
Source code for the SX126xDVK1xAS Dev Kit. This example code has only been tested on the Nucleo L476RG
Dependencies: mbed DmTftLibrary SX126xLib
DemoApplication.h
00001 /* 00002 ______ _ 00003 / _____) _ | | 00004 ( (____ _____ ____ _| |_ _____ ____| |__ 00005 \____ \| ___ | (_ _) ___ |/ ___) _ \ 00006 _____) ) ____| | | || |_| ____( (___| | | | 00007 (______/|_____)_|_|_| \__)_____)\____)_| |_| 00008 (C)2016 Semtech 00009 00010 Description: Display driver header 00011 00012 Maintainer: Gregory Cristian & Gilbert Menth 00013 */ 00014 00015 #ifndef DEMO_APPLICATION_H 00016 #define DEMO_APPLICATION_H 00017 00018 00019 /*! 00020 * \brief Used to display firmware version on TFT (Utilities menu) 00021 */ 00022 #define FIRMWARE_VERSION ( ( char* )"Firmware Version: 180706" ) 00023 00024 /*! 00025 * \brief Define range of central frequency [Hz] 00026 */ 00027 #define DEMO_CENTRAL_FREQ_MIN 150000000UL 00028 #define DEMO_CENTRAL_FREQ_MAX 950000000UL 00029 00030 /*! 00031 * \brief Define 3 preset central frequencies [Hz] 00032 */ 00033 #define DEMO_CENTRAL_FREQ_PRESET1 169000000UL 00034 #define DEMO_CENTRAL_FREQ_PRESET2 280000000UL 00035 #define DEMO_CENTRAL_FREQ_PRESET3 434000000UL 00036 #define DEMO_CENTRAL_FREQ_PRESET4 490000000UL 00037 #define DEMO_CENTRAL_FREQ_PRESET5 783000000UL 00038 #define DEMO_CENTRAL_FREQ_PRESET6 868000000UL 00039 #define DEMO_CENTRAL_FREQ_PRESET7 915000000UL 00040 #define DEMO_CENTRAL_FREQ_PRESET8 930000000UL 00041 #define DEMO_CENTRAL_FREQ_PRESET9 510000000UL 00042 00043 /*! 00044 * \brief Define min and max Tx power [dBm] 00045 */ 00046 #define SX1261_POWER_TX_MIN -17 00047 #define SX1261_POWER_TX_MAX 15 00048 00049 #define SX1262_POWER_TX_MIN -10 00050 #define SX1262_POWER_TX_MAX 22 00051 00052 /*! 00053 * \brief Define current demo mode 00054 */ 00055 enum DemoMode 00056 { 00057 MASTER = 0, 00058 SLAVE 00059 }; 00060 00061 /*! 00062 * \brief Define GFSK bitrate 00063 */ 00064 typedef enum 00065 { 00066 DEMO_BR_100 = 100, 00067 DEMO_BR_600 = 600, 00068 DEMO_BR_4800 = 4800, 00069 DEMO_BR_9600 = 9600, 00070 DEMO_BR_19200 = 19200, 00071 DEMO_BR_57600 = 57600, 00072 DEMO_BR_100000 = 100000, 00073 DEMO_BR_250000 = 250000, 00074 }DemoBitrate_t; 00075 00076 /*! 00077 * \brief Define GFSK frequency deviation 00078 */ 00079 typedef enum 00080 { 00081 DEMO_FDEV_5000 = 5000, 00082 DEMO_FDEV_10000 = 10000, 00083 DEMO_FDEV_25000 = 25000, 00084 DEMO_FDEV_50000 = 50000, 00085 DEMO_FDEV_75000 = 75000, 00086 DEMO_FDEV_100000 = 100000, 00087 DEMO_FDEV_150000 = 150000, 00088 }DemoFrequencyDev_t; 00089 00090 /*! 00091 * \brief List of states for demo state machine 00092 */ 00093 enum DemoInternalStates 00094 { 00095 APP_IDLE = 0, // nothing to do (or wait a radio interrupt) 00096 SEND_PING_MSG, 00097 SEND_PONG_MSG, 00098 APP_RX, // Rx done 00099 APP_RX_TIMEOUT, // Rx timeout 00100 APP_RX_ERROR, // Rx error 00101 APP_TX, // Tx done 00102 APP_TX_TIMEOUT, // Tx error 00103 PER_TX_START, // PER master 00104 PER_RX_START, // PER slave 00105 CAD_DONE, // CAD Done 00106 CAD_DONE_CHANNEL_DETECTED // Channel Detected following a CAD 00107 }; 00108 00109 /*! 00110 * \brief Demo Settings structure of Eeprom structure 00111 */ 00112 typedef struct 00113 { 00114 uint8_t Entity; // Master or Slave 00115 uint8_t HoldDemo; // Put demo in hold status 00116 uint8_t BoostedRx; // Use Boosted Rx if true 00117 uint32_t Frequency; // Demo frequency 00118 uint8_t LastDeviceConnected;// Last Device Connected 00119 int8_t TxPower; // Demo Tx power 00120 uint8_t RadioPowerMode; // Radio Power Mode [0: LDO, 1:DC_DC] 00121 uint8_t PayloadLength; // Demo payload length 00122 uint8_t ModulationType; // Demo modulation type (LORA, GFSK) 00123 uint32_t ModulationParam1; // Demo Mod. Param1 (depend on modulation type) 00124 uint32_t ModulationParam2; // Demo Mod. Param2 (depend on modulation type) 00125 uint8_t ModulationParam3; // Demo Mod. Param3 (depend on modulation type) 00126 uint8_t ModulationParam4; // Demo Mod. Param4 (depend on modulation type) 00127 uint16_t PacketParam1; // Demo Pack. Param1 (depend on packet type) 00128 uint8_t PacketParam2; // Demo Pack. Param2 (depend on packet type) 00129 uint8_t PacketParam3; // Demo Pack. Param3 (depend on packet type) 00130 uint8_t PacketParam4; // Demo Pack. Param4 (depend on packet type) 00131 uint8_t PacketParam5; // Demo Pack. Param5 (depend on packet type) 00132 uint8_t PacketParam6; // Demo Pack. Param6 (depend on packet type) 00133 uint8_t PacketParam7; // Demo Pack. Param7 (depend on packet type) 00134 uint8_t PacketParam8; // Demo Pack. Param8 (depend on packet type) 00135 uint32_t MaxNumPacket; // Demo Max Num Packet for PingPong and PER 00136 uint16_t InterPacketDelay; // Demo Inter-Packet Delay for PingPong and PER 00137 uint32_t CntPacketTx; // Tx packet transmitted 00138 uint32_t CntPacketRxOK; // Rx packet received OK 00139 uint32_t CntPacketRxOKSlave;// Rx packet received OK (slave side) 00140 uint32_t CntPacketRxKO; // Rx packet received KO 00141 uint32_t CntPacketRxKOSlave;// Rx packet received KO (slave side) 00142 uint16_t RxTimeOutCount; // Rx packet received KO (by timeout) 00143 int8_t RssiValue; // Demo Rssi Value 00144 int8_t SnrValue; // Demo Snr Value (only for LR24 mod. type) 00145 uint32_t FreqErrorEst; // Estimation of the frequency error on the Rx side 00146 }DemoSettings_t; 00147 00148 /*! 00149 * \brief Define freq offset for config central freq in "Radio Config Freq" menu 00150 */ 00151 enum FreqBase 00152 { 00153 FB1 = 1, // 1 Hz 00154 FB10 = 10, // 10 Hz 00155 FB100 = 100, // 100 Hz 00156 FB1K = 1000, // 1 kHz 00157 FB10K = 10000, // 10 kHz 00158 FB100K = 100000, // 100 kHz 00159 FB1M = 1000000, // 1 MHz 00160 FB10M = 10000000 // 10 MHz 00161 }; 00162 00163 00164 /*! 00165 * \brief Simple Function which return the device connected. 00166 * 00167 * \retval deviceConnected device type connected 00168 */ 00169 uint8_t GetConnectedDevice( void ); 00170 00171 /*! 00172 * \brief Simple Function which return the board matching frequency 00173 * 00174 * \retval freq 1: 868 MHz 0: 915 MHz 00175 */ 00176 uint8_t GetMatchingFrequency( void ); 00177 00178 /*! 00179 * \brief Init RAM copy of Eeprom structure and init radio with it. 00180 * 00181 */ 00182 void InitDemoApplication( void ); 00183 00184 /*! 00185 * \brief Init vars of demo and fix APP_IDLE state to demo state machine. 00186 */ 00187 void StopDemoApplication( void ); 00188 00189 /*! 00190 * \brief Run demo reading constantly the RSSI. 00191 * 00192 * \retval demoStatusUpdate page refresh status ( >0 : refresh) 00193 */ 00194 uint8_t RunDemoTestRssi( void ); 00195 00196 /*! 00197 * \brief Run Demo in sleep mode. 00198 * 00199 * \retval demoStatusUpdate page refresh status ( >0 : refresh) 00200 */ 00201 uint8_t RunDemoSleepMode( void ); 00202 00203 /*! 00204 * \brief Run Demo in standby RC mode. 00205 * 00206 * \retval demoStatusUpdate page refresh status ( >0 : refresh) 00207 */ 00208 uint8_t RunDemoStandbyRcMode( void ); 00209 00210 /*! 00211 * \brief Run Demo in standby XOSC mode. 00212 * 00213 * \retval demoStatusUpdate page refresh status ( >0 : refresh) 00214 */ 00215 uint8_t RunDemoStandbyXoscMode( void ); 00216 00217 /*! 00218 * \brief Run Demo Tx in continuous mode without modulation. 00219 * 00220 * \retval demoStatusUpdate page refresh status ( >0 : refresh) 00221 */ 00222 uint8_t RunDemoTxCw( void ); 00223 00224 /*! 00225 * \brief Run Demo Tx in continuous modulation. 00226 * 00227 * \retval demoStatusUpdate page refresh status ( >0 : refresh) 00228 */ 00229 uint8_t RunDemoTxContinuousModulation( void ); 00230 00231 /*! 00232 * \brief Run Demo Rx in continuous mode. 00233 * 00234 * \retval demoStatusUpdate page refresh status ( >0 : refresh) 00235 */ 00236 uint8_t RunDemoRxContinuous( void ); 00237 00238 /*! 00239 * \brief Run demo PingPong. 00240 * 00241 * \retval demoStatusUpdate page refresh status ( >0 : refresh) 00242 */ 00243 uint8_t RunDemoApplicationPingPong( void ); 00244 00245 /*! 00246 * \brief Compute payload of Rx frame and update current counts and indicators. 00247 * 00248 * \param [in] buffer buffer with frame to compute 00249 * \param [in] buffersize size of frame data in the buffer 00250 */ 00251 void ComputePingPongPayload( uint8_t *buffer, uint8_t bufferSize ); 00252 00253 /*! 00254 * \brief Run demo PER. 00255 * 00256 * \retval demoStatusUpdate page refresh status ( >0 : refresh) 00257 */ 00258 uint8_t RunDemoApplicationPer( void ); 00259 00260 /*! 00261 * \brief Compute payload of Rx frame and update current counts and indicators. 00262 * 00263 * \param [in] buffer buffer with frame to compute 00264 * \param [in] buffersize size of frame data in the buffer 00265 */ 00266 void ComputePerPayload( uint8_t *buffer, uint8_t bufferSize ); 00267 00268 #endif // DEMO_APPLICATION_H
Generated on Tue Jul 12 2022 15:49:22 by
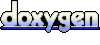