
Source code for the SX126xDVK1xAS Dev Kit. This example code has only been tested on the Nucleo L476RG
Dependencies: mbed DmTftLibrary SX126xLib
DisplayDriver.h
00001 /* 00002 ______ _ 00003 / _____) _ | | 00004 ( (____ _____ ____ _| |_ _____ ____| |__ 00005 \____ \| ___ | (_ _) ___ |/ ___) _ \ 00006 _____) ) ____| | | || |_| ____( (___| | | | 00007 (______/|_____)_|_|_| \__)_____)\____)_| |_| 00008 (C)2016 Semtech 00009 00010 Description: Display driver header 00011 00012 Maintainer: Gregory Cristian & Gilbert Menth 00013 */ 00014 00015 #ifndef TFT_DISPLAY_DRIVER_H 00016 #define TFT_DISPLAY_DRIVER_H 00017 00018 00019 #include "DmTftBase.h" 00020 00021 00022 #define SCREEN_WIDTH 240 00023 #define SCREEN_HEIGHT 320 00024 00025 #define TEXT_COLOR WHITE 00026 #define BACK_COLOR BLACK 00027 #define PAGE_COLOR CYAN 00028 #define OBJECT_ERROR RED 00029 #define BUTTON_BORDER BLUE 00030 #define MENU_TEXT GREEN 00031 #define TEXT_VALUE YELLOW 00032 #define CIRCLE_FCOLOR GRAY1 00033 00034 00035 typedef enum 00036 { 00037 GO_STATUS_NOERR, 00038 GO_STATUS_BAD_COORD, 00039 GO_STATUS_BAD_ARG, 00040 GO_STATUS_BAD_CONTEXT, 00041 }GraphObjectStatus_t; 00042 00043 typedef enum 00044 { 00045 GO_TEXT, 00046 GO_RECTANGLE, 00047 GO_CIRCLE, 00048 GO_TRIANGLE, 00049 GO_IMAGE, 00050 GO_LINE, 00051 }GraphObjectType_t; 00052 00053 typedef struct 00054 { 00055 uint8_t Id; 00056 GraphObjectType_t Type; 00057 uint16_t Xpos; 00058 uint16_t Ypos; 00059 uint16_t Height; 00060 uint16_t Width; 00061 uint16_t LineWidth; 00062 uint16_t BackColor; 00063 uint16_t FrontColor; 00064 bool DoFill; 00065 uint16_t FillColor; 00066 uint8_t* Source; 00067 bool TouchActive; 00068 }GraphObject_t; 00069 00070 00071 /*! 00072 * \brief Initialses the hardware and variables associated with the display. 00073 */ 00074 void DisplayDriverInit( void ); 00075 00076 /*! 00077 * \brief Calibrates the touch screen. 00078 */ 00079 void DisplayDriverCalibrate( void ); 00080 00081 /*! 00082 * \brief Draws a graphical object. 00083 * 00084 * \param [in] *goObject Object to draw. 00085 * \param [in] *source If object is a text : *source is the text to print 00086 * If object is a image: *source is the image 00087 * For the other type of goObject, *source is ignored 00088 * \param [in] doFill Indicate if the goObject (only for GO_RECTANGLE, 00089 * GO_CIRCLE & GO_TRIANGLE) should be filled or not. 00090 * \param [in] activeTouch Indicate if the coordinates of the goObject can be 00091 * used for touchscreen or not. 00092 * \retval status GO_STATUS_NOERR if ok or, 00093 * GO_STATUS_BAD_COORD if the object go out of screen 00094 */ 00095 GraphObjectStatus_t GraphObjectDraw( GraphObject_t* goObject, uint8_t* source, \ 00096 bool doFill, bool activeTouch); 00097 00098 /*! 00099 * \brief Clear a graphical object. 00100 * 00101 * \param [in] *goObject Object to clear. 00102 * \param [in] doFill Indicate if the goObject (only for GO_RECTANGLE, 00103 * GO_CIRCLE & GO_TRIANGLE) should be filled or not. 00104 * \retval status GO_STATUS_NOERR if ok or, 00105 * GO_STATUS_BAD_COORD if the object go out of screen 00106 */ 00107 GraphObjectStatus_t GraphObjectClear( GraphObject_t* goObject, bool doFill ); 00108 00109 /*! 00110 * \brief Get the first object that have the touched coordinate (if activateTouch 00111 * of the object is TRUE). 00112 * 00113 * \param [in] *objects Tab of grophical objects. 00114 * \param [in] objectsCount Indicate the numbre of goObject in the tab. 00115 * \param [out] touchedObject Put the ID of the first touched and activated 00116 * object of the tab. 00117 * \retval status GO_STATUS_NOERR if ok. 00118 */ 00119 GraphObjectStatus_t GraphObjectTouched( GraphObject_t* objects, \ 00120 uint8_t objectsCount, \ 00121 uint8_t* touchedObject); 00122 00123 /*! 00124 * \brief Draws a logo on the display. 00125 * 00126 * \param [in] *thisBmp The file to be printed onto the display. 00127 * \param [in] xPos Position across the display in pixels for the top 00128 * right corner of the logo starting at the left edge 00129 * of display. 00130 * \param [in] yPos Position across the display in pixels for the top 00131 * right corner of the logo starting at the top of 00132 * the display. 00133 */ 00134 void DisplayDriverDrawLogo( uint8_t *thisBmp, uint8_t xPos, uint8_t yPos ); 00135 00136 #endif //TFT_DISPLAY_DRIVER_H
Generated on Tue Jul 12 2022 15:49:22 by
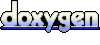