Demonstration of Class-A LoRaWAN device using NAMote-72
Dependencies: LoRaWAN-lib mbed lib_mpl3115a2 lib_mma8451q lib_gps SX1272Lib
Dependents: LoRaWAN-NAMote72-BVS-confirmed-tester-0-7v1_copy
board.cpp
00001 /* 00002 / _____) _ | | 00003 ( (____ _____ ____ _| |_ _____ ____| |__ 00004 \____ \| ___ | (_ _) ___ |/ ___) _ \ 00005 _____) ) ____| | | || |_| ____( (___| | | | 00006 (______/|_____)_|_|_| \__)_____)\____)_| |_| 00007 (C)2015 Semtech 00008 00009 Description: Target board general functions implementation 00010 00011 License: Revised BSD License, see LICENSE.TXT file include in the project 00012 00013 Maintainer: Miguel Luis and Gregory Cristian 00014 */ 00015 #include "mbed.h" 00016 #include "board.h" 00017 00018 /*! 00019 * Unique Devices IDs register set ( STM32 ) 00020 */ 00021 #define ID1 ( 0x1FF800D0 ) 00022 #define ID2 ( 0x1FF800D4 ) 00023 #define ID3 ( 0x1FF800E4 ) 00024 00025 DigitalOut RedLed( PB_1 ); // Active Low 00026 DigitalOut YellowLed( PB_10 ); // Active Low 00027 DigitalOut GreenLed( PC_3 ); // Active Low 00028 DigitalOut UsrLed( PA_5 ); // Active High 00029 00030 00031 GPS Gps( PB_6, PB_7, PB_11 ); // Gps(tx, rx, en); 00032 00033 DigitalIn I2cInterrupt( PB_4 ); 00034 I2C I2c(I2C_SDA, I2C_SCL); 00035 00036 MPL3115A2 Mpl3115a2( I2c, I2cInterrupt ); 00037 MMA8451Q Mma8451q(I2c, I2cInterrupt); 00038 00039 DigitalOut Pc7( PC_7 ); 00040 DigitalIn Pc1( PC_1 ); 00041 00042 // Used for Push button application demo 00043 DigitalIn PC0( PC_0, PullUp ); 00044 00045 AnalogIn *Battery; 00046 00047 #define AIN_VREF 3300 // STM32 internal refernce 00048 #define AIN_VBAT_DIV 2 // Resistor divider 00049 00050 SX1272MB2xAS Radio( NULL ); 00051 00052 /*! 00053 * Nested interrupt counter. 00054 * 00055 * \remark Interrupt should only be fully disabled once the value is 0 00056 */ 00057 static uint8_t IrqNestLevel = 0; 00058 00059 void BoardDisableIrq( void ) 00060 { 00061 __disable_irq( ); 00062 IrqNestLevel++; 00063 } 00064 00065 void BoardEnableIrq( void ) 00066 { 00067 IrqNestLevel--; 00068 if( IrqNestLevel == 0 ) 00069 { 00070 __enable_irq( ); 00071 } 00072 } 00073 00074 void BoardInit( void ) 00075 { 00076 // Initalize LEDs 00077 RedLed = 1; // Active Low 00078 GreenLed = 1; // Active Low 00079 YellowLed = 1; // Active Low 00080 UsrLed = 0; // Active High 00081 00082 TimerTimeCounterInit( ); 00083 00084 switch( BoardGetVersion( ) ) 00085 { 00086 case BOARD_VERSION_2: 00087 Battery = new AnalogIn( PA_0 ); 00088 Gps.en_invert = true; 00089 break; 00090 case BOARD_VERSION_3: 00091 Battery = new AnalogIn( PA_1 ); 00092 Gps.en_invert = false; 00093 break; 00094 default: 00095 break; 00096 } 00097 Gps.init( ); 00098 Gps.enable( 1 ); 00099 00100 Mpl3115a2.init( ); 00101 Mma8451q.orient_detect( ); 00102 } 00103 00104 00105 uint8_t BoardGetBatteryLevel( void ) 00106 { 00107 // Per LoRaWAN spec; 0 = Charging; 1...254 = level, 255 = N/A 00108 return ( Battery->read_u16( ) >> 8 ) + ( Battery->read_u16( ) >> 9 ); 00109 } 00110 00111 uint32_t BoardGetBatteryVoltage( void ) 00112 { 00113 return ( Battery->read( ) * AIN_VREF * AIN_VBAT_DIV ); 00114 } 00115 00116 uint32_t BoardGetRandomSeed( void ) 00117 { 00118 return ( ( *( uint32_t* )ID1 ) ^ ( *( uint32_t* )ID2 ) ^ ( *( uint32_t* )ID3 ) ); 00119 } 00120 00121 void BoardGetDevEUI( uint8_t *id ) 00122 { 00123 uint32_t *pDevEuiHWord = ( uint32_t* )&id[4]; 00124 00125 if( *pDevEuiHWord == 0 ) 00126 { 00127 *pDevEuiHWord = BoardGetRandomSeed( ); 00128 } 00129 00130 } 00131 00132 void BoardGetUniqueId( uint8_t *id ) 00133 { 00134 id[7] = ( ( *( uint32_t* )ID1 )+ ( *( uint32_t* )ID3 ) ) >> 24; 00135 id[6] = ( ( *( uint32_t* )ID1 )+ ( *( uint32_t* )ID3 ) ) >> 16; 00136 id[5] = ( ( *( uint32_t* )ID1 )+ ( *( uint32_t* )ID3 ) ) >> 8; 00137 id[4] = ( ( *( uint32_t* )ID1 )+ ( *( uint32_t* )ID3 ) ); 00138 id[3] = ( ( *( uint32_t* )ID2 ) ) >> 24; 00139 id[2] = ( ( *( uint32_t* )ID2 ) ) >> 16; 00140 id[1] = ( ( *( uint32_t* )ID2 ) ) >> 8; 00141 id[0] = ( ( *( uint32_t* )ID2 ) ); 00142 } 00143 00144 BoardVersion_t BoardGetVersion( void ) 00145 { 00146 Pc7 = 1; 00147 char first = Pc1; 00148 Pc7 = 0; 00149 00150 if( first && !Pc1 ) 00151 { 00152 return BOARD_VERSION_2; 00153 } 00154 else 00155 { 00156 return BOARD_VERSION_3; 00157 } 00158 }
Generated on Fri Jul 15 2022 22:53:35 by
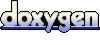