PN532 NFC library for Seeed Studio's NFC Shield
Fork of PN532 by
Embed:
(wiki syntax)
Show/hide line numbers
emulatetag.h
Go to the documentation of this file.
00001 /**************************************************************************/ 00002 /*! 00003 @file emulatetag.h 00004 @author Armin Wieser 00005 @license BSD 00006 00007 Implemented using NFC forum documents & library of libnfc 00008 */ 00009 /**************************************************************************/ 00010 00011 00012 #ifndef __EMULATETAG_H__ 00013 #define __EMULATETAG_H__ 00014 00015 #include "PN532.h " 00016 00017 #define NDEF_MAX_LENGTH 128 // altough ndef can handle up to 0xfffe in size, arduino cannot. 00018 typedef enum {COMMAND_COMPLETE, TAG_NOT_FOUND, FUNCTION_NOT_SUPPORTED, MEMORY_FAILURE, END_OF_FILE_BEFORE_REACHED_LE_BYTES} responseCommand; 00019 00020 class EmulateTag{ 00021 00022 public: 00023 EmulateTag(PN532Interface &interface) : pn532(interface), uidPtr(0), tagWrittenByInitiator(false) { } 00024 00025 bool init(); 00026 00027 bool emulate(const uint16_t tgInitAsTargetTimeout = 0); 00028 00029 /* 00030 * @param uid pointer to byte array of length 3 (uid is 4 bytes - first byte is fixed) or zero for uid 00031 */ 00032 void setUid(uint8_t* uid = 0); 00033 00034 void setNdefFile(const uint8_t* ndef, const int16_t ndefLength); 00035 00036 void getContent(uint8_t** buf, uint16_t* length){ 00037 *buf = ndef_file + 2; // first 2 bytes = length 00038 *length = (ndef_file[1] << 8) + ndef_file[0]; 00039 } 00040 00041 bool writeOccured(){ 00042 return tagWrittenByInitiator; 00043 } 00044 00045 private: 00046 PN532 pn532; 00047 uint8_t ndef_file[NDEF_MAX_LENGTH]; 00048 uint8_t* uidPtr; 00049 void setResponse(responseCommand cmd, uint8_t* buf, uint8_t* sendlen, uint8_t sendlenOffset =0); 00050 bool tagWrittenByInitiator; 00051 }; 00052 00053 #endif
Generated on Tue Jul 12 2022 17:32:26 by
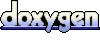