LoRaWAN MAC layer implementation
Dependents: LoRaWAN-demo-72_tjm LoRaWAN-demo-72_jlc LoRaWAN-demo-elmo frdm_LoRa_Connect_Woodstream_Demo_tjm ... more
LoRa MAC layer cryptography implementation
Functions | |
void | LoRaMacComputeMic (const uint8_t *buffer, uint16_t size, const uint8_t *key, uint32_t address, uint8_t dir, uint32_t sequenceCounter, uint32_t *mic) |
Computes the LoRaMAC frame MIC field. | |
void | LoRaMacPayloadEncrypt (const uint8_t *buffer, uint16_t size, const uint8_t *key, uint32_t address, uint8_t dir, uint32_t sequenceCounter, uint8_t *encBuffer) |
void | LoRaMacPayloadDecrypt (const uint8_t *buffer, uint16_t size, const uint8_t *key, uint32_t address, uint8_t dir, uint32_t sequenceCounter, uint8_t *decBuffer) |
void | LoRaMacJoinComputeMic (const uint8_t *buffer, uint16_t size, const uint8_t *key, uint32_t *mic) |
void | LoRaMacJoinDecrypt (const uint8_t *buffer, uint16_t size, const uint8_t *key, uint8_t *decBuffer) |
void | LoRaMacJoinComputeSKeys (const uint8_t *key, const uint8_t *appNonce, uint16_t devNonce, uint8_t *nwkSKey, uint8_t *appSKey) |
Detailed Description
This module covers the implementation of cryptographic functions of the LoRaMAC layer.
Function Documentation
void LoRaMacComputeMic | ( | const uint8_t * | buffer, |
uint16_t | size, | ||
const uint8_t * | key, | ||
uint32_t | address, | ||
uint8_t | dir, | ||
uint32_t | sequenceCounter, | ||
uint32_t * | mic | ||
) |
Computes the LoRaMAC frame MIC field.
Computes the LoRaMAC frame MIC field
- Parameters:
-
[IN] buffer - Data buffer [IN] size - Data buffer size [IN] key - AES key to be used [IN] address - Frame address [IN] dir - Frame direction [0: uplink, 1: downlink] [IN] sequenceCounter - Frame sequence counter [OUT] mic - Computed MIC field [IN] buffer Data buffer [IN] size Data buffer size [IN] key AES key to be used [IN] address Frame address [IN] dir Frame direction [0: uplink, 1: downlink] [IN] sequenceCounter Frame sequence counter [OUT] mic Computed MIC field
Definition at line 79 of file LoRaMacCrypto.cpp.
void LoRaMacJoinComputeMic | ( | const uint8_t * | buffer, |
uint16_t | size, | ||
const uint8_t * | key, | ||
uint32_t * | mic | ||
) |
Computes the LoRaMAC Join Request frame MIC field
- Parameters:
-
[IN] buffer - Data buffer [IN] size - Data buffer size [IN] key - AES key to be used [OUT] mic - Computed MIC field
Definition at line 158 of file LoRaMacCrypto.cpp.
void LoRaMacJoinComputeSKeys | ( | const uint8_t * | key, |
const uint8_t * | appNonce, | ||
uint16_t | devNonce, | ||
uint8_t * | nwkSKey, | ||
uint8_t * | appSKey | ||
) |
Computes the LoRaMAC join frame decryption
- Parameters:
-
[IN] key - AES key to be used [IN] appNonce - Application nonce [IN] devNonce - Device nonce [OUT] nwkSKey - Network session key [OUT] appSKey - Application session key
Definition at line 183 of file LoRaMacCrypto.cpp.
void LoRaMacJoinDecrypt | ( | const uint8_t * | buffer, |
uint16_t | size, | ||
const uint8_t * | key, | ||
uint8_t * | decBuffer | ||
) |
Computes the LoRaMAC join frame decryption
- Parameters:
-
[IN] buffer - Data buffer [IN] size - Data buffer size [IN] key - AES key to be used [OUT] decBuffer - Decrypted buffer
Definition at line 171 of file LoRaMacCrypto.cpp.
void LoRaMacPayloadDecrypt | ( | const uint8_t * | buffer, |
uint16_t | size, | ||
const uint8_t * | key, | ||
uint32_t | address, | ||
uint8_t | dir, | ||
uint32_t | sequenceCounter, | ||
uint8_t * | decBuffer | ||
) |
Computes the LoRaMAC payload decryption
- Parameters:
-
[IN] buffer - Data buffer [IN] size - Data buffer size [IN] key - AES key to be used [IN] address - Frame address [IN] dir - Frame direction [0: uplink, 1: downlink] [IN] sequenceCounter - Frame sequence counter [OUT] decBuffer - Decrypted buffer
Definition at line 153 of file LoRaMacCrypto.cpp.
void LoRaMacPayloadEncrypt | ( | const uint8_t * | buffer, |
uint16_t | size, | ||
const uint8_t * | key, | ||
uint32_t | address, | ||
uint8_t | dir, | ||
uint32_t | sequenceCounter, | ||
uint8_t * | encBuffer | ||
) |
Computes the LoRaMAC payload encryption
- Parameters:
-
[IN] buffer - Data buffer [IN] size - Data buffer size [IN] key - AES key to be used [IN] address - Frame address [IN] dir - Frame direction [0: uplink, 1: downlink] [IN] sequenceCounter - Frame sequence counter [OUT] encBuffer - Encrypted buffer
Definition at line 108 of file LoRaMacCrypto.cpp.
Generated on Tue Jul 12 2022 14:27:12 by
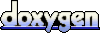