00133 */ 00134 void ( *rxError )( IrqErrorCode_t errCode ); 00135 00136 /*! 00137 * \brief Callback on Channel Activity Detection done interrupt 00138 * 00139 * \param [out] cadFlag Flag for channel activity detected or not 00140 */ 00141 void ( *cadDone )( bool cadFlag ); 00142 00143 public: 00144 /*! 00145 * \brief Constructor for radio class 00146 * Sets the callbacks functions pointers 00147 * 00148 * \param [in] callbacks The structure of callbacks function pointers 00149 * to be called on radio interrupts 00150 * 00151 */ 00152 Radio( RadioCallbacks_t *callbacks ) 00153 { 00154 this->txDone = callbacks->txDone; 00155 this->rxDone = callbacks->rxDone; 00156 this->rxPreambleDetect = callbacks->rxPreambleDetect; 00157 this->rxSyncWordDone = callbacks->rxSyncWordDone; 00158 this->rxHeaderDone = callbacks->rxHeaderDone; 00159 this->txTimeout = callbacks->txTimeout; 00160 this->rxTimeout = callbacks->rxTimeout; 00161 this->rxError = callbacks->rxError; 00162 this->cadDone = callbacks->cadDone; 00163 } 00164 virtual ~Radio( void ){ }; 00165 00166 /*! 00167 * \brief Resets the radio 00168 */ 00169 virtual void Reset( void ) = 0; 00170 00171 /*! 00172 * \brief Gets the current radio status 00173 * 00174 * \retval status Radio status 00175 */ 00176 virtual RadioStatus_t GetStatus( void ) = 0; 00177 00178 /*! 00179 * \brief Writes the given command to the radio 00180 * 00181 * \param [in] opcode Command opcode 00182 * \param [in] buffer Command parameters byte array 00183 * \param [in] size Command parameters byte array size 00184 */ 00185 virtual void WriteCommand( RadioCommands_t opcode, uint8_t *buffer, uint16_t size ) = 0; 00186 00187 /*! 00188 * \brief Reads the given command from the radio 00189 * 00190 * \param [in] opcode Command opcode 00191 * \param [in] buffer Command parameters byte array 00192 * \param [in] size Command parameters byte array size 00193 */ 00194 virtual void ReadCommand( RadioCommands_t opcode, uint8_t *buffer, uint16_t size ) = 0; 00195 00196 /*! 00197 * \brief Writes multiple radio registers starting at address 00198 * 00199 * \param [in] address First Radio register address 00200 * \param [in] buffer Buffer containing the new register's values 00201 * \param [in] size Number of registers to be written 00202 */ 00203 virtual void WriteRegister( uint16_t address, uint8_t *buffer, uint16_t size ) = 0; 00204 00205 /*! 00206 * \brief Writes the radio register at the specified address 00207 * 00208 * \param [in] address Register address 00209 * \param [in] value New register value 00210 */ 00211 virtual void WriteReg( uint16_t address, uint8_t value ) = 0; 00212 00213 /*! 00214 * \brief Reads multiple radio registers starting at address 00215 * 00216 * \param [in] address First Radio register address 00217 * \param [out] buffer Buffer where to copy the registers data 00218 * \param [in] size Number of registers to be read 00219 */ 00220 virtual void ReadRegister( uint16_t address, uint8_t *buffer, uint16_t size ) = 0; 00221 00222 /*! 00223 * \brief Reads the radio register at the specified address 00224 * 00225 * \param [in] address Register address 00226 * 00227 * \retval value The register value 00228 */ 00229 virtual uint8_t ReadReg( uint16_t address ) = 0; 00230 00231 /*! 00232 * \brief Writes Radio Data Buffer with buffer of size starting at offset. 00233 * 00234 * \param [in] offset Offset where to start writing 00235 * \param [in] buffer Buffer pointer 00236 * \param [in] size Buffer size 00237 */ 00238 virtual void WriteBuffer( uint8_t offset, uint8_t *buffer, uint8_t size ) = 0; 00239 00240 /*! 00241 * \brief Reads Radio Data Buffer at offset to buffer of size 00242 * 00243 * \param [in] offset Offset where to start reading 00244 * \param [out] buffer Buffer pointer 00245 * \param [in] size Buffer size 00246 */ 00247 virtual void ReadBuffer( uint8_t offset, uint8_t *buffer, uint8_t size ) = 0; 00248 }; 00249 00250 #endif // __RADIO_H__ 00251
Generated on Tue Jul 12 2022 15:50:43 by
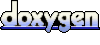