new Xadow GPS module
Dependents: xadow_smartstrap_for_pebble Avnet_ATT_Cellular_IOT Xadow-M0_Xadow-OLED_Accelerometer
XadowGPS.cpp
00001 00002 #include <stdlib.h> 00003 #include "mbed.h" 00004 #include "XadowGPS.h" 00005 #include "USBSerial.h" 00006 00007 00008 00009 unsigned char gps_utc_date_time[GPS_UTC_DATE_TIME_SIZE] = {0}; 00010 static char cmd[2]; 00011 00012 extern I2C i2c; 00013 extern USBSerial dbg_serial; 00014 00015 00016 unsigned char gps_check_online(void) 00017 { 00018 unsigned char data[GPS_SCAN_SIZE+2]; 00019 unsigned char i; 00020 00021 //dlc_i2c_configure(GPS_DEVICE_ADDR, 100); 00022 //dlc_i2c_send_byte(GPS_SCAN_ID); 00023 cmd[0] = GPS_SCAN_ID; 00024 i2c.write(GPS_DEVICE_ADDR, cmd, 1); 00025 00026 for(i=0;i<(GPS_SCAN_SIZE+2);i++) 00027 { 00028 i2c.read(GPS_DEVICE_ADDR, (char *)&data[i], 1); //the gps module's i2c supports only 1 byte read/write per burst 00029 } 00030 00031 if(data[5] == (GPS_DEVICE_ADDR>>1))return 1; 00032 else return 0; 00033 } 00034 00035 unsigned char* gps_get_utc_date_time(void) 00036 { 00037 unsigned char data[GPS_UTC_DATE_TIME_SIZE+2]; 00038 unsigned char i; 00039 00040 //dlc_i2c_configure(GPS_DEVICE_ADDR, 100); 00041 //dlc_i2c_send_byte(GPS_UTC_DATE_TIME_ID); 00042 00043 cmd[0] = GPS_UTC_DATE_TIME_ID; 00044 i2c.write(GPS_DEVICE_ADDR, cmd, 1); 00045 00046 for(i=0;i<(GPS_UTC_DATE_TIME_SIZE+2);i++) 00047 { 00048 //data[i] = dlc_i2c_receive_byte(); 00049 i2c.read(GPS_DEVICE_ADDR, (char *)&data[i], 1); //the gps module's i2c supports only 1 byte read/write per burst 00050 } 00051 00052 for(i=0;i<GPS_UTC_DATE_TIME_SIZE;i++) 00053 gps_utc_date_time[i] = data[i+2]; 00054 00055 return gps_utc_date_time; 00056 } 00057 00058 unsigned char gps_get_status(void) 00059 { 00060 unsigned char data[GPS_STATUS_SIZE+2]; 00061 unsigned char i; 00062 00063 //dlc_i2c_configure(GPS_DEVICE_ADDR, 100); 00064 //dlc_i2c_send_byte(GPS_STATUS_ID); 00065 00066 cmd[0] = GPS_STATUS_ID; 00067 i2c.write(GPS_DEVICE_ADDR, cmd, 1); 00068 00069 for(i=0;i<(GPS_STATUS_SIZE+2);i++) 00070 { 00071 //data[i] = dlc_i2c_receive_byte(); 00072 i2c.read(GPS_DEVICE_ADDR, (char *)&data[i], 1); //the gps module's i2c supports only 1 byte read/write per burst 00073 } 00074 00075 return data[2]; 00076 } 00077 00078 float gps_get_latitude(void) 00079 { 00080 unsigned char data[GPS_LATITUDE_SIZE+2]; 00081 unsigned char i; 00082 00083 //dlc_i2c_configure(GPS_DEVICE_ADDR, 100); 00084 //dlc_i2c_send_byte(GPS_LATITUDE_ID); 00085 cmd[0] = GPS_LATITUDE_ID; 00086 i2c.write(GPS_DEVICE_ADDR, cmd, 1); 00087 00088 for(i=0;i<(GPS_LATITUDE_SIZE+2);i++) 00089 { 00090 i2c.read(GPS_DEVICE_ADDR, (char *)&data[i], 1); //the gps module's i2c supports only 1 byte read/write per burst 00091 } 00092 00093 return atof((const char *)&data[2]); 00094 } 00095 00096 unsigned char gps_get_ns(void) 00097 { 00098 unsigned char data[GPS_NS_SIZE+2]; 00099 unsigned char i; 00100 00101 //dlc_i2c_configure(GPS_DEVICE_ADDR, 100); 00102 //dlc_i2c_send_byte(GPS_NS_ID); 00103 cmd[0] = GPS_NS_ID; 00104 i2c.write(GPS_DEVICE_ADDR, cmd, 1); 00105 00106 for(i=0;i<(GPS_NS_SIZE+2);i++) 00107 { 00108 i2c.read(GPS_DEVICE_ADDR, (char *)&data[i], 1); //the gps module's i2c supports only 1 byte read/write per burst 00109 } 00110 00111 if(data[2] == 'N' || data[2] == 'S')return data[2]; 00112 else return data[2] = '-'; 00113 00114 } 00115 00116 float gps_get_longitude(void) 00117 { 00118 unsigned char data[GPS_LONGITUDE_SIZE+2]; 00119 unsigned char i; 00120 00121 //dlc_i2c_configure(GPS_DEVICE_ADDR, 100); 00122 //dlc_i2c_send_byte(GPS_LONGITUDE_ID); 00123 cmd[0] = GPS_LONGITUDE_ID; 00124 i2c.write(GPS_DEVICE_ADDR, cmd, 1); 00125 00126 for(i=0;i<(GPS_LONGITUDE_SIZE+2);i++) 00127 { 00128 i2c.read(GPS_DEVICE_ADDR, (char *)&data[i], 1); //the gps module's i2c supports only 1 byte read/write per burst 00129 } 00130 00131 return atof((const char *)&data[2]); 00132 } 00133 00134 unsigned char gps_get_ew(void) 00135 { 00136 unsigned char data[GPS_EW_SIZE+2]; 00137 unsigned char i; 00138 00139 //dlc_i2c_configure(GPS_DEVICE_ADDR, 100); 00140 //dlc_i2c_send_byte(GPS_EW_ID); 00141 cmd[0] = GPS_EW_ID; 00142 i2c.write(GPS_DEVICE_ADDR, cmd, 1); 00143 00144 for(i=0;i<(GPS_EW_SIZE+2);i++) 00145 { 00146 i2c.read(GPS_DEVICE_ADDR, (char *)&data[i], 1); //the gps module's i2c supports only 1 byte read/write per burst 00147 } 00148 00149 if(data[2] == 'E' || data[2] == 'W')return data[2]; 00150 else return data[2] = '-'; 00151 } 00152 00153 float gps_get_speed(void) 00154 { 00155 unsigned char data[GPS_SPEED_SIZE+2]; 00156 unsigned char i; 00157 00158 //dlc_i2c_configure(GPS_DEVICE_ADDR, 100); 00159 //dlc_i2c_send_byte(GPS_SPEED_ID); 00160 cmd[0] = GPS_SPEED_ID; 00161 i2c.write(GPS_DEVICE_ADDR, cmd, 1); 00162 00163 for(i=0;i<(GPS_SPEED_SIZE+2);i++) 00164 { 00165 i2c.read(GPS_DEVICE_ADDR, (char *)&data[i], 1); //the gps module's i2c supports only 1 byte read/write per burst 00166 } 00167 00168 return atof((const char *)&data[2]); 00169 } 00170 00171 float gps_get_course(void) 00172 { 00173 unsigned char data[GPS_COURSE_SIZE+2]; 00174 unsigned char i; 00175 00176 //dlc_i2c_configure(GPS_DEVICE_ADDR, 100); 00177 //dlc_i2c_send_byte(GPS_COURSE_ID); 00178 cmd[0] = GPS_COURSE_ID; 00179 i2c.write(GPS_DEVICE_ADDR, cmd, 1); 00180 00181 for(i=0;i<(GPS_COURSE_SIZE+2);i++) 00182 { 00183 i2c.read(GPS_DEVICE_ADDR, (char *)&data[i], 1); //the gps module's i2c supports only 1 byte read/write per burst 00184 } 00185 00186 return atof((const char *)&data[2]); 00187 } 00188 00189 unsigned char gps_get_position_fix(void) 00190 { 00191 unsigned char data[GPS_POSITION_FIX_SIZE+2]; 00192 unsigned char i; 00193 00194 //dlc_i2c_configure(GPS_DEVICE_ADDR, 100); 00195 //dlc_i2c_send_byte(GPS_POSITION_FIX_ID); 00196 cmd[0] = GPS_POSITION_FIX_ID; 00197 i2c.write(GPS_DEVICE_ADDR, cmd, 1); 00198 00199 for(i=0;i<(GPS_POSITION_FIX_SIZE+2);i++) 00200 { 00201 i2c.read(GPS_DEVICE_ADDR, (char *)&data[i], 1); //the gps module's i2c supports only 1 byte read/write per burst 00202 } 00203 00204 return data[2] - '0'; 00205 } 00206 00207 unsigned char gps_get_sate_used(void) 00208 { 00209 unsigned char data[GPS_SATE_USED_SIZE+2]; 00210 unsigned char i; 00211 unsigned char value; 00212 00213 //dlc_i2c_configure(GPS_DEVICE_ADDR, 100); 00214 //dlc_i2c_send_byte(GPS_SATE_USED_ID); 00215 cmd[0] = GPS_SATE_USED_ID; 00216 i2c.write(GPS_DEVICE_ADDR, cmd, 1); 00217 00218 for(i=0;i<(GPS_SATE_USED_SIZE+2);i++) 00219 { 00220 i2c.read(GPS_DEVICE_ADDR, (char *)&data[i], 1); //the gps module's i2c supports only 1 byte read/write per burst 00221 //dbg_serial.printf("data[%d]: %02x ", i, data[i]); 00222 } 00223 if(data[3] >= '0' && data[3] <= '9' )value = (data[3] - '0') * 10; 00224 else value = 0; 00225 if(data[2] >= '0' && data[2] <= '9' )value += (data[2] - '0'); 00226 else value += 0; 00227 00228 return value; 00229 } 00230 00231 float gps_get_altitude(void) 00232 { 00233 unsigned char data[GPS_ALTITUDE_SIZE+2]; 00234 unsigned char i; 00235 00236 //dlc_i2c_configure(GPS_DEVICE_ADDR, 100); 00237 //dlc_i2c_send_byte(GPS_ALTITUDE_ID); 00238 cmd[0] = GPS_ALTITUDE_ID; 00239 i2c.write(GPS_DEVICE_ADDR, cmd, 1); 00240 00241 for(i=0;i<(GPS_ALTITUDE_SIZE+2);i++) 00242 { 00243 i2c.read(GPS_DEVICE_ADDR, (char *)&data[i], 1); //the gps module's i2c supports only 1 byte read/write per burst 00244 } 00245 00246 return atof((const char *)&data[2]); 00247 } 00248 00249 char gps_get_mode(void) 00250 { 00251 char data[GPS_MODE_SIZE+2]; 00252 unsigned char i; 00253 00254 //dlc_i2c_configure(GPS_DEVICE_ADDR, 100); 00255 //dlc_i2c_send_byte(GPS_MODE_ID); 00256 cmd[0] = GPS_MODE_ID; 00257 i2c.write(GPS_DEVICE_ADDR, cmd, 1); 00258 00259 for(i=0;i<(GPS_MODE_SIZE+2);i++) 00260 { 00261 i2c.read(GPS_DEVICE_ADDR, (char *)&data[i], 1); //the gps module's i2c supports only 1 byte read/write per burst 00262 } 00263 00264 return data[2]; 00265 } 00266 00267 unsigned char gps_get_mode2(void) 00268 { 00269 unsigned char data[GPS_MODE2_SIZE+2]; 00270 unsigned char i; 00271 00272 //dlc_i2c_configure(GPS_DEVICE_ADDR, 100); 00273 //dlc_i2c_send_byte(GPS_MODE2_ID); 00274 cmd[0] = GPS_MODE2_ID; 00275 i2c.write(GPS_DEVICE_ADDR, cmd, 1); 00276 00277 for(i=0;i<(GPS_MODE2_SIZE+2);i++) 00278 { 00279 i2c.read(GPS_DEVICE_ADDR, (char *)&data[i], 1); //the gps module's i2c supports only 1 byte read/write per burst 00280 } 00281 00282 return data[2] - '0'; 00283 } 00284 00285 unsigned char gps_get_sate_in_veiw(void) 00286 { 00287 unsigned char data[GPS_SATE_IN_VIEW_SIZE+2]; 00288 unsigned char i; 00289 00290 //dlc_i2c_configure(GPS_DEVICE_ADDR, 100); 00291 00292 //dlc_i2c_send_byte(GPS_SATE_IN_VIEW_ID); 00293 cmd[0] = GPS_SATE_IN_VIEW_ID; 00294 i2c.write(GPS_DEVICE_ADDR, cmd, 1); 00295 00296 for(i=0;i<(GPS_SATE_IN_VIEW_SIZE+2);i++) 00297 { 00298 //data[i] = dlc_i2c_receive_byte(); 00299 i2c.read(GPS_DEVICE_ADDR, (char *)&data[i], 1); 00300 } 00301 00302 return data[2]; 00303 }
Generated on Tue Jul 19 2022 03:13:40 by
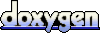