PN532 NFC library for Seeed Studio's NFC Shield
Fork of PN532 by
Embed:
(wiki syntax)
Show/hide line numbers
snep.cpp
00001 00002 #include "snep.h" 00003 #include "PN532_debug.h" 00004 00005 int8_t SNEP::write(const uint8_t *buf, uint8_t len, uint16_t timeout) 00006 { 00007 if (0 >= llcp.activate(timeout)) { 00008 DMSG("failed to activate PN532 as a target\n"); 00009 return -1; 00010 } 00011 00012 if (0 >= llcp.connect(timeout)) { 00013 DMSG("failed to set up a connection\n"); 00014 return -2; 00015 } 00016 00017 // response a success SNEP message 00018 headerBuf[0] = SNEP_DEFAULT_VERSION; 00019 headerBuf[1] = SNEP_REQUEST_PUT; 00020 headerBuf[2] = 0; 00021 headerBuf[3] = 0; 00022 headerBuf[4] = 0; 00023 headerBuf[5] = len; 00024 if (0 >= llcp.write(headerBuf, 6, buf, len)) { 00025 return -3; 00026 } 00027 00028 uint8_t rbuf[16]; 00029 if (6 > llcp.read(rbuf, sizeof(rbuf))) { 00030 return -4; 00031 } 00032 00033 // check SNEP version 00034 if (SNEP_DEFAULT_VERSION != rbuf[0]) { 00035 DMSG("The received SNEP message's major version is different\n"); 00036 // To-do: send Unsupported Version response 00037 return -4; 00038 } 00039 00040 // expect a put request 00041 if (SNEP_RESPONSE_SUCCESS != rbuf[1]) { 00042 DMSG("Expect a success response\n"); 00043 return -4; 00044 } 00045 00046 llcp.disconnect(timeout); 00047 00048 return 1; 00049 } 00050 00051 int16_t SNEP::read(uint8_t *buf, uint8_t len, uint16_t timeout) 00052 { 00053 if (0 >= llcp.activate(timeout)) { 00054 DMSG("failed to activate PN532 as a target\n"); 00055 return -1; 00056 } 00057 00058 if (0 >= llcp.waitForConnection(timeout)) { 00059 DMSG("failed to set up a connection\n"); 00060 return -2; 00061 } 00062 00063 uint16_t status = llcp.read(buf, len); 00064 if (6 > status) { 00065 return -3; 00066 } 00067 00068 00069 // check SNEP version 00070 if (SNEP_DEFAULT_VERSION != buf[0]) { 00071 DMSG("The received SNEP message's major version is different\n"); 00072 // To-do: send Unsupported Version response 00073 return -4; 00074 } 00075 00076 // expect a put request 00077 if (SNEP_REQUEST_PUT != buf[1]) { 00078 DMSG("Expect a put request\n"); 00079 return -4; 00080 } 00081 00082 // check message's length 00083 uint32_t length = (buf[2] << 24) + (buf[3] << 16) + (buf[4] << 8) + buf[5]; 00084 // length should not be more than 244 (header + body < 255, header = 6 + 3 + 2) 00085 if (length > (status - 6)) { 00086 DMSG("The SNEP message is too large: "); 00087 DMSG_INT(length); 00088 DMSG_INT(status - 6); 00089 DMSG("\n"); 00090 return -4; 00091 } 00092 for (uint8_t i = 0; i < length; i++) { 00093 buf[i] = buf[i + 6]; 00094 } 00095 00096 // response a success SNEP message 00097 headerBuf[0] = SNEP_DEFAULT_VERSION; 00098 headerBuf[1] = SNEP_RESPONSE_SUCCESS; 00099 headerBuf[2] = 0; 00100 headerBuf[3] = 0; 00101 headerBuf[4] = 0; 00102 headerBuf[5] = 0; 00103 llcp.write(headerBuf, 6); 00104 00105 return length; 00106 }
Generated on Tue Jul 12 2022 17:32:26 by
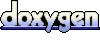