library for Bluetooth Shield from Seeed Studio
Fork of BluetoothSerial by
BluetoothSerial.cpp
00001 00002 #include "BluetoothSerial.h" 00003 #include <string.h> 00004 00005 #define LOG(args...) // std::printf(args) 00006 00007 BluetoothSerial::BluetoothSerial(PinName tx, PinName rx) : _serial(tx, rx) 00008 { 00009 00010 } 00011 00012 void BluetoothSerial::setup() 00013 { 00014 _serial.baud(BLUETOOTH_SERIAL_DEFAULT_BAUD); 00015 } 00016 00017 00018 void BluetoothSerial::master(const char *name, uint8_t autoc) 00019 { 00020 _serial.puts("\r\n+STWMOD=1\r\n"); 00021 _serial.printf("\r\n+STNA=%s\r\n", name); 00022 _serial.printf("\r\n+STAUTO=%d\r\n", autoc ? 1 : 0); 00023 } 00024 00025 00026 void BluetoothSerial::slave(const char *name, uint8_t autoc, uint8_t oaut) 00027 { 00028 _serial.puts("\r\n+STWMOD=0\r\n"); 00029 _serial.printf("\r\n+STNA=%s\r\n", name); 00030 _serial.printf("\r\n+STOAUT=%d\r\n", oaut ? 1 : 0); 00031 _serial.printf("\r\n+STAUTO=%d\r\n", autoc ? 1 : 0); 00032 } 00033 00034 int BluetoothSerial::connect() 00035 { 00036 clear(); 00037 _serial.puts("\r\n+INQ=1\r\n"); // Make the bluetooth module inquirable 00038 LOG("BT: INQUIRING\r\n"); 00039 00040 const char *prefix = "CONNECT:"; 00041 uint8_t prefix_len = sizeof("CONNECT:") - 1; 00042 for (uint8_t i = 0; i < 12; i++) { 00043 int len = readline(_buf, sizeof(_buf)); 00044 if (len > 0) { 00045 LOG("%s\r\n", _buf); 00046 if (!memcmp(_buf, prefix, prefix_len)) { // check prefix 00047 const char *suffix = "OK"; 00048 uint8_t suffix_len = sizeof("OK") - 1; 00049 00050 if (!memcmp(_buf + prefix_len, suffix, suffix_len)) { // check suffix 00051 LOG("BT: CONNECTED\r\n"); 00052 return 1; 00053 } 00054 00055 suffix = "FAIL"; 00056 suffix_len = sizeof("FAIL") - 1; 00057 00058 if (!memcmp(_buf + prefix_len, suffix, suffix_len)) { // check suffix 00059 return 0; 00060 } 00061 } 00062 } 00063 } 00064 00065 return 0; 00066 } 00067 00068 int BluetoothSerial::connect(const char *name) 00069 { 00070 char *mac; 00071 int name_len = strlen(name); 00072 00073 clear(); 00074 _serial.puts("\r\n+INQ=1\r\n"); 00075 LOG("BT: INQUERING\r\n"); 00076 while (1) { 00077 int len = readline(_buf, sizeof(_buf)); // +RTINQ=XX,XX,X,X,X,X;DEVICE_NAME 00078 if (len > 0) { 00079 LOG("%s\r\n", _buf); 00080 if (!memcmp(_buf, "+RTINQ=", sizeof("+RTINQ=") - 1)) { // check prefix 00081 00082 if (!memcmp(_buf + len - name_len, name, name_len)) { // check suffix 00083 _buf[len - name_len - 1] = '\0'; 00084 mac = (char*)_buf + sizeof("+RTINQ=") - 1; 00085 LOG("Connecting device: %s\r\n", mac); 00086 00087 break; 00088 } 00089 } 00090 00091 } 00092 00093 } 00094 00095 LOG("BT: CONNECTING\r\n"); 00096 _serial.printf("\r\n+CONN=%s\r\n", mac); 00097 00098 const char *prefix = "CONNECT:"; 00099 int prefix_len = sizeof("CONNECT:") - 1; 00100 for (uint8_t i = 0; i < 6; i++) { 00101 int len = readline(_buf, sizeof(_buf), 0); 00102 if (len >= 0) { 00103 LOG("%s\r\n", _buf); 00104 if (!memcmp(_buf, prefix, prefix_len)) { // check prefix 00105 const char *suffix = "OK"; 00106 uint8_t suffix_len = sizeof("OK") - 1; 00107 00108 if (!memcmp(_buf + prefix_len, suffix, suffix_len)) { // check suffix 00109 LOG("BT: CONNECTED\r\n"); 00110 return 1; 00111 } 00112 00113 suffix = "FAIL"; 00114 suffix_len = sizeof("FAIL") - 1; 00115 00116 if (!memcmp(_buf + prefix_len, suffix, suffix_len)) { // check suffix 00117 LOG("TB: CONNECTION FAILED\r\n"); 00118 return 0; 00119 } 00120 } 00121 } 00122 } 00123 00124 return 0; 00125 } 00126 00127 00128 int BluetoothSerial::_getc() 00129 { 00130 return _serial.getc(); 00131 } 00132 00133 int BluetoothSerial::_putc(int c) 00134 { 00135 return _serial.putc(c); 00136 } 00137 00138 int BluetoothSerial::readline(uint8_t *buf, int len, uint32_t timeout) 00139 { 00140 int get = 0; 00141 int count = timeout; 00142 while (count >= 0) { 00143 if (_serial.readable()) { 00144 char c = _serial.getc(); 00145 buf[get] = c; 00146 if (c == '\n' && get && buf[get - 1] == '\r') { 00147 buf[get - 1] = '\0'; 00148 return get - 1; 00149 } 00150 get++; 00151 if (get >= len) { 00152 LOG("Too long line, the buffer is not enough\r\n"); 00153 return -(get + 1); 00154 } 00155 00156 count = timeout; 00157 } 00158 00159 if (timeout != 0) { 00160 count--; 00161 } 00162 } 00163 00164 return -(get + 1); 00165 } 00166 00167 void BluetoothSerial::clear() 00168 { 00169 int count = 0; 00170 00171 LOG("Clear previous command output\r\n"); 00172 do { 00173 count++; 00174 if (_serial.readable()) { 00175 int get = _serial.getc(); 00176 count = 0; 00177 00178 LOG("%c", get); 00179 } 00180 } while (count < BLUETOOTH_SERIAL_TIMEOUT); 00181 LOG("done\r\n"); 00182 } 00183 00184 00185
Generated on Sat Jul 16 2022 00:15:55 by
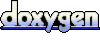